运算符
Java语言支持如下运算符:优先级()
-
算术运算符:+,-,*,/,%,++,--
-
赋值运算符 =
-
关系运算符>,<,>=,<=,==,!=instanceof
-
逻辑运算符:&&,||,!
public class Demo01 {
public static void main(String[] args) {
//二元运算符
//Crtl+D 复制当前行到下一行
int a = 10 ;
int b = 20 ;
int c = 25;
int d = 30;
System.out.println(a+b);
System.out.println(a-b);
System.out.println(a*b);
System.out.println(a/(double)b);
}
}
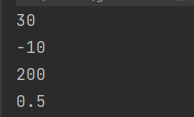
public class Demo02 {
public static void main(String[] args) {
long a = 123456789L;
int b = 123;
short c =10;
byte d = 8;
System.out.println(a+b+c+d);//long
System.out.println(b+c+d);//int
System.out.println(c+d);//int
}
}
public class Demo03 {
public static void main(String[] args) {
//关系运算符返回的结果: 正确.错误 布尔值
//if
int a = 10;
int b = 20;
int c = 21;
//取余:模运算
System.out.println(c%a);//c / a 21 /10 =2...1
System.out.println(a>b);
System.out.println(a<b);
System.out.println(a==b);
System.out.println(a!=b);
}
}
public class Demo04 {
public static void main(String[] args) {
//++ -- 自增,自臧 一元运算符
int a = 3;
int b = a++; //执行完这行代码后,先给b赋值,再自增
//a++ a = a + 1
System.out.println(a);
//++a a = a + 1
int c = ++a; //执行完这行代码后,先自增,再给b赋值
System.out.println(a);
System.out.println(b);
System.out.println(c);
//幂运算 2^3 2^2^2 = 8 很多运算,我们会使用一些工具类来操作!
double pow = Math.pow(2, 3);
System.out.println(pow);
}
}
//逻辑运算符
public class Demo05 {
public static void main(String[] args) {
//与(and) 或(or) 非(取反)
boolean a = true;
boolean b = false;
System.out.println("a && b:"+(a&&b));//逻辑与运算:两个变量都为真,结果才为true
System.out.println("a || b:"+(a||b));//逻辑与运算:两个变量有一个为真,结果才为true
System.out.println("!(a && b):"+!(a&&b));//如果是真,则变为假,如果是假则变为真
//短路运算
int c = 5;
boolean d = (c<4&&(c++<4));
System.out.println(d);
System.out.println(c);
}
}
-
位运算符:&,|,^,~,>>,<<,>>>
-
条件运算符?:
-
扩展赋值运算符:+=,-=,*=,/=
public class Demo06 {
public static void main(String[] args) {
/*
A = 0011 1100
B = 0000 1101
A&B = 0000 1100
A|B = 0011 1101
A^B = 0011 0001
~B = 1111 0010
2*8 = 16 2*2*2*2
效率极高!!!
<< *2
>> /2
0000 0000 0
0000 0001 1
0000 0010 2
0000 0100 4
0000 1000 8
0001 0000 16
*/
System.out.println(2<<3);
}
}
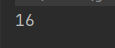
public class Demo07 {
public static void main(String[] args) {
int a = 10;
int b = 20;
a+=b;//a = a + b
a-=b;//a = a - b
System.out.println(a);
//字符串连接符 + , String
System.out.println(""+a+b);
System.out.println(a+b+"");
}
}
//三元运算符
public class Demo08 {
public static void main(String[] args) {
// x ? y : z
//x = true,则结果为y,否则结果为z
int score = 5;
String type = score <60 ?"不及格":"及格";//必须掌握
//if
System.out.println(type);
}
}