join
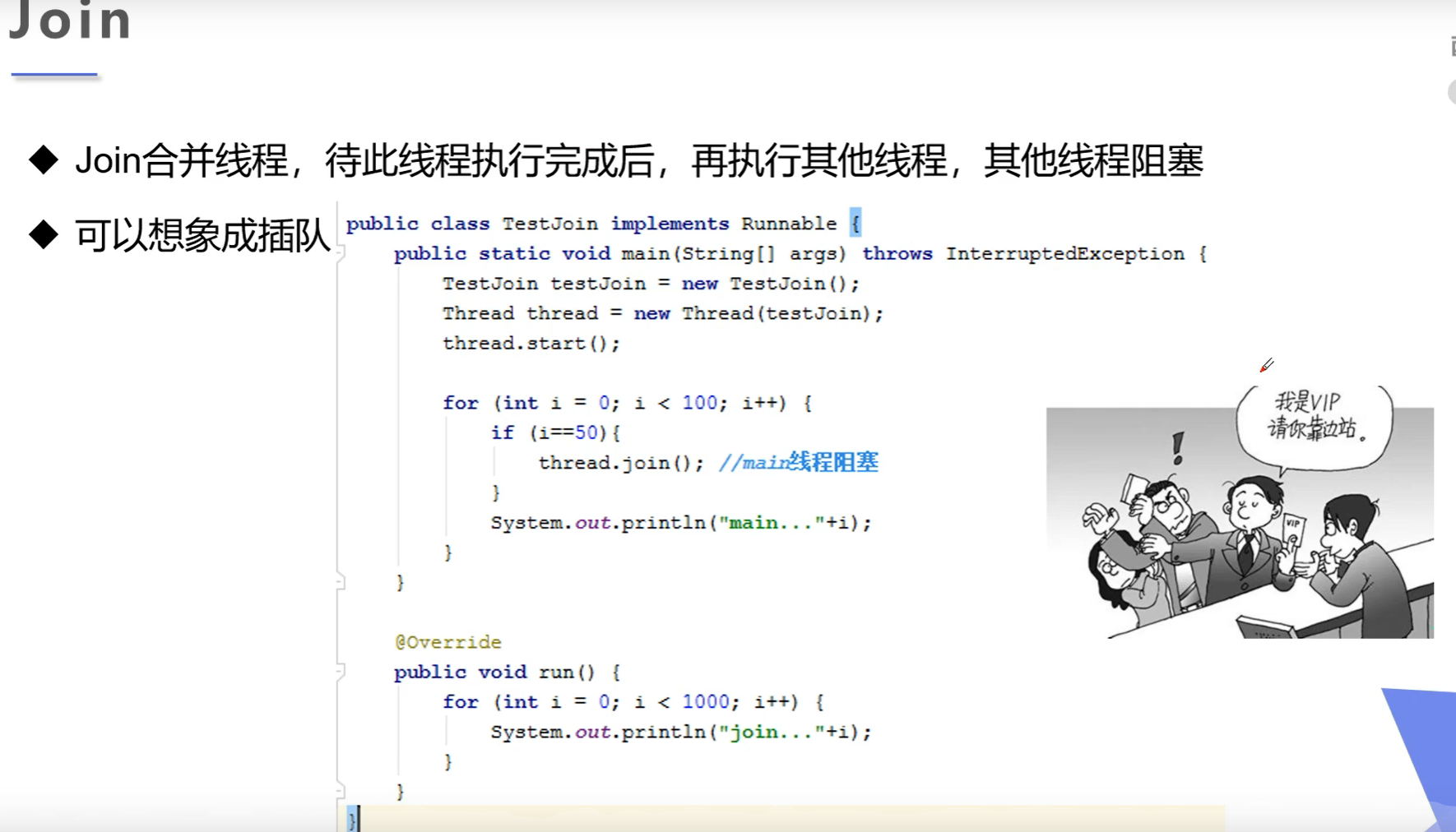
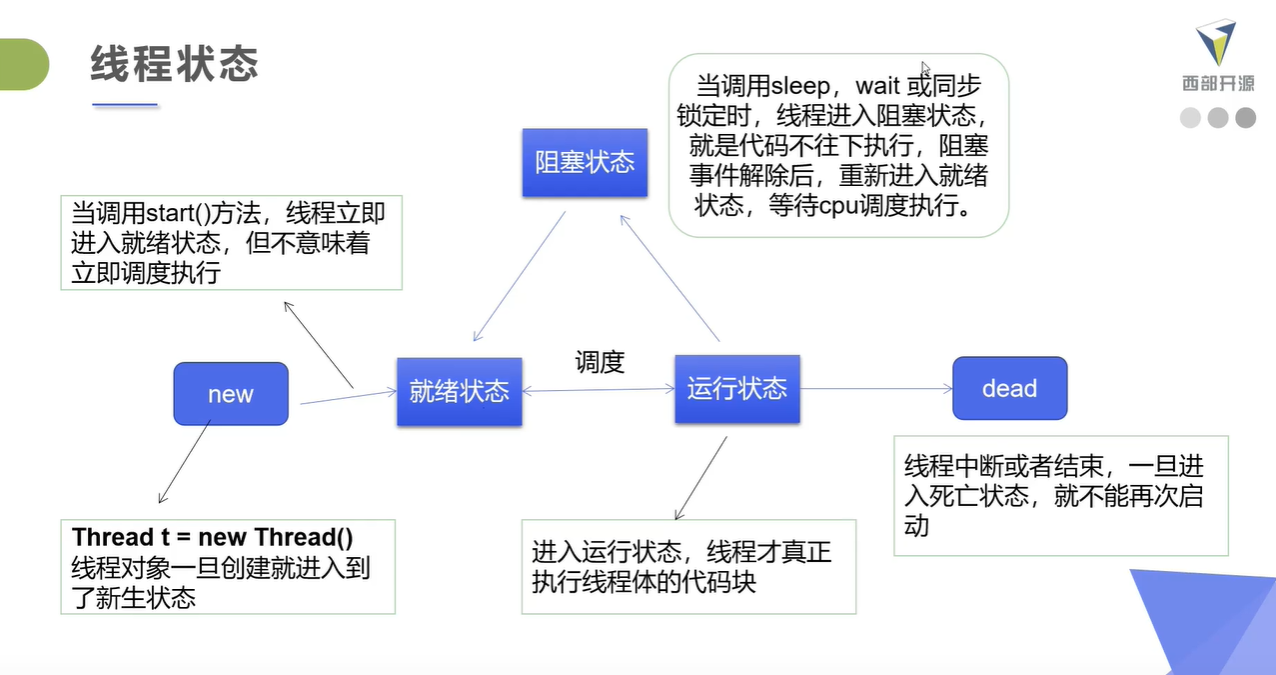
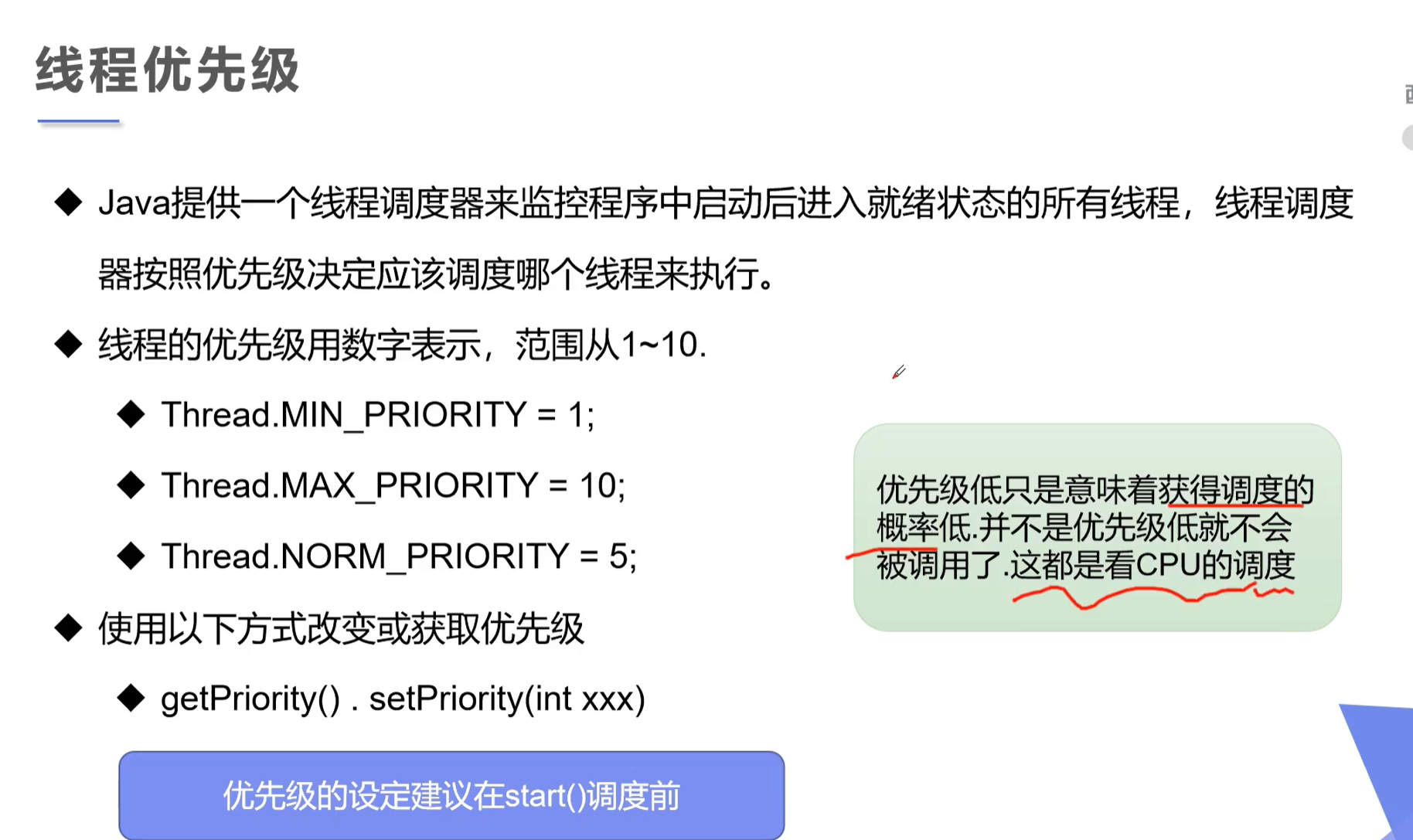
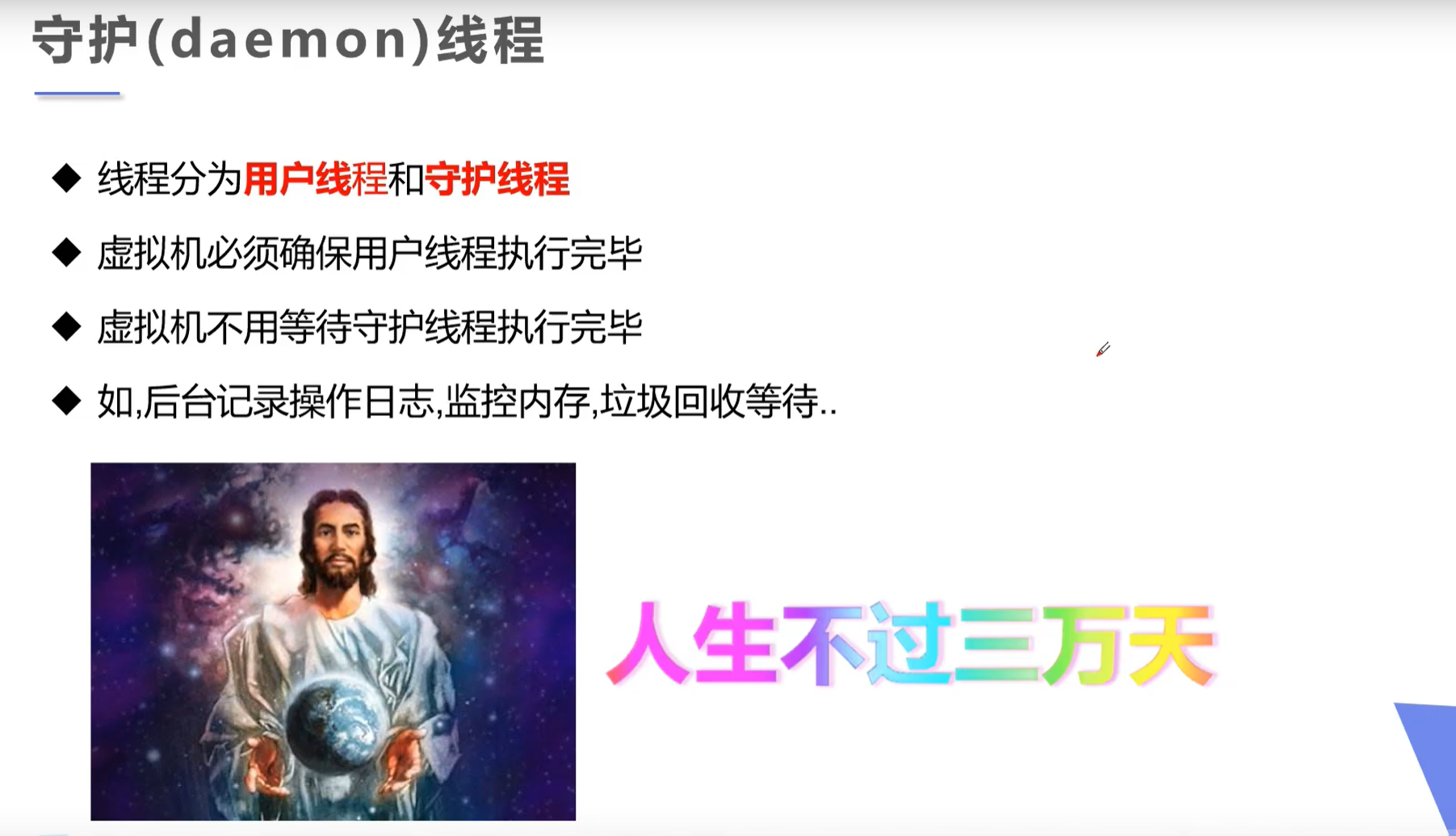
import javax.swing.*;
//测试守护线程
//上帝守护你
public class TestDaemon {
public static void main(String[] args) {
God god =new God();
You you =new You();
Thread thread =new Thread(god);
thread.setDaemon(true); //默认是false 表示是用户线程,正常的线程都是用户线程。。。
new Thread(you).start();
}
}
//上帝
//你
class God implements Runnable{
@Override
public void run() {
while (true){
System.out.println("上帝保佑你");
}
}
}
class You implements Runnable{
@Override
public void run() {
for (int i = 0; i < 36500; i++) {
System.out.println("你一生都开心的活着");
}
System.out.println("=====goodbye ! world!===");
}
}
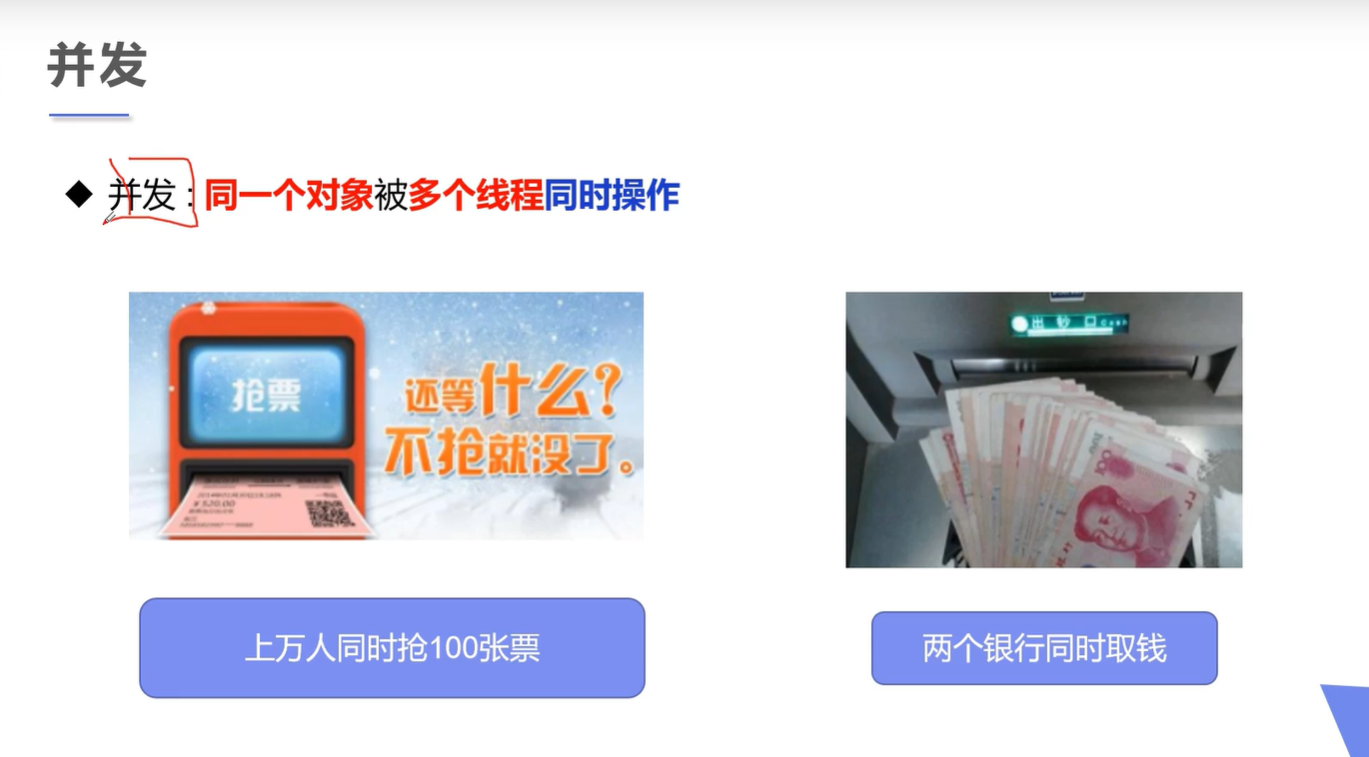
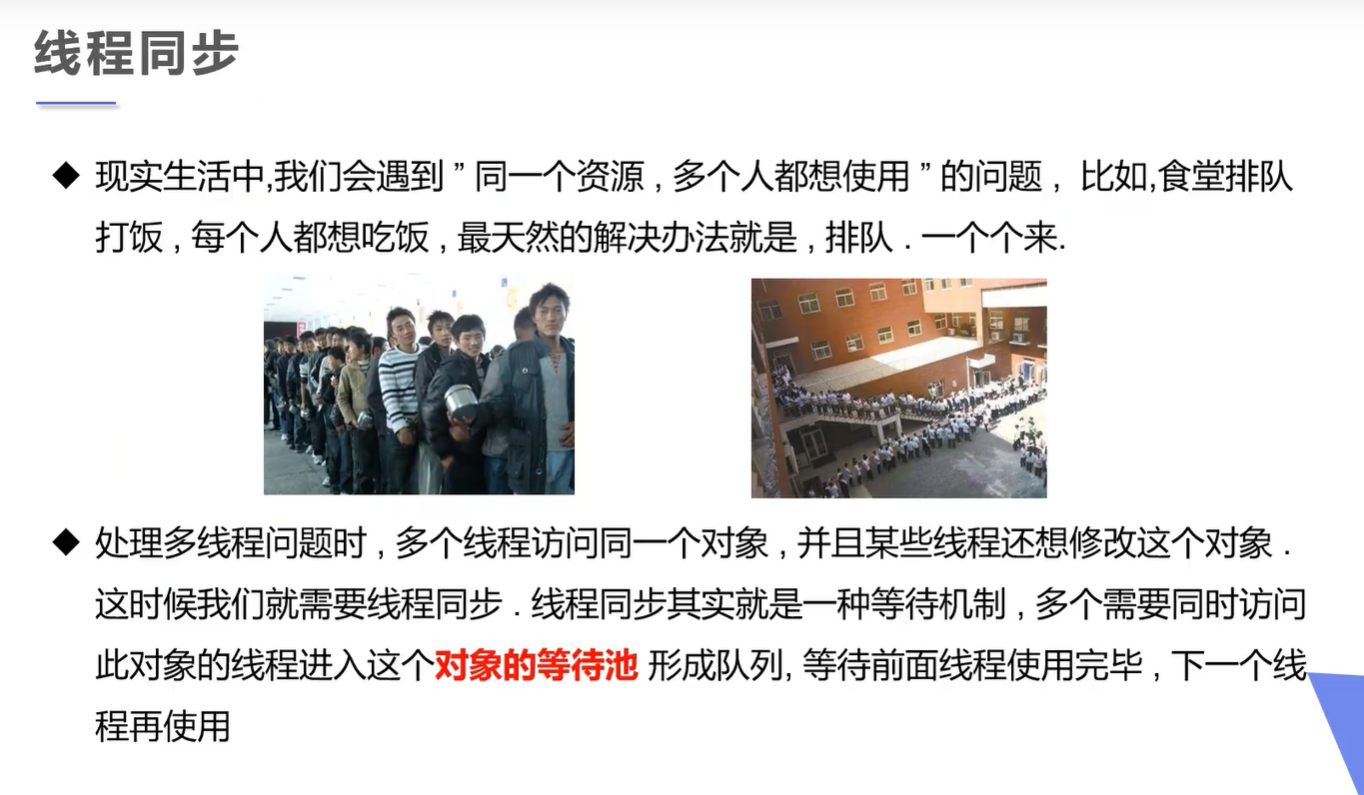
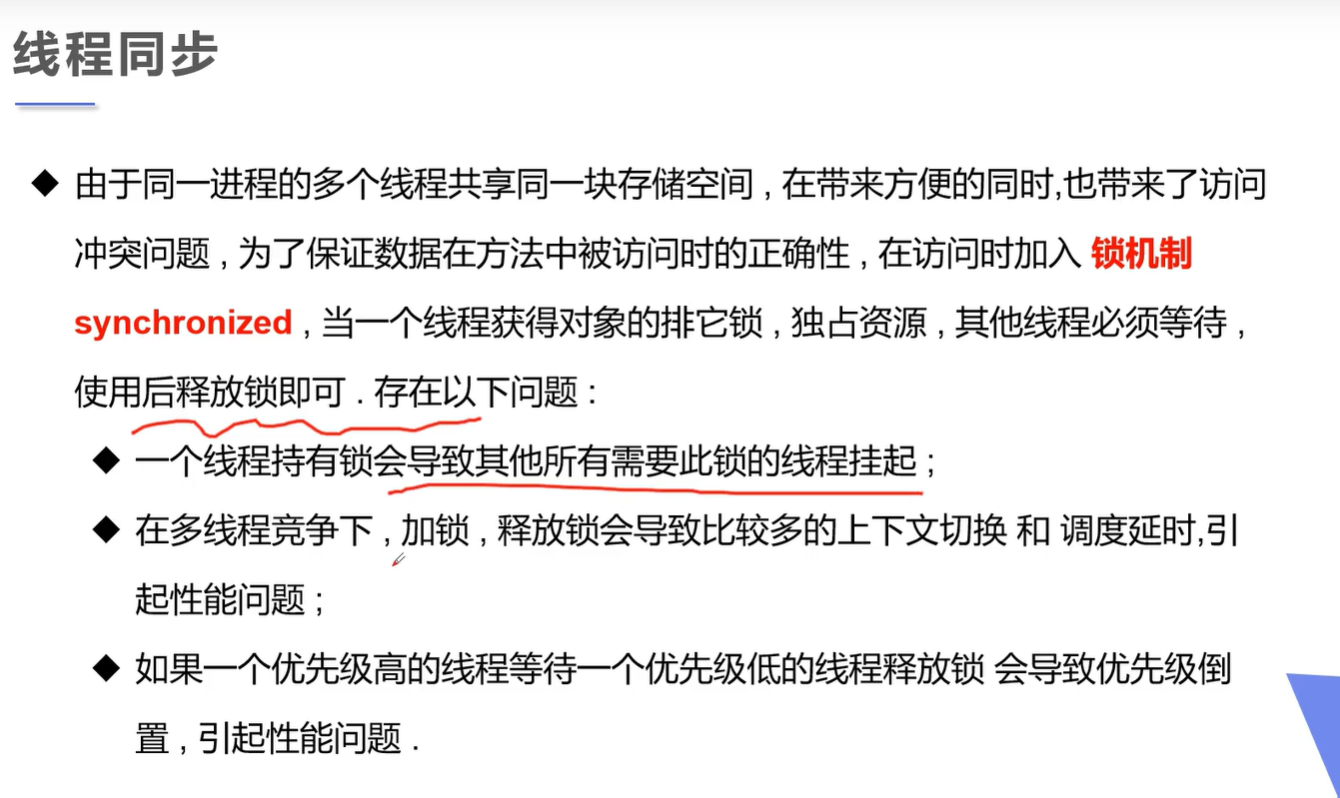
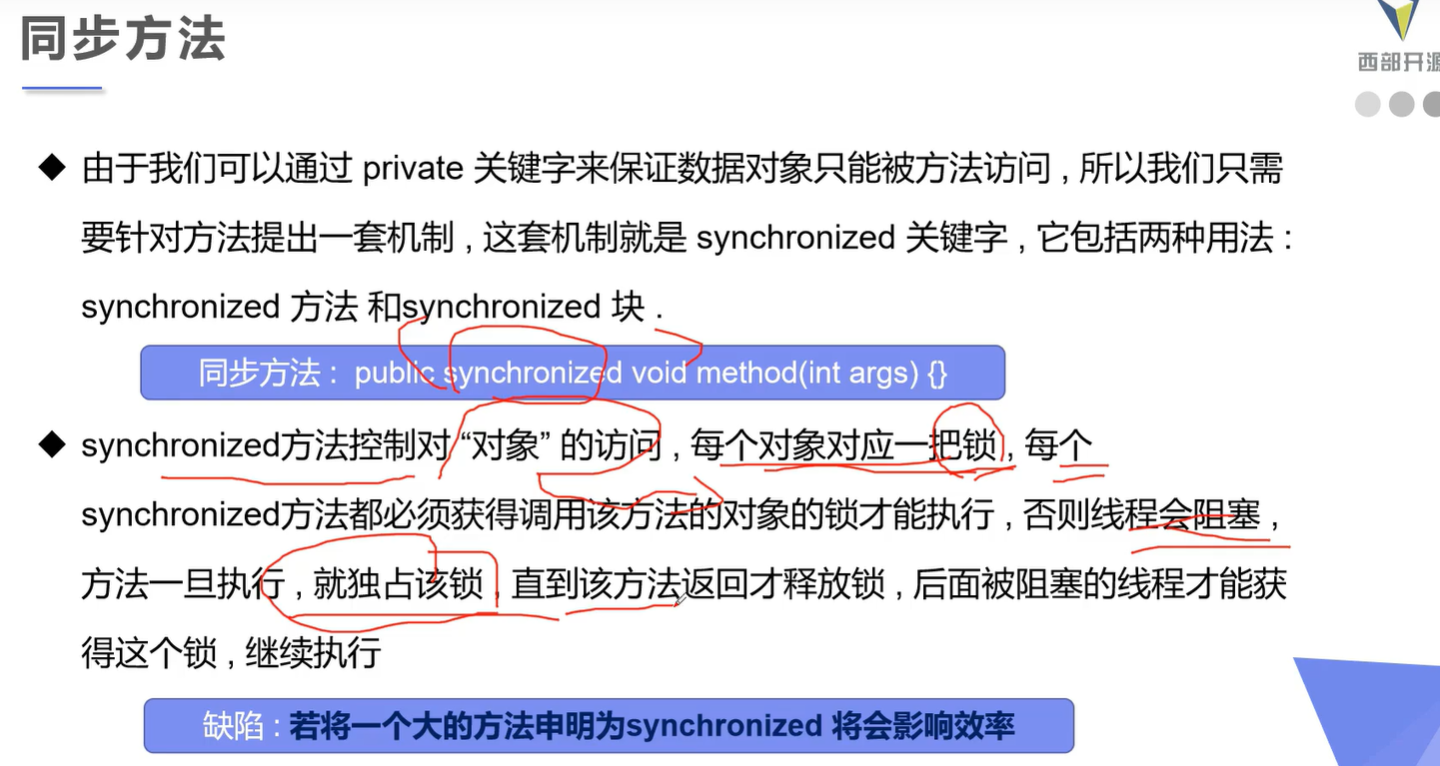
