Springboot中使用RestTemplate
配置RestTemplate类:
package com.deepbluebi.aip.smartoffice.config;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.http.client.ClientHttpRequestFactory;
import org.springframework.http.client.SimpleClientHttpRequestFactory;
import org.springframework.web.client.RestTemplate;
import org.springframework.http.converter.StringHttpMessageConverter;
import java.nio.charset.Charset;
/**
* 描述:
*
* @author xxx
* @create 2019-07-25-15:55
*/
@Configuration
public class RestTemplateConfig {
@Bean
public RestTemplate restTemplate(ClientHttpRequestFactory factory){
RestTemplate restTemplate= new RestTemplate(factory);
// 支持中文编码
restTemplate.getMessageConverters().set(1,
new StringHttpMessageConverter(Charset.forName("UTF-8")));
return restTemplate;
}
@Bean
public ClientHttpRequestFactory simpleClientHttpRequestFactory(){
SimpleClientHttpRequestFactory factory = new SimpleClientHttpRequestFactory();
factory.setReadTimeout(5000);//单位为ms
factory.setConnectTimeout(5000);//单位为ms
return factory;
}
}
使用JSON参数发送Post请求
// 方式一
public ResultData<String> getDhgLoginUrl(@RequestParam String loginName) {
String resultSring = "";
try {
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.APPLICATION_JSON);
ObjectMapper objectMapper = new ObjectMapper();
ObjectNode jsonObject = objectMapper.createObjectNode();
jsonObject.put("clientId", clientId);
jsonObject.put("paramType", "1");
jsonObject.put("loginName", loginName);
jsonObject.put("redirectUrl", dhgUrl);
HttpEntity<String> request = new HttpEntity<String>(jsonObject.toString(), headers);
String resultJsonString = restTemplate.postForObject(dhgUrl + "/sso/V2/getToken", request, String.class);
JsonNode root = objectMapper.readTree(resultJsonString);
