注册表分类
1.HKEY_CLASS_ROOT 主键:该主键用于保存系统中注册的各类文件的控制名以及文件关信息。如xmind

2.HKEY_CURRENT_USER 主键:该主键用于保存登录到系统的当前用户的计算机的环境变量、桌面设置、应用程序设置、个人程序组、打印机和网络连接等、
3.HKEY_LOCAL_MACHINE 主键:该主键保存了计算机的硬件、软件及操作系统中的配置信息,例如硬件状态、外部设备、网络设备、软件的安装及设置
4.HKEY_USERS 主键:该主键用于保存计算机所有用户的配置信息
5.HKEY_CURRENT_CONFIG主键:该主键用于存放当前计算机的硬件配置信息,此主键实际上是HKEY_LOCAL_MACHINE 中的一部分,它的子键与HKEY_LCOAL_MACHINE\Config\0001分支下的数据完全相同
Registry 和RegistryKey 类
1.Registry类
Registry类是个静态类,不能被实例化,它的作用只是实例化RegistryKey类,以便开始在注册表中浏览信息。通过该类的只读字段提供 RegistryKey实例,这些字段共有7个
字段 |
说明 |
ClassRoot |
定义文档的类型(或类)以及与哪些类型关联的属性,该字段读取 Windows注册表项HKEY_CLASSES_ROOT |
CurrentConfig |
包含有关非用户特定的硬件配置信息。该字段读取Windows注册表基项HKEY_CURRENT_CONFIG |
CurrentUser |
包含有关当前用户首选项的信息。该字段读取Windows 注册表基项HKEY_CURRENT_USER |
DynData |
包含动态注册数据。该字段读取Windows 注册表基项HKEY_DYN_DATA |
LocalMachine |
包含本地计算机的配置数据。该字段读取Windows 注册表基项HKEY_LOCAL_MACHINE |
PerformanceData |
包含软件组件的性能信息。该字段读取Windows注册基项HKEY_PERFORMANCE_DATA |
User |
包含有关默认用户配置的信息。该字段读取Windows注册表基项HKEY_USERS |
说明
RegistryKey rk=Registry.LocalMachine; //表示要获得一个HKLM键的RegistryKey实例
2. RegistryKey
RegistryKey 实例表示一个注册表项,这个类的方法可以浏览子健、创建子健、创建新键、读取或修改注册表中的值。也就是说该类可以完成对注册表的操作,除了设置键的安全级别之外。
RegistryKey 类的常用属性说明
属性 |
说明 |
Name |
检索项的名称 |
SubKeyCount |
检索当前项的子项数目 |
ValueCount |
检索项中值的计数 |
RegistryKey 类的常用方法说明
方法 |
说明 |
Close |
关闭键 |
CreateSubKey |
创建指定名称的子键(如果该键已存在就打开它) |
DeleteSubKey |
删除指定子键 |
DeleteSubKeyTree |
彻底删除子键及其所有包含子键 |
DeleteValue |
从键中删除一个指定的值 |
GetSubKeyNames |
返回包含子键名称的字符串数组 |
GetValue |
返回指定的值 |
GetValueNames |
返回一个包含所有键值名称的字符串数组 |
OpenSubKey |
返回表示给定子键的RegistryKey 实例引用 |
SetValue |
设置指定的值 |
Code
using Microsoft.Win32;
using System.Text;
namespace ConsoleApp1
{
internal class Program
{
static void Main(string[] args)
{
StringBuilder sb=new StringBuilder();
RegistryKey rk = Registry.CurrentUser; //创建RegistryKey 实例
