OOP第二次博客作业
关于Java与面向对象:
一、前言:
随着时间的变化,学期已经接近半个学期,作业题目集的难度也是一次比一次更大。并不会只局限于做一个小的程序编写了,不仅仅去考查一些基本的语法方面,更多的会是我们自己对题目中要实现的功能去进行自己的设计,包括类图,里面包含的属性等等。
到目前为止,对于自己的一些基本编写的能力还是有很多进行提高的,从第一次题目集总结到现在,有的时候真的会面对题目发愁,不想动手也不想动脑子思考,也是从这次作业开始,出现了有些题目没有去做的情况。也非常抱怨自己实在是没有努力去学习去攻克难关,在多数情况下都是懒惰的。当然自己的兴趣和天赋对计算机的学习可能真的没有啥吸引力,也很希望自己可以早点探索到学习此专业的一些好奇心和乐趣。
知识点:
1、Scanner类中nextLine()等方法
2、String类中split()等方法
3、Integer类中parseInt()等方法的用法
4、LocalDate类中of()、isAfter()、isBefore()、until()等方法的使用
5、ChronoUnit类中DAYS、WEEKS、MONTHS等单位的用法
6、Arraylist的基本使用方法
7、Java中哈希集(HashSet)概念,实现以及操作
8、正则表达式的基本使用
9、类与类之间的聚合关系
关于作业题量:
三次作业题目量还是相对第一次还是少了一些。
关于作业题目难度:
三次作业的题目难度偏大。
设计与分析:
第四次作业:总共有7道题目,分别为:
7-1 菜单计价程序-3 | 30 |
7-2 有重复的数据 | 10 |
7-3 去掉重复的数据 | 10 |
7-4 单词统计与排序 | 15 |
7-5 面向对象编程(封装性) | 10 |
7-6 GPS测绘中度分秒转换 | 10 |
7-7 判断两个日期的先后,计算间隔天数、周数 | 15 |
第四次作业主要让我见识到了hashset的使用、arraylist的使用等等,通过查询Java API文档,了解Scanner类中nextLine()等方法、String类中split()等方法、Integer类中parseInt()等方法的用法,了解LocalDate类中of()、isAfter()、isBefore()、until()等方法的使用规则,了解ChronoUnit类中DAYS、WEEKS、MONTHS等单位的用法。对于这些类的使用,不能体验出在Java当中有些自带的类可以轻松解决很多本需要自己手写去写的方法或者更好突破完成的功能。
比如hashset和array list的数组,使用他们的方法让我的程序源代码更加的简单清晰。比如去获取HashSet的概念、Java文档中HashSet的实现、HashSet的构造函数、HashSet()、 HashSet(int initialCapacity)、HashSet(int initialCapacity, float loadFactor)、HashSet(Collection)、HashSet的操作、添加元素add()、删除元素remove()、判断是否包含元素contains()、判断是否为空isEmpty()、获得大小size()、遍历HashSet、HashSet操作表格等知识。
以及了解到array list的重要性,Arraylist 是javase基础中重要的知识点,以后在工作中也可能常常用到。
第五次作业:总共有6道题目,分别为:
7-1 正则表达式训练-QQ号校验 | 5 |
7-2 字符串训练-字符排序 | 1 |
7-3 正则表达式训练-验证码校验 | 3 |
7-4 正则表达式训练-学号校验 | 7 |
7-5 日期问题面向对象设计(聚合一) | 50 |
7-6 日期问题面向对象设计(聚合二) | 34 |
第五次作业主要训练了我们的正则表达式的运用、类与类之间的聚合关系的处理聚合(Aggregation)关系表示整体与部分的关系。在聚合关系中,成员对象是整体的一部分,但是成员对象可以脱离整体对象独立存在。在UML中,聚合关系用带空心菱形的直线表示,如汽车(Car)与引擎(Engine)、轮胎(Wheel)、车灯(Light)。还有一题字符串的练习题,加深了对arrays的运用。
第六次作业:总共有1道题目,分别为:
7-1 菜单计价程序-4
|
100 |
第六次作业总共就一题,是在第四次的作业的7-1中进行的一个迭代。
去掉重复的数据:
这里使用到了的就是ArrayList
1 import java.util.ArrayList; 2 import java.util.Scanner; 3 4 public class Main{ 5 public static void main(String[] args){ 6 Scanner input = new Scanner(System.in); 7 int n = input.nextInt(); 8 if(n<1||n>100000){ 9 System.exit(0); 10 } 11 ArrayList<Integer> list = new ArrayList<>(); 12 for(int i = 0;i < n;i ++){ 13 int addx = input.nextInt(); 14 if(addx<1||addx>100000){ 15 System.exit(0); 16 } 17 list.add(addx); 18 } 19 for(int i = 0;i < list.size() - 1;i ++){ 20 for(int j = list.size() - 1;j > i;j --){ 21 if(list.get(j).equals(list.get(i))){ 22 list.remove(j); 23 } 24 } 25 } 26 for(int i = 0;i < list.size();i ++){ 27 if(i == list.size() - 1){ 28 System.out.println(list.get(i)); 29 }else{ 30 System.out.print(list.get(i) + " "); 31 } 32 } 33 } 34 }
有重复的数据:
1 import java.util.Scanner; 2 import java.util.HashSet; 3 4 public class Main { 5 6 public static void main(String[] args) { 7 try (// TODO Auto-generated method stub 8 Scanner input = new Scanner(System.in)) { 9 HashSet<Integer> list = new HashSet<Integer>(); 10 int key = 0; 11 int n = input.nextInt(); 12 if(n<1||n>100000){ 13 System.out.println("Error"); 14 System.exit(1); 15 } 16 if(n==1) { 17 System.out.println("NO"); 18 System.exit(0); 19 } 20 list.add(input.nextInt()); 21 for(int i = 1;i < n;i ++){ 22 if(list.add(input.nextInt())){ 23 key = 1; 24 continue; 25 }else{ 26 key = 0; 27 break; 28 } 29 } 30 if(key == 1){ 31 System.out.print("NO"); 32 }else{ 33 System.out.print("YES"); 34 } 35 } 36 } 37 }
