umi项目集成高德地图的js-sdk ——亲测有效
1.开发前准备
- 注册账号信息
- 创建新应用
2.集成到项目
- 引入到项目中
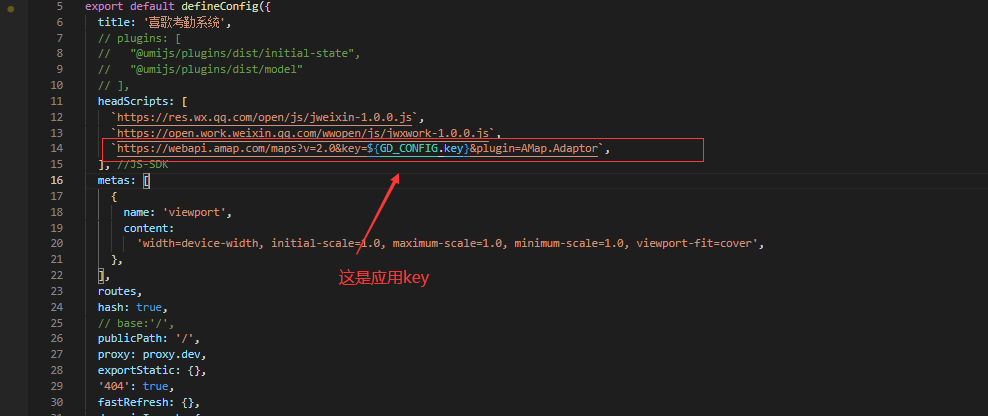
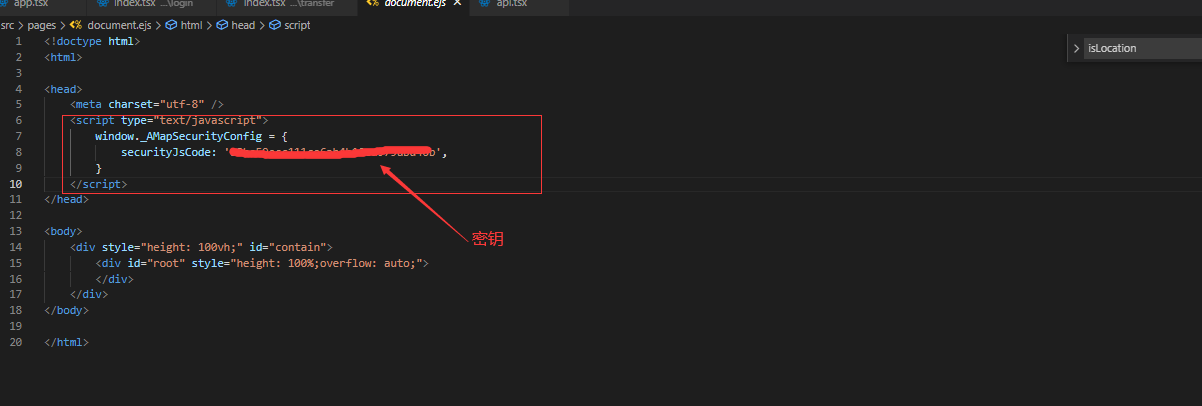
- 相关代码util代码封装
//@ts-ignore const AMap = window.AMap; /** * 其他坐标转换成高德坐标转换 */ export function convertFrom( lnglat?: [longitude: number, latitude: number], type?: any, ) { if (!type) { type = 'gps'; } if (!lnglat) { lnglat = [104.071262, 30.692193]; } AMap.convertFrom(lnglat, type, (status: string, result: any) => { if (result.info === 'ok') { let resLnglat = result.locations[0]; lnglat = [resLnglat.lng, resLnglat.lat]; console.log(3, lnglat); } }); console.log(4, lnglat); return lnglat; } // convertFrom(lnglat, 'gps'); /** * 计算两者距离 */ export function user_shop_distance( shop: { latitude: number; longitude: number; }, user: { latitude: number; longitude: number; }, ) { let p1 = [shop.longitude, shop.latitude]; let p2 = [user.longitude, user.latitude]; let dis = Math.round(AMap.GeometryUtil.distance(p1, p2)); // Toast(dis + ''); return dis <= 10; } /**高德浏览器定位 */ // export function gdGeolocation() { // //@ts-ignore // AMap.plugin('AMap.Geolocation', function () { // var geolocation = new AMap.Geolocation({ // // 是否使用高精度定位,默认:true // enableHighAccuracy: true, // convert: true, // // 设置定位超时时间,默认:无穷大 // timeout: 10000, // // 定位按钮的停靠位置的偏移量 // offset: [10, 20], // // 定位成功后调整地图视野范围使定位位置及精度范围视野内可见,默认:false // zoomToAccuracy: true, // // 定位按钮的排放位置, RB表示右下 // position: 'RB', // }); // geolocation.getCurrentPosition(function (status: string, result: any) { // if (status == 'complete') { // onComplete(result); // } else { // onError(result); // } // }); // function onComplete(data: any) { // // data是具体的定位信息 // console.log('onComplete', JSON.stringify(data)); // alert('onComplete' + JSON.stringify(data)); // } // function onError(data: any) { // alert('onError' + JSON.stringify(data)); // // 定位出错 // console.log('onError', JSON.stringify(data)); // } // }); // } /**逆向地理编码方法 */ export function Geocoder(lnglat?: [longitude: number, latitude: number]) { AMap.plugin('AMap.Geocoder', () => { var geocoder = new AMap.Geocoder({ // city 指定进行编码查询的城市,支持传入城市名、adcode 和 citycode city: '010', extensions: 'base', //默认值:base,返回基本地址信息; 取值为:all,返回地址信息及附近poi、道路、道路交叉口等信息 batch: false, //是否批量查询 batch=true为批量查询,batch=false为单点查询, batch=false时即使传入多个点也只返回第一个点结果 }); geocoder.getAddress(lnglat, (status: any, result: any) => { let res = result; if (status === 'complete' && result.info === 'OK') { // result为对应的地理位置详细信息 // console.log(result.regeocode.formattedAddress); let res = result.regeocode.formattedAddress; console.log('res', res); } console.log(res); }); }); } /** * * @param lnglat 经纬度 * @param type 坐标体系 'gps' ,'' */ export function gpstoaddress( lnglat?: [longitude: number, latitude: number], type?: any, ) { var reslut = ''; if (!type) { type = 'gps'; } if (!lnglat) { lnglat = [经度, 纬度]; } try { AMap.plugin('AMap.Geocoder,AMap.convertFrom', function () { AMap.convertFrom(lnglat, type, function (status: string, result: any) { console.log(result); if (result.info === 'ok') { let resLnglat = result.locations[0]; lnglat = [resLnglat.lng, resLnglat.lat]; console.log(lnglat); } }); let geocoder = new AMap.Geocoder({ // city 指定进行编码查询的城市,支持传入城市名、adcode 和 citycode city: '010', extensions: 'all', batch: false, //是否批量查询 batch=true为批量查询,batch=false为单点查询, batch=false时即使传入多个点也只返回第一个点结果 }); geocoder.getAddress(lnglat, (status: any, res: any) => { if (status === 'complete' && res.info === 'OK') { reslut = res.regeocode.formattedAddress; console.log(reslut); } }); }); } catch (error) { console.log(error); return reslut; } return reslut; } const convertFroms = ( getzuobiao: (res: any) => void, lnglat?: [longitude: number, latitude: number], type?: any, ) => { AMap.convertFrom(lnglat, type, (status: string, result: any) => { if (result.info === 'ok') { let resLnglat = result.locations[0]; // lnglat = [resLnglat.lng, resLnglat.lat]; // console.log(3, lnglat); getzuobiao([resLnglat.lng, resLnglat.lat]); } }); }; /** * 获取定位地址信息 */ export const getGeocoder = ( fun: (res: string) => void, lnglat?: [longitude: number, latitude: number], type?: string, ) => { convertFroms( (obj) => { AMap.plugin('AMap.Geocoder', async () => { let geocoder = new AMap.Geocoder({ // city 指定进行编码查询的城市,支持传入城市名、adcode 和 citycode city: '010', extensions: 'base', //默认值:base,返回基本地址信息; 取值为:all,返回地址信息及附近poi、道路、道路交叉口等信息 batch: false, //是否批量查询 batch=true为批量查询,batch=false为单点查询, batch=false时即使传入多个点也只返回第一个点结果 }); await geocoder.getAddress(obj, (status: any, result: any) => { if (status === 'complete' && result.info === 'OK') { // result为对应的地理位置详细信息 fun(result.regeocode.formattedAddress); } }); }); }, lnglat, type ?? 'gps', ); }; /** * 获取gps经纬度 */ export function getGpsLongLat( fun: (longitude: number, latitude: number) => void, ) { if (navigator.geolocation) { navigator.geolocation.getCurrentPosition(async function (position) { let latitude = position.coords.latitude; //获取纬度 let longitude = position.coords.longitude; //获取经度 // 经度每隔0.00001度,距离相差约1米; fun(longitude, latitude); }); } else { alert('不支持定位功能'); } }
- 引用
//获取定位地址信息 getGeocoder( async (res) => { let result = await addShopLocation({ shopId: initialState?.user?.shopId ?? '', latitude, longitude, shopAddress: res, }); Toast(JSON.stringify(result)); if (result.success) { Toast(result.message); setIisLocation(true); } }, [longitude, latitude], 'gps', );
3.测试
获取定位成功,获取地址成功,并水印在图片上
4.注意事项
- 测试地址应该移动端测试,并开启gps定位,最好用https来引用
- 代码只是测试代码,运用之前还是需要适当自己修改
不用重来才叫快;能够积累才叫多