Promise实践
var doSomething = function() { return new Promise((resolve, reject) => { resolve('返回值'); }); }; let doSomethingElse = function() { return '新的值'; } doSomething().then(function () { return doSomethingElse(); }).then(resp => { console.warn(resp); console.warn('1 =========<'); }); doSomething().then(function () { doSomethingElse(); }).then(resp => { console.warn(resp); console.warn('2 =========<'); }); doSomething().then(doSomethingElse()).then(resp => { console.warn(resp); console.warn('3 =========<'); }); doSomething().then(doSomethingElse).then(resp => { console.warn(resp); console.warn('4 =========<'); });
Promise.prototype.then
当Promise中的状态(pending ---> resolved or rejected)发生变化时才会执行then方法。
- 调用then返回的依旧是一个Promise实例 ( 所以才可以链式调用... )
new Promise((res, rej)=> { res('a'); }).then(val=> { return 'b'; }); // 等同于 new Promise((res, rej)=> { res('a'); }).then(val=> { return new Promise((res, rej)=> { res('b'); }); });
- then中的回调总会异步执行
new Promise((res, rej)=> { console.log('a'); res(''); }).then(()=> { console.log('b'); }); console.log('c'); // a c b
- 如果你不在Promise的参数函数中调用resolve或者reject那么then方法永远不会被触发
new Promise((res, rej)=> { console.log('a'); }).then(()=> { console.log('b'); }); console.log('c'); // a c
Promise.resolve()
除了通过new Promise()的方式,我们还有两种创建Promise对象的方法,Promise.resolve()相当于创建了一个立即resolve的对象。如下两段代码作用相同:
Promise.resolve('a'); new Promise((res, rej)=> { res('a'); });
当然根据传入的参数不同,Promise.resolve()也会做出不同的操作。
- 参数是一个 Promise 实例
如果参数是 Promise 实例,那么Promise.resolve将不做任何修改、原封不动地返回这个实例。
- 参数是一个thenable对象
thenable对象指的是具有then方法的对象,比如下面这个对象。
let thenable = { then: function(resolve, reject) { resolve(42); } };
Promise.resolve方法会将这个对象转为 Promise对象,然后就立即执行thenable对象的then方法。
- 参数不是具有then方法的对象,或根本就不是对象
如果参数是一个原始值,或者是一个不具有then方法的对象,则Promise.resolve方法返回一个新的 Promise 对象,状态为resolved。
- 不带有任何参数
Promise.resolve方法允许调用时不带参数,直接返回一个resolved状态的 Promise 对象。
class Man { constructor(name) { this.name = name; this.sayName(); this.rope = Promise.resolve(); // 定义全局Promise作链式调用 } sayName() { console.log(`hello, ${this.name}`); } sleep(time) { this.rope = this.rope.then(()=> { return new Promise((res, rej)=> { setTimeout(()=> { res(); }, time*1000); }); }); return this; } eat(food) { this.rope = this.rope.then(()=> { console.log(`${this.name} eat ${food}`); }); return this; } } new Man('lan').sleep(3).eat('apple').sleep(5).eat('banana');
class Man1345 { constructor(name) { this.name = name; this.sayName(); } sayName() { console.log(`hello, ${this.name}`); } sleep(time) { this.rope = new Promise((res, rej)=> { setTimeout(()=> { res(); }, time*1000); }); return this; } eat(food) { this.rope = this.rope.then(()=> { console.log(`${this.name} eat ${food}`); }); return this; } } new Man('lan').sleep(3).eat('apple').sleep(5).eat('banana');
// 第一段正确的代码的执行为 var p1 = new Promise().then('停顿3s').then('打印食物').then('停顿5s').then('打印食物'); // 第二段代码的执行行为,p1、p2异步并行执行 var p1 = new Promise().then('停顿3s').then('打印食物'); var p2 = new Promise().then('停顿5s').then('打印食物');
Promise对象在创建即执行(new--关键字)
then(拿前面异步的返回值)
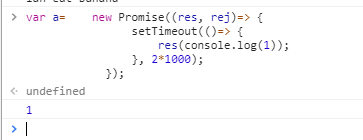