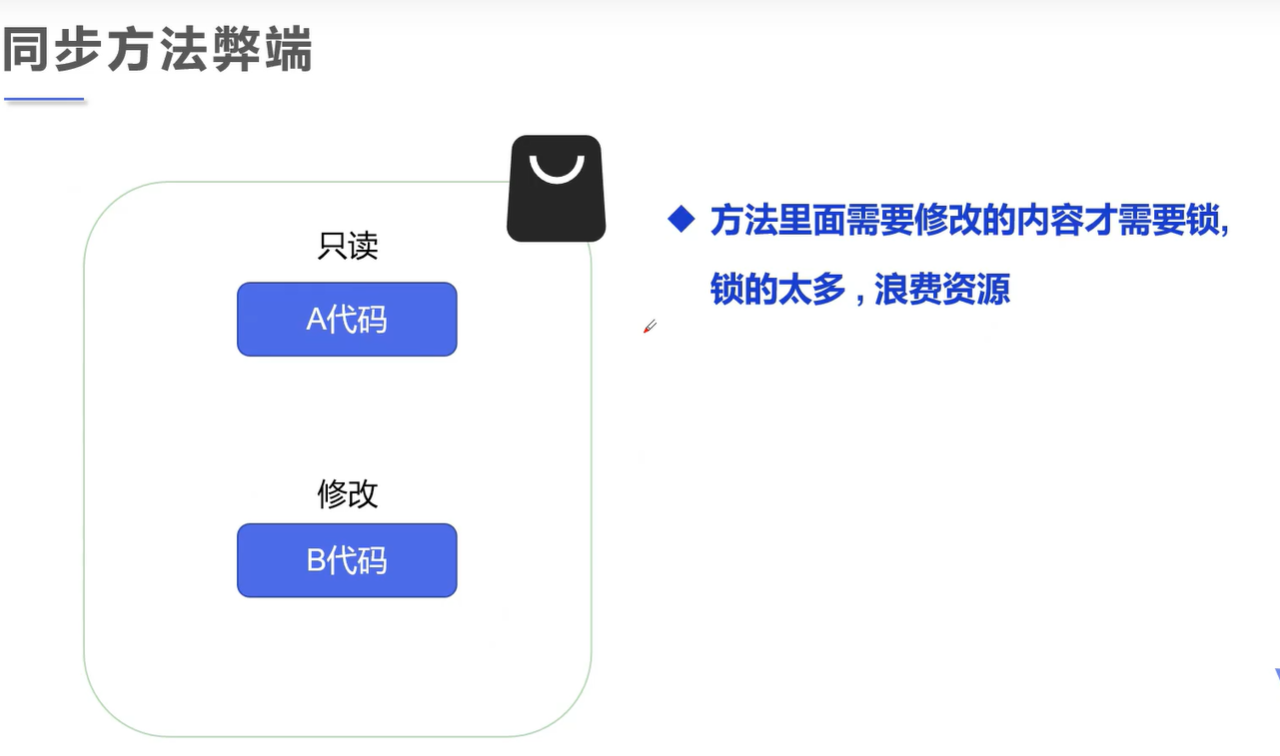
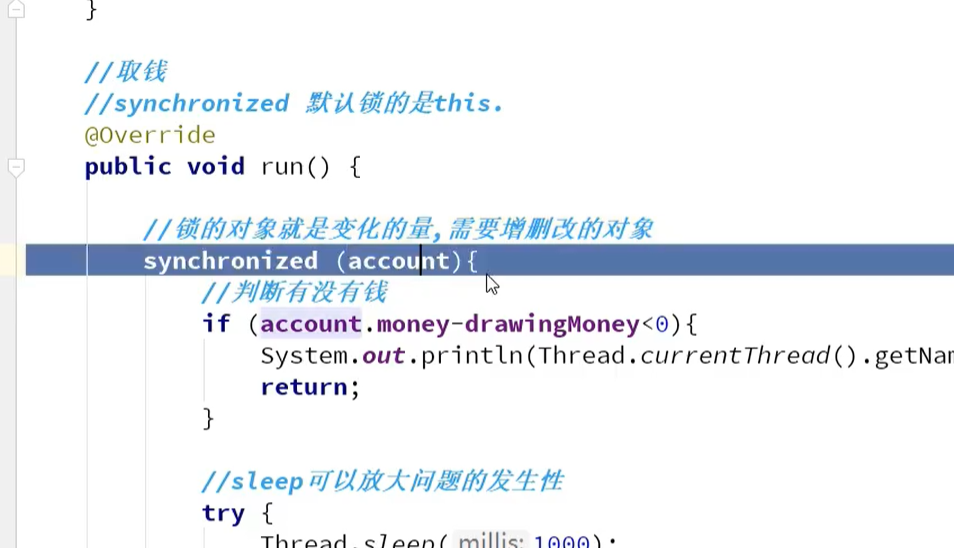
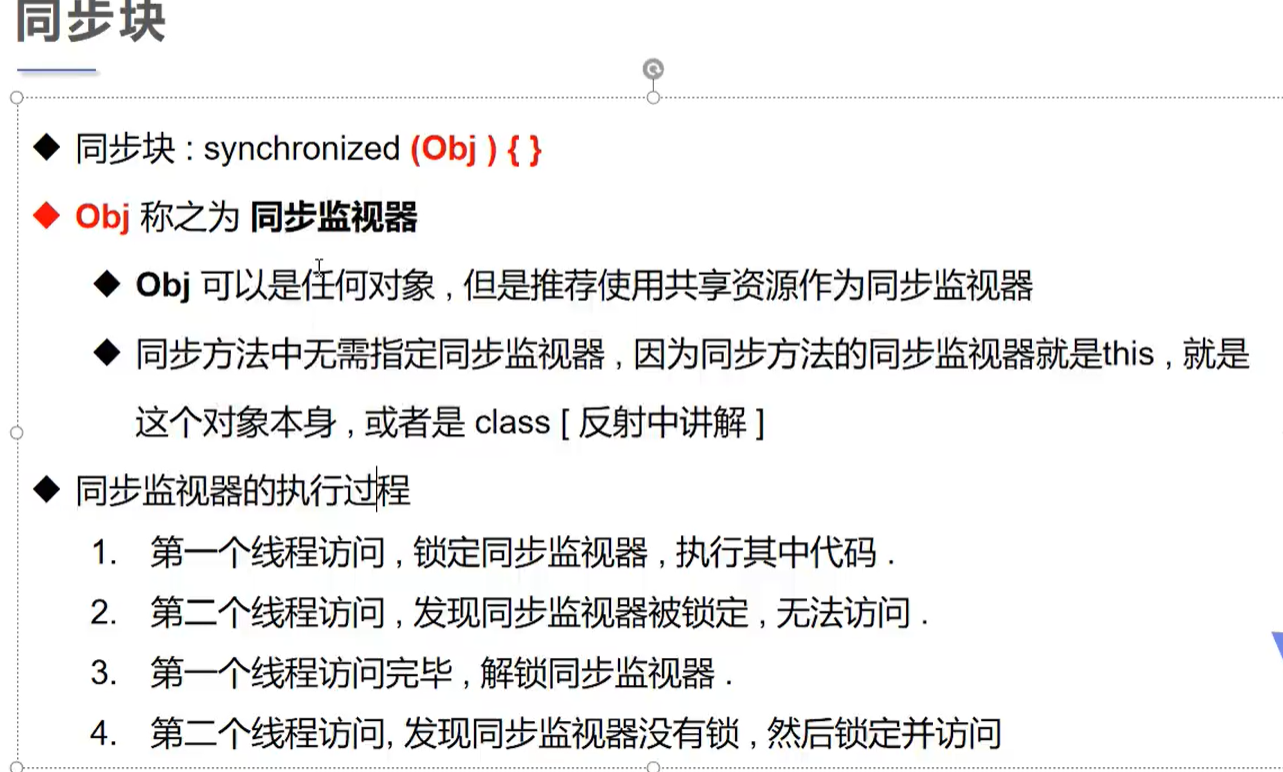
死锁
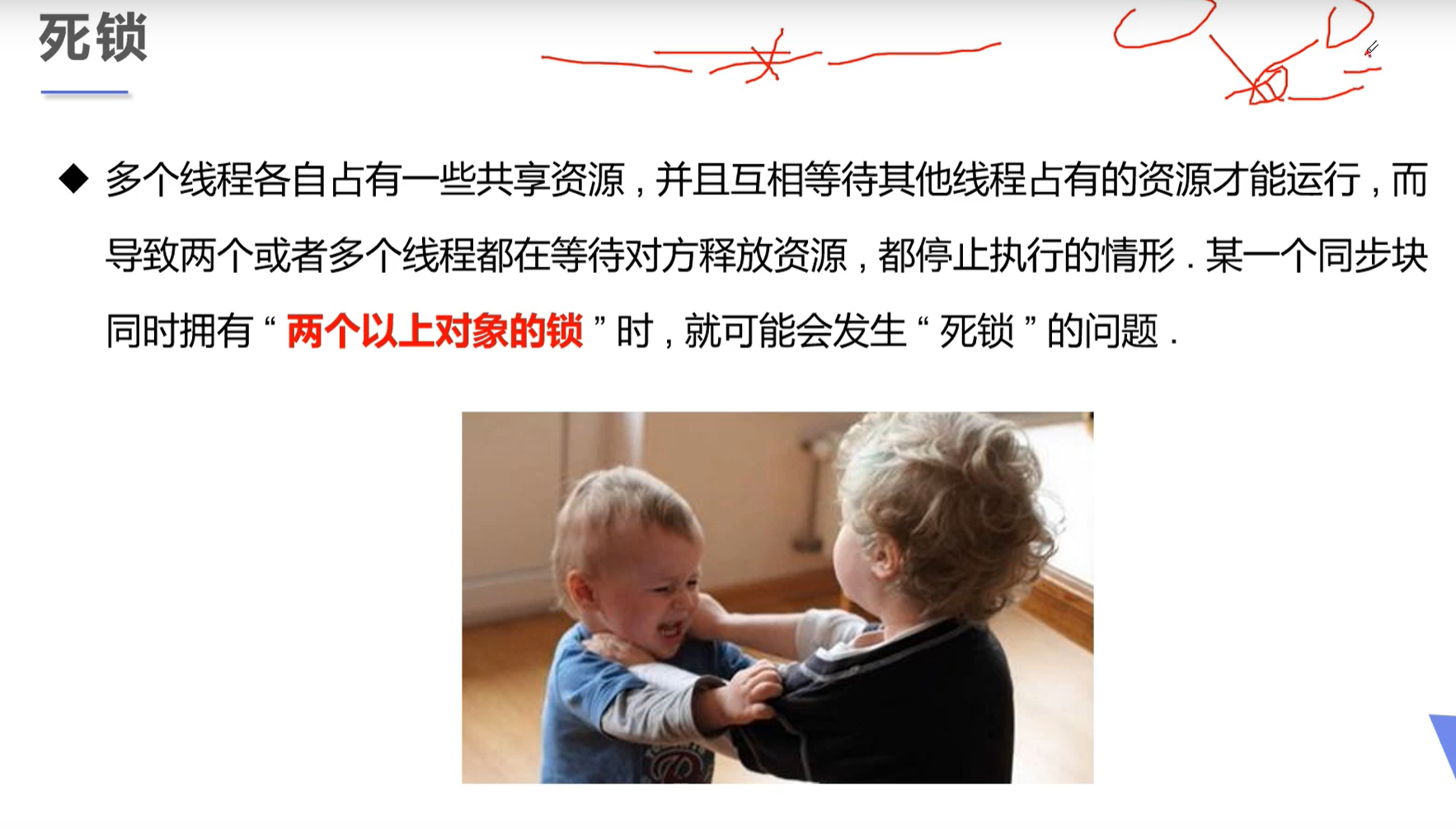
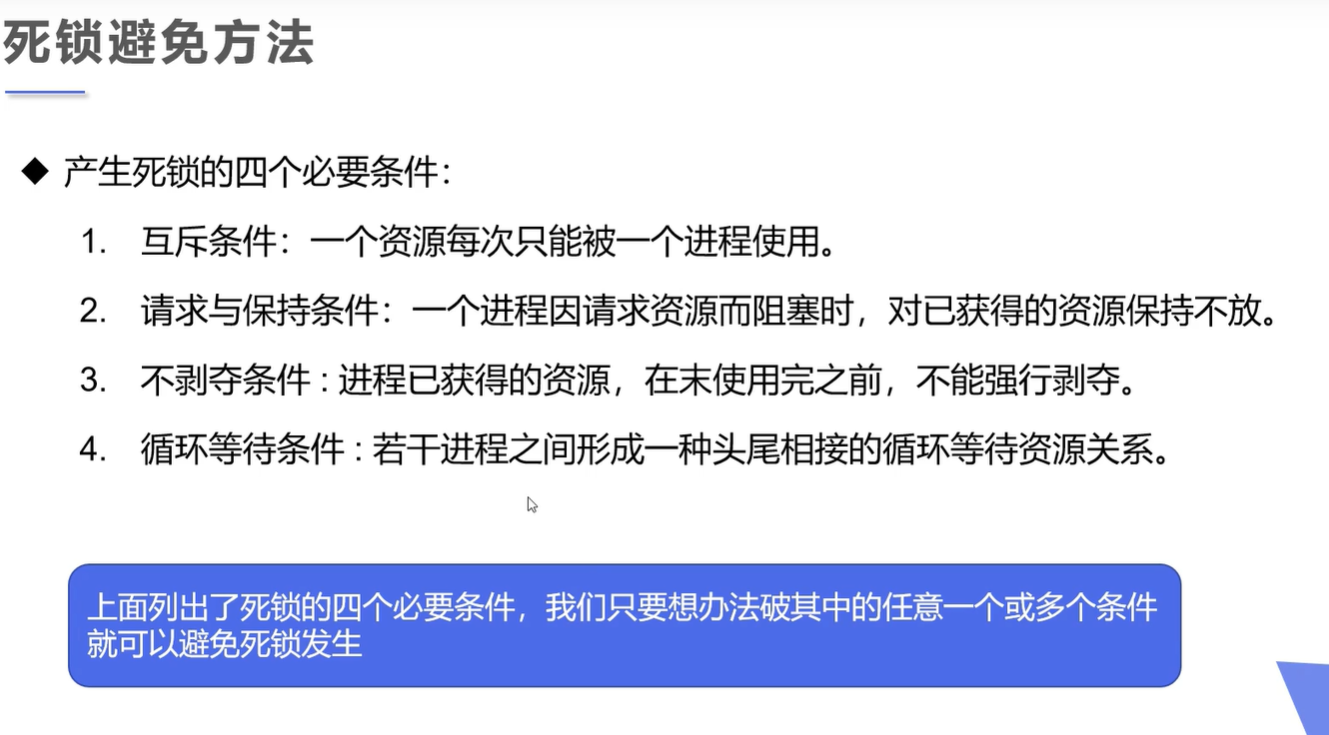
//死锁:多个线程互相抱着对方需要的资源,然后形成僵持
public class DeadLock {
public static void main(String[] args) {
Makeup g1 =new Makeup(0,"灰姑娘");
Makeup g2 =new Makeup(1,"白雪公主");
g1.start();
g2.start();
}
}
//口红
class Lipstick{
}
//镜子
class Mirror{}
class Makeup extends Thread{
//需要的资源只有一份,用static来保证只有一份
static Lipstick lipstick =new Lipstick();
static Mirror mirror =new Mirror();
int choice;//选择
String girlName; //使用化妆品的人
Makeup(int choice,String girlName){
this.choice =choice;
this.girlName =girlName;
}
@Override
public void run() {
//化妆
try {
makeup();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
//化妆,互相持有对方的债,就是需要拿到对方的资源
private void makeup() throws InterruptedException {
if (choice==0){