题目集五的(7-5)(7-6)->日期问题面向对象设计:
参考题目7-2的要求,设计如下几个类:DateUtil、Year、Month、Day,其中年、月、日的取值范围依然为:year∈[1900,2050] ,month∈[1,12] ,day∈[1,31] , 两道题的设计类图如下:
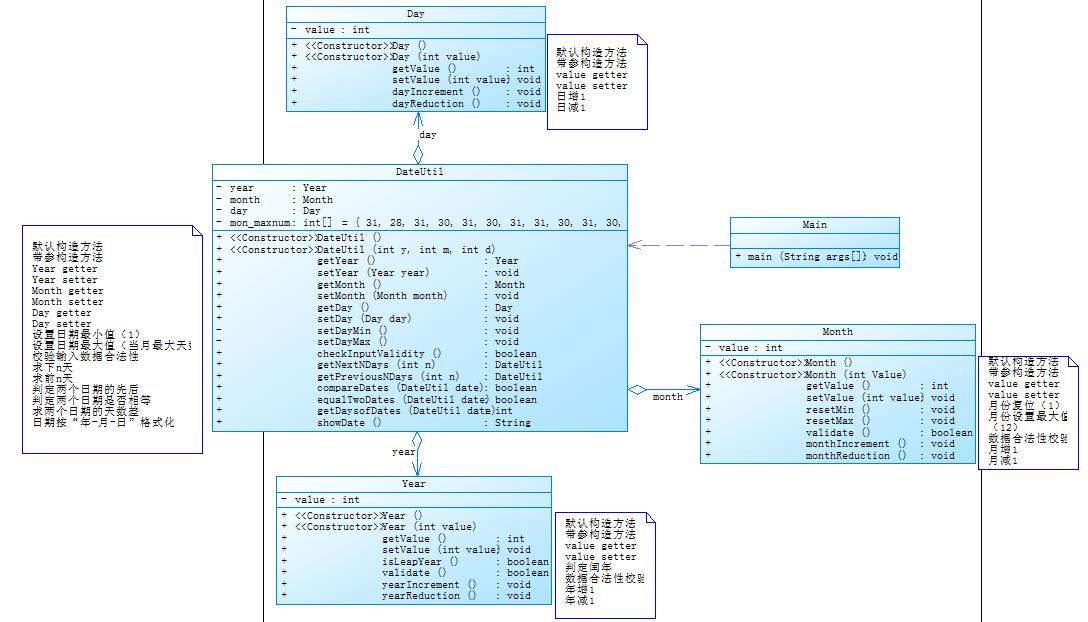
应用程序共测试三个功能:
- 求下n天
- 求前n天
- 求两个日期相差的天数
注意:严禁使用Java中提供的任何与日期相关的类与方法,并提交完整源码,包括主类及方法(已提供,不需修改)
输入格式:
有三种输入方式(以输入的第一个数字划分[1,3]):
- 1 year month day n //测试输入日期的下n天
- 2 year month day n //测试输入日期的前n天
- 3 year1 month1 day1 year2 month2 day2 //测试两个日期之间相差的天数
输出格式:
- 当输入有误时,输出格式如下:
Wrong Format
- 当第一个数字为1且输入均有效,输出格式如下:
year-month-day
- 当第一个数字为2且输入均有效,输出格式如下:
year-month-day
- 当第一个数字为3且输入均有效,输出格式如下:
天数值
输入样例1:
在这里给出一组输入。例如:
3 2014 2 14 2020 6 14
输出样例1:
在这里给出相应的输出。例如:
2312
输入样例2:
在这里给出一组输入。例如:
2 1935 2 17 125340
输出样例2:
在这里给出相应的输出。例如:
1591-12-17
输入样例3:
在这里给出一组输入。例如:
1 1999 3 28 6543
输出样例3:
在这里给出相应的输出。例如:
2017-2-24
输入样例4:
在这里给出一组输入。例如:
0 2000 5 12 30
输出样例4:
在这里给出相应的输出。例如:
Wrong Format
代码如下:
7-5
1 import java.util.Scanner; 2 3 public class Main { 4 public static void main(String[] args) { 5 Scanner input = new Scanner(System.in); 6 int year = 0; 7 int month = 0; 8 int day = 0; 9 10 int choice = input.nextInt(); 11 12 if (choice == 1) { // test getNextNDays method 13 int m = 0; 14 year = Integer.parseInt(input.next()); 15 month = Integer.parseInt(input.next()); 16 day = Integer.parseInt(input.next()); 17 18 DateUtil date = new DateUtil(year, month, day); 19 20 if (!date.checkInputValidity()) { 21 System.out.println("Wrong Format"); 22 System.exit(0); 23 } 24 25 m = input.nextInt(); 26 27 if (m < 0) { 28 System.out.println("Wrong Format"); 29 System.exit(0); 30 } 31 32 System.out.println(date.getNextNDays(m).showDate()); 33 } else if (choice == 2) { // test getPreviousNDays method 34 int n = 0; 35 year = Integer.parseInt(input.next()); 36 month = Integer.parseInt(input.next()); 37 day = Integer.parseInt(input.next()); 38 39 DateUtil date = new DateUtil(year, month, day); 40 41 if (!date.checkInputValidity()) { 42 System.out.println("Wrong Format"); 43 System.exit(0); 44 } 45 46 n = input.nextInt(); 47 48 if (n < 0) { 49 System.out.println("Wrong Format"); 50 System.exit(0); 51 } 52 53 System.out.println(date.getPreviousNDays(n).showDate()); 54 } else if (choice == 3) { // test getDaysofDates method 55 year = Integer.parseInt(input.next()); 56 month = Integer.parseInt(input.next()); 57 day = Integer.parseInt(input.next()); 58 59 int anotherYear = Integer.parseInt(input.next()); 60 int anotherMonth = Integer.parseInt(input.next()); 61 int anotherDay = Integer.parseInt(input.next()); 62 63 DateUtil fromDate = new DateUtil(year, month, day); 64 DateUtil toDate = new DateUtil(anotherYear, anotherMonth, anotherDay); 65 66 if (fromDate.checkInputValidity() && toDate.checkInputValidity()) { 67 int k = fromDate.getDaysofDates(toDate); 68 System.out.println(k); 69 } else { 70 System.out.println("Wrong Format"); 71 System.exit(0); 72 } 73 } else { 74 System.out.println("Wrong Format"); 75 System.exit(0); 76 } 77 } 78 } 79 80 class Year{ 81 int value; 82 83 public Year() { 84 } 85 86 public Year(int value) { 87 this.value = value; 88 } 89 90 public int getValue() { 91 return