第二章 JavaScript案例(中)
1. js事件
HTML代码
<!DOCTYPE html>
<html lang="en" onUnload="ud()">
<head>
<meta charset="UTF-8">
<title>Title</title>
<script>
function demo() {
alert("hello");
}
function onOver(ooj) {
ooj.innerHTML = "鼠标移入显示";
}
function onOut(ooj) {
ooj.innerHTML = "鼠标移出显示";
}
function changeDemo(bg) {
alert("内容改变了啊")
}
function changeDemo1(bg) {
bg.style.background = "red";
}
function changeDemo2(bg) {
bg.style.background = "blue";
}
function mgs() {
alert("内容加载完毕")
}
function bu(bg) {
var b = bg.value;
alert("鼠标移开事件(您输入的是)" + b);
}
function ud() {
alert("您关闭了网页")
}
</script>
<style>
.div {
width: 100px;
height: 100px;
background-color: cadetblue;
}
</style>
</head>
<body onload="mgs()" onunload="ud()">
<!--网页加载完毕事件,网页关闭事件(关闭后执行看不见)-->
<button onclick="demo()">按钮</button>
<!--单机事件-->
<div class="div" onmouseout="onOut(this)" onmouseover="onOver(this)">开始显示</div>
<form>
<input type="text" onchange="changeDemo(this)"/>
<!--文本内容改变事件-->
<input type="text" onselect="changeDemo1(this)" onfocus="changeDemo2(this)"/>
<!--文本内容选中事件--> <!--鼠标聚集(选中)事件-->
<input type="text" onblur="bu(this)"/>
<!--移开光标事件事件-->
</form>
</body>
</html>
2. js异常
试一试不输入
HTML代码
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>异常处理</title>
<script>
function demo() {
try {
alert(str);//str为空
}catch(err){
alert(err);
}
}
demo();
function demo1(){
var e = document.getElementById("txt").value;
try{
if(e==""){
throw "您没有输入文字";//自定义异常
}
}catch(err){
alert(err);
}
}
</script>
</head>
<body>
<form>
<input type="text" id="txt">
<input type="button" onclick="demo1()" value="按钮">
</form>
</body>
</html>
3. js条件语句
HTML代码
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>语句</title>
<script>
var i = 9;//修改i值
if (i > 10) {
document.write("我大于10"+"</br>");
} else if (i < 10) {
document.write("我小于10"+"</br>");
} else {
document.write("我等于十"+"</br>")
}
</script>
<script>
var i = 5;//修改i值
switch (i) {
case 1:
document.write("i=1")
break;
case 2:
document.write("i=2")
break;
default:
document.write("i=" + i+"</br>");
break;
}
// 跳转语句break(跳出循环——结束循环) continue(跳过当次循环) return(返回一个值,结束)
</script>
<script>
for(var i=0;i<10;i++){
if(i==5){
break;
// continue;
}
document.write("i="+i+"</br>");
}
</script>
<script>
var i = [1,2,3,4,5,6];
var j;
for(j in i){//如果j是i中的一个元素
document.write(i[j]+"</br>");
}
</script>
<script>
var i = 1;
while(i<10){
document.write("i="+i+"</br>");
i++;
}
document.write("</br></br></br></br>")
</script>
<script>
var i = 10;
do{//会执行一次do里面的语句在判断
document.write(i+"</br>");
}while(i<2){
document.write("i="+i+"</br>");
i++;
}
</script>
</head>
<body>
</body>
</html>
4. js句柄监听事件
效果是打印hello
HTML代码
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>句柄添加监听事件</title>
<script type="text/javascript" src="tzy.js"></script>
</head>
<body>
<button id="mybtn" onclick="demo()">按钮</button>
<script>
//注意这种引用样式必须放在button后面不然出现
//Uncaught TypeError: Cannot read property 'addEventListener' of null
//错误:页面未加载完成
var x = document.getElementById("mybtn");
x.addEventListener("click", hello);
x.addEventListener("click", world);//添加
x.removeEventListener("click", world);//移除
function hello() {
alert("hello");
}
function world() {
alert("world");
}
</script>
</body>
</html>
JS代码(tzy.js)
注释上面中间的JS代码,放入外文件,用外链接方式引用
//注意这种引用样式必须放在button后面不然出现
//Uncaught TypeError: Cannot read property 'addEventListener' of null
