1.题目简述
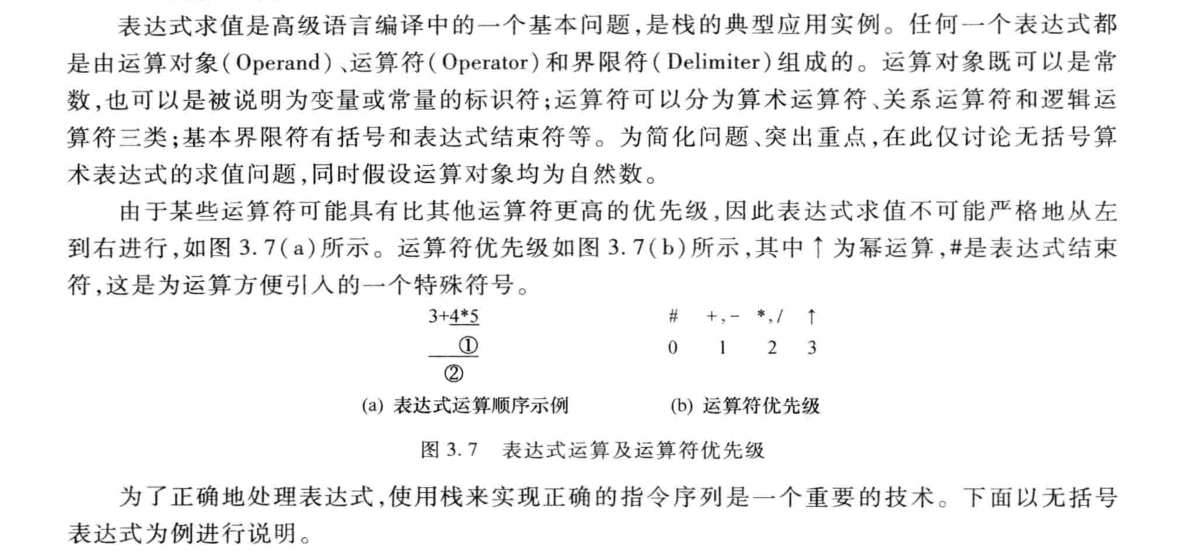
2.算法思路
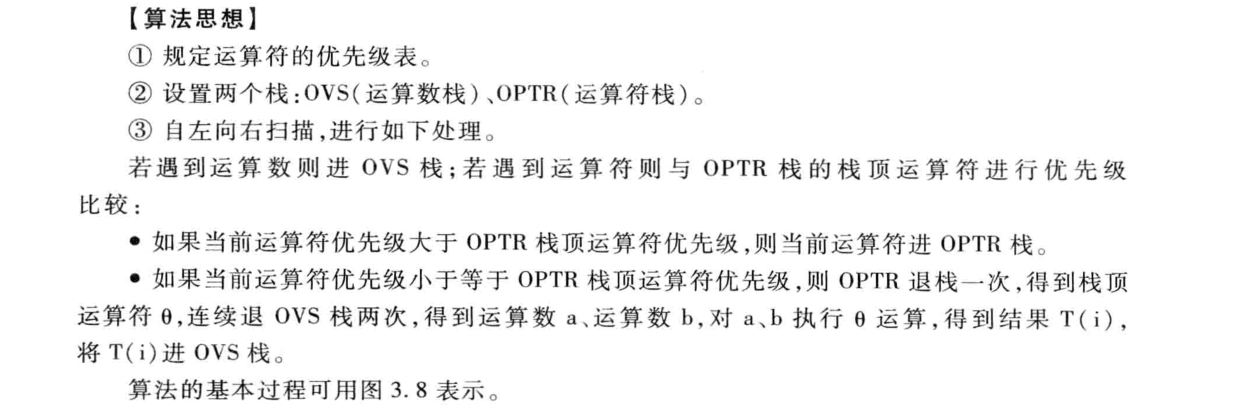