value; 92 } 93 94 public void setValue(int value) { 95 this.value = value; 96 } 97 98 public boolean isLeapYear(){ 99 if((value%4 == 0&&value % 100 != 0)||value % 400 == 0){ 100 return true; 101 }else{ 102 return false; 103 } 104 } 105 106 public boolean isLeapYear(int a){ 107 if((a%4 == 0&&a % 100 != 0)||a % 400 == 0){ 108 return true; 109 }else{ 110 return false; 111 } 112 } 113 public boolean validate(){ 114 if(value <=2020 &&value >= 1820) 115 return true; 116 else 117 return false; 118 } 119 120 public void yearIncrement(){ 121 value += 1; 122 } 123 124 public void yearReduction(){ 125 value -= 1; 126 } 127 } 128 129 class Month{ 130 int value; 131 Year year; 132 public Month(){ 133 } 134 135 public Month(int yearValue,int monthValue){ 136 this.year = new Year(yearValue); 137 this.value = monthValue; 138 } 139 140 public int getValue() { 141 return value; 142 } 143 144 public void setValue(int value) { 145 this.value = value; 146 } 147 148 149 public Year getYear() { 150 return year; 151 } 152 153 public void setYear(Year year) { 154 this.year = year; 155 } 156 157 public void resetMin(){ 158 value = 1; 159 } 160 161 public void resetMax(){ 162 value = 12; 163 } 164 165 public boolean validate(){ 166 if(value >= 1&&value <= 12) 167 return true; 168 else 169 return false; 170 } 171 172 public void monthIncrement(){ 173 value += 1; 174 } 175 176 public void monthReduction(){ 177 value -= 1; 178 } 179 } 180 class Day{ 181 int value; 182 int[] mon_maxnum = {0, 31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31}; 183 Month month; 184 public Day(){ 185 } 186 187 public Day(int yearValue,int monthValue,int dayValue){ 188 this.month = new Month(yearValue,monthValue); 189 this.value = dayValue; 190 } 191 192 public int getValue(){ 193 return value; 194 } 195 196 public void setValue(int value){ 197 this.value=value; 198 } 199 200 public Month getMonth(){ 201 return month; 202 } 203 204 public void setMonth(Month value){ 205 this.month = value; 206 } 207 public void resetMin() { 208 value = 1; 209 } 210 public void resetMax() { 211 value = mon_maxnum[month.getValue()]; 212 } 213 public boolean validate(){ 214 if(this.getMonth().getYear().isLeapYear(value)) 215 mon_maxnum[1]=29; 216 if(value >= 1&&value <= mon_maxnum[month.getValue()]) 217 return true; 218 else 219 return false; 220 } 221 222 public void dayIncrement() { 223 value=value+1; 224 } 225 226 public void dayReduction() { 227 value=value-1; 228 } 229 } 230 231 class DateUtil{ 232 Day day; 233 public DateUtil() { }; 234 public DateUtil(int y,int m,int d) { 235 this.day = new Day(y,m,d); 236 } 237 238 public Day getDay() { 239 return day; 240 } 241 242 public void setDay(Day d) { 243 this.day = d; 244 } 245 246 public boolean checkInputValidity(){ 247 if(this.getDay().getMonth().getYear().validate()&&this.getDay().getMonth().validate()&&day.validate()) 248 return true; 249 else 250 return false; 251 } 252 253 public boolean compareDates(DateUtil date) { 254 if(date.getDay().getMonth().getYear().getValue()<this.getDay().getMonth().getYear().getValue()) 255 return false; 256 else if(date.getDay().getMonth().getYear().getValue()==this.getDay().getMonth().getYear().getValue()&&date.getDay().getMonth().getValue()<this.getDay().getMonth().getValue()) 