synchronized (lipstick){//获得口红的锁
System.out.println(this.girlName+"获得口红的锁");
Thread.sleep(1000);
}
synchronized (mirror){
//一秒钟或获得镜子
System.out.println(this.girlName+"获得镜子的锁");
}
}else{
synchronized (mirror){//获得镜子的锁
System.out.println(this.girlName+"获得镜子的锁");
Thread.sleep(2000);
}
synchronized (lipstick){
System.out.println(this.girlName+"获得口红的锁");
}
}
}
}
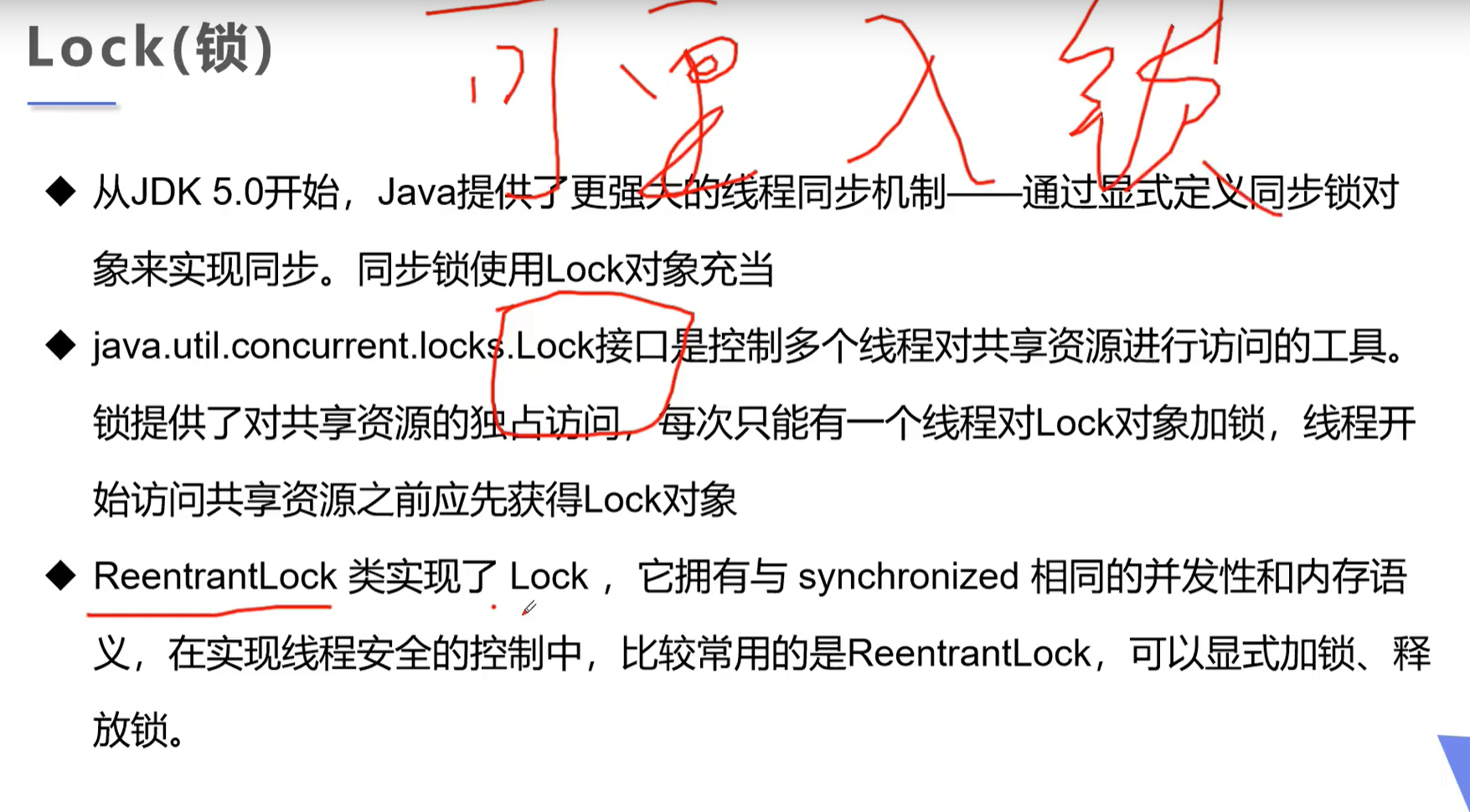
Lock
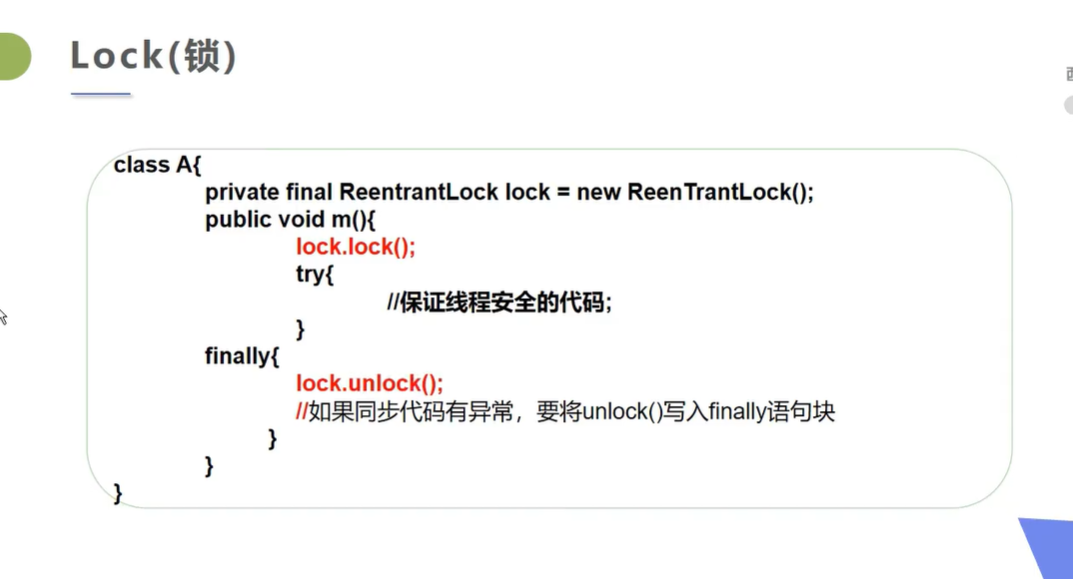
import java.util.concurrent.locks.ReentrantLock;
public class TestLock {
public static void main(String[] args) {
TestLock2 testLock2 =new TestLock2();
new Thread(testLock2).start();
new Thread(testLock2).start();
new Thread(testLock2).start();
}
}
class TestLock2 implements Runnable{
int ticketNums =10;
//定义lock锁
private final ReentrantLock lock =new ReentrantLock();
@Override
public void run() {
while(true){
try{
lock.lock();
if (ticketNums >0) {
