Ultra-QuickSort
Time Limit: 7000MS | Memory Limit: 65536K | |
Total Submissions: 68874 | Accepted: 25813 |
Description
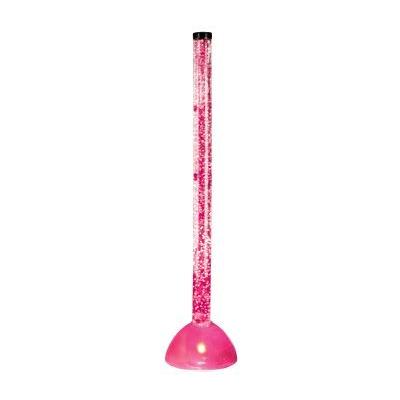
Ultra-QuickSort produces the output
Your task is to determine how many swap operations Ultra-QuickSort needs to perform in order to sort a given input sequence.
Input
The input contains several test cases. Every test case begins with a line that contains a single integer n < 500,000 -- the length of the input sequence. Each of the the following n lines contains a single integer 0 ≤ a[i] ≤ 999,999,999, the i-th input sequence element. Input is terminated by a sequence of length n = 0. This sequence must not be processed.
Output
For every input sequence, your program prints a single line containing an integer number op, the minimum number of swap operations necessary to sort the given input sequence.
Sample Input
5 9 1 0 5 4 3 1 2 3 0
Sample Output
6 0
Source
题目大意:求一组数据的逆序对,有多组数据,每组数据以一个n开头,读入以0结尾。
做法:归并排序(裸题)
代码如下:
1 #include <cstdio> 2 #include <cstring> 3 #include <iostream> 4 #include <algorithm> 5 #define N 500007 6 #define LL long long 7 using namespace std; 8 int n; 9 LL a[N], b[N], ans; 10 11 inline LL read() 12 { 13 LL s = 0; 14 char ch = getchar(); 15 while (ch < '0' || ch > '9') ch = getchar(); 16 while (ch >= '0' && ch <= '9') s = s * 10 + ch - '0', ch = getchar(); 17 return s; 18 } 19 20 inline void merge(int l, int mid, int r) 21 { 22 int i = l, j = mid + 1; 23 for (int k = l; k <= r; k++) 24 if (j > r || i <= mid && a[i] < a[j]) b[k] = a[i++]; 25 else b[k] = a[j++], ans += mid - i + 1; 26 for (int k = l; k <= r; k++) a[k] = b[k]; 27 } 28 29 inline void mergeSort(int a, int b) 30 { 31 int mid = (a + b) / 2; 32 if (a < b) 33 { 34 mergeSort(a, mid); 35 mergeSort(mid + 1, b); 36 merge(a, mid, b); 37 } 38 } 39 40 int main() 41 { 42 while(scanf("%d", &n) && n != 0) 43 { 44 ans = 0; 45 for (int i = 1; i <= n; i++) 46 a[i] = read(); 47 mergeSort(1, n); 48 cout << ans << endl; 49 } 50 }