jquery实现多张图片移动伸缩
之前写了单张图片的移动缩放,用的是原生JS
这篇是在之前的基础上增加了可以放多张图片,可以单独宽度缩放,单独高度缩放的功能
也可以只做单张图片的功能
图片效果:
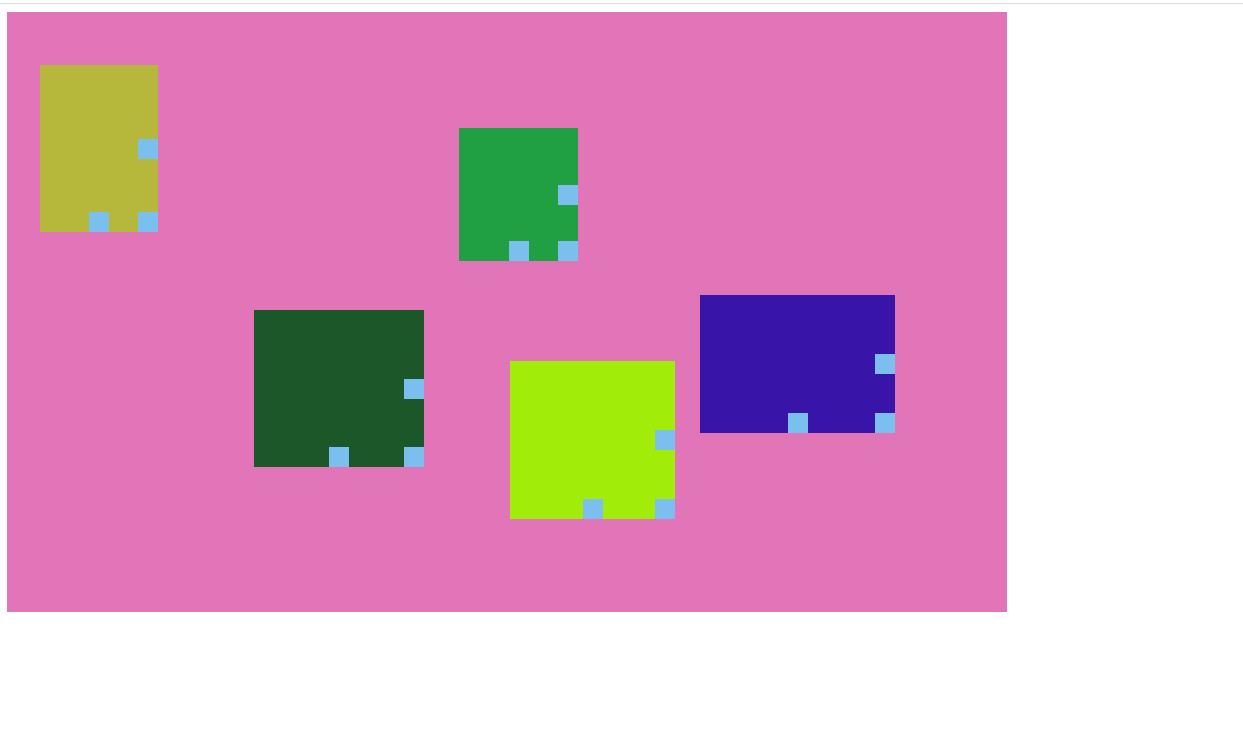
写法是用jQuery,使用的话需要自己下载jQuery安装包
代码
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <meta http-equiv="X-UA-Compatible" content="ie=edge"> <title>Document</title> <style> #father { width: 1000px; height: 600px; background-color: rgb(226, 117, 184); position: relative; } .box { width: 100px; height: 100px; background-color: aquamarine; position: absolute; cursor: move; } .picture { width: 100%; height: 100%; position: absolute; top: 0; left: 0; } .scale { width: 20px; height: 20px; overflow: hidden; cursor: se-resize; position: absolute; right: 0; bottom: 0; background-color: rgb(122, 191, 238); } .scaleright { width: 20px; height: 20px; overflow: hidden; cursor: e-resize; position: absolute; right: 0; top: 50%; margin-top: -10px; background-color: rgb(122, 191, 238); } .scaledown { width: 20px; height: 20px; overflow: hidden; cursor: s-resize; position: absolute; right: 50%; bottom: 0; margin-right: -10px; background-color: rgb(122, 191, 238); } </style> </head> <body> <div id="father"> <div class="box"> <!-- <img class="picture" src="" alt=""> --> <div class="scale"></div> <div class="scaleright"></div> <div class="scaledown"></div> </div> </div> <script src="./jquery-3.1.1.min.js"></script> <script type="text/javascript"> // 模拟动态生成多个图片 function getRandom(min, max) { return Math.floor(Math.random() * (max - min + 1)) + min; } // 随机颜色 function getRandomColor() { var c1 = getRandom(0, 255); var c2 = getRandom(0, 255); var c3 = getRandom(0, 255); return 'rgb(' + c1 + ',' + c2 + ',' + c3 + ')' } var imgList = [ {id: 1, url: '', color: getRandomColor(), width: getRandom(100, 200), height: getRandom(100, 200), top: getRandom(0, 400), left: getRandom(0, 800)}, {id: 2, url: '', color: getRandomColor(), width: getRandom(100, 200), height: getRandom(100, 200), top: getRandom(0, 400), left: getRandom(0, 800)}, {id: 3, url: '', color: getRandomColor(), width: getRandom(100, 200), height: getRandom(100, 200), top: getRandom(0, 400), left: getRandom(0, 800)}, {id: 4, url: '', color: getRandomColor(), width: getRandom(100, 200), height: getRandom(100, 200), top: getRandom(0, 400), left: getRandom(0, 800)}, {id: 5, url: '', color: getRandomColor(), width: getRandom(100, 200), height: getRandom(100, 200), top: getRandom(0, 400), left: getRandom(0, 800)}, ] // 如果只有一张图片,省略这一步 var html = '' imgList.forEach(function(item) { html += '<div class="box" style="background-color:'+item.color+';width:'+item.width+'px;height:'+item.height+'px;top:'+item.top+'px;left:'+item.left+'px;">'+ // '<img class="picture" src="'+item.url+'" alt="">'+ '<div class="scale"></div>'+ '<div class="scaleright"></div>'+ '<div class="scaledown"></div>'+ '</div>' }) $('#father').html(html) var fa = $('#father') // 元素移动 $('.box').on('mousedown', function(e){ e.preventDefault() e.stopPropagation() var box = $(this) var disX = e.clientX - box.position().left var disY = e.clientY - box.position().top fa.on('mousemove', function(ev) { ev.preventDefault() var x = ev.clientX -disX; var y = ev.clientY -disY; // 图形移动的边界判断 x = x <= 0 ? 