<%@ page language="java" import="java.util.*" pageEncoding="utf-8"%>
<%@page import="java.sql.SQLException"%>
<%@page import="com.gd.dao.BaseDao"%>
<%@page import="com.gd.entity.Msg"%>
<%@page import="java.sql.DriverManager"%>
<%@page import="java.sql.PreparedStatement"%>
<%@page import="java.sql.ResultSet"%>
<%@page import="java.sql.Connection"%>
<html>
<head>
<title>Title</title>
</head>
<body>
<%
request.setCharacterEncoding("utf-8");
String uname = request.getParameter("uname");
session.setAttribute("uname", uname);
Connection conn = null;
PreparedStatement ps = null;
ResultSet rs = null;
try {
Class.forName("com.mysql.jdbc.Driver");
//加载驱动
String url = "jdbc:mysql://localhost:3306/user";
String user = "root";
String password = "itcast";
conn = DriverManager.getConnection(url, user, password);
// 连接对象
//conn = BaseDao.getConnection();
%>
<a href="editemail.jsp">写邮件</a>
<table align="center">
<tr>
<td>username</td>
<td>title</td>
<td>msgcontent</td>
<td>state</td>
<td>sendto</td>
<td>msg_create_date</td>
</tr>
<%
Msg msg = new Msg();
ps = conn.prepareStatement("select * from msg where username=?");
ps.setString(1, uname);
rs = ps.executeQuery();
while (rs.next()) {
%>
<tr>
<td><%=rs.getString("username")%></td>
<td><a href="content.jsp?id=<%=rs.getInt("msgid")%>"><%=rs.getString("title")%></a></td>
<td><%=rs.getString("msgcontent")%></td>
<td>
<%
if (rs.getString("state").equals("1")) {
%> <%
out.print("未读");
%> <%
} else {
%> <%
out.print("已读");
%> <%
}
%>
</td>
<td><%=rs.getString("sendto")%></td>
<td><%=rs.getString("msg_create_date")%></td>
</tr>
<%
}
%>
</table>
<br>
<%
} catch (Exception e) {
e.printStackTrace();
} finally {
//BaseDao.closeAll(conn, ps, rs);
try {
if (rs != null) {
rs.close();
}
} finally {
try {
if (ps != null) {
ps.close();
}
} finally {
if (conn != null) {
conn.close();
}
}
}
}
%>
</body>
</html>
<%@ page language="java" import="java.util.*" pageEncoding="utf-8"%>
<%@page import="java.sql.SQLException"%>
<%@page import="com.gd.dao.BaseDao"%>
<%@page import="com.gd.entity.Msg"%>
<%@page import="java.sql.DriverManager"%>
<%@page import="java.sql.PreparedStatement"%>
<%@page import="java.sql.ResultSet"%>
<%@page import="java.sql.Connection"%>
<%
String path = request.getContextPath();
String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<base href="<%=basePath%>">
<title>My JSP 'insert.jsp' starting page</title>
<meta http-equiv="pragma" content="no-cache">
<meta http-equiv="cache-control" content="no-cache">
<meta http-equiv="expires" content="0">
<meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
<meta http-equiv="description" content="This is my page">
<!--
<link rel="stylesheet" type="text/css" href="styles.css">
-->
</head>
<body>
<%
request.setCharacterEncoding("utf-8");
String uname = (String) session.getAttribute("uname");
String ctitle = request.getParameter("ctitle");
String ccontent = request.getParameter("ccontent");
String sendtoid = request.getParameter("sendtoid");
String contitle = (String) session.getAttribute("newcontitle");
session.setAttribute("uname", uname);
Connection conn = null;
PreparedStatement ps = null;
ResultSet rs = null;
try {
Class.forName("com.mysql.jdbc.Driver");
//加载驱动
String url = "jdbc:mysql://localhost:3306/users";
String user = "root";
String password = "admin";
conn = DriverManager.getConnection(url, user, password);
// 连接对象
//conn = BaseDao.getConnection();
%>
<%
if(contitle!=null){
Msg msg = new Msg();
ps = conn.prepareStatement("select * from msg where title=?and username=?");
ps.setString(1, contitle);
ps.setString(2, uname);
rs = ps.executeQuery();
while (rs.next()) {
ps = conn.prepareStatement("insert into msg(username,title,msgcontent,sendto,state,msg_create_date)" + "values('"
+ rs.getString("sendto") + "','" + ctitle + "','" + ccontent + "','"
+ uname + "','" + "1" + "','"+"2020-05-11"+"')");
ps.executeUpdate();
request.getRequestDispatcher("index.jsp").forward(request, response);
%>
<%
}
}else{
Msg msg = new Msg();
ps = conn.prepareStatement("insert into msg(username,title,msgcontent,sendto,state,msg_create_date)" + "values('"
+ sendtoid + "','" + ctitle + "','" + ccontent + "','"
+ uname + "','" + "1" + "','"+"2020-05-11"+"')");
ps.executeUpdate();
request.getRequestDispatcher("index.jsp").forward(request, response);
}
%>
<%
} catch (Exception e) {
e.printStackTrace();
} finally {
//BaseDao.closeAll(conn, ps, rs);
try {
if (rs != null) {
rs.close();
}
} finally {
try {