257 return false; 258 else if(date.getDay().getMonth().getYear().getValue()==this.getDay().getMonth().getYear().getValue()&&date.getDay().getMonth().getValue()==this.getDay().getMonth().getValue()&&date.getDay().getValue()<this.getDay().getValue()) 259 return false; 260 else 261 return true; 262 } 263 264 public boolean equalTwoDates(DateUtil date){ 265 if(this.getDay().getValue()==date.getDay().getValue()&&this.getDay().getMonth().getValue()==date.getDay().getMonth().getValue()&& this.getDay().getMonth().getYear().getValue()==date.getDay().getMonth().getYear().getValue()) 266 return true; 267 else 268 return false; 269 } 270 271 public DateUtil getNextNDays(int n){ 272 int mon_maxnum[]={0,31,28,31,30,31,30,31,31,30,31,30,31}; 273 while(n > 365){ 274 if(this.getDay().getMonth().getYear().isLeapYear()&&this.getDay().getMonth().getValue()<=2){ 275 if(this.getDay().getValue()==29&&this.getDay().getMonth().getValue()==2){ 276 this.getDay().resetMin(); 277 this.getDay().getMonth().setValue(3); 278 } 279 this.getDay().getMonth().getYear().yearIncrement(); 280 n -= 366; 281 }else if(this.getDay().getMonth().getYear().isLeapYear(this.getDay().getMonth().getYear().getValue()+1)&&this.getDay().getMonth().getValue()>2){ 282 this.getDay().getMonth().getYear().yearIncrement(); 283 n -= 366; 284 }else{ 285 this.getDay().getMonth().getYear().yearIncrement(); 286 n -= 365; 287 } 288 } 289 for(int i = 0;i < n;i ++){ 290 this.getDay().dayIncrement(); 291 if(this.getDay().getMonth().getYear().isLeapYear() && this.getDay().getMonth().getValue() == 2){ 292 if(this.getDay().getValue() > 29){ 293 this.getDay().getMonth().monthIncrement(); 294 this.getDay().resetMin(); 295 } 296 } 297 else if(this.getDay().getValue() > mon_maxnum[this.getDay().getMonth().getValue()]){ 298 this.getDay().getMonth().monthIncrement(); 299 this.getDay().resetMin(); 300 if(this.getDay().getMonth().getValue() > 12){ 301 this.getDay().getMonth().resetMin(); 302 this.getDay().getMonth().getYear().yearIncrement(); 303 } 304 } 305 } 306 return new DateUtil(this.getDay().getMonth().getYear().getValue(), this.getDay().getMonth().getValue(), this.getDay().getValue()); 307 } 308 public DateUtil getPreviousNDays(int n){ 309 int mon_maxnum[]={0,31,28,31,30,31,30,31,31,30,31,30,31}; 310 while(n>365){ 311 if(this.getDay().getMonth().getYear().isLeapYear()&&this.getDay().getMonth().getValue()>2){ 312 this.getDay().getMonth().getYear().yearReduction(); 313 n -= 366; 314 }else if(this.getDay().getMonth().getYear().isLeapYear(this.getDay().getMonth().getYear().getValue() - 1)&&this.getDay().getMonth().getValue() <= 2){ 315 this.getDay().getMonth().getYear().yearReduction(); 316 n -= 366; 317 }else{ 318 this.getDay().getMonth().getYear().yearReduction(); 319 n -= 365; 320 } 321 } 322 for(int i=0;i<n;i++){ 323 this.getDay().dayReduction(); 324 if(this.getDay().getValue() < 1){ 325 this.getDay().getMonth().monthReduction(); 326 if(this.getDay().getMonth().getValue() < 1){ 327 this.getDay().getMonth().resetMax(); 328 this.getDay().getMonth().getYear().yearReduction(); 329 } 330 if(this.getDay().getMonth().getYear().isLeapYear()&&this.getDay().getMonth().getValue() == 2){ 331 this.getDay().setValue(29); 332 }else{ 333 