0 : x; x = x >= fa.outerWidth()-box.outerWidth() ? fa.outerWidth()-box.outerWidth() : x; y = y <= 0 ? 0 : y; y = y >= fa.outerHeight()-box.outerHeight() ? fa.outerHeight()-box.outerHeight() : y; box.css({ left: x, top: y }); }) fa.mouseleave(function(){ fa.off(); }) fa.mouseup(function(){ fa.off(); }) }) // 元素宽缩放 $('.scaleright').on('mousedown', function(e){ e.preventDefault(); e.stopPropagation(); var box = $(this).parent('.box'); var pos = { 'w': box.outerWidth(), 'x': e.clientX, 'y': e.clientY }; fa.on('mousemove', function(ev){ ev.preventDefault(); // 设置图片的最小缩放为30*30 var w = Math.max(30, ev.clientX - pos.x + pos.w) // 设置图片的最大宽高 var l = box.position().left w = w >= fa.outerWidth() - l ? fa.outerWidth() - l : w box.css({ width: w }) // 如果鼠标向上或者向下移动超出小图标范围移除事件 var op = ev.clientY - pos.y if (Math.abs(op) > $('.scaleright').height()) { fa.off(); } fa.mouseleave(function(){ fa.off(); }) fa.mouseup(function(){ fa.off(); }) }) }) // 元素高缩放 $('.scaledown').on('mousedown', function(e){ e.preventDefault(); e.stopPropagation(); var box = $(this).parent('.box'); var pos = { 'h': box.outerHeight(), 'x': e.clientX, 'y': e.clientY }; fa.on('mousemove', function(ev){ ev.preventDefault(); // 设置图片的最小缩放为30*30 var h = Math.max(30,ev.clientY - pos.y + pos.h) // 设置图片的最大宽高 var t = box.position().top h = h >= fa.outerHeight() - t ? fa.outerHeight() - t : h box.css({ height: h }) // 如果鼠标向左或者向右移动超出小图标范围移除事件 var op = ev.clientX - pos.x if (Math.abs(op) > $('.scaleright').width()) { fa.off(); } fa.mouseleave(function(){ fa.off(); }) fa.mouseup(function(){ fa.off(); }) }) }) // 元素宽高缩放 $('.scale').on('mousedown', function(e){ e.preventDefault(); e.stopPropagation(); var box = $(this).parent('.box'); var pos = { 'w': box.outerWidth(), 'h': box.outerHeight(), 'x': e.clientX, 'y': e.clientY }; fa.on('mousemove', function(ev){ ev.preventDefault(); // 设置图片的最小缩放为30*30 var w = Math.max(30, ev.clientX - pos.x + pos.w) var h = Math.max(30,ev.clientY - pos.y + pos.h) // console.log(w,h) // 设置图片的最大宽高 var l = box.position().left var t = box.position().top w = w >= fa.outerWidth() - l ? fa.outerWidth() - l : w h = h >= fa.outerHeight() - t ? fa.outerHeight() - t : h box.css({ width: w, height: h }) fa.mouseleave(function(){ fa.off(); }) fa.mouseup(function(){ fa.off(); }) }) }) </script> </body> </html>
其他的原理在之前的文章有说到,这里不再详述,说一下,单独向左缩放,和单独向下缩放
因为这两种都是单边缩放,会存在鼠标移除缩放小图标的情况,所以这两种需要加上移出缩放框移出事件
jQuery的事件绑定与移出使用'on'和'off' 之前有bind和unbind不过jQuery3.0之后的版本已经删除