//错误:页面未加载完成
function demo(){
var x = document.getElementById("mybtn");
x.addEventListener("click", hello);
x.addEventListener("click", world);//添加
x.removeEventListener("click", world);//移除
}
function hello() {
alert("hello");
}
function world() {
alert("world");
}
5. jsDOM
HTML代码
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>JsDOM对象</title>
<script type="text/javascript" src="tzy.js"></script>
</head>
<body>
<p name = "pn">hello</p>
<p name = "pn">hello</p>
<p name = "pn">hello</p>
<p name = "pn">hello</p>
<a id = "aid" title = "title属性"></a>
<ul><li id="li1">1</li><li>2 3</li><li>3</li></ul>
<div id="div">
<p id="pid">我是p节点</p>
<p id="pid1">我是p1节点</p>
</div>
<script>
function getName() {
var count = document.getElementsByName("pn");//根据name获取
alert("count长度"+count.length);//看是否获取到
var p = count[2];
p.innerHTML = "world";
var count1 = document.getElementsByTagName("p");//根据标签名获取
alert("count1长度"+count1.length);
var p1 = count1[3];
p1.innerHTML = "hahaha";
}
getName();
function getSetAttr() {
var a = document.getElementById("aid");//根据id获取
var attr = a.getAttribute("title");//获取当前元素某个属性值
alert(attr);
a.setAttribute("id","动态被设置为id")//设置当前元素某个属性值
var attr1 =a.getAttribute("id");
alert(attr1);
}
getSetAttr();
function getChildNode(){
var childnode = document.getElementsByTagName("ul")[0].childNodes;//获取子节点项,注意ul里面空格也会算子节点,所以要去掉空格
alert("ul的字节点数"+childnode.length);
alert("ul里的第一个子节点类型"+childnode[0].nodeType);//有疑问
alert("ul里的第二个子节点类型"+childnode[1].nodeType);
}
getChildNode();
function getParentNode() {
var li1 = document.getElementById("li1");
alert("li1的父节点"+li1.parentNode.nodeName);//获取父节点及名字
}
getParentNode();
function createNode(){
var body = document.body;//获取body
var input = document.createElement("input");//创建节点
input.type = "button";
input.value = "自建按钮";
body.appendChild(input);//将节点添加到body中
//createTextNode()添加文本节点
}
createNode();
function addNode() {
var div = document.getElementById("div");
var node = document.getElementById("pid");
var newnode = document.createElement("p");
newnode.innerHTML = "这是我添加的p节点";
div.insertBefore(newnode,node);//添加节点到某个节点前
}
addNode();
function removeNode() {
var div = document.getElementById("div");
var p = div.removeChild(div.childNodes[2]);//删除p节点因为空格算一个节点
}
removeNode();
function getSize(){
//offsetheight 网页尺寸(不包含滚动条)
//scrollheight 网页尺寸(包含滚动条)
var height = document.body.offsetHeight||document.documentElement.offsetHeight;//兼容性写法
var width = document.body.offsetWidth;
alert("宽度"+width+"高度"+height);
}
getSize();
</script>
</body>
</html>
6. js计时器与倒计时
HTML代码
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>倒计时</title>
</head>
<body onload="getwin()">
<p id="ptime">现在时间</p>
<p id="ptime1">距离2018年时间</p>
<button id="btn" onclick="stopTime()">停止时间按钮</button>
<button id="btn1" onclick="stopwin()">停止弹出框按钮</button>
<script>
var mytime = setInterval(function(){getTime();},1000);//间隔一秒执行一次该方法
function getTime() {
var nowtime = new Date();//获取当前时间
var time = nowtime.toLocaleTimeString();//转换日期十分秒
var endTime=new Date("2018/1/1,0:00:00") //设定倒计时结束时间
var timeXX=(endTime.getTime()-nowtime.getTime())/(1000);//距离2018年的秒数
var date=parseInt(timeXX/(24*60*60)); //换算成天
var xs=parseInt(timeXX/(60*60)%24); //得到小时
var fz=parseInt(timeXX/60%60); //得到分钟
var ms=parseInt(timeXX%60); //得到秒数
var year=nowtime.getFullYear(); //获取现在的年