this.getDay().resetMax(); 334 } 335 } 336 } 337 return new DateUtil(this.getDay().getMonth().getYear().getValue(), this.getDay().getMonth().getValue(), this.getDay().getValue()); 338 } 339 public int getDaysofDates(DateUtil date){ 340 DateUtil b1 = this; 341 DateUtil b2 = date; 342 if(this.equalTwoDates(date)){//如果两天的日期相等 343 return 0; 344 } 345 else if(!this.compareDates(date)){//如果日期大小不对 346 b1 = date; 347 b2 = this; 348 } 349 int mon_maxnum[] = {0,31,28,31,30,31,30,31,31,30,31,30,31}; 350 int i ,j ,ts = 0; 351 for(i = b1.getDay().getMonth().getYear().getValue() + 1;i < b2.getDay().getMonth().getYear().getValue();i++){//两个日期的年数之和 352 ts = ts+365; 353 if(new Year(i).isLeapYear()) 354 ts ++; 355 } 356 if(b1.getDay().getMonth().getYear().getValue() == b2.getDay().getMonth().getYear().getValue()&&b1.getDay().getMonth().getValue() == b2.getDay().getMonth().getValue()){//年份相同,月份相同,日不同 357 ts = b2.getDay().getValue()-b1.getDay().getValue(); 358 } 359 else if(b1.getDay().getMonth().getYear().getValue() == b2.getDay().getMonth().getYear().getValue()&&b1.getDay().getMonth().getValue() != b2.getDay().getMonth().getValue()){//年份相同,月份不同 360 if(b1.getDay().getMonth().getYear().isLeapYear())//是闰年 361 mon_maxnum[2] = 29; 362 ts = ts + mon_maxnum[b1.getDay().getMonth().getValue()]-b1.getDay().getValue();//小日期该月剩余的天数 363 ts = ts + b2.getDay().getValue();//大日期的天数 364 for(j=b1.getDay().getMonth().getValue()+1;j<=b2.getDay().getMonth().getValue()-1;j++)//月份天数和 365 ts += mon_maxnum[j]; 366 } 367 else if(b1.getDay().getMonth().getYear().getValue()!=b2.getDay().getMonth().getYear().getValue()){//年份不同 368 ts = ts + mon_maxnum[b1.getDay().getMonth().getValue()]-b1.getDay().getValue();//小日期在该月剩余的天数 369 ts = ts + b2.getDay().getValue();//大日期在该月已经过的天数 370 for(j=b1.getDay().getMonth().getValue()+1;j<=12;j++)//小日期在该年剩余的天数 371 ts = ts + mon_maxnum[j]; 372 for(j = b2.getDay().getMonth().getValue()-1;j>0;j--)//大日期在该年已经过的天数 373 ts = ts + mon_maxnum[j]; 374 if(b1.getDay().getMonth().getYear().isLeapYear()&&b1.getDay().getMonth().getValue()<=2)//如果小日期该年为闰年且该天在1月或2月 375 ts++; 376 if(b2.getDay().getMonth().getYear().isLeapYear()&&b2.getDay().getMonth().getValue()>2)//如果大日期该年为闰年且该天在1月或2月后 377 ts++; 378 } 379 return ts; 380 } 381 382 public String showDate(){ 383 return this.getDay().getMonth().getYear().getValue()+"-"+this.getDay().getMonth().getValue()+"-"+this.getDay().getValue(); 384 } 385 }
7-6:
1 import java.util.Scanner; 2 3 public class Main { 4 public static void main(String[] args) { 5 Scanner input = new Scanner(System.in); 6 int year = 0; 7 int month = 0; 8 int day = 0; 9 10 int choice = input.nextInt(); 11 12 if (choice == 1) { // test getNextNDays method 13 int m = 0; 14 year = Integer.parseInt(input.next()); 15 month = Integer.parseInt(input.next()); 16 day = Integer.parseInt(input.next()); 17 18 DateUtil date = new DateUtil(year, month, day); 19 20 if (!date.checkInputValidity()) { 21 System.out.println("Wrong Format"); 22 System.exit(0); 23 } 24 25 m = input.nextInt(); 26 27 if (m < 0) { 28 System.out.println("Wrong Format"); 29 System.exit(0); 30 } 31 System.out.print(year+"-"+month+"-"+day+" next "+ m + " days is:"); 32 System.out.println(date.getNextNDays(m).showDate()); 33 } else if (choice == 2) { // test