RegistryKey rkChild = rk.OpenSubKey(@"Software"); //使用OpenSubKey 方法打开HKEY_LOCAL_USER\Software 子项
foreach (string item in rkChild.GetSubKeyNames()) //遍历检索该子项下所有的子项名称
{
sb.AppendLine($"子项名称:{item}");
foreach(string strVName in rkChild.GetValueNames()) {
sb.AppendLine($"****子项名称:{strVName}");
}
}
Console.WriteLine( sb.ToString() );
rk.Close();
Console.ReadKey();
}
}
}
创建注册表
using Microsoft.Win32;
namespace ConsoleApp2
{
internal class Program
{
static void Main(string[] args)
{
try {
RegistryKey rk = Registry.CurrentUser;
RegistryKey rkSoftware = rk.OpenSubKey("Software",true);
RegistryKey rkMyKey = rkSoftware.CreateSubKey("我的测试键");
RegistryKey rkFirst = rkMyKey.CreateSubKey("子项键");
rkFirst.SetValue("MyChildKey","123");
Console.WriteLine("注册表创建成功!");
}
catch (Exception ex)
{
Console.WriteLine("注册表创建异常");
}
Console.ReadKey();
}
}
}
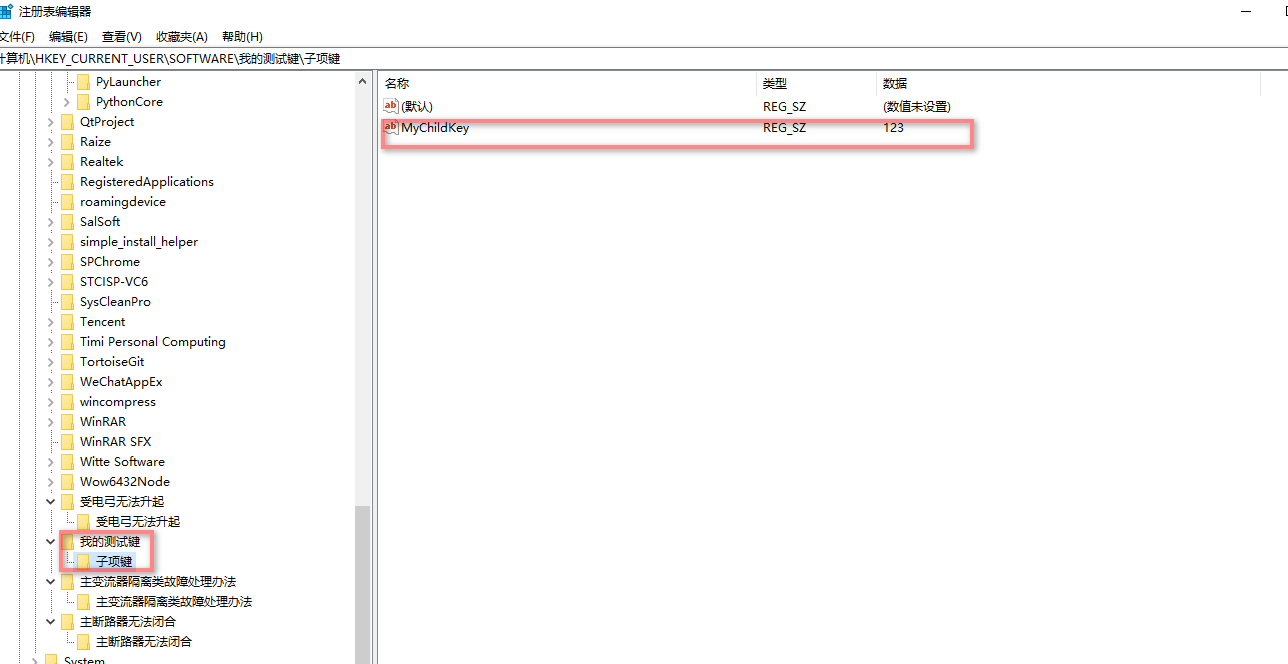
修改注册表
using Microsoft.Win32;
namespace ConsoleApp3
{
internal class Program
{
static void Main(string[] args)
{
RegistryKey rk = Registry.CurrentUser;
RegistryKey rkSoftWare = rk.OpenSubKey("Software", true);//第一个参数表示子键名,第二个参数表示打开方式 false 只读 true 读写
RegistryKey rkMyKey = rkSoftWare.OpenSubKey("我的测试键", true);
RegistryKey rkChild = rkMyKey.OpenSubKey("子项键",true);
rkChild.SetValue("MyChildKey","修改后的值321");
Console.WriteLine("注册表修改成功!");
rk.Close();
Console.ReadKey();
}
}
}
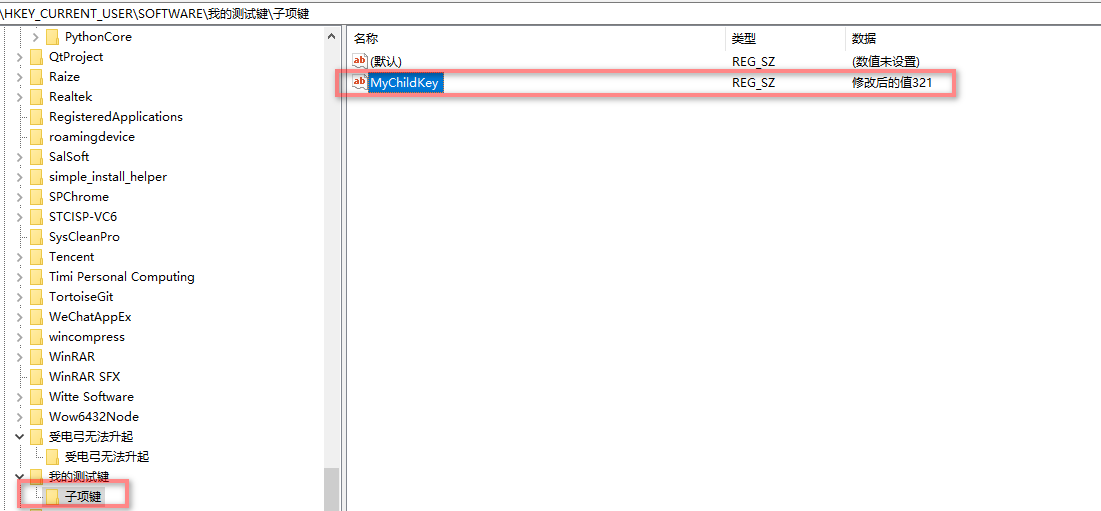
删除注册表
using Microsoft.Win32;
namespace ConsoleApp4
{
internal class Program
{
static void Main(string[] args)
{
RegistryKey rk = Registry.CurrentUser;
RegistryKey myKey = rk.OpenSubKey("Software", true);
myKey.DeleteSubKeyTree("我的测试键",false);
rk.Close();
Console.WriteLine("删除注册表成功");
Console.ReadKey();
}
}
}
综合小实例-注册机编写
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using Microsoft.Win32;
using Sunny.UI;
using Sunny.UI.Win32;
namespace WindowsFormsApp1
{