resultSring = dhgUrl + "/sso/token?clientId=" + clientId + "&redirectUri=" + redirectUri + "&code=" + root.get("data").get("token").textValue();
} catch (Exception e) {
e.printStackTrace();
}
return ResultData.data(resultSring);
}
// 方式二
public ResultData<String> getSrmLoginUrl(String loginName) {
String resultSring = "";
try {
CommonParam apiParam = new CommonParam();
//操作者所属公司编码
apiParam.setOperateCompanyCode(companyCode);
//当前时间对应的时间戳(秒数)
apiParam.setTimestamps(System.currentTimeMillis() / 1000);
String sign = buildCurrentSign(JSON.toJSONString(apiParam), appSecret);
apiParam.setSign(sign);
BodyParam body = new BodyParam();
body.setMobileArea("+86");
ReqDTO reqDTO = new ReqDTO();
reqDTO.setCommonParam(apiParam);
reqDTO.setBody(body);
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.APPLICATION_JSON);
HttpEntity<String> request = new HttpEntity<String>(JSON.toJSONString(reqDTO), headers);
String resultJsonString = restTemplate.postForObject(srmUrl, request, String.class);
JSONObject data = JSON.parseObject(resultJsonString);
data = (JSONObject) data.get("data");
resultSring = data.getString("ssoUrl");
} catch (Exception e) {
log.error("获取SrmLoginUrl失败:{}", e.getMessage(), e);
}
return ResultData.data(resultSring);
}
使用form-data发送Post请求
String url = "http://crm.szbxl.com:18280/qrlogin.action";
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.MULTIPART_FORM_DATA);
MultiValueMap map = new LinkedMultiValueMap();
map.add("m", "qr");
HttpEntity requestBody = new HttpEntity(map, headers);
String resultJsonString = restTemplate.postForObject(url, requestBody, String.class);
JSONObject results = JSONObject.parseObject(resultJsonString);
# 值为json
JSONObject data = (JSONObject) results.get("data");
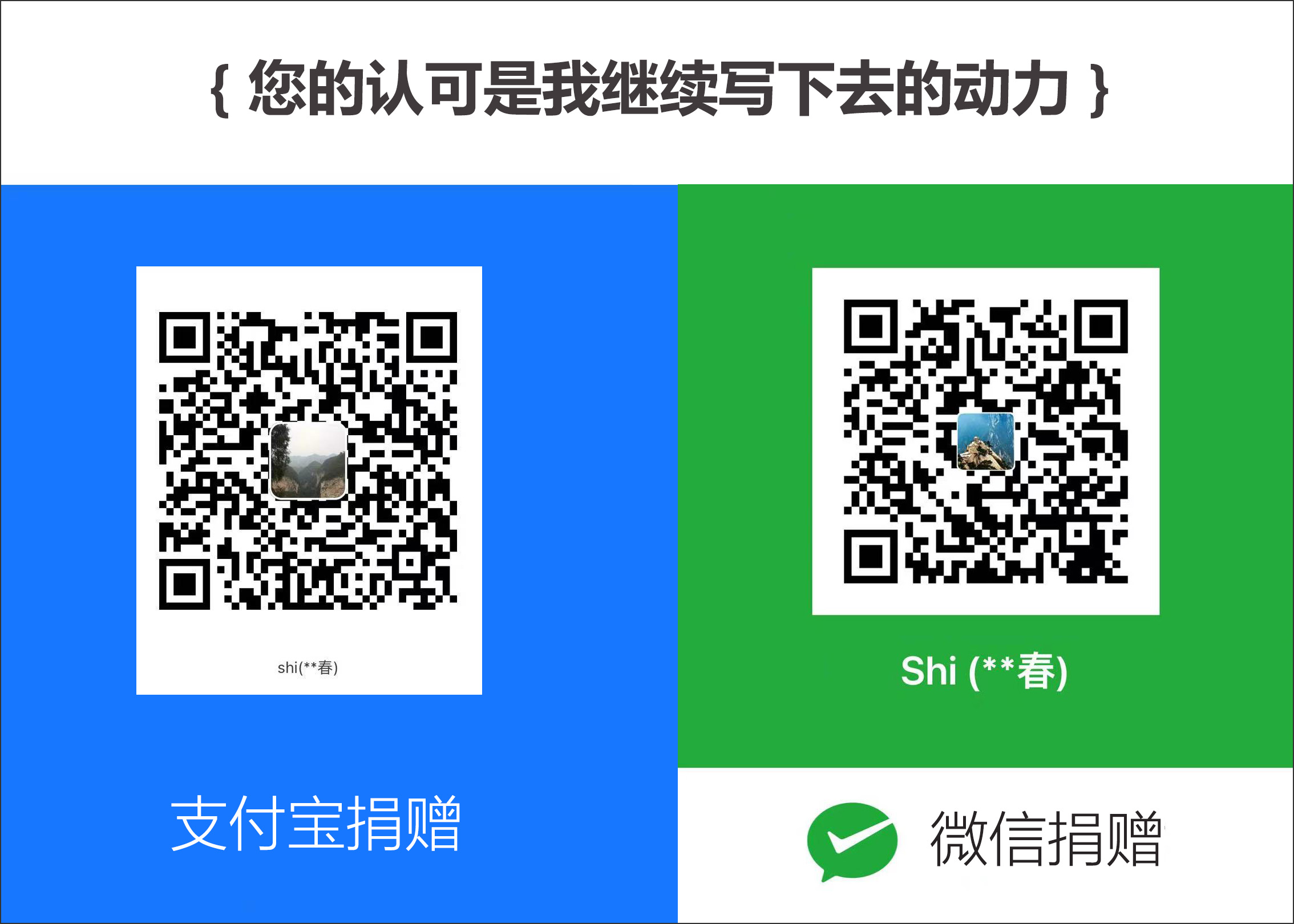