getPreviousNDays method 34 int n = 0; 35 year = Integer.parseInt(input.next()); 36 month = Integer.parseInt(input.next()); 37 day = Integer.parseInt(input.next()); 38 39 DateUtil date = new DateUtil(year, month, day); 40 41 if (!date.checkInputValidity()) { 42 System.out.println("Wrong Format"); 43 System.exit(0); 44 } 45 46 n = input.nextInt(); 47 48 if (n < 0) { 49 System.out.println("Wrong Format"); 50 System.exit(0); 51 } 52 System.out.print(year+"-"+month+"-"+day+" previous "+ n +" days is:"); 53 System.out.println(date.getPreviousNDays(n).showDate()); 54 } else if (choice == 3) { // test getDaysofDates method 55 year = Integer.parseInt(input.next()); 56 month = Integer.parseInt(input.next()); 57 day = Integer.parseInt(input.next()); 58 59 int anotherYear = Integer.parseInt(input.next()); 60 int anotherMonth = Integer.parseInt(input.next()); 61 int anotherDay = Integer.parseInt(input.next()); 62 63 DateUtil fromDate = new DateUtil(year, month, day); 64 DateUtil toDate = new DateUtil(anotherYear, anotherMonth, anotherDay); 65 66 if (fromDate.checkInputValidity() && toDate.checkInputValidity()) { 67 int k = fromDate.getDaysofDates(toDate); 68 System.out.print("The days between "+year+"-"+month+"-"+day+" and "+anotherYear+"-"+anotherMonth+"-"+anotherDay+" are:"); 69 System.out.print(k); 70 } else { 71 System.out.println("Wrong Format"); 72 System.exit(0); 73 } 74 } else { 75 System.out.println("Wrong Format"); 76 System.exit(0); 77 } 78 } 79 } 80 81 class Year{ 82 int value; 83 84 public Year() { 85 } 86 87 public Year(int value) { 88 this.value = value; 89 } 90 91 public int getValue() { 92 return value; 93 } 94 95 public void setValue(int value) { 96 this.value = value; 97 } 98 99 public boolean isLeapYear(){ 100 if((value%4 == 0&&value % 100 != 0)||value % 400 == 0){ 101 return true; 102 }else{ 103 return false; 104 } 105 } 106 107 public boolean isLeapYear(int a){ 108 if((a%4 == 0&&a % 100 != 0)||a % 400 == 0){ 109 return true; 110 }else{ 111 return false; 112 } 113 } 114 public boolean validate(){ 115 if(value <=2020 &&value >= 1820) 116 return true; 117 else 118 return false; 119 } 120 121 public void yearIncrement(){ 122 value += 1; 123 } 124 125 public void yearReduction(){ 126 value -= 1; 127 } 128 } 129 130 class Month{ 131 int value; 132 public Month(){ 133 } 134 135 public Month(int value){ 136 this.value = value; 137 } 138 139 public int getValue() { 140 return value; 141 } 142 143 public void setValue(int value) { 144 this.value = value; 145 } 146 147 public void resetMin(){ 148 value = 1; 149 } 150 151 public void resetMax(){ 152 value = 12; 153 } 154 155 public boolean validate(){ 156 if(value >= 1&&value <= 12) 157 return true; 158 else 159 return false; 160 } 161 162 public void monthIncrement(){ 163 value += 1; 164 } 165 166 public void monthReduction(){ 167 value -= 1; 168 } 169 } 170 class Day{ 171 int value; 172 public Day(){ 173 } 174 175 public Day(int value){ 176 this.value = value; 177 } 178 179 public int getValue(){ 180 return value; 181 } 182 183 public void setValue(int value){ 184 this.value=value; 185 } 186 187 public void resetMin(){ 188 value=1; 189 } 190 191 public void dayIncrement() { 192 value=value+1; 193 } 194 195 public void dayReduction() { 