var month=nowtime.getMonth()+1; //获取现在的月 必须+1
var data=nowtime.getDate(); //获取现在的日
var h=nowtime.getHours();//获取现在的小时
var m=nowtime.getMinutes();//获取现在的分钟
var s=nowtime.getSeconds();//获取现在的秒数
h=checkTime(h);
m=checkTime(m);
s=checkTime(s);
var d=nowtime.getDay(); //获取星期(因星期打印出事0~6,所以用数组形式转化成对应的星期)
var weekday=['星期日','星期一','星期二','星期三','星期四','星期五','星期六'];
document.getElementById("ptime").innerHTML=year+"年"+month+"月"+data+"日"+" "+weekday[d]+" "+h+":"+m+":"+s;
//打印出当前年月日
document.getElementById('ptime1').innerHTML='离2018年:'+date+'天'+xs+'小时'+fz+'分钟'+ms+'秒';
}
function getwin() {
alert("我是3秒一个的弹出框");
win = setTimeout(function(){getwin();},3000);//延迟3秒出现一个提示框
}
function stopTime() {
clearInterval(mytime);
}
function stopwin() {
clearTimeout(win);
}
function checkTime(i){
//设置小于10的时间数字格式,例如5秒显示成05秒
if(i<10){
return '0'+i;
}else{
return i;
}
}
</script>
</body>
</html>
7. jsBOM
HTML代码
<!doctype html>
<html lang="en">
<head>
<title>day02_js</title>
<script type="text/javascript" language="javascript" src="tzy.js"></script>
<meta charset="UTF-8">
</head>
<body>
<form>
<h2>1.BOM演示针对window</h2>
<input type="button" onclick="testConfirm()" value="confirm测试"/><br/><br/>
<input type="button" onclick="testPromp()" value="promp测试"/><br/><br/>
<input type="button" onclick="openTest()" value="open测试"/><br/><br/>
<input type="button" onclick="closeTest()" value="close测试"/><br/><br/>
<input type="button" onclick="refreshTest()" value="refresh测试"/><br/><br/>
<input/><br/><br/>
<input type="button" onclick="naviTest()" value="控制台查看信息测试"/><br/><br/>
<input type="button" onclick="TimeTest()" value="计时开始"/>
<input type="button" onclick="clearTime()" value="计时结束"/>
<input id="i1"/><br/><br/>
</form>
</body>
</html>
JS代码(tzy.js)
function testConfirm(){
var f = window.confirm("你确定此操作吗?"); //确定加取消的弹出框 参数自定义,返回值为点击确定=true,点击取消=false;
alert(f); //打印返回的boolean值
}
function testPromp(){
var f = window.prompt("请输入用户名","周杰伦"); //提示语加text加确定加取消弹出框 第一个参数提示语,第二个参数默认值
alert(f); //打印输入框中的值 点即取消则返回null
}
function openTest(){
//window.open("http://www.baidu.com");//打开一个新页面
var config = "toolbar=yes,width=500,height=300"; //toolbar显示工具栏
window.open("http://www.baidu.com","1",config) // 参数1为自定义,否则不能按照config的大小打开新的窗口
}
function closeTest(){
window.close(); //关闭当前页面
}
function refreshTest(){
window.location.reload(); //页面刷新
}
function naviTest(){
var arr = new Array(5);
console.dir(arr); //console.dir()可以在控制台显示一个对象所有的属性和方法。
//console.log(window.navigator.appVersion); //会在浏览器控制台打印出信息。 查看当前浏览的版本
//console.dir(window.navigator.appVersion);
}
var timer; //window定时器
function TimeTest(){
timer = window.setInterval('func()',100);
}
var count = 0;
function func(){
count++;
document.getElementById("i1").value= count;
}
function clearTime(){
window.clearInterval(timer);
}
补充:DOM操作
案例一
HTML代码
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<link rel="stylesheet" href="tzy.css" type="text/css">
<script type="text/javascript" src="tzy.js" language="JavaScript"></script>
</head>
<body>
<div id="div"><b id="b">我是一只小小鸟</b></div>
<form>
<input type="button" value="随机改变字体颜色按钮" onclick="gbzt()">
<input type="button" value="随机改变背景颜色按钮" onclick="gbbj()"></br>
<input type="text" id="text">
内容<input type="button" value="改变内容" onclick="gbtext()"></br>
宽度<input type="text" id="gbwid">px
<input type="button" value="改变背景宽度" onclick="gbwidth()"></br>
