perl 自定义包/模块的使用(正则表达式匹配email地址和ip)
1. 正则表达式
email: =~ /^([0-9a-zA-Z]{3,}|[0-9a-zA-Z]+(-|_)?[0-9a-zA-Z_-]+\.?[0-9a-zA-Z]+)\@[0-9a-zA-Z]+\.[0-9a-zA-Z]+(\.\w+)?/;
ip =~/([0-9]{1,3}\.?){4}/;
. Match any character except a wrap character.
\w Match "word" character and [_0-9a-zA-Z]
\W Match non-word character [^_0-9a-zA-Z]
\s Match whitespace character [ \r\t\n\f]
\S Match non-whitespace character [^ \r\t\n\f]
\d Match digit character [0-9]
\D Match non-digit character [^0-9]
\t Match tab
\n Match newline
^ Match the head
$ Match the end
* Match 0 or more times
+ Match 1 or more times
? Match 0 or 1 times
{n} Match exactly n times
{n,} Match at least n times
{n,m} Match at least n but not more than m times
a|b match a or match b
2.自定义包/模块
包和模块的区别:
1.一个包的定义可以跨多个模块,一个模块中也可以有多个包定义;
2.定义模块时模块名必须和文件名一致,包无此要求;
注意:包和模块文件末尾需要加1;(return 1)
比如: 定义包名为getip.pm 在包文件里写入package getip;

1 #!/usr/bin/perl -w 2 package packagetest; 3 use strict; 4 sub get_local_ip 5 { 6 chomp(my $ip=`ifconfig eth0|grep -oE '([0-9]{1,3}\\.?){4}'|head -n 1`); 7 return $ip; 8 } 9 sub check_email 10 { 11 my $temp=$_[0]; 12 if($temp=~/^([0-9a-zA-Z]{3,}|[0-9a-zA-Z]+(-|_)?[0-9a-zA-Z_-]+\.?[0-9a-zA-Z]+)\@[0-9a-zA-Z]+\.[0-9a-zA-Z]+(\.\w+)?/) 13 { 14 return $temp; 15 } 16 else 17 { 18 return $temp="illegal email address!" 19 } 20 } 21 1;
3.如何使用
使用自定义包,在perl文件加入
package packagetest;(和包放在同一目录下)
使用函数:
包名::函数名

1 #!/usr/bin/perl -w 2 require "package.pm"; 3 package packagetest; 4 $localip=&packagetest::get_local_ip; 5 print $localip; 6 print "\n"; 7 $mail=&packagetest::check_email('someone_example@cn.com'); 8 print "$mail\n"; 9 [root@localhost perl]# more getip.pl 10 #!/usr/bin/perl -w 11 require "package.pm"; 12 package packagetest; 13 $localip=&packagetest::get_local_ip; 14 print $localip; 15 print "\n"; 16 $mail=&packagetest::check_email('someone_example@cn.com'); 17 print "$mail\n";
总结:
1. perl很开放,正则表达式也很深奥,很多情况perl不报错但是结果是错的。
2.perl模块很多,有些模块依赖别的模块,需要一一安装。
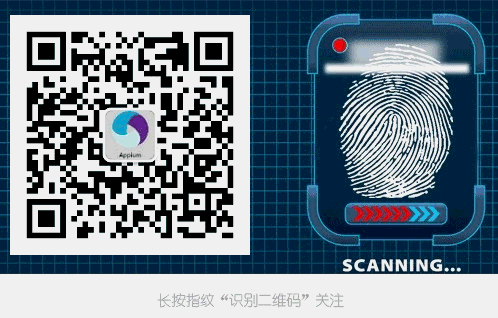