196 value=value-1; 197 } 198 } 199 200 class DateUtil{ 201 Year year; 202 Month month; 203 Day day; 204 int mon_maxnum[]={0,31,28,31,30,31,30,31,31,30,31,30,31}; 205 public DateUtil() { }; 206 public DateUtil(int y,int m,int d) { 207 this.year = new Year(y); 208 this.month = new Month(m); 209 this.day = new Day(d); 210 } 211 212 public Day getDay() { 213 return day; 214 } 215 216 public void setDay(Day d) { 217 this.day = d; 218 } 219 220 public Year getYear() { 221 return year; 222 } 223 public void setYear(Year year) { 224 this.year = year; 225 } 226 public Month getMonth() { 227 return month; 228 } 229 public void setMonth(Month month) { 230 this.month = month; 231 } 232 public void setDayMin(){ 233 234 } 235 public void setDayMax(){ 236 237 } 238 public boolean checkInputValidity(){ 239 if(year.isLeapYear()) { 240 mon_maxnum[2] = 29; 241 } 242 if(this.getYear().validate()&&this.getMonth().validate()&&day.getValue()>0 &&day.getValue()<mon_maxnum[month.getValue()]) { 243 return true; 244 }else { 245 return false; 246 } 247 } 248 249 public boolean compareDates(DateUtil date) { 250 if(date.getYear().getValue() < this.getYear().getValue()) 251 return false; 252 else if(date.getYear().getValue() == this.getYear().getValue()&& 253 date.getMonth().getValue() < this.getMonth().getValue()) 254 return false; 255 else if(date.getYear().getValue() == this.getYear().getValue()&& 256 date.getMonth().getValue() == this.getMonth().getValue()&&date.getDay().getValue()<this.getDay().getValue()) 257 return false; 258 else 259 return true; 260 } 261 262 public boolean equalTwoDates(DateUtil date){ 263 if(this.getDay().getValue()==date.getDay().getValue()&&this.getMonth().getValue()==date.getMonth().getValue()&& this.getYear().getValue()==date.getYear().getValue()) 264 return true; 265 else 266 return false; 267 } 268 269 public DateUtil getNextNDays(int n){ 270 int mon_maxnum[]={0,31,28,31,30,31,30,31,31,30,31,30,31}; 271 while(n > 365){ 272 if(this.getYear().isLeapYear()&&this.getMonth().getValue()<=2){ 273 if(this.getDay().getValue()==29&&this.getMonth().getValue()==2){ 274 this.getDay().resetMin(); 275 this.getMonth().setValue(3); 276 } 277 this.getYear().yearIncrement(); 278 n -= 366; 279 }else if(this.getYear().isLeapYear(this.getYear().getValue()+1)&&this.getMonth().getValue()>2){ 280 this.getYear().yearIncrement(); 281 n -= 366; 282 }else{ 283 this .getYear().yearIncrement(); 284 n -= 365; 285 } 286 } 287 for(int i = 0;i < n;i ++){ 288 this.getDay().dayIncrement(); 289 if(this.getYear().isLeapYear() && this.getMonth().getValue() == 2){ 290 if(this.getDay().getValue() > 29){ 291 this.getMonth().monthIncrement(); 292 this.getDay().resetMin(); 293 } 294 } 295 else if(this.getDay().getValue() > mon_maxnum[this.getMonth().getValue()]){ 296 this.getMonth().monthIncrement(); 297 this.getDay().resetMin(); 298 if(this.getMonth().getValue() > 12){ 299 this.getMonth().resetMin(); 300 this.getYear().yearIncrement(); 301 } 302 } 303 } 304 return new DateUtil(this.getYear().getValue(), this.getMonth().getValue(), this.getDay().getValue()); 305 } 306 public DateUtil getPreviousNDays(int n){ 307 while(n>365){ 308 if(this.getYear().isLeapYear()&&this.getMonth().getValue()>2){ 309 this.getYear().yearReduction(); 