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println(ticketNums--);
}else {
break;
}
}finally {
//解锁
lock.unlock();
}
}
}
}
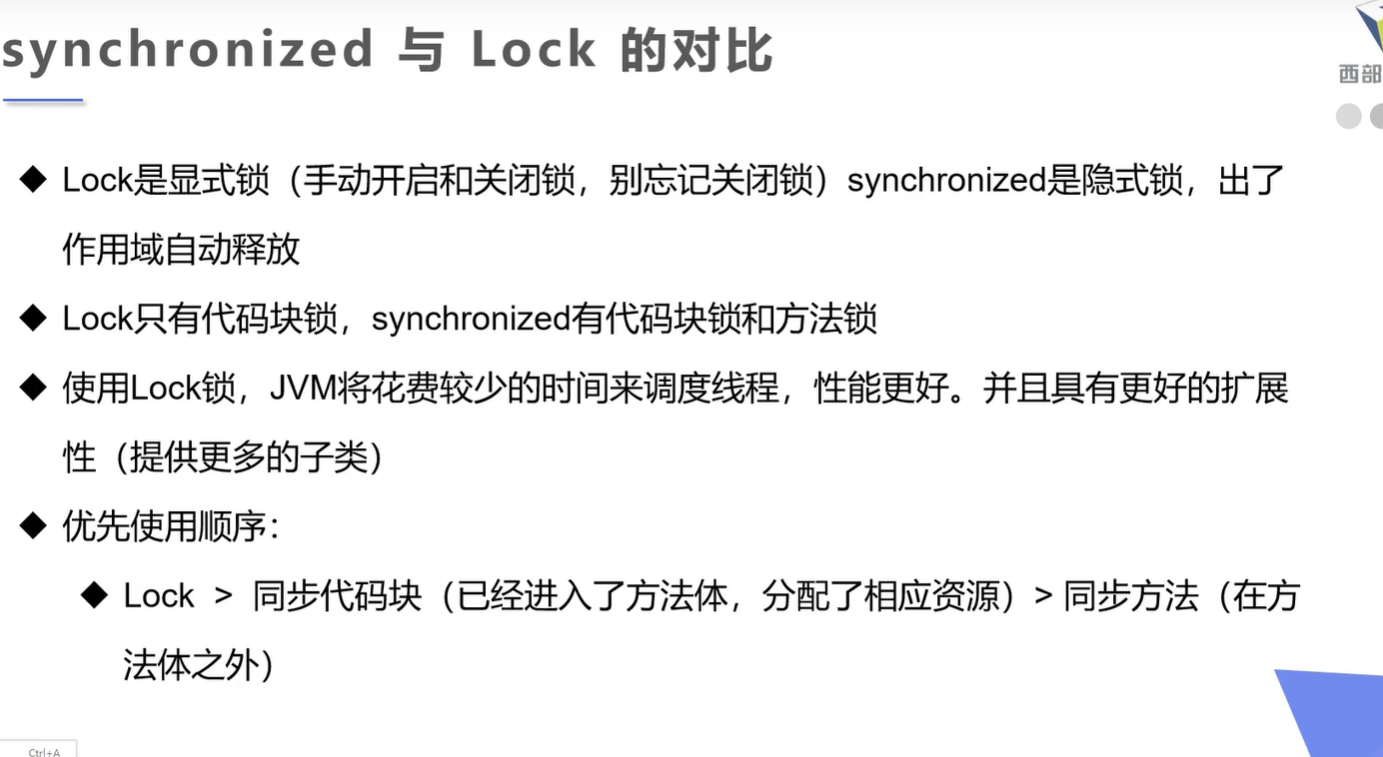
线程协作
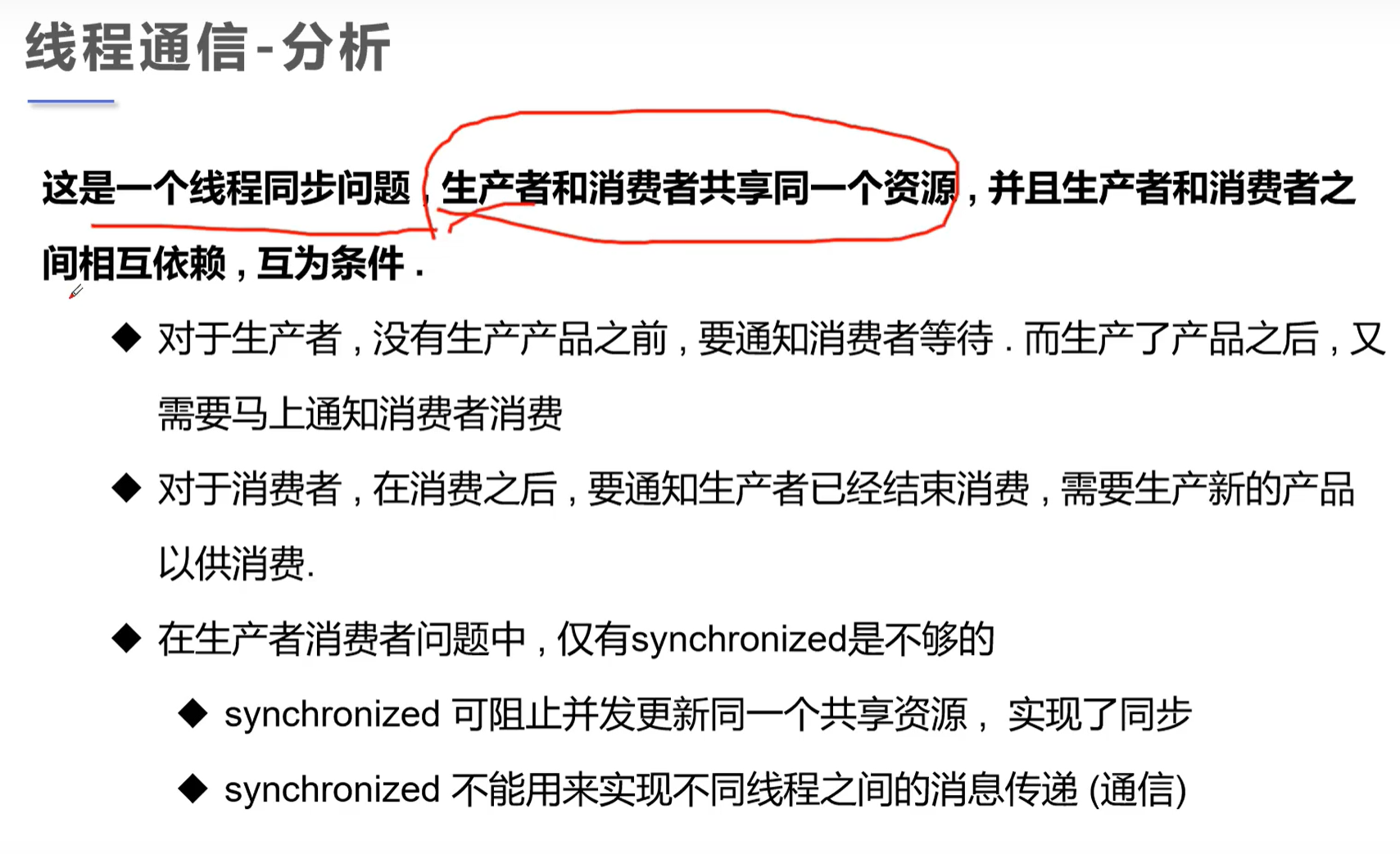
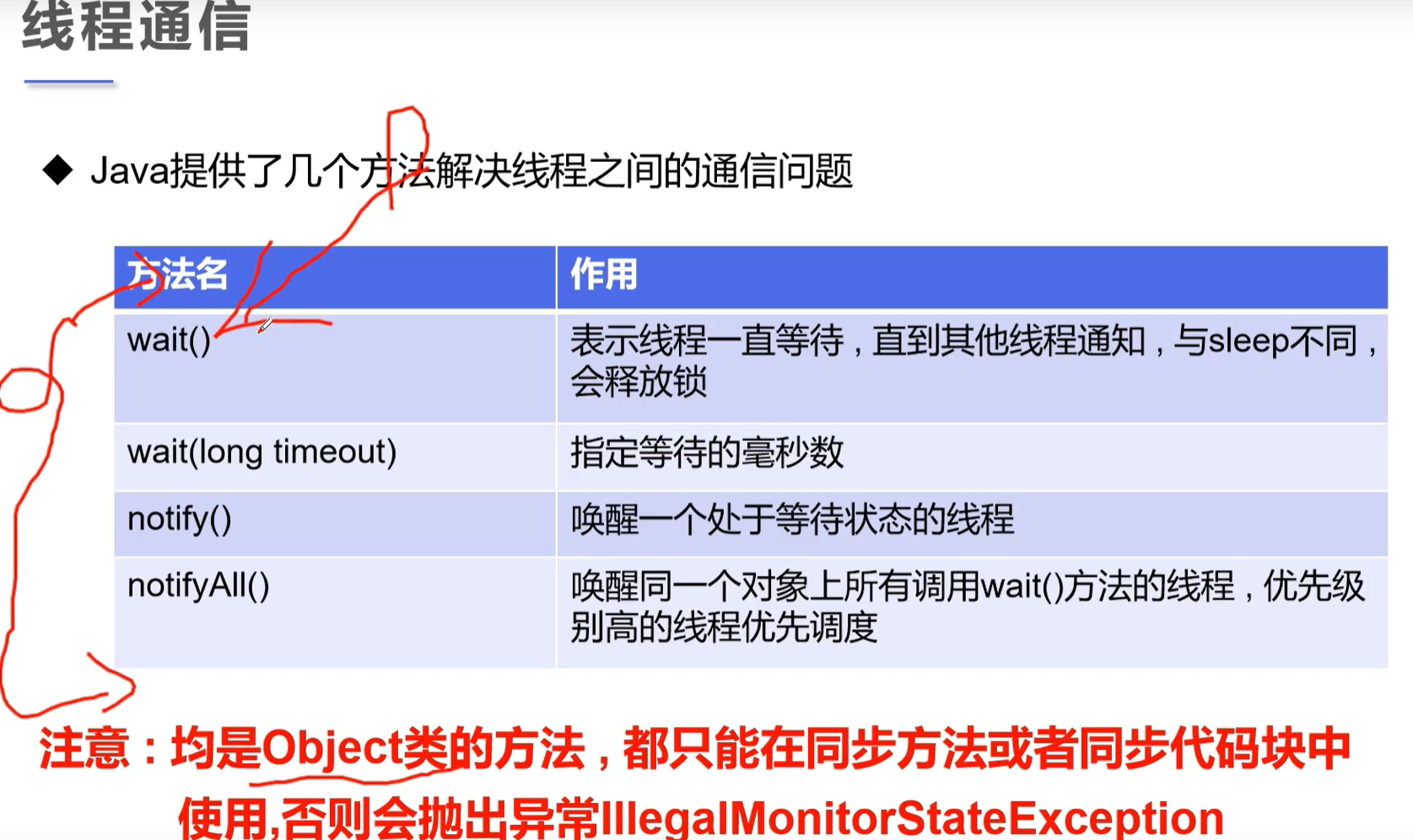
并发协作模型“生产者/消费者模型”--->管程法
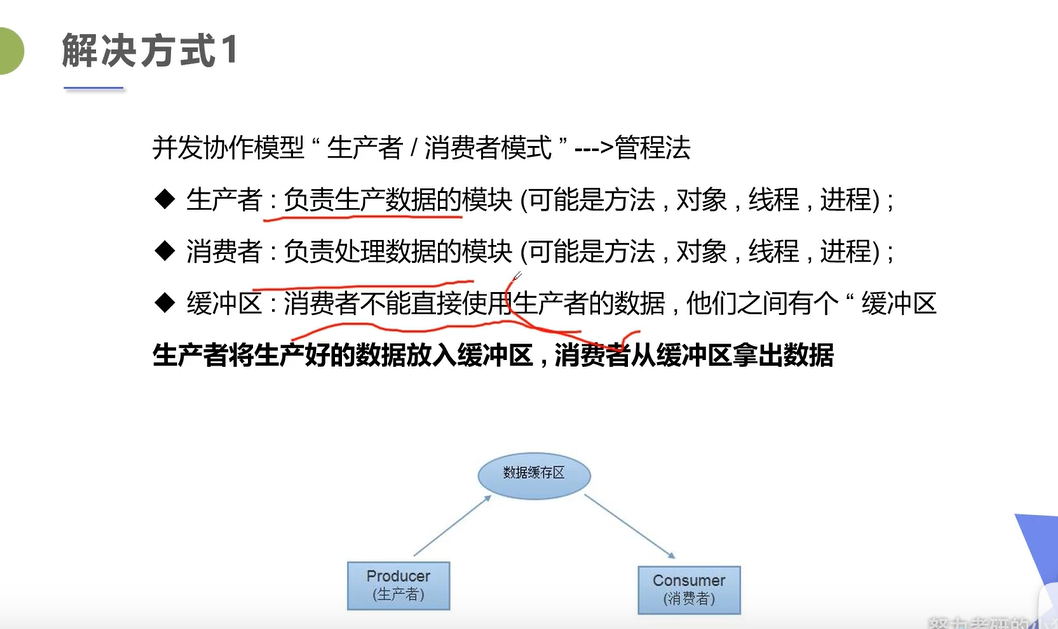
//测试:生产者消费者模型 --》 利用缓冲区解决:管程法
//生产者,消费者,产品,缓冲区
public class TestPC {
public static void main(String[] args) {
SynContainer container =new SynContainer();
new Productor(container).start();
new Consummer(container).start();
}
}
//生产者
class Productor extends Thread{
SynContainer container;
public Productor(SynContainer container){
this.container = container;
}
//生产
@Override
public void run() {
for (int i = 0; i < 100; i++) {
System.out.println("生产了"+i+"只鸡");
container.push(new Chicken(i));
}
}
}
//消费者
class Consummer extends Thread{
SynContainer container;