if (ps != null) {
ps.close();
}
} finally {
if (conn != null) {
conn.close();
}
}
}
}
%>
<br>
</body>
</html>
<%@ page language="java" import="java.util.*" pageEncoding="utf-8"%>
<%
String path = request.getContextPath();
String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<base href="<%=basePath%>">
<title>My JSP 'index.jsp' starting page</title>
<meta http-equiv="pragma" content="no-cache">
<meta http-equiv="cache-control" content="no-cache">
<meta http-equiv="expires" content="0">
<meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
<meta http-equiv="description" content="This is my page">
<!--
<link rel="stylesheet" type="text/css" href="styles.css">
-->
</head>
<body>
<form action="dologin.jsp" method="post">
用户名:<input type="text" name="uname" value="kitty" /><Br>
密码 :<input type="password" name="upwd" value="777"/><br>
<input type="submit" value="登录">
</form>
</body>
</html>
<%@ page language="java" import="java.util.*" pageEncoding="utf-8"%>
<%@page import="java.sql.SQLException"%>
<%@page import="com.gd.dao.BaseDao"%>
<%@page import="com.gd.entity.Msg"%>
<%@page import="java.sql.DriverManager"%>
<%@page import="java.sql.PreparedStatement"%>
<%@page import="java.sql.ResultSet"%>
<%@page import="java.sql.Connection"%>
<%
String path = request.getContextPath();
String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<base href="<%=basePath%>">
<title>My JSP 'detail.jsp' starting page</title>
<meta http-equiv="pragma" content="no-cache">
<meta http-equiv="cache-control" content="no-cache">
<meta http-equiv="expires" content="0">
<meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
<meta http-equiv="description" content="This is my page">
<!--
<link rel="stylesheet" type="text/css" href="styles.css">
-->
</head>
<body>
<%
request.setCharacterEncoding("utf-8");
String uname = (String) session.getAttribute("uname");
session.setAttribute("uname", uname);
Connection conn = null;
PreparedStatement ps = null;
ResultSet rs = null;
try {
Class.forName("com.mysql.jdbc.Driver");
//加载驱动
String url = "jdbc:mysql://localhost:3306/user";
String user = "root";
String password = "itcast";
conn = DriverManager.getConnection(url, user, password);
// 连接对象
//conn = BaseDao.getConnection();
%>
<form action="insert.jsp" name="huifu" method="post">
sendto :<input type="text" name="sendtoid"><br> <br>
标题:<input type="text" name="ctitle"><br> <br>
正文:<input type="text" name="ccontent"><br>
<br>
<input type="submit" value="发送">
<a href="main.jsp">返回</a>
</form>
<%
} catch (Exception e) {
e.printStackTrace();
} finally {
//BaseDao.closeAll(conn, ps, rs);
try {
if (rs != null) {
rs.close();
}
} finally {
try {
if (ps != null) {
ps.close();
}
} finally {
if (conn != null) {
conn.close();
}
}
}
}
%>
</body>
</html>
<%@page import="com.gd.dao.UsersDao" %>
<%@page import="com.gd.entity.Users" %>
<%@ page import="javax.swing.*" %>
<%@ page language="java" import="java.util.*" pageEncoding="utf-8"%>
<%
request.setCharacterEncoding("utf-8");
String uname = request.getParameter("uname");
String upwd = request.getParameter("upwd");
UsersDao ud = new UsersDao();
if (uname.equals("")) {
//用户名为空的情况
String msg = "用户名不能为空!";
int type = JOptionPane.YES_NO_OPTION;
String title = "信息提示";
JOptionPane.showMessageDialog(null, msg, title, type);
request.getRequestDispatcher("index.jsp").forward(request, response);
} else {
//密码账号登录成功
if (ud.login(uname, upwd)) {
Users u = new Users();
u.setUsername(uname);
u.setPassword(upwd);
session.setAttribute("user", u);
request.getRequestDispatcher("main.jsp").forward(request, response);
} else {
//账号密码不匹配
String msg = "用户名或密码不正确,登录失败!";
int type = JOptionPane.YES_NO_OPTION;
String title = "信息提示";
JOptionPane.showMessageDialog(null, msg, title, type);
request.getRequestDispatcher("index.jsp").forward(request, response);
}
}
%>
package com.gd.dao;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
public class UsersDao extends BaseDao {
public boolean login(String uname, String upwd) throws SQLException {
Connection conn = getConnection();
String sql = "select * from users where username=? and password=?";
PreparedStatement ps = conn.prepareStatement(sql);
ps.setString(1, uname);
ps.setString(2, upwd);
ResultSet rs = ps.executeQuery();
if (rs.next()) {
realse(rs, ps, conn);
return true;
} else {
realse(rs, ps, conn);
return false;
}
}
}
package com.gd.dao;
public class MsgDao {
//发送,回复---insert操作
//邮件列表 --select * from msg where username=....