310 n -= 366; 311 }else if(this.getYear().isLeapYear(this.getYear().getValue() - 1)&&this.getMonth().getValue() <= 2){ 312 this.getYear().yearReduction(); 313 n -= 366; 314 }else{ 315 this.getYear().yearReduction(); 316 n -= 365; 317 } 318 } 319 for(int i=0;i<n;i++){ 320 this.getDay().dayReduction(); 321 if(this.getDay().getValue() < 1){ 322 this.getMonth().monthReduction(); 323 if(this.getMonth().getValue() < 1){ 324 this.getMonth().resetMax(); 325 this.getYear().yearReduction(); 326 } 327 if(this.getYear().isLeapYear()&&this.getMonth().getValue() == 2){ 328 this.getDay().setValue(29); 329 }else{ 330 this.getDay().setValue(mon_maxnum[this.getMonth().getValue()]);; 331 } 332 } 333 } 334 return new DateUtil(this.getYear().getValue(), this.getMonth().getValue(), this.getDay().getValue()); 335 } 336 public int getDaysofDates(DateUtil date){ 337 DateUtil b1 = this; 338 DateUtil b2 = date; 339 if(this.equalTwoDates(date)){//如果两天的日期相等 340 return 0; 341 } 342 else if(!this.compareDates(date)){//如果日期大小不对 343 b1 = date; 344 b2 = this; 345 } 346 int mon_maxnum[] = {0,31,28,31,30,31,30,31,31,30,31,30,31}; 347 int i ,j ,ts = 0; 348 for(i = b1.getYear().getValue() + 1;i < b2.getYear().getValue();i++){//两个日期的年数之和 349 ts = ts+365; 350 if(new Year(i).isLeapYear()) 351 ts ++; 352 } 353 if(b1.getYear().getValue() == b2.getYear().getValue()&&b1.getMonth().getValue() == b2.getMonth().getValue()){//年份相同,月份相同,日不同 354 ts = b2.getDay().getValue()-b1.getDay().getValue(); 355 } 356 else if(b1.getYear().getValue() == b2.getYear().getValue()&&b1.getMonth().getValue() != b2.getMonth().getValue()){//年份相同,月份不同 357 if(b1.getYear().isLeapYear())//是闰年 358 mon_maxnum[2] = 29; 359 ts = ts + mon_maxnum[b1.getMonth().getValue()]-b1.getDay().getValue();//小日期该月剩余的天数 360 ts = ts + b2.getDay().getValue();//大日期的天数 361 for(j=b1.getDay().getValue()+1;j<=b2.getMonth().getValue()-1;j++)//月份天数和 362 ts += mon_maxnum[j]; 363 } 364 else if(b1.getYear().getValue()!=b2.getYear().getValue()){//年份不同 365 ts = ts + mon_maxnum[b1.getMonth().getValue()]-b1.getDay().getValue();//小日期在该月剩余的天数 366 ts = ts + b2.getDay().getValue();//大日期在该月已经过的天数 367 for(j=b1.getMonth().getValue()+1;j<=12;j++)//小日期在该年剩余的天数 368 ts = ts + mon_maxnum[j]; 369 for(j = b2.getMonth().getValue()-1;j>0;j--)//大日期在该年已经过的天数 370 ts = ts + mon_maxnum[j]; 371 if(b1.getYear().isLeapYear()&&b1.getMonth().getValue()<=2)//如果小日期该年为闰年且该天在1月或2月 372 ts++; 373 if(b2.getYear().isLeapYear()&&b2.getMonth().getValue()>2)//如果大日期该年为闰年且该天在1月或2月后 374 ts++; 375 } 376 return ts; 377 } 378 379 public String showDate(){ 380 return this.getYear().getValue()+"-"+this.getMonth().getValue()+"-"+this.getDay().getValue(); 381 } 382 }
这两道题在前面的题目集3(7-4)日期类设计做过,但这次根据类图来敲写代码,两道题的区别体现在聚合一是一个类跟另一个的套娃性质的链状结构聚合,Month和Year之间是聚合关系,Day和Month之间是聚合关系,DateUtil和Day之间是聚合关系但是聚合二是一个类和其他所有类的聚合DateUtil和Day,Month,Year。主要就是把之前的题目集3的初级代码再次经过修改去实现代码的美化,从而体现本次作业需要去实现的类与类之间的关系。将YEAR、MONTH、DAY本在一个类中的私有属性单独生成各自对应的类,从这次的修改中发现并不会觉得多了几个类是负担不好用,反而是更加的舒服。
踩坑心得:
对于菜单的训练深刻体会到,在自己设计一个相对复杂的工程时,必须先把每一个地方需要的方法以及类与类之间的联系弄清楚,再来进行代码的填充。不然在等代码敲写完之后再发现原本建立好的并不能实现所需功能。
改进建议:
将菜单的类与类之间联系重新弄过一遍,删除过于鸡肋的类,分解为多个。
总结:
本三次题目集学会了正则表达式的基本运用,知道了原来输入的数据可以靠这些来进行限制以及判断。同时接触到了Array List的使用方法,在需要有多个数据的时候可以考虑使用此类去完成。其他的关于类与类之间的关系,需要总结自己需要在什么情况的时候去使用。