golang解析cad的dxf文件
DXF 是 Drawing Exchange Format或Drawing Interchange Format的简称,是AutoCAD支持的开放数据文件格式。
http://docs.autodesk.com/ACD/2011/CHS/filesDXF/WS1a9193826455f5ff18cb41610ec0a2e719-7a3d.htm
CAD 作图
- 画一个矩形,在矩形中画一个圆,选中后定义为一个块
- 保存为dxf文件,格式使用AutoCAD R12/LT2 DXF
示例代码
golang 包
github.com/rpaloschi/dxf-go
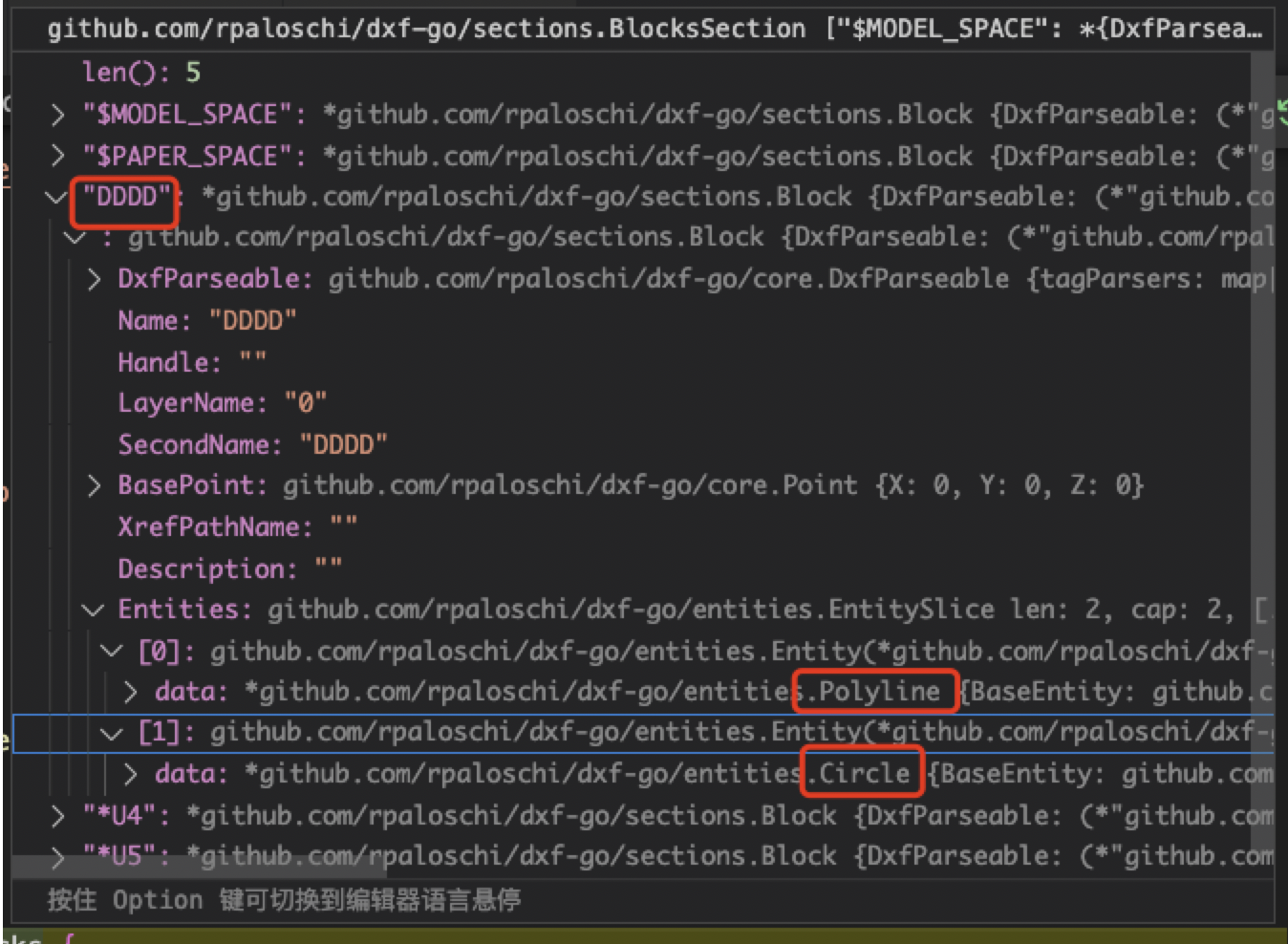
/*
* @Date: 2022-06-19 15:47:08
* @LastEditors: Jason Chen
* @LastEditTime: 2022-07-02 18:49:10
*/
package controller
import (
"fmt"
"log"
"os"
"testing"
// document package
"github.com/rpaloschi/dxf-go/document"
// entities package
"github.com/rpaloschi/dxf-go/entities"
"github.com/axgle/mahonia"
)
func Test_Parse(t *testing.T) {
dxfPath := "smaple.dxf"
file, err := os.Open(dxfPath)
if err != nil {
log.Fatal(err)
}
doc, err := document.DxfDocumentFromStream(file)
if err != nil {
log.Fatal(err)
}
for _, block := range doc.Blocks {
for _, entity := range block.Entities {
if polyline, ok := entity.(*entities.Polyline); ok {
fmt.Println("polyline: ")
for index, item := range polyline.Vertices {
fmt.Printf("[%v] (%v, %v) \n", index, item.Location.X, item.Location.Y)
}
// process polyline here...
} else if line, ok := entity.(*entities.Line); ok {
msg := fmt.Sprintf("line: start(%v),end(%v)", line.Start, line.End)
fmt.Println(msg)
} else if lwpolyline, ok := entity.(*entities.LWPolyline); ok {
fmt.Println(lwpolyline)
// process lwpolyline here...
} else if circle, ok := entity.(*entities.Circle); ok {
msg := fmt.Sprintf("circle: 圆心(%v),半径(%v)", circle.Center, circle.Radius)
fmt.Println(msg)
} else if text, ok := entity.(*entities.Text); ok {
fmt.Println("text: " + ConvertToString(text.Value, "gbk", "utf-8"))
}
//...
}
}
}
// 字符转码
// e.g. 中文字符乱码问题 ConvertToString(str1, "gbk", "utf-8")
func ConvertToString(src string, srcCode string, tagCode string) string {
srcCoder := mahonia.NewDecoder(srcCode)
srcResult := srcCoder.ConvertString(src)
tagCoder := mahonia.NewDecoder(tagCode)
_, cdata, _ := tagCoder.Translate([]byte(srcResult), true)
result := string(cdata)
return result
}
欢迎在评论区留下你宝贵的意见,不论好坏都是我前进的动力(cnblogs 排名提升)!
如果喜欢,记得点赞、推荐、关注、收藏、转发 ... ;)