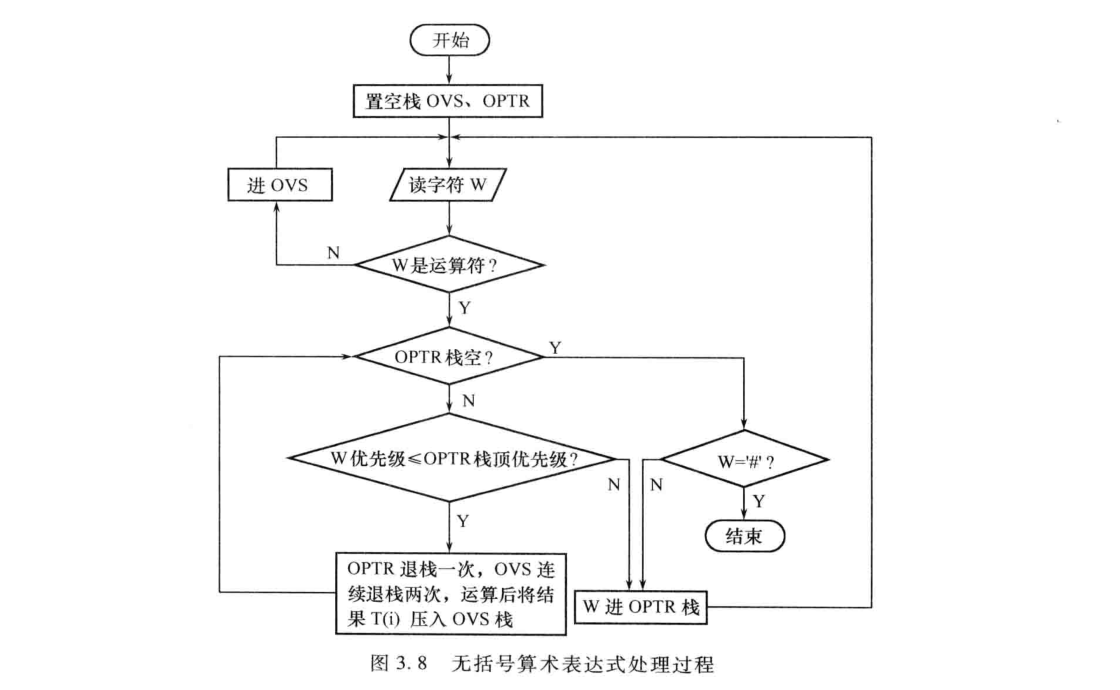
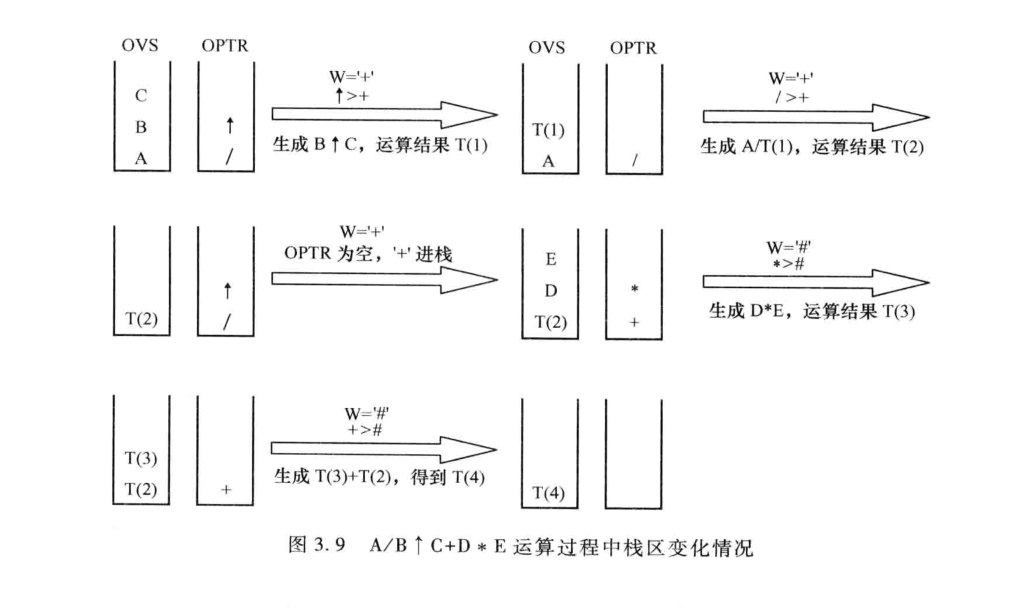
3.代码
//
// Created by trmbh on 2023-09-11.
