场景设计
- 实现登陆基本功能,输出相应结果,脚本通
- 多用户实现随机登陆
- 添加初始化方法on_start: 每个用户只运行一次
- 添加检查点: catch_responses = True
脚本设计
# 导入对应的库
from locust import HttpUser, task, between
import os
# 任务类
class TestLogin(TaskSet):
# 定义一个登陆任务
@task
def to_login(self):
"""
1. 登陆需要账号密码,body以json格式
2. 登陆是post请求 '/v1/token'
3. 打印res值
"""
data = {"account": "wangxiao@qq.com", "password": "123456"}
res = self.client.post("/v1/token", json=data)
print(res.text)
class WebUser(HttpUser):
tasks = [TestLogin]
# 任务执行之间等待的时间,最小2秒,最大5秒
wait_time = between(2, 5)
# 被测地址:这个接口是自己写的一个登陆接口,实际可以需要自己抓包,更换真实环境中的地址
host = "http://127.0.0.1:5000"
if __name__ == '__main__':
os.system("locust -f testsm_demo.py")
# 设置用户1,每秒启动用户1,确定脚本是通,从打印出来的内容我们可以看出,脚本是通过的(这里你也可以打开locust webUI界面)
[2020-08-19 10:53:19,249] md2bkpyc/INFO/locust.runners: All users hatched: WebUser: 1 (0 already running)
{
"error_code": 0,
"token": "eyJhbGciOiJIUzUxMiIsImlhdCI6MTU5NzgwNTYwNCwiZXhwIjoxNTk3ODEyODA0fQ.eyJ1aWQiOjEsInNjb3BlIjpudWxsfQ.GS-lHngBcboPvnipWOl1ad5bkSEYweLwzSMa8fZpYVoz5ZDndV0gJOPvUiu8oiXIZi6AR7amLUcG7KKcBsf0Cg"
}
多用户实现随机登陆
- 定义一组登陆账号
- 导入choice方法,从data中随机选一个登陆
__author__ = 'wangxiao'
# 导入对应的库
from locust import HttpUser, task, between, TaskSet
import os
from random import choice
