首先在com.neo目录下需要创建几个包:
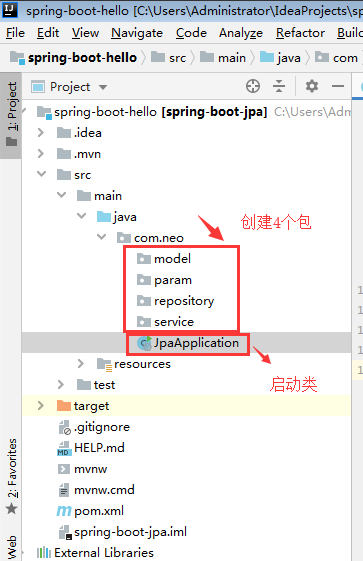
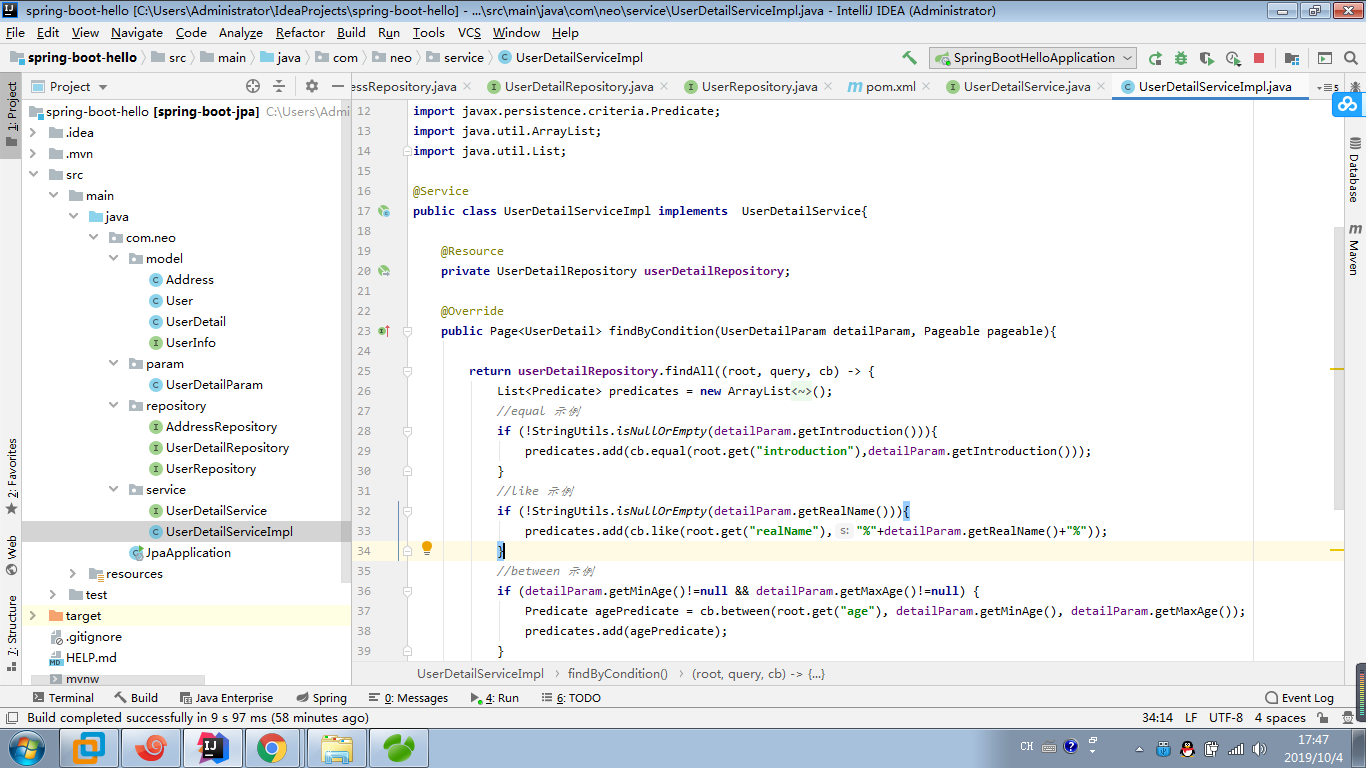
我们需要在pom.xml文件需要加入的文件:
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion>
<groupId>com.neo</groupId> <artifactId>spring-boot-Jpa</artifactId> <version>1.0.0</version> <packaging>jar</packaging>
<name>spring-boot-Jpa</name> <description>Demo project for Spring Boot</description>
<parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>2.1.0.RELEASE</version> <relativePath/> <!-- lookup parent from repository --> </parent>
<properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding> <java.version>1.8</java.version> </properties>
<dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-jpa</artifactId> </dependency> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> </dependencies>
<build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build>
</project>
|
|
|
|
接着,model包下,我们是创建的实体类,分别是:Address、User、UserDetail、UserInfo(接口)
Address:
package com.neo.model;
import javax.persistence.Column; import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.Id;
@Entity public class Address {
@Id @GeneratedValue private Long id; @Column(nullable = false) private Long userId; private String province; private String city; private String street;
public Long getId() { return id; }
public void setId(Long id) { this.id = id; }
public Long getUserId() { return userId; }
public void setUserId(Long userId) { this.userId = userId; }
public String getProvince() { return province; }
public void setProvince(String province) { this.province = province; }
public String getCity() { return city; }
public void setCity(String city) { this.city = city; }
public String getStreet() { return street; }
public void setStreet(String street) { this.street = street; } }
|
|
|
|
User:
package com.neo.model;
import javax.persistence.Column; import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.Id;
@Entity public class User {
@Id @GeneratedValue private Long id; @Column(nullable = false, unique = true) private String userName; @Column(nullable = false) private String passWord; @Column(nullable = false, unique = true) private String email; @Column(nullable = true, unique = true) private String nickName; @Column(nullable = false) private String regTime;
public User() { }
public User(String userName, String passWord, String email, String nickName, String regTime) { this.userName = userName; this.passWord = passWord; this.email = email; this.nickName = nickName; this.regTime = regTime; }
public Long getId() { return id; }
public void setId(Long id) { this.id = id; }
public String getUserName() { return userName; }
public void setUserName(String userName) { this.userName = userName; }
public String getPassWord() { return passWord; }
public void setPassWord(String passWord) { this.passWord = passWord; }
public String getEmail() { return email; }
public void setEmail(String email) { this.email = email; }
public String getNickName() { return nickName; }
public void setNickName(String nickName) { this.nickName = nickName; }
public String getRegTime() { return regTime; }
public void setRegTime(String regTime) { this.regTime = regTime; } }
|
|
|
|
UserDetail:
package com.neo.model;
import javax.persistence.Column; import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.Id;
@Entity public class UserDetail {
@Id @GeneratedValue private Long id; @Column(nullable = false, unique = true) private Long userId; private Integer age; private String realName; private String status; private String hobby; private String introduction; private String lastLoginIp;
public Long getId() { return id; }