高度<input type="text" id="gbhei">px
<input type="button" value="改变背景高度" onclick="gbheight()"></br>
<input type="reset" value="清空输入框"></br>
<input type="button" value="字体隐藏" onclick="ycb()">
<input type="button" value="全部隐藏" onclick="ycdiv()"></br>
<input type="button" value="字体显示" onclick="xsb()">
<input type="button" value="全部显示" onclick="xsdiv()"></br>
<input type="button" value="是否重置" onclick="firm()">
</form>
</body>
</html>
JS代码(tzy.js)
var chars = ['0','1','2','3','4','5','6','7','8','9','A','B','C','D','E','F'];
var color;
function randomColor() {
color="";
for(var i=0;i<6;i++){
var count = Math.floor(Math.random()*16);
color += chars[count];
}
}
function gbzt() {
randomColor();
document.getElementById("div").style.color="#"+color;
}
function gbbj() {
randomColor();
document.getElementById("div").style.backgroundColor="#"+color;
}
function gbwidth() {
var val = document.getElementById("gbwid").value;
if(isNaN(val)||val==""){
alert("必须输入数字,不能为空")
}else{
document.getElementById("div").style.width=val+'px';
}
}
function gbheight() {
var val = document.getElementById("gbhei").value;
if(isNaN(val)||val==""){
alert("必须输入数字,不能为空")
}else{
document.getElementById("div").style.height=val+'px';
}
}
function gbtext() {
var val = document.getElementById("text").value;
document.getElementById("b").innerText = val;
}
function ycb() {
document.getElementById("b").style.display = "none";
}
function ycdiv() {
document.getElementById("div").style.display = "none";
}
function xsb() {
document.getElementById("b").style.display = "block";
}
function xsdiv() {
document.getElementById("div").style.display = "block";
document.getElementById("b").style.display = "block"
}
function firm(){
var yesorno = window.confirm("您确定取消所有设置么?")
if(yesorno==true){
window.location.replace("tzy1.html");
}else{
alert("没事瞎点什么!!!")
}
}
css代码(tzy.css)
div{
color: forestgreen;
background-color: coral;
width: 100px;
height: 100px;
}
案例二
HTML代码
<!doctype html>
<html lang="en">
<head>
<title>day03</title>
<meta charset="UTF-8">
<script type="text/javascript" language="javascript" src="tzy.js"></script>
<style>
table{
border-collapse:collapse;
}
td{
border:1px solid gray;
}
tr input{
width:25px;
}
</style>
</head>
<body onload="testNode()">
<form>
<h2>表单的验证与提交</h2>
管理员账号:<input id="account" class="txt" onfocus="this.className='txt_focus';" onblur="valiAcc(this)"/>
<span id="accountInfo">10个长度以内的字母、数字的组合</span><br/>
电话号码:<input id="phone" class="txt" onfocus="this.className='txt_focus';" onblur="valiPhone(this)"/>
<span id="phoneInfo">形如:010-12345678</span><br/><br/>
<input type="submit" value="保存" onclick="alert(valiAll())" />
<h2>节点查询展示</h2>
<div id="div01">
<p id="p01">我是段落1</p>
<p id="p02">我是段落2</p>
<input type="radio" name="sex"/>男
<input type="radio" name="sex"/>女
</div>
<input type="radio" name="sex"/>无
<h2>购物车</h2>
<table id="shoppingCar">
<tr>
<td style="width:100px;">商品名称</td>
<td style="width:100px;">单价</td>
<td style="width:200px;">数量</td>
<td style="width:100px;">小计</td>
</tr>
<tr>
<td style="width:100px;">book1</td>
<td style="width:100px;">10.0</td>
<td style="width:200px;">
<input type="button" value="-" onclick="decrease(this)"/>
<input type="text" value="1" readonly="readonly"/>
<input type="button" value="+" onclick="increase(this)"/>
</td>
<td style="width:100px;">10.0</td>
