实验6 模板类、文件I/O和异常处理
task4:
Vector.hpp源代码:

1 #include<iostream> 2 3 using namespace std; 4 5 template <typename T> 6 class Vector 7 { 8 public: 9 Vector(int nn) :n{ nn } { 10 if (n < 0)throw length_error("Vector: negative size"); 11 elem = (T*)malloc(n * sizeof(T)); 12 if (!elem)exit(OVERFLOW); 13 } 14 Vector(int nn,T val) :n{ nn } { 15 if (n < 0)throw length_error("Vector: negative size"); 16 elem = (T*)malloc(n * sizeof(T)); 17 if (!elem)exit(OVERFLOW); 18 for (int i=0;i<n;i++) 19 { 20 elem[i] = val; 21 } 22 } 23 Vector(const Vector<T>& b){ 24 n = b.n; 25 elem = (T*)malloc(n * sizeof(T)); 26 if (!elem)exit(OVERFLOW); 27 for(int i=0;i<n;i++) 28 { 29 elem[i] = b.elem[i]; 30 } 31 } 32 ~Vector() { 33 n = 0; 34 free(elem); 35 } 36 int getsize() 37 { 38 return n; 39 } 40 T& at(int i) 41 { 42 if (i < 0||i>=n)throw out_of_range("Vector: index out of range"); 43 return elem[i]; 44 } 45 T& operator[](int i) 46 { 47 if (i < 0 || i>=n)throw out_of_range("Vector: index out of range"); 48 return elem[i]; 49 } 50 template <typename T1> 51 friend void output(const Vector<T1> a); 52 private: 53 T* elem; 54 int n; 55 }; 56 template <typename T> 57 void output(const Vector<T> a) 58 { 59 for (int i=0;i<a.n;i++) 60 { 61 cout << a.elem[i] << " "; 62 } 63 cout << endl; 64 }
task4.cpp源代码:

1 #include <iostream> 2 #include "Vector.hpp" 3 4 void test1() { 5 using namespace std; 6 7 int n; 8 cout << "Enter n: "; 9 cin >> n; 10 11 Vector<double> x1(n); 12 for(auto i = 0; i < n; ++i) 13 x1.at(i) = i * 0.7; 14 15 cout << "x1: "; output(x1); 16 17 Vector<int> x2(n, 42); 18 const Vector<int> x3(x2); 19 20 cout << "x2: "; output(x2); 21 cout << "x3: "; output(x3); 22 23 x2.at(0) = 77; 24 x2.at(1) = 777; 25 cout << "x2: "; output(x2); 26 cout << "x3: "; output(x3); 27 } 28 29 void test2() { 30 using namespace std; 31 32 int n, index; 33 while(cout << "Enter n and index: ", cin >> n >> index) { 34 try { 35 Vector<int> v(n, n); 36 v.at(index) = -999; 37 cout << "v: "; output(v); 38 } 39 catch (const exception &e) { 40 cout << e.what() << endl; 41 } 42 } 43 } 44 45 int main() { 46 cout << "测试1: 模板类接口测试\n"; 47 test1(); 48 49 cout << "\n测试2: 模板类异常处理测试\n"; 50 test2(); 51 }
运行结果:
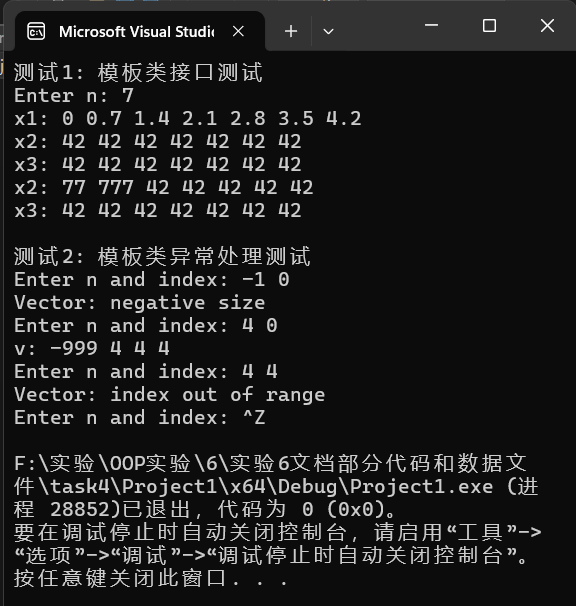
task5:
task5.cpp源码:

1 #include <iostream> 2 #include <iomanip> 3 #include <string> 4 #include<vector> 5 #include <fstream> 6 #include <algorithm> 7 8 using std::string; 9 using std::ostream; 10 using std::istream; 11 using std::setw; 12 using std::setprecision; 13 using std::setiosflags; 14 using std::ios_base; 15 16 class student { 17 public: 18 student() = default; 19 ~student() = default; 20 int get_score() const { return s; } 21 friend ostream& operator<<(ostream& out, const student& c); 22 friend istream& operator>>(istream& in, student& c); 23 friend bool cmp1(student& a, student& b); 24 friend bool cmp2(student& a, student& b); 25 private: 26 string no; // 学号 27 string name; // 姓名 28 string major; // 专业 29 int s; // 解题数 30 }; 31 ostream& operator<<(ostream& out, const student& c) { 32 out << setiosflags(ios_base::left); 33 out << setw(15) << c.no 34 << setw(20) << c.name 35 << setw(15) << c.major 36 << setw(5) << c.s; 37 return out; 38 } 39 istream& operator>>(istream& in, student& c) { 40 in >> c.no >> c.name >> c.major >> c.s; 41 return in; 42 } 43 44 void output(std::ostream& out, const std::vector<student>& v) { 45 for (auto& i : v) 46 out << i << std::endl; 47 } 48 void save(const std::string& filename, std::vector<student>& v) { 49 using std::ofstream; 50 ofstream out(filename); 51 if (!out.is_open()) { 52 std::cout << "fail to open file to write\n"; 53 return; 54 } 55 output(out, v); 56 out.close(); 57 } 58 59 void load(const std::string& filename, std::vector<student>& v) { 60 using std::ifstream; 61 ifstream in(filename); 62 if (!in.is_open()) { 63 std::cout << "fail to open file to read\n"; 64 return; 65 } 66 std::string title_line; 67 getline(in, title_line); 68 student t; 69 while (in >> t) 70 v.push_back(t); 71 in.close(); 72 } 73 bool cmp1(student& a, student& b) 74 { 75 return a.major.compare(b.major) < 0; 76 } 77 bool cmp2(student& a, student& b) 78 { 79 return a.s > b.s; 80 } 81 void Sort(std::vector<student>& v) 82 { 83 sort(v.begin(), v.end(), cmp2); 84 sort(v.begin(), v.end(), cmp1); 85 } 86 void test() { 87 using namespace std; 88 vector<student> v; 89 load("data5.txt", v); 90 Sort(v); 91 output(cout, v); 92 save("ans5.txt", v); 93 } 94 int main() { 95 test(); 96 }
date5.txt:
运行结果:
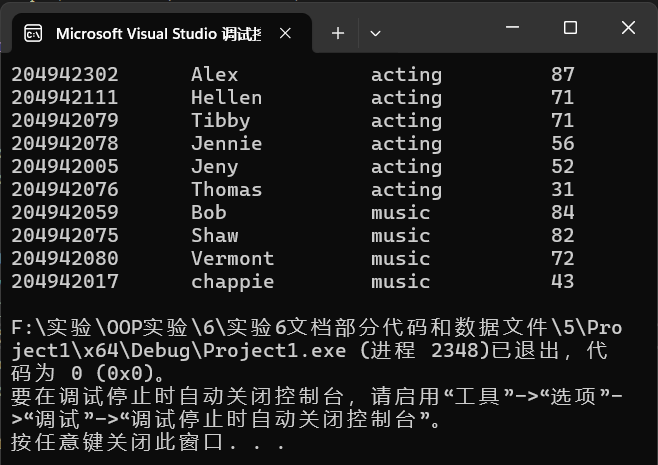