4、Controller的使用
@Controller
@ResponseBody- 等同于RESTController
- RequestMapping的使用
- 给同一个方法指定多个RequestMapping
- 给类添加RequestMapping
- 指定RequestMapping的()method属性
如何处理URL里面的参数?- @PathVariable的使用:
- http://127.0.0.1:8080/hello/say/243
- http://127.0.0.1:8080/hello/243/say
- @RequestParam的使用方式
- http://127.0.0.1:8080/hello/say?id=22223
- 给url当中的参数设置默认值
- 组合注解的使用
- GetMapping
- 完
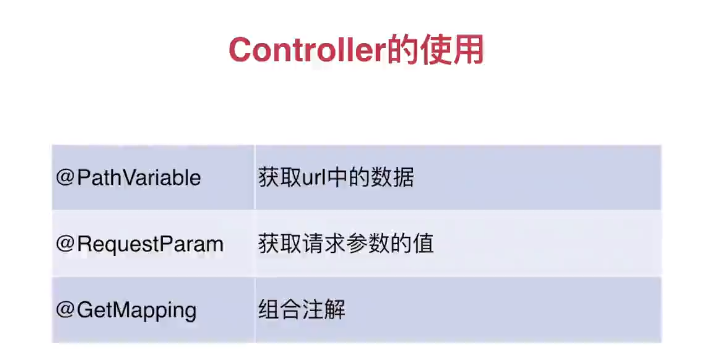
package com.girl;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.*;
/**
* Created by sunnyangzs on 2018/3/25.
*/
@RestController
@RequestMapping("/hello")
public class HelloController {
// @Value("${cupSize}")
// private String cupSize;
//
// @Value("${age}")
// private Integer age;
//
// @Value("${content}")
// private String content;
@Autowired
private GirlProperties girlProperties;
@RequestMapping(value = {"/say/{id}"},method = RequestMethod.GET)
public String say(@PathVariable("id") Integer id){
// return girlProperties.getCupSize();
return "id: "+id;
}
}
//把id写在了say的后面
35
1
package com.girl;
2
3
import org.springframework.beans.factory.annotation.Autowired;
4
import org.springframework.beans.factory.annotation.Value;
5
import org.springframework.stereotype.Controller;
6
import org.springframework.web.bind.annotation.*;
7
8
/**
9
* Created by sunnyangzs on 2018/3/25.
10
*/
11
12
@RestController
13
@RequestMapping("/hello")
14
public class HelloController {
15
16
// @Value("${cupSize}")
17
// private String cupSize;
18
//
19
// @Value("${age}")
20
// private Integer age;
21
//
22
// @Value("${content}")
23
// private String content;
24
25
@Autowired
26
private GirlProperties girlProperties;
27
28
@RequestMapping(value = {"/say/{id}"},method = RequestMethod.GET)
29
public String say(@PathVariable("id") Integer id){
30
// return girlProperties.getCupSize();
31
return "id: "+id;
32
}
33
34
}
35
//把id写在了say的后面
package com.girl;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.*;
/**
* Created by sunnyangzs on 2018/3/25.
*/
@RestController
@RequestMapping("/hello")
public class HelloController {
// @Value("${cupSize}")
// private String cupSize;
//
// @Value("${age}")
// private Integer age;
//
// @Value("${content}")
// private String content;
@Autowired
private GirlProperties girlProperties;
@RequestMapping(value = {"/{id}/say"},method = RequestMethod.GET)
public String say(@PathVariable("id") Integer id){
// return girlProperties.getCupSize();
return "id: "+id;
}
}
//把id写在了say的前面
35
1
package com.girl;
2
3
import org.springframework.beans.factory.annotation.Autowired;
4
import org.springframework.beans.factory.annotation.Value;
5
import org.springframework.stereotype.Controller;
6
import org.springframework.web.bind.annotation.*;
7
8
/**
9
* Created by sunnyangzs on 2018/3/25.
10
*/
11
12
@RestController
13
@RequestMapping("/hello")
14
public class HelloController {
15
16
// @Value("${cupSize}")
17
// private String cupSize;
18
//
19
// @Value("${age}")
20
// private Integer age;
21
//
22
// @Value("${content}")
23
// private String content;
24
25
@Autowired
26
private GirlProperties girlProperties;
27
28
@RequestMapping(value = {"/{id}/say"},method = RequestMethod.GET)
29
public String say(@PathVariable("id") Integer id){
30
// return girlProperties.getCupSize();
31
return "id: "+id;
32
}
33
34
}
35
//把id写在了say的前面
package com.girl;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.*;
/**
* Created by sunnyangzs on 2018/3/25.