# 任务类
class TestLogin(TaskSet):
@task
def to_login(self):
data = [{"account": "wangxiao@qq.com", "password": "123456"},
{"account": "tester06@qq.com", "password": "123456"},
{"account": "admin@admin.com", "password": "123456"}
]
res = self.client.post("/v1/token", json=choice(data))
print(res.text)
class WebUser(HttpUser):
tasks = [TestLogin]
wait_time = between(2, 5)
host = "http://127.0.0.1:5000"
if __name__ == '__main__':
os.system("locust -f testsm_demo.py")
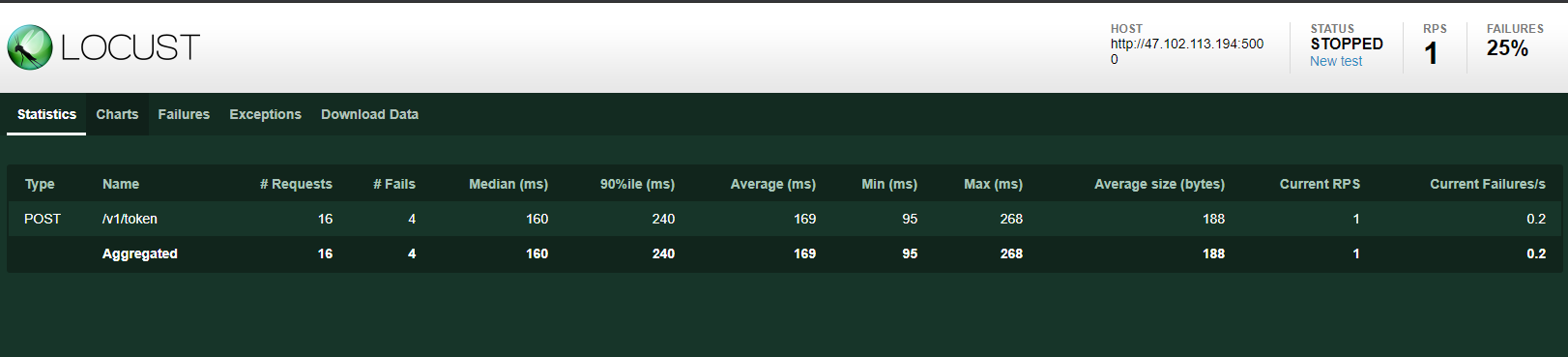
添加初始化方法on_start
- on_start 与on_stop方法对应,每个虚拟用户执行操作时前会运行一次on_start 推迟执行 on_stop
- 重构方法,把data放到on_start中
__author__ = 'wangxiao'
# 导入对应的库
from locust import HttpUser, task, between, TaskSet
import os
from random import choice
# 任务类
class TestLogin(TaskSet):
def on_start(self):
self.data = [{"account": "wangxiao@qq.com", "password": "123456"},
{"account": "tester06@qq.com", "password": "123456"},
{"account": "admin@admin.com", "password": "123456"}
]
def on_stop(self):
print("task结束")
@task
def to_login(self):
res = self.client.post("/v1/token", json=choice(self.data))
print(res.text)
class WebUser(HttpUser):
tasks = [TestLogin]
wait_time = between(2, 5)
host = "http://127.0.0.1:5000"
if __name__ == '__main__':
