[ACM] hdu 1016 Prime Ring Problem (DFS)
Prime Ring Problem
Time Limit: 4000/2000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/Others)
Total Submission(s): 23268 Accepted Submission(s): 10363
Problem Description
A ring is compose of n circles as shown in diagram. Put natural number 1, 2, ..., n into each circle separately, and the sum of numbers in two adjacent circles should be a prime.
Note: the number of first circle should always be 1.
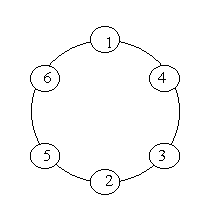
Note: the number of first circle should always be 1.
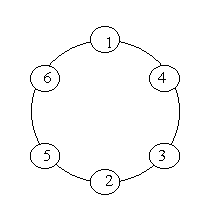
Input
n (0 < n < 20).
Output
The output format is shown as sample below. Each row represents a series of circle numbers in the ring beginning from 1 clockwisely and anticlockwisely. The order of numbers must satisfy the above requirements. Print solutions in
lexicographical order.
You are to write a program that completes above process.
Print a blank line after each case.
You are to write a program that completes above process.
Print a blank line after each case.
Sample Input
6 8
Sample Output
Case 1: 1 4 3 2 5 6 1 6 5 2 3 4 Case 2: 1 2 3 8 5 6 7 4 1 2 5 8 3 4 7 6 1 4 7 6 5 8 3 2 1 6 7 4 3 8 5 2
Source
解题思路:
本质是dfs生成全排列,只不过本题有约束条件。由上学期期末考试时2个多小时做不出来这道题,到现在很快做出这道题,看来自己真进步了不少。继续加油!
代码:
#include <iostream> #include <string.h> #include <algorithm> using namespace std; int num[22]; int visit[22]; int n; bool prime(int n) { if(n==1) return 0; for(int i=2;i<n;i++) if(n%i==0) { return 0; } return 1; } void dfs(int cur) { if(cur>n&&prime(num[1]+num[n]))//输出条件 { for(int i=1;i<=n-1;i++) cout<<num[i]<<" ";cout<<num[n];//注意格式,最后一个数后面没有空格 cout<<endl; } else { for(int i=2;i<=n;i++) { if(prime(i+num[cur-1])&&!visit[i])//注意是num[cur-1]而不是num[i-1],因为要比较相邻的两个数,num[cur]前面的数是num[cur-1] { visit[i]=1; num[cur]=i; dfs(cur+1); visit[i]=0; } } } } int main() { int c=1; while(cin>>n) { num[1]=1; visit[1]=1; memset(visit,0,sizeof(visit)); cout<<"Case "<<c++<<":"<<endl; dfs(2); cout<<endl; } return 0; }
运行: