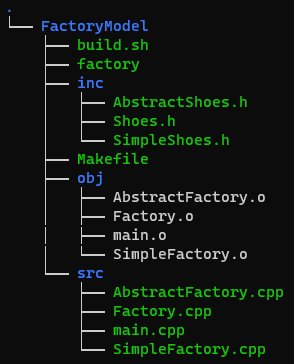
Makefile
Code
TARGET = factory
CC=g++
DIR = $(shell pwd)
DIR_OBJ = $(DIR)/obj
DIR_SRC = $(DIR)/src
DIR_INC = $(DIR)/inc
SRC = $(wildcard $(DIR_SRC)/*.cpp)
SRC_NO_DIR = $(notdir $(SRC))
SRC_OBJ = $(patsubst %cpp, %o, $(SRC_NO_DIR))
INC = -I$(DIR_INC) \
FLAG = -g
all:$(TARGET)
@echo "======= all make successs ======="
$(TARGET):$(addprefix $(DIR_OBJ)/, $(SRC_OBJ))
$(CC) -o $@ $^ $(INC) $(FLAG)
@echo "======= Linux make successs ======="
$(DIR_OBJ)/%.o:$(DIR_SRC)/%.cpp
@mkdir -p $(DIR_OBJ)
$(CC) -c $< -o $@ $(INC) $(FLAG)
.PHONY:clean
clean:
-rm -rf $(DIR_OBJ)
-rm $(TARGET)
@echo "======= make clean success ======="
build.sh
Code
#!/bin/sh
make clean
make
main
Code
#include
#include "SimpleShoes.h"
#include "Shoes.h"
#include "AbstractShoes.h"
// 主函数
int main(int argc, char * argv[])
{
cout << "Start to run Hello World" << endl;
cout << "=== Start to run main_simple_factory ===" << endl;
main_simple_factory();
cout << "=== Start to run main_factory ===" << endl;
main_factory();
cout << "=== Start to run main_abstract_factory ===" << endl;
main_abstract_factory();
return 0;
}
C++版本
简单工厂
simplefactory.cpp
Code
#include
#include "SimpleShoes.h"
using namespace std;
// 简单工厂模式
void main_simple_factory(void)
{
Factory factory;
ShoesBase * pNK = factory.creatShoes(NK);
pNK->show();
ShoesBase * pLN = factory.creatShoes(LN);
pLN->show();
delete pNK;
delete pLN;
}
simpleshoes.h
Code
#ifndef __SIMPLESHOES_H__
#define __SIMPLESHOES_H__
using namespace std;
enum PRODUCTTYPE {NK, LN};
// 抽象类-基类 鞋
class ShoesBase
{
public:
virtual void show() = 0;
virtual ~ShoesBase() {};
};
// 具体产品类-耐克鞋
class NikeShoes:public ShoesBase
{
public:
void show() { cout << "Nike shoes" << endl; }
};
// 具体产品类-李宁鞋
class LiNingShoes:public ShoesBase
{
public:
void show() { cout << "LiNingShoes shoes" << endl; }
};
// 抽象类-基类 工厂
class Factory
{
public:
ShoesBase * creatShoes(PRODUCTTYPE type)
{
switch (type)
{
case NK:
cout << "new NK shoes" << endl;
return new NikeShoes();
break;
case LN:
cout << "new LN shoes" << endl;
return new LiNingShoes();
break;
default:
break;
}
}
};
// 函数声明
void main_simple_factory(void);
#endif
普通工厂
factory.cpp
Code
#include
#include "Shoes.h"
// 工厂模式
void main_factory(void)
{
NBLFactory factory1;
NBLShoes * pShoes1 = factory1.creatShoes();
pShoes1->show();
HXEKFactory factory2;
HXEKShoes * pShoes2 = factory2.creatShoes();
pShoes2->show();
delete pShoes2;
delete pShoes1;
}
shoes.h
Code
#ifndef __SHOES_H__
#define __SHOES_H__
using namespace std;
enum TYPE
{
NBL,
HXEK
};
// 抽象类-基类-鞋
class BaseShoes_F
{
public:
virtual void show() = 0;
virtual ~BaseShoes_F() {};
};
// 具体类-鞋
class NBLShoes:public BaseShoes_F
{
public:
void show()