public void setId(Long id) { this.id = id; }
public Long getUserId() { return userId; }
public void setUserId(Long userId) { this.userId = userId; }
public Integer getAge() { return age; }
public void setAge(Integer age) { this.age = age; }
public String getRealName() { return realName; }
public void setRealName(String realName) { this.realName = realName; }
public String getStatus() { return status; }
public void setStatus(String status) { this.status = status; }
public String getHobby() { return hobby; }
public void setHobby(String hobby) { this.hobby = hobby; }
public String getIntroduction() { return introduction; }
public void setIntroduction(String introduction) { this.introduction = introduction; }
public String getLastLoginIp() { return lastLoginIp; }
public void setLastLoginIp(String lastLoginIp) { this.lastLoginIp = lastLoginIp; }
@Override public String toString() { return "UserDetail{" + "id=" + id + ", userId=" + userId + ", age=" + age + ", realName='" + realName + '\'' + ", status='" + status + '\'' + ", hobby='" + hobby + '\'' + ", introduction='" + introduction + '\'' + ", lastLoginIp='" + lastLoginIp + '\'' + '}'; } }
|
|
|
|
UserInfo:
package com.neo.model;
public interface UserInfo { String getUserName(); String getEmail(); String getHobby(); String getIntroduction(); }
|
|
|
|
接着,param包下,我们是创建的实体类:UserDetailParam
UserDetailParam:
package com.neo.param;
public class UserDetailParam { private String userId; private Integer minAge; private Integer maxAge; private String realName; private String introduction; private String city;
public String getUserId() { return userId; }
public void setUserId(String userId) { this.userId = userId; }
public Integer getMinAge() { return minAge; }
public void setMinAge(Integer minAge) { this.minAge = minAge; }
public Integer getMaxAge() { return maxAge; }
public void setMaxAge(Integer maxAge) { this.maxAge = maxAge; }
public String getRealName() { return realName; }
public void setRealName(String realName) { this.realName = realName; }
public String getIntroduction() { return introduction; }
public void setIntroduction(String introduction) { this.introduction = introduction; }
public String getCity() { return city; }
public void setCity(String city) { this.city = city; } }
|
|
|
|
接着,repository包下,我们是创建接口类:AddressRepository、UserDetailRepository、UserRepository
AddressRepository:
package com.neo.repository;
import com.neo.model.Address; import org.springframework.data.jpa.repository.JpaRepository;
public interface AddressRepository extends JpaRepository<Address, Long> { }
|
|
|
|
UserDetailRepository:
package com.neo.repository;
import com.neo.model.UserDetail; import org.springframework.data.jpa.repository.JpaRepository; import org.springframework.data.jpa.repository.JpaSpecificationExecutor;
public interface UserDetailRepository extends JpaSpecificationExecutor<UserDetail>, JpaRepository<UserDetail, Long> { }
|
|
|
|
UserRepository:
package com.neo.repository;
import com.neo.model.User; import org.springframework.data.jpa.repository.JpaRepository;
public interface UserRepository extends JpaRepository<User, Long> { }
|
|
|
|
接着,service包下,我们是创建接口与类:UserDetailService(接口)、 UserDetailServiceImpl (实现类)
UserDetailService:
package com.neo.service;
import com.neo.model.UserDetail; import com.neo.param.UserDetailParam; import org.springframework.data.domain.Page; import org.springframework.data.domain.Pageable;
public interface UserDetailService { public Page<UserDetail> findByCondition(UserDetailParam detailParam, Pageable pageable); }
|
|
|
|
UserDetailServiceImpl
package com.neo.service;