*/
@RestController
@RequestMapping("/hello")
public class HelloController {
// @Value("${cupSize}")
// private String cupSize;
//
// @Value("${age}")
// private Integer age;
//
// @Value("${content}")
// private String content;
@Autowired
private GirlProperties girlProperties;
@RequestMapping(value = {"/say"},method = RequestMethod.GET)
public String say(@RequestParam("id") Integer id){
// return girlProperties.getCupSize();
return "id: "+id;
}
}
1
35
1
package com.girl;
2
3
import org.springframework.beans.factory.annotation.Autowired;
4
import org.springframework.beans.factory.annotation.Value;
5
import org.springframework.stereotype.Controller;
6
import org.springframework.web.bind.annotation.*;
7
8
/**
9
* Created by sunnyangzs on 2018/3/25.
10
*/
11
12
@RestController
13
@RequestMapping("/hello")
14
public class HelloController {
15
16
// @Value("${cupSize}")
17
// private String cupSize;
18
//
19
// @Value("${age}")
20
// private Integer age;
21
//
22
// @Value("${content}")
23
// private String content;
24
25
@Autowired
26
private GirlProperties girlProperties;
27
28
@RequestMapping(value = {"/say"},method = RequestMethod.GET)
29
public String say(@RequestParam("id") Integer id){
30
// return girlProperties.getCupSize();
31
return "id: "+id;
32
}
33
34
}
35
package com.girl;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.*;
/**
* Created by sunnyangzs on 2018/3/25.
*/
@RestController
@RequestMapping("/hello")
public class HelloController {
@Autowired
private GirlProperties girlProperties;
@RequestMapping(value = {"/say"},method = RequestMethod.GET)
public String say(@RequestParam("id") Integer myId){
// return girlProperties.getCupSize();
return "id: "+myId;
}
}
1
package com.girl;
2
3
import org.springframework.beans.factory.annotation.Autowired;
4
import org.springframework.beans.factory.annotation.Value;
5
import org.springframework.stereotype.Controller;
6
import org.springframework.web.bind.annotation.*;
7
8
/**
9
* Created by sunnyangzs on 2018/3/25.
10
*/
11
12
@RestController
13
@RequestMapping("/hello")
14
public class HelloController {
15
16
@Autowired
17
private GirlProperties girlProperties;
18
19
@RequestMapping(value = {"/say"},method = RequestMethod.GET)
20
public String say(@RequestParam("id") Integer myId){
21
// return girlProperties.getCupSize();
22
return "id: "+myId;
23
}
24
25
}
26
package com.girl;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.*;
/**
* Created by sunnyangzs on 2018/3/25.
*/
@RestController
@RequestMapping("/hello")
public class HelloController {
@Autowired
private GirlProperties girlProperties;
@RequestMapping(value = {"/say"},method = RequestMethod.GET)
public String say(@RequestParam(value = "id",required = false,defaultValue = "0") Integer myId){
// return girlProperties.getCupSize();
return "id: "+myId;
}
}
x
1
package com.girl;
2
3
import org.springframework.beans.factory.annotation.Autowired;
4
import org.springframework.beans.factory.annotation.Value;
5
import org.springframework.stereotype.Controller;
6
import org.springframework.web.bind.annotation.*;
7
8
/**
9
* Created by sunnyangzs on 2018/3/25.
10
*/
11
12
@RestController
13
@RequestMapping("/hello")
14
public class HelloController {
15
16
@Autowired
17
private GirlProperties girlProperties;
18
19
@RequestMapping(value = {"/say"},method = RequestMethod.GET)
20
public String say(@RequestParam(value = "id",required = false,defaultValue = "0") Integer myId){
21
// return girlProperties.getCupSize();
22
return "id: "+myId;
23
}
24
25
}
26
package com.girl;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.*;
/**
* Created by sunnyangzs on 2018/3/25.
*/
@RestController
@RequestMapping("/hello")
public class HelloController {
@Autowired
private GirlProperties girlProperties;
//@RequestMapping(value = {"/say"},method = RequestMethod.GET)
@GetMapping(value="/say")
public String say(@RequestParam(value = "id",required = false,defaultValue = "0") Integer myId){
// return girlProperties.getCupSize();
return "id: "+myId;
}
}
1
26
1
package com.girl;
2
3
import org.springframework.beans.factory.annotation.Autowired;
4
import org.springframework.beans.factory.annotation.Value;
5
import org.springframework.stereotype.Controller;
6
import org.springframework.web.bind.annotation.*;
7
8
/**
9
* Created by sunnyangzs on 2018/3/25.
10
*/
11
12
@RestController
13
@RequestMapping("/hello")
14
public class HelloController {
15
16
@Autowired
17
private GirlProperties girlProperties;
18
19
//@RequestMapping(value = {"/say"},method = RequestMethod.GET)
20
@GetMapping(value="/say")
21
public String say(@RequestParam(value = "id",required = false,defaultValue = "0") Integer myId){
22
// return girlProperties.getCupSize();
23
return "id: "+myId;
24
}
25
26
}
27