</tr>
<tr>
<td style="width:100px;">book2</td>
<td style="width:100px;">5.0</td>
<td style="width:200px;">
<input type="button" value="-" onclick="decrease(this)"/>
<input type="text" value="1" readonly="readonly"/>
<input type="button" value="+" onclick="increase(this)"/>
</td>
<td style="width:100px;">5.0</td>
</tr>
<tr>
<td style="width:100px;">book3</td>
<td style="width:100px;">15.0</td>
<td style="width:200px;">
<input type="button" value="-" onclick="decrease(this)"/>
<input type="text" value="1" readonly="readonly"/>
<input type="button" value="+" onclick="increase(this)"/>
</td>
<td style="width:100px;">15.0</td>
</tr>
<tr>
<td colspan="3" style="text-align:right" >总计:</td>
<td>30.0</td>
</tr>
</table>
<h2>节点增加展示</h2>
<div id="div02">
<p>我是第1个段落</p>
</div>
<input type="button" value="增加段落" onclick="addNode()"/>
<input type="button" value="首行增加段落" onclick="addNode2()"/>
<input type="button" value="删除段落" onclick="deleteNode()"/>
<h2>动态创建页面元素</h2>
<input type="button" onclick="productNode(this)" value="添加新元素"/>
</form>
</body>
</html>
JS代码(tzy.js)
function valiAcc(obj){
obj.className= "txt";
var account = obj.value;
var regex = /^[0-9a-zA-Z]{1,10}$/g;
var flag = regex.test(account);
//判断
if(flag){
document.getElementById("accountInfo").className="vali_success";
document.getElementById("accountInfo").innerText="";
}else{
document.getElementById("accountInfo").className="vali_fail";
document.getElementById("accountInfo").innerText="10个长度以内的字母、数字组合";
}
return flag;
}
function valiPhone(obj){
obj.className= "txt";
var account = obj.value;
var regex = /^\d{3}-\d{8}$/g;
var flag = regex.test(account);
//判断
if(flag){
document.getElementById("phoneInfo").className="vali_success";
document.getElementById("phoneInfo").innerText="";
}else{
document.getElementById("phoneInfo").className="vali_fail";
document.getElementById("phoneInfo").innerText="形如:010-12345678";
}
return flag;
}
function valiAll(){
var acc = document.getElementById("account");
var phone = document.getElementById("phone");
return valiAcc(acc) && valiPhone(phone);
}
function testNode(){
var divObj = document.getElementById("div01");
var parentNode = divObj.parentNode;
//console.log(parentNode.nodeName+","+parentNode.nodeType);
var nodeArr = divObj.childNodes;
for(var i = 0;i<nodeArr.length;i++){
//console.log(nodeArr[i].nodeName+","+nodeArr[i].nodeType);
}
var p02 = document.getElementById("p02");
var p01 = p02.previousSibling.previousSibling;
//console.log(p01.nodeName+","+p01.nodeType);
var sexArr = document.getElementsByName("sex");
//console.log(sexArr.length);
var inputArr = document.getElementsByTagName("input");
var inputAr= divObj.getElementsByTagName("input");
console.log(inputAr.length);
}
//购物车减
function decrease(obj){
var txtObj = obj.nextSibling.nextSibling; //nextSibling 获取下一个节点
if(txtObj.value>0){
txtObj.value--;
}
cal();
}
//购物车加
function increase(obj){
var txtObj = obj.previousSibling.previousSibling; //previousSibling 获取上一个节点
txtObj.value++;
cal();
}
function cal(){
var tbObj = document.getElementById("shoppingCar"); //获取table对象
var trArr = tbObj.getElementsByTagName("tr"); //获取所有的行对象
//定一个计数器,用存储总价值
var totalPrice = 0;
for(var i=1;i<trArr.length-1;i++){
//获取当前行对象
var trObj = trArr[i];
//获取单价
var price = trObj.getElementsByTagName("td")[1].innerText;
//获取数量