import com.mysql.cj.util.StringUtils; import com.neo.model.UserDetail; import com.neo.param.UserDetailParam; import com.neo.repository.UserDetailRepository; import org.springframework.data.domain.Page; import org.springframework.data.domain.Pageable; import org.springframework.stereotype.Service;
import javax.annotation.Resource; import javax.persistence.criteria.Predicate; import java.util.ArrayList; import java.util.List;
@Service public class UserDetailServiceImpl implements UserDetailService{
@Resource private UserDetailRepository userDetailRepository;
@Override public Page<UserDetail> findByCondition(UserDetailParam detailParam, Pageable pageable){
return userDetailRepository.findAll((root, query, cb) -> { List<Predicate> predicates = new ArrayList<Predicate>(); //equal 示例 if (!StringUtils.isNullOrEmpty(detailParam.getIntroduction())){ predicates.add(cb.equal(root.get("introduction"),detailParam.getIntroduction())); } //like 示例 if (!StringUtils.isNullOrEmpty(detailParam.getRealName())){ predicates.add(cb.like(root.get("realName"),"%"+detailParam.getRealName()+"%")); } //between 示例 if (detailParam.getMinAge()!=null && detailParam.getMaxAge()!=null) { Predicate agePredicate = cb.between(root.get("age"), detailParam.getMinAge(), detailParam.getMaxAge()); predicates.add(agePredicate); } //greaterThan 大于等于示例 if (detailParam.getMinAge()!=null){ predicates.add(cb.greaterThan(root.get("age"),detailParam.getMinAge())); } return query.where(predicates.toArray(new Predicate[predicates.size()])).getRestriction(); }, pageable);
} }
|
|
|
|
src\main\resources目录下的application.properties配置
spring.datasource.url=jdbc:mysql://localhost:3306/test?serverTimezone=UTC&useUnicode=true&characterEncoding=utf-8&useSSL=true spring.datasource.username=root spring.datasource.password=root spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
spring.jpa.properties.hibernate.hbm2ddl.auto=create spring.jpa.properties.hibernate.dialect=org.hibernate.dialect.MySQL5InnoDBDialect #sql\u8F93\u51FA spring.jpa.show-sql=true #format\u4E00\u4E0Bsql\u8FDB\u884C\u8F93\u51FA spring.jpa.properties.hibernate.format_sql=true
|
|
|
|
src\test\java\com\neo\repository下创建JpaSpecificationTests、UserDetailRepositoryTests、UserRepositoryTests类
JpaSpecificationTests:
package com.neo.repository;
import com.neo.model.UserDetail; import com.neo.param.UserDetailParam; import com.neo.service.UserDetailService; import com.neo.service.UserDetailServiceImpl; import org.junit.Test; import org.junit.runner.RunWith; import org.springframework.boot.test.context.SpringBootTest; import org.springframework.data.domain.Page; import org.springframework.data.domain.PageRequest; import org.springframework.data.domain.Pageable; import org.springframework.data.domain.Sort; import org.springframework.test.context.junit4.SpringRunner;
import javax.annotation.Resource;
@RunWith(SpringRunner.class) @SpringBootTest public class JpaSpecificationTests {
@Resource private UserDetailService userDetailService;
@Test public void testFindByCondition() { int page=0,size=10; Sort sort = new Sort(Sort.Direction.DESC, "id"); Pageable pageable = PageRequest.of(page, size, sort); UserDetailParam param=new UserDetailParam(); param.setIntroduction("程序员"); param.setMinAge(10); param.setMaxAge(30); Page<UserDetail> page1=userDetailService.findByCondition(param,pageable); for (UserDetail userDetail:page1){ System.out.println("userDetail: "+userDetail.toString()); } }
}
|
|
|
|
UserDetailRepositoryTests:
package com.neo.repository;