{
cout << "NBLShoes shoes" << endl;
}
};
class HXEKShoes:public BaseShoes_F
{
public:
void show()
{
cout << "HXEKShoes shoes" << endl;
}
};
// 抽象类-基类-工厂
class BaseFactory_F
{
public:
virtual BaseShoes_F * creatShoes() = 0;
virtual ~BaseFactory_F() {};
};
// 具体类-工厂
class NBLFactory:public BaseFactory_F
{
public:
NBLShoes * creatShoes()
{
cout << "NBLFactory make shoes" << endl;
return new NBLShoes();
}
};
class HXEKFactory:public BaseFactory_F
{
public:
HXEKShoes * creatShoes()
{
cout << "HXEKFactory make shoes" << endl;
return new HXEKShoes();
}
};
// 函数声明
void main_factory(void);
#endif
抽象工厂
abstractfactory.cpp
Code
#include
#include "AbstractShoes.h"
// 抽象工厂模式
void main_abstract_factory(void)
{
AAA_Factory factory1;
BBB_Factory factory2;
AbstractBaseShoes * pShoes1 = NULL;
AbstractBaseTooth * pThooth1 = NULL;
pShoes1 = factory1.creatShoes();
pThooth1 = factory1.creatTooth();
AbstractBaseShoes * pShoes2 = NULL;
AbstractBaseTooth * pThooth2 = NULL;
pShoes2 = factory2.creatShoes();
pThooth2 = factory2.creatTooth();
delete pShoes1;
delete pShoes2;
delete pThooth1;
delete pThooth2;
}
abstractshoes.h
Code
#ifndef __ABSTRACTSHOES_H__
#define __ABSTRACTSHOES_H__
using namespace std;
enum SHOES_TYPE
{
AAA_SHOES_TYPE,
BBB_SHOES_TYPE
};
enum TOOTH_TYPE
{
AAA_TOOTH_TYPE,
BBB_TOOTH_TYPE
};
// 抽象类-鞋
class AbstractBaseShoes
{
public:
virtual ~AbstractBaseShoes() {};
virtual void show() = 0;
};
class AAA_Shoes:public AbstractBaseShoes
{
public:
void show()
{
cout << "AAA_Shoes show" << endl;
}
};
class BBB_Shoes:public AbstractBaseShoes
{
public:
void show()
{
cout << "BBB_Shoes show" << endl;
}
};
// 抽象类-牙刷
class AbstractBaseTooth
{
public:
virtual ~AbstractBaseTooth() {};
virtual void show() = 0;
};
class AAA_Tooth:public AbstractBaseTooth
{
public:
void show()
{
cout << "AAA_Tooth show" << endl;
}
};
class BBB_Tooth:public AbstractBaseTooth
{
public:
void show()
{
cout << "BBB_Tooth show" << endl;
}
};
// 抽象类-工厂
class AbstractBassFactory
{
public:
virtual AbstractBaseShoes * creatShoes() = 0;
virtual AbstractBaseTooth * creatTooth() = 0;
};
class AAA_Factory:public AbstractBassFactory
{
public:
AbstractBaseShoes * creatShoes()
{
cout << "AAA_Factory make Shoes" << endl;
return new AAA_Shoes();
}
AbstractBaseTooth * creatTooth()
{
cout << "AAA_Factory make Tooth" << endl;
return new AAA_Tooth();
}
};
class BBB_Factory:public AbstractBassFactory
{
public:
AbstractBaseShoes * creatShoes()
{
cout << "BBB_Factory make Shoes" << endl;
return new BBB_Shoes();
}
AbstractBaseTooth * creatTooth()
{
cout << "BBB_Factory make Tooth" << endl;
return new BBB_Tooth();
}
};
// 函数声明
void main_abstract_factory(void);
#endif
打印运行结果
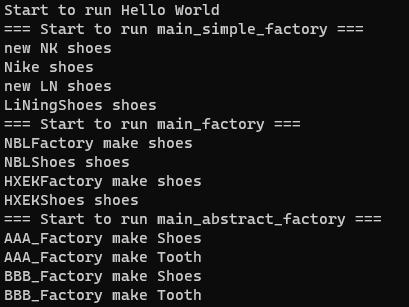
参考链接