//根据标题查内容 select
//阅读状态改变,,,未读 已读 update
//删除邮件 delete
}
package com.gd.dao;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
public class BaseDao {
// 获取连接
public Connection getConnection() {
Connection conn = null;
try {
Class.forName("com.mysql.jdbc.Driver");
// 2.建立连接
conn = DriverManager.getConnection(
"jdbc:mysql://localhost:3306/user", "root", "itcast");
} catch (Exception e) {
e.printStackTrace();
}
return conn;
}
// 关闭连接
public static void realse(ResultSet rs, PreparedStatement ps, Connection conn) {
{
//6.关闭资源,释放资源
if (rs != null) {
try {
rs.close();
} catch (SQLException e) { // ignore }
rs = null;
}
}
if (ps != null) {
try {
ps.close();
} catch (SQLException e) { // ignore }
ps = null;
}
}
if (conn != null) {
try {
conn.close();
} catch (SQLException e) { // ignore }
rs = null;
}
}
}
}
}
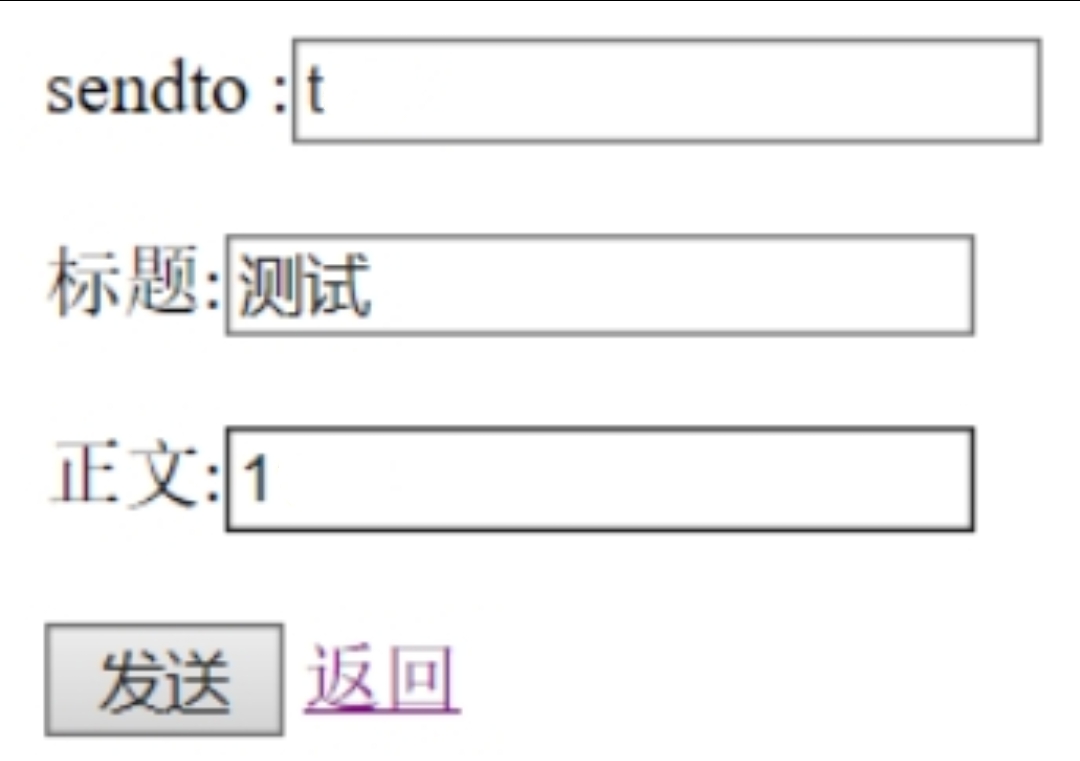