public Consummer(SynContainer container){
this.container =container;
}
@Override
public void run() {
for (int i = 0; i < 100; i++) {
System.out.println("消费了--》"+container.pop().id+"只鸡");
}
}
}
//产品
class Chicken{
int id;//产品编号
public Chicken(int id){
this.id =id;
}
}
//缓冲区
class SynContainer{
//需要一个容器大小
Chicken[] chickens =new Chicken[10];
//容器计数器
int count =0;
//生产者放入产品
public synchronized void push(Chicken chicken){
//如果容器满了,就需要等待消费消费
if (count == chickens.length){
//通知消费者消费,生产等待
try {
this.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
//如果没有满,我们就需要丢入产品
chickens[count] =chicken;
count++;
//可以通知消费者消费了。
this.notifyAll();
}
//消费者消费产品
public synchronized Chicken pop(){
//判断能否消费
if(count ==0){
//等待生产者生产,消费者等待
try {
this.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
//如果可以消费
count --;
Chicken chicken =chickens[count];
//吃完了,通知生产者生产
this.notifyAll();
return chicken;
}
}
生产者/消费者模式 信号灯法
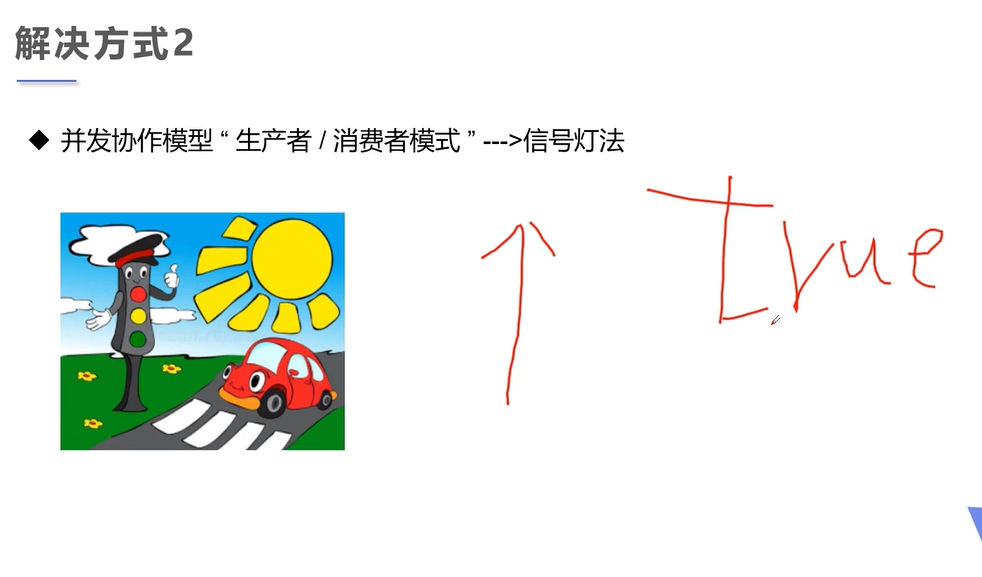
//测试生产者消费者问题2:信号灯法,标志位解决
public class TestPc2 {
public static void main(String[] args) {
TV tv =new TV();
new Player(tv).start();
new Watcher(tv).start();
}
}
//生产者 --> 演员
class Player extends Thread{
TV tv;
public Player(TV tv){
this.tv =tv;
}
@Override
public void run() {
for (int i = 0; i < 20; i++) {
if (i%2 ==0){
this.tv.play("快乐大本营播放中");
}else {
this.tv.play("抖音:记录美好生活");
}
}
}
}
//消费者 --》 观众
class Watcher extends Thread{
TV tv;
public Watcher(TV tv){
this.tv=tv;
}
@Override
public void run() {
for (int i = 0; i < 20; i++) {
tv.watch();
}
}
}
//产品 --》节目
class TV{
//演员表演,观众等待
//观众观看,演员等待
String voice; //表演的节目
boolean flag =true;
//表演
public synchronized void play(String voice){
if (!flag){
try {
this.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
System.out.println("演员表演了:"+voice);
//通知观众观看
this.notifyAll();//通知唤醒