os.system("locust -f testsm_demo.py")
设置检查点: catch_responses = True
- catch_response: 布尔值,True or False, 用于发送请求,作为with语句返回上下文管理器的参数,将根据不同的响应将请求内容标记成功or失败,所以这边用 catch_response来捕捉,注意要用with语句来返回上下文
- success() failure(): 响应类中充当了上下文管理器,他提供了两个方法success() or failure()
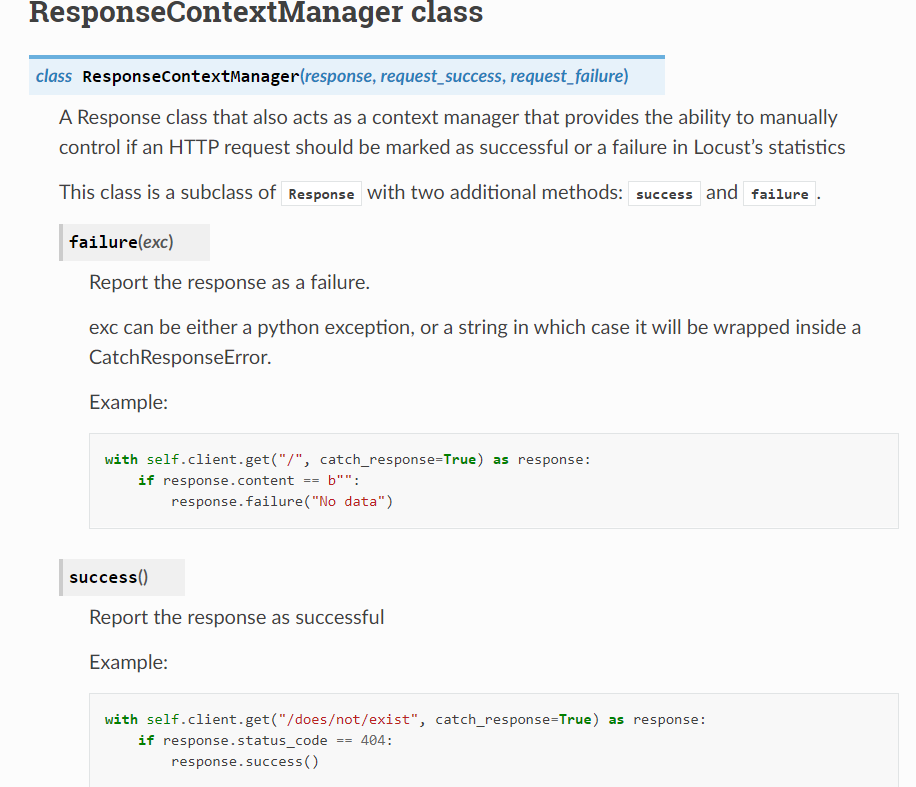
__author__ = 'wangxiao'
# 导入对应的库
from locust import HttpUser, task, between, TaskSet
import os
from random import choice
# 任务类
class TestLogin(TaskSet):
def on_start(self):
self.data = [{"account": "wangxiao@qq.com", "password": "123456"},
{"account": "tester06@qq.com", "password": "123456"},
{"account": "admin@admin.com", "password": "123456"},
{"account": "admin@admin.com", "password": "12334456"}
]
@task
def to_login(self):
with self.client.post("/v1/token", json=choice(self.data), catch_response=True) as response:
res = response.json()
if res['error_code'] == 0:
response.success()
else:
response.failure(res['msg'])
def on_stop(self):
print("task结束")
class WebUser(HttpUser):
tasks = [TestLogin]
wait_time = between(2, 5)
host = "http://127.0.0.1:5000"
if __name__ == '__main__':
os.system("locust -f testsm_demo.py")
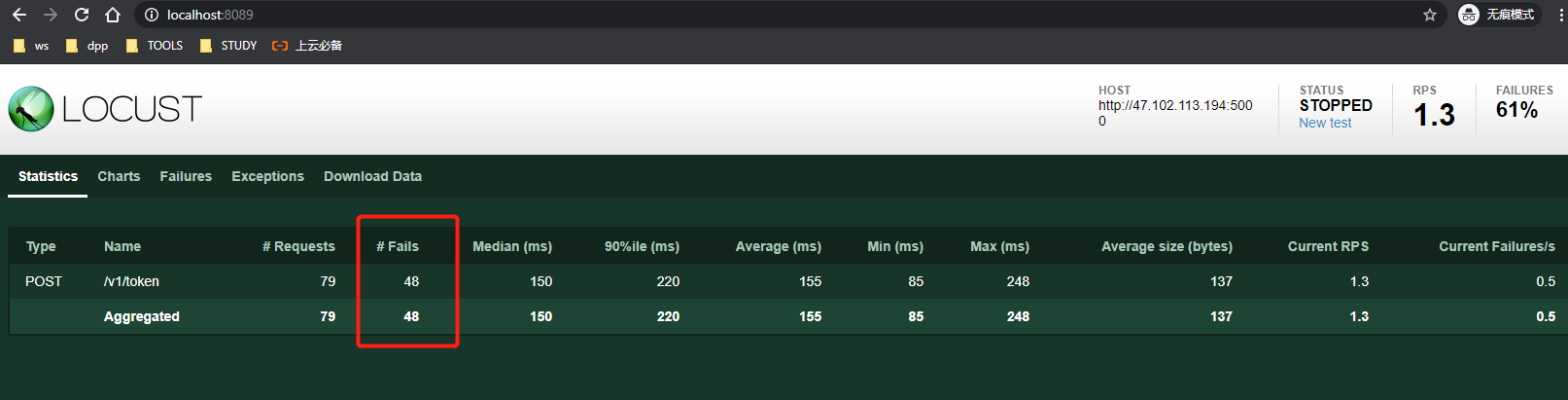
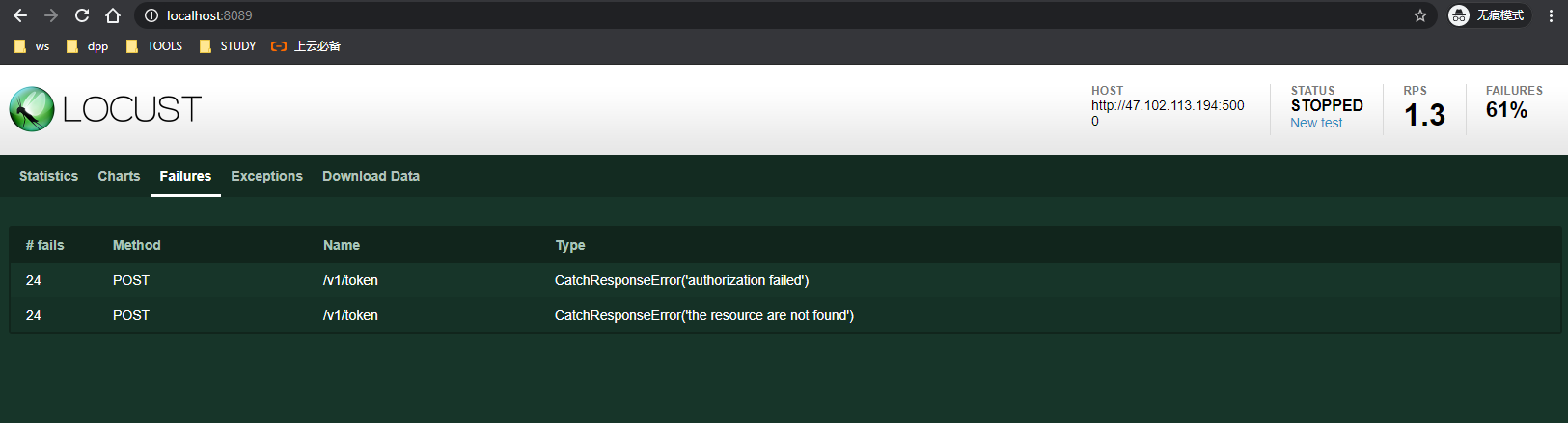