public partial class Form1 : UIForm
{
public Form1()
{
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e)
{
RegistryKey retKey = Registry.LocalMachine.OpenSubKey("Software", true)
.CreateSubKey("mywxk")
.CreateSubKey("mywxk.ini");
uiTextBox1.Text = SoftReg.getRNum();
foreach (string strName in retKey.GetSubKeyNames()) {
if(strName ==SoftReg.getRNum())
{
Text = "限制软件使用次数(已注册)";
uiButton1.Enabled = false;
return;
}
}
Text = "限制软件使用次数(软件未注册)";
uiButton1.Enabled = true;
MessageBox.Show("您现在使用的是试用版,该软件可以免费使用30次!","提示",MessageBoxButtons.OK,MessageBoxIcon.Information);
Int32 tLong=0;
try
{
RegistryKey rkSoftware = Registry.LocalMachine.OpenSubKey("Software");
// tLong = (Int32)Registry.GetValue("HKEY_LOCAL_MACHINE\\SOFTWARE\\tryTimes","UseTimes",0);
foreach (string item in rkSoftware.GetSubKeyNames())
{
if (item == "tryTimes")
{
break;
}
else
{
RegistryKey rkW = Registry.LocalMachine.OpenSubKey("Software", true).CreateSubKey("tryTimes");
RegistryKey rkSet= rkW.CreateSubKey("tryTimes", true);
rkSet.SetValue("UseTimes", 0);
}
}
tLong = (Int32)rkSoftware.OpenSubKey("tryTimes", false).GetValue("UseTimes");//获取该软件使用次数
MessageBox.Show($"您已使用了{tLong}次","提示",MessageBoxButtons.OK,MessageBoxIcon.Information);
}
catch {
Registry.SetValue("HKEY_LOCAL_MACHINE\\SOFTWARE\\tryTimes", "UseTimes", 0,RegistryValueKind.DWord);
//首次使用软件
}
tLong = (Int32)Registry.GetValue("HKEY_LOCAL_MACHINE\\SOFTWARE\\tryTimes", "UseTimes", 0);//获取软件使用次数
if (tLong < 30)
{
int Times = tLong + 1; //计算软件本次是第几次使用
Registry.SetValue("HKEY_LOCAL_MACHINE\\SOFTWARE\\tryTimes", "UseTimes", Times); //修改软件使用次数
}
else
{
MessageBox.Show("使用次数已到,若要继续使用,请联系厂家注册正版!","警告",MessageBoxButtons.OK,MessageBoxIcon.Warning);
Application.Exit();
}
}
private void uiButton2_Click(object sender, EventArgs e)
{
Close();
}
}
}
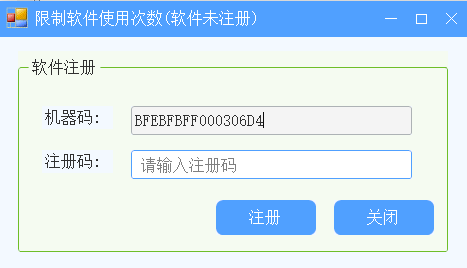