常用排序算法-选择排序
选择法排序:
找出最小值,依次第一个位置互换。
例如数组:33,6,-5,59,-12
第一次遍历: 找出最小值-12与数组第一个值33互换
第二次遍历:找出除数组第一个值以外的剩下的最小值-5,与数组第二个位置的值6互换
......
外部循环设定遍历次数,数组5个元素最多需要4次遍历,因为每次决定数组的一个位置,最后一个不用比较。(n-1)
#include<iostream> //selection sort using namespace std; void display(int array[], int n) { for(int count=0;count<n;count++) //print all items of array { cout<<array[count]<<'\t'; } cout<<endl; } int selection_sort(int array[], int n) { int temp=0,count=0 ; //set an temporary variable int flag=0; cout<<"debug information:"<<endl; for(int i=0;i<n-1;i++) { int min=array[i]; //difine a variable to store min number flag=i; //define a flag for(int j=i+1;j<n;j++) { if(array[j]<min) { min=array[j]; flag=j; } } if(i!=flag) //if the min number isn't array[i] then swap { temp=array[i]; array[i]=array[flag]; array[flag]=temp; count++; } display(array,n ); } // cout<<count<<endl; return 0; } int main() { int array[5]={33,6,-5,59,-12}; int num_array=sizeof(array)/sizeof(int); //获取数组长度 cout<<"before sort the array is :"<<endl; display(array,num_array); selection_sort(array,num_array); cout<<"after sort the array is :"<<endl; display(array,num_array); system("pause"); return 0; }
冒泡法排序算法
http://www.cnblogs.com/tobecrazy/archive/2013/03/13/2958337.html
插入法排序算法
http://www.cnblogs.com/tobecrazy/archive/2013/03/26/2983292.html
转载请注明出处:http://www.cnblogs.com/tobecrazy/
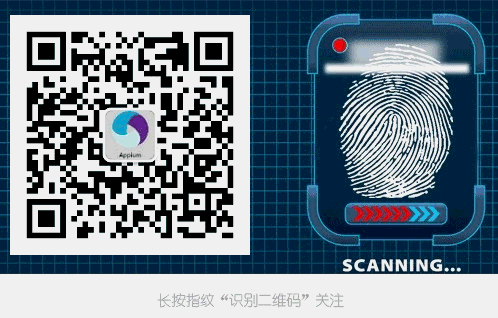