var num = trObj.getElementsByTagName("input")[1].value;
//当前商品的小计
var xj = price*num;
//给小计栏赋值
var tdArr = trObj.getElementsByTagName("td");
tdArr[tdArr.length-1].innerText = xj.toFixed(2);
totalPrice += xj;
}
trArr[trArr.length-1].lastChild.previousSibling.innerText = totalPrice.toFixed(2);
}
var n = 1;
function addNode(){
n++;
var divObj = document.getElementById("div02");
var pObj = document.createElement("p"); // <p></p>
pObj.innerText="我是第"+n+"个段落";
divObj.appendChild(pObj);
}
function addNode2(){
var divObj = document.getElementById("div02");
var pObj = document.createElement("p");
pObj.innerText="我是第"+0+"个段落";
divObj.insertBefore(pObj,divObj.getElementsByTagName("p")[0]);
}
function deleteNode(){
if(n>0){
n--;
}
var divObj = document.getElementById("div02");
var pArr = divObj.getElementsByTagName("p");
if(pArr.length>0){
divObj.removeChild(pArr[pArr.length-1]);
}
}
function productNode(obj){
var newButton = document.createElement("input"); //<input></input>
newButton.type="button";
newButton.value="New Button";
newButton.onclick=function(){
alert("hello");
}
obj.parentNode.insertBefore(newButton,obj);
var aObj=document.createElement("a");
aObj.innerHTML="to百度";
aObj.target="_blank";
aObj.href="http://www.baidu.com";
obj.parentNode.appendChild(aObj);
}
双色球案例
HTML代码
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>双色球生成器</title>
</head>
<body>
<div style="margin: 0 auto;padding: 0px;background-color: cadetblue;text-align: center;width:auto;height: auto">
<h1>双色球生成器</h1>
<form>
<input type="button" value="机选按钮" onclick="random()"/>
</form>
</div>
<script>
function random() {
var array = new Array(7);
for(var i=0;i<array.length;i++){
array[i] = Math.ceil(Math.random()*33);//向上转型
if(i===6){
array[i] = Math.ceil(Math.random()*16)
}
}
for(var i=0;i<array.length-1;i++){
for(var j=0;j<array.length-2;j++){
if(array[j]>array[j+1]){
a = array[j];
array[j] = array[j+1];
array[j+1] = a;
}
}
}
alert("第1个红球:"+array[0]+"\n"+"第2个红球:"+array[1]+"\n"+"第3个红球:"+array[2]+"\n"+
"第4个红球:"+array[3]+"\n"+"第5个红球:"+array[4]+"\n"+"第6个红球:"+array[5]+"\n"+"蓝球:| "+array[6]);
}
</script>
</body>
</html>
轮播图案例
HTML代码
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>广告轮播</title>
<script type="text/javascript" src="tzy.js" language="JavaScript"></script>
<link rel="stylesheet" href="tzy.css" type="text/css">
</head>
<body onload="lunbozantin()">
<div>
<p><b>我是轮播图片</b></p>
<img style="width: 508px;height: 299px;" src="http://images2018.cnblogs.com/blog/1235870/201808/1235870-20180823110552195-398576301.png" alt="lol图片" id="tupian" onMouseOver="stoplunbo()" onMouseOut="lunbozantin()">
</div>
</body>
</html>
JS代码(tzy.js)
var arr = new Array("http://images2018.cnblogs.com/blog/1235870/201808/1235870-20180823110552195-398576301.png","http://images2018.cnblogs.com/blog/1235870/201808/1235870-20180823110608817-462634665.png","http://images2018.cnblogs.com/blog/1235870/201808/1235870-20180823110619409-2102515392.png","http://images2018.cnblogs.com/blog/1235870/201808/1235870-20180823110628900-1106270637.png");
var count = 1;
var b;
function lunbo() {
a = document.getElementById("tupian");
a.src =arr[count];
count++;
if(count==4){
count=0;
}
}
function stoplunbo(){
clearInterval(b);
}function lunbozantin() {
b = setInterval(lunbo,3000);
}
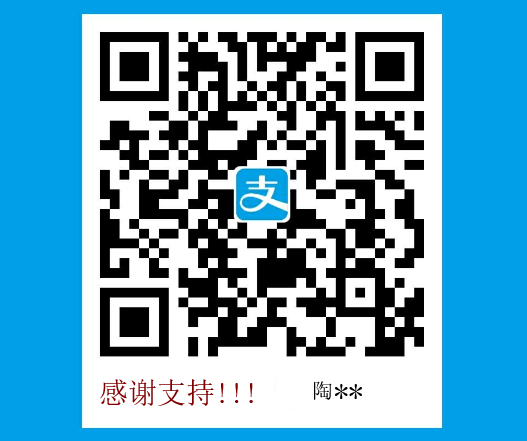
您的资助是我最大的动力!
金额随意,欢迎来赏!