this.voice =voice;
this.flag =!this.flag;
}
//观看
public synchronized void watch(){
if (flag){
try {
this.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
System.out.println("观看了"+voice);
//通知演员表演
this.notifyAll();
this.flag =!this.flag;
}
}
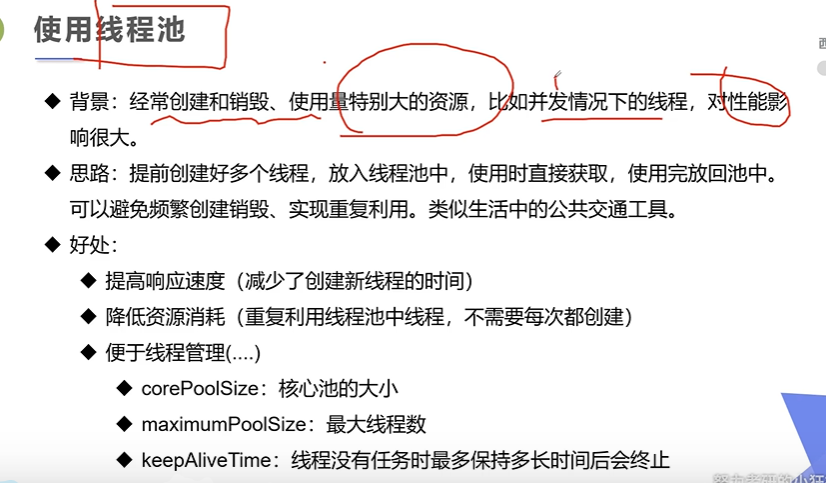
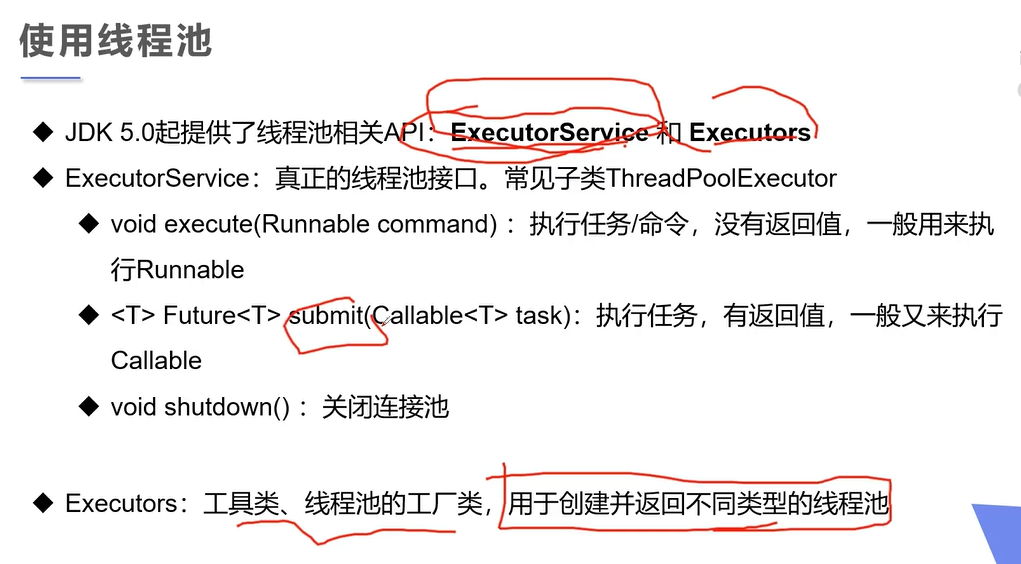
import java.util.concurrent.Executor;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class TestPool {
public static void main(String[] args) {
//1。创建服务,创建线程池
ExecutorService service = Executors.newFixedThreadPool(10);
//执行
service.execute(new Mythread());
service.execute(new Mythread());
service.execute(new Mythread());
service.execute(new Mythread());
//2.关闭连接
service.shutdown();
}
}
class Mythread implements Runnable{
@Override
public void run() {
for (int i = 0; i < 100; i++) {
System.out.println(Thread.currentThread().getName()+i);
}
}
}
总结
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.Future;
import java.util.concurrent.FutureTask;
public class ThreadNew {
public static void main(String[] args) {
new Mythread1().start();
new Thread(new Mythread2()).start();
FutureTask<Integer> futureTask =new FutureTask<Integer>(new MyThread3());
new Thread(futureTask).start();
try {
Integer integer =futureTask.get();
System.out.println(integer);
} catch (InterruptedException e) {
e.printStackTrace();
} catch (ExecutionException e) {
e.printStackTrace();
}
}
}
//1.继承Thread类
class Mythread1 extends Thread{
@Override
public void run() {
System.out.println("MyThread1");
}
}
//2.实现Runnable接口
class Mythread2 implements Runnable{
@Override
public void run() {
System.out.println("MyThread2");
}
}
//3.实现Callable接口
class MyThread3 implements Callable<Integer>{
@Override
public Integer call() throws Exception {
System.out.println("MyThread3");
return 100;
}
}