- C++多态
-
#include <iostream> #include <iomanip> using namespace std; class Base { public: virtual void f() { cout << "base" << endl; } }; class Derived : public Base{ public: void f() { cout << "derived" << endl; } }; class Base2 { public: virtual void g() { cout << "base2 process g" << endl; } // dynamic_cast only work under polymorphic settings }; class Derived2 : public Base2{ public: void f() { cout << "derived2" << endl; } }; int main() { /* Base *b = new Derived; b->f(); //base Base *b = new Derived; Derived *a = static_cast<Derived *>(b); a->f(); //derived Base *b = new Derived; b->f(); //derived, derived overwrite base in virtual table Derived *a = dynamic_cast<Derived *>(b); a->f(); //derived, same with b->f() */ Base2 *b = new Derived2; //b->f(); // compile error Derived2 *a = dynamic_cast<Derived2 *>(b); a->f(); //derived2, same with b->f() return 0; }
- 可调用函数, 匿名函数
-
auto f = [](int a, int b){return a + b;}; cout << f(1,2) << endl; //3 cout << [](int a, int b){return a + b;}(1,2) << endl; //3 int a = 0; int b = 0; int c = 0; //cout << [=](int a, int b){c = 1; return a + b;}(1,2) << "\t" << c << endl; // error: cannot assign to a variable captured by copy in a non-mutable lambda //cout << [](int a, int b){int c = 1; return a + b;}(1,2) << "\t" << c << a << endl; //3 \t 0 cout << [&](int a, int b){c = 1; return a + b;}(1,2) << "\t" << c << "\t" << a << endl; //3 \t 1 cout << [&c](int a, int b){c = 1; return a + b;}(1,2) << "\t" << c << endl; //3 \t 1
C++ 宏下的 # 和 ##
- #的功能是将其后面的宏参数进行字符串化操作
- ##被称为连接符(concatenator)
-
/// Macro for registering a layer type. /// Example: REGISTER_LAYER(crf_error, CRFDecodingErrorLayer); #define REGISTER_LAYER(__type_name, __class_name) \ static InitFunction __reg_type_##__type_name( \ []() { Layer::registrar_.registerClass<__class_name>(#__type_name); })
- C++explicit关键字详解
- http://www.cnblogs.com/ymy124/p/3632634.html
- C++中的explicit关键字只能用于修饰只有一个参数的类构造函数, 它的作用是表明该构造函数是显示的, 而非隐式的, 跟它相对应的另一个关键字是implicit, 意思是隐藏的,类构造函数默认情况下即声明为implicit(隐式). explicit关键字的作用就是防止类构造函数的隐式自动转换. explicit关键字只对有一个参数的类构造函数有效, 如果类构造函数参数大于或等于两个时, 是不会产生隐式转换的, 所以explicit关键字也就无效了。 但是 当除了第一个参数以外的其他参数都有默认值的时候, explicit关键字依然有效, 此时, 当调用构造函数时只传入一个参数, 等效于只有一个参数的类构造函数
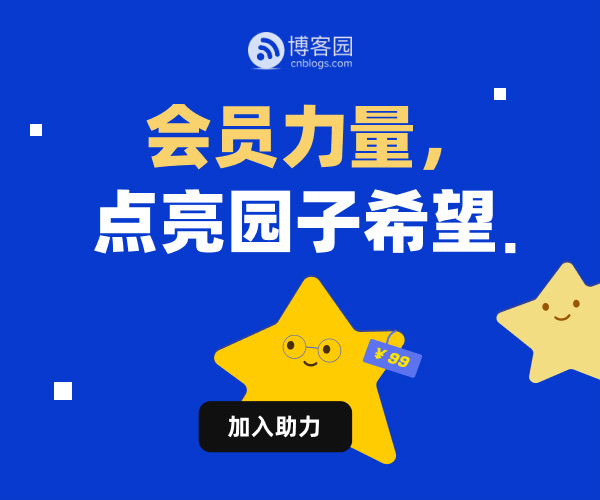