//
#include<stdio.h>
#include<stdlib.h>
#include<string.h>
#include<math.h>
#define FALSE 0
#define TRUE 1
#define StackElementType char
typedef struct Node {
StackElementType data;
struct Node *next;
} LinkStackNode, *LinkStack;
/* 初始化栈区 */
int InitLinkStack(LinkStack *top) {
*top = (LinkStack) malloc(sizeof(LinkStackNode));
if (top == NULL) return FALSE
(*top)->next = NULL;
return TRUE
}
/* 入栈操作 */
int Push(LinkStack top, StackElementType x) {
LinkStackNode *temp;
temp = (LinkStackNode *) malloc(sizeof(LinkStackNode));
temp->data = x;
temp->next = top->next;
top->next = temp;
return TRUE;
}
/* 出栈操作 */
int Pop(LinkStack top, StackElementType *x) {
if (top->next == NULL) return FALSE;
LinkStackNode *temp;
temp = top->next;
top->next = temp->next;
*x = temp->data;
free(temp);
return TRUE;
}
/* 获得栈首数据 */
int GetTop(LinkStack top, StackElementType *x) {
if (top->next == NULL) return FALSE;
*x = top->next->data;
return TRUE;
}
/* 判断操作符 */
int IsOPTR(char c) {
switch (c) {
case '+':
case '-':
case '*':
case '/':
case '^': // 添加幂运算操作符
case '#':
return TRUE; // 是操作符
default:
return FALSE; // 不是操作符
}
}
/* 判断运算符优先级 */
char Compare(char c1, char c2) {
switch (c1) {
case '#':
if (c2 == '#') return '=';
else return '<';
case '+':
case '-':
if (c2 == '#') return '>';
else if (c2 == '+' || c2 == '-') return '=';
else return '<';
case '*':
case '/':
if (c2 == '#' || c2 == '+' || c2 == '-') return '>';
else if (c2 == '*' || c2 == '/') return '=';
else return '<';
case '^':
if (c2 == '^') return '=';
else return '>';
default:
return '!';
}
}
/* 判断是否为空 */
int isEmpty(LinkStack top) {
if (top->data == '#') return FALSE;
else return TRUE;
}
/* 表达式运算 */
int PerformOperation(int x, int y, char c) {
switch (c) {
case '^':
return pow(x, y);
case '*':
return x * y;
case '/':
if (y != 0) return x / y;
else return FALSE;
case '+':
return x + y;
case '-':
return x - y;
}
}
/* 表达式求值 */
int EvaluateExpression(char *expression) {
LinkStack operandStack, operatorStack;
StackElementType currentChar, nextChar, topOperator;
int operand1, operand2, result;
char opChar1,opChar2;
/* 初始化操作数栈和符号栈 */
InitLinkStack(&operandStack);
InitLinkStack(&operatorStack);
/* 先向符号栈导入#,方便后续操作*/
Push(operatorStack, '#');
GetTop(operatorStack, &topOperator);
while (*expression != '#' || topOperator != '#') {
if (!IsOPTR(*expression)) {
Push(operandStack, *expression);
} else {
switch (Compare(*expression, topOperator)) {
case '<':
case '=':
Pop(operandStack, &opChar1);
Pop(operandStack, &opChar2);
Pop(operatorStack, &topOperator);
operand1 = (int) (opChar1 - '0');
operand2 = (int) (opChar2 - '0');
result = PerformOperation(operand2, operand1, topOperator);
Push(operandStack, (char)(result + '0'));
if (*expression != '#') {
Push(operatorStack, *expression);
}
break;
case '>':
Push(operatorStack, *expression);
break;
}
}
if (*expression != '#') {
expression++;
}
GetTop(operatorStack, &topOperator);
}
GetTop(operandStack, ¤tChar);
return (int)(currentChar - '0');
}
int main() {
char str[100];
printf("请输入表达式(以#结尾):");
/* 第二个参数为最大字符数限制, 第三个参数为从标准输入流读入*/
fgets(str, sizeof(str), stdin); // 使用fgets接收输入
// 移除输入字符串中的换行符
size_t len = strlen(str);
if (len > 0 && str[len - 1] == '\n') {
str[len - 1] = '\0';
}
printf("表达式计算结果:%d\n", EvaluateExpression(str));
return 0;
}