常用排序算法-冒泡排序
冒泡排序
冒泡法排序就是将需要排序内容从第一个元素开始,与相邻元素比较大小,将较大的/较小的放到后边,第一次遍历最后一个元素是最大/最小。
依次类推,第二次将倒数第二大/第二小元素放到倒数第二的位置。直到全部元素按照顺序排列。
比如5个元素:
第一次遍历 需要比较4次(相邻元素之间比较,最坏情况)
第二次遍历 比较3次
...
第四次遍历 比较1次
所以算法复杂度是n*(n-1)/2

1 #include<iostream> 2 using namespace std; 3 void display(int array[], int n) 4 { 5 for(int count=0;count<n;count++) //print all items of array 6 { 7 cout<<array[count]<<'\t'; 8 } 9 cout<<endl; 10 } 11 int bubble_sort(int array[], int n) 12 { 13 int temp=0,count=0 ; //set an temporary variable 14 cout<<"debug information:"<<endl; 15 for(int i=0;i<n;i++) 16 { 17 for(int j=0;j<n-i-1;j++) 18 { 19 if(array[j]>array[j+1]) 20 { 21 temp=array[j+1]; 22 array[j+1]=array[j]; 23 array[j]=temp; 24 count++; 25 } 26 display(array,n ); 27 } 28 29 } 30 cout<<count<<endl; 31 return 0; 32 } 33 int main() 34 { 35 int array[5]={35,14,3,02,-1}; 36 int num_array=sizeof(array)/sizeof(int); //获取数组长度 37 cout<<"before sort the array is :"<<endl; 38 display(array,num_array); 39 bubble_sort(array,num_array); 40 cout<<"after sort the array is :"<<endl; 41 display(array,num_array); 42 system("pause"); 43 return 0; 44 }
选择法排序算法
http://www.cnblogs.com/tobecrazy/archive/2013/03/14/2960526.html
插入法排序算法
http://www.cnblogs.com/tobecrazy/archive/2013/03/26/2983292.html
转载请注明出处:http://www.cnblogs.com/tobecrazy/
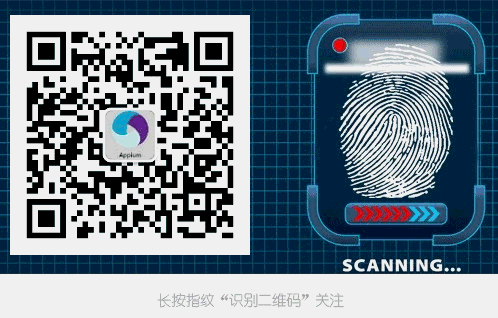