import com.neo.model.Address; import com.neo.model.User; import com.neo.model.UserDetail; import com.neo.model.UserInfo; import org.junit.Test; import org.junit.runner.RunWith; import org.springframework.boot.test.context.SpringBootTest; import org.springframework.data.domain.PageRequest; import org.springframework.data.domain.Pageable; import org.springframework.data.domain.Sort; import org.springframework.test.context.junit4.SpringRunner;
import javax.annotation.Resource; import java.text.DateFormat; import java.util.Date; import java.util.List;
@RunWith(SpringRunner.class) @SpringBootTest public class UserDetailRepositoryTests {
@Resource private AddressRepository addressRepository; @Resource private UserDetailRepository userDetailRepository;
@Test public void testSaveAddress() { Address address=new Address(); address.setUserId(1L); address.setCity("北京"); address.setProvince("北京"); address.setStreet("分钟寺"); addressRepository.save(address); }
@Test public void testSaveUserDetail() { Date date = new Date(); DateFormat dateFormat = DateFormat.getDateTimeInstance(DateFormat.LONG, DateFormat.LONG); String formattedDate = dateFormat.format(date); UserDetail userDetail=new UserDetail(); userDetail.setUserId(3L); userDetail.setHobby("吃鸡游戏"); userDetail.setAge(28); userDetail.setIntroduction("一个爱玩的人"); userDetailRepository.save(userDetail); }
@Test public void testUserInfo() { List<UserInfo> userInfos=userDetailRepository.findUserInfo("钓鱼"); for (UserInfo userInfo:userInfos){ System.out.println("userInfo: "+userInfo.getUserName()+"-"+userInfo.getEmail()+"-"+userInfo.getHobby()+"-"+userInfo.getIntroduction()); } } }
|
|
|
|
UserRepositoryTests:
package com.neo.repository;
import com.neo.model.User; import org.junit.Test; import org.junit.runner.RunWith; import org.springframework.boot.test.context.SpringBootTest; import org.springframework.data.domain.PageRequest; import org.springframework.data.domain.Pageable; import org.springframework.data.domain.Sort; import org.springframework.test.context.junit4.SpringRunner;
import javax.annotation.Resource; import java.text.DateFormat; import java.util.Date;
@RunWith(SpringRunner.class) @SpringBootTest public class UserRepositoryTests {
@Resource private UserRepository userRepository;
@Test public void testSave() { Date date = new Date(); DateFormat dateFormat = DateFormat.getDateTimeInstance(DateFormat.LONG, DateFormat.LONG); String formattedDate = dateFormat.format(date); userRepository.save(new User("aa", "aa123456","aa@126.com", "aa", formattedDate)); userRepository.save(new User("bb", "bb123456","bb@126.com", "bb", formattedDate)); userRepository.save(new User("cc", "cc123456","cc@126.com", "cc", formattedDate));
// Assert.assertEquals(3, userRepository.findAll().size()); // Assert.assertEquals("bb", userRepository.findByUserNameOrEmail("bb", "bb@126.com").getNickName()); // userRepository.delete(userRepository.findByUserName("aa")); }
@Test public void testBaseQuery() { Date date = new Date(); DateFormat dateFormat = DateFormat.getDateTimeInstance(DateFormat.LONG, DateFormat.LONG); String formattedDate = dateFormat.format(date); User user=new User("ff", "ff123456","ff@126.com", "ff", formattedDate); userRepository.findAll(); userRepository.findById(3L); userRepository.save(user); user.setId(2L); userRepository.delete(user); userRepository.count(); userRepository.existsById(3L); }
@Test public void testCustomSql() { userRepository.modifyById("neo",3L); userRepository.deleteById(3L); userRepository.findByEmail("ff@126.com"); }
@Test public void testPageQuery() { int page=1,size=2; Sort sort = new Sort(Sort.Direction.DESC, "id"); Pageable pageable = PageRequest.of(page, size, sort); userRepository.findALL(pageable); userRepository.findByNickName("aa", pageable); }
}
|
|
|
|