ant design pro路由跳转
如果是菜单跳转,只需要在cofig/route.ts文件中增加页面信息,点击菜单就能跳转到对应页面
如果是页面链接跳转,有两种,
1.可以通过link链接类似vue的router-link
2.通过history.push 类似vue的router.push
link用法
import { Link } from 'umi'; export default () => { return ( <div> {/* 点击跳转到指定 /about 路由 */} <Link to="/about">About</Link> {/* 点击跳转到指定 /courses 路由, 附带 query { sort: 'name' } */} <Link to="/courses?sort=name">Courses</Link> {/* 点击跳转到指定 /list 路由, 附带 query: { sort: 'name' } 附带 hash: 'the-hash' 附带 state: { fromDashboard: true } */} <Link to={{ pathname: '/list', search: '?sort=name', hash: '#the-hash', state: { fromDashboard: true }, }} > List </Link> {/* 点击跳转到指定 /profile 路由, 附带所有当前 location 上的参数 */} <Link to={(location) => { return { ...location, pathname: '/profile' }; }} /> {/* 点击跳转到指定 /courses 路由, 但会替换当前 history stack 中的记录 */} <Link to="/courses" replace /> {/* innerRef 允许你获取基础组件(这里应该就是 a 标签或者 null) */} <Link to="/courses" innerRef={(node) => { // `node` refers to the mounted DOM element // or null when unmounted }} /> </div> ); };
history 用法
import { history } from 'umi'; // history 栈里的实体个数 console.log(history.length); // 当前 history 跳转的 action,有 PUSH、REPLACE 和 POP 三种类型 console.log(history.action); // location 对象,包含 pathname、search 和 hash console.log(history.location.pathname); console.log(history.location.search); console.log(history.location.hash); 可用于路由跳转, import { history } from 'umi'; // 跳转到指定路由 history.push('/list'); // 带参数跳转到指定路由 history.push('/list?a=b'); history.push({ pathname: '/list', query: { a: 'b', }, }); // 跳转到上一个路由 history.goBack();
// 跳转到上一个路由 history.goBack();
获取跳转的参数
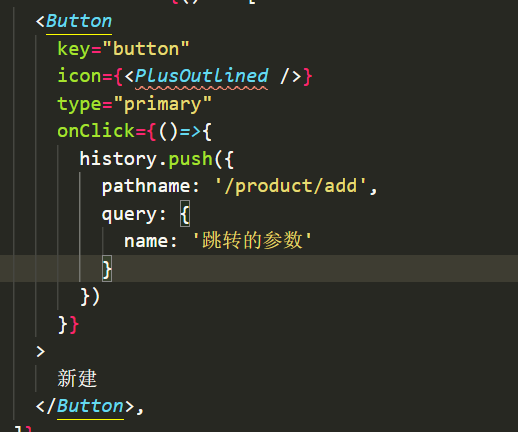
跳转过去通过props可以获取到
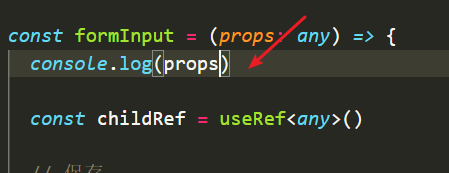
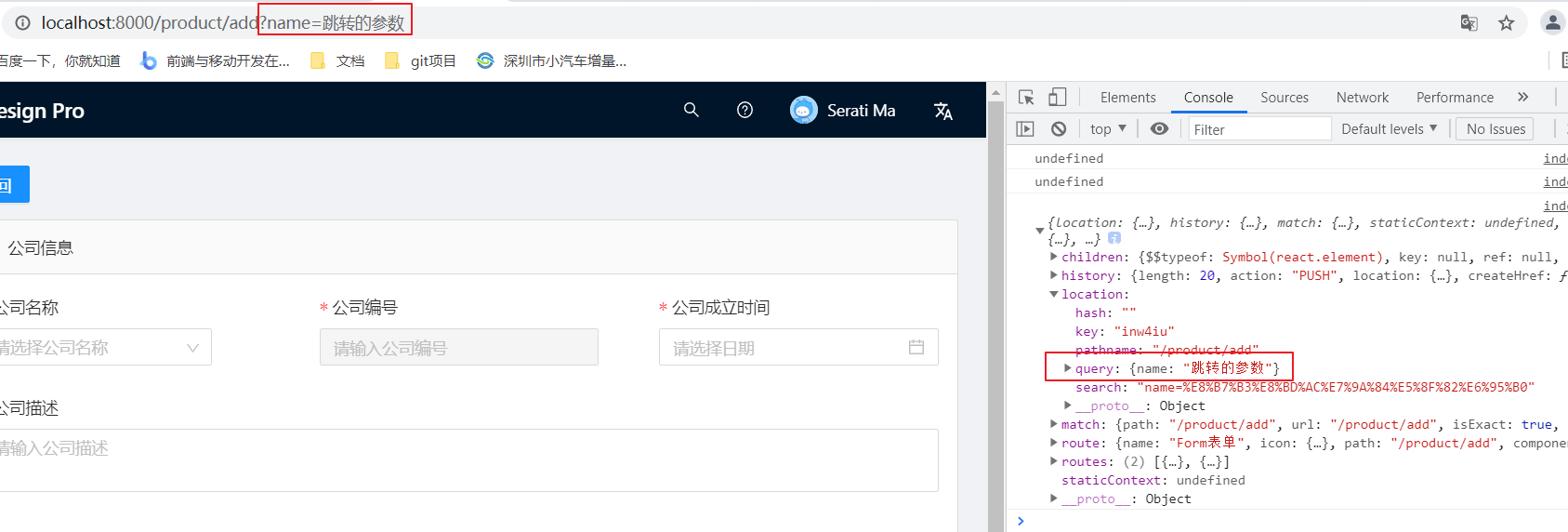
也可以通过useLocation获取到
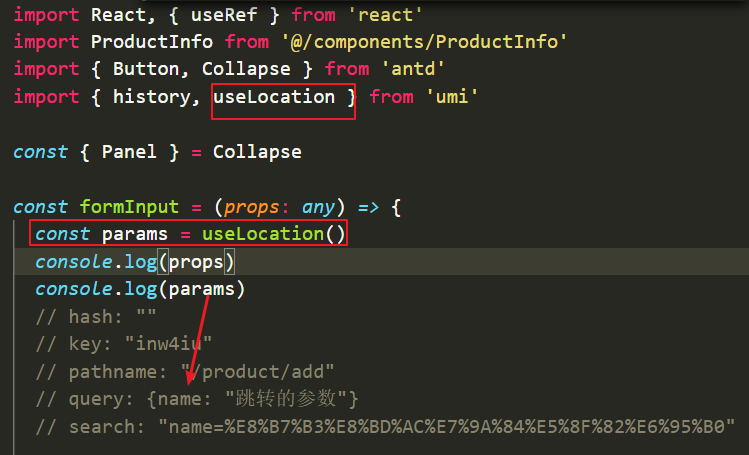
如果是这种地址格式 /users/:id 通过useParams获取
import { useParams } from 'umi'; export default () => { const params = useParams(); return ( <div> <ul> <li>params: {JSON.stringify(params)}</li> </ul> </div> ); };