codeforces#839 B Game of the Rows 思维,贪心
Daenerys Targaryen has an army consisting of k groups of soldiers, the i-th group contains ai soldiers. She wants to bring her army to the other side of the sea to get the Iron Throne. She has recently bought an airplane to carry her army through the sea. The airplane has nrows, each of them has 8 seats. We call two seats neighbor, if they are in the same row and in seats {1, 2}, {3, 4}, {4, 5}, {5, 6} or {7, 8}.

Daenerys Targaryen wants to place her army in the plane so that there are no two soldiers from different groups sitting on neighboring seats.
Your task is to determine if there is a possible arranging of her army in the airplane such that the condition above is satisfied.
The first line contains two integers n and k (1 ≤ n ≤ 10000, 1 ≤ k ≤ 100) — the number of rows and the number of groups of soldiers, respectively.
The second line contains k integers a1, a2, a3, ..., ak (1 ≤ ai ≤ 10000), where ai denotes the number of soldiers in the i-th group.
It is guaranteed that a1 + a2 + ... + ak ≤ 8·n.
If we can place the soldiers in the airplane print "YES" (without quotes). Otherwise print "NO" (without quotes).
You can choose the case (lower or upper) for each letter arbitrary.
2 2 5 8
YES
1 2 7 1
NO
1 2 4 4
YES
1 4 2 2 1 2
YES
In the first sample, Daenerys can place the soldiers like in the figure below:
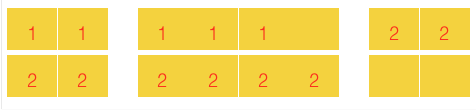
In the second sample, there is no way to place the soldiers in the plane since the second group soldier will always have a seat neighboring to someone from the first group.
In the third example Daenerys can place the first group on seats (1, 2, 7, 8), and the second group an all the remaining seats.
In the fourth example she can place the first two groups on seats (1, 2) and (7, 8), the third group on seats (3), and the fourth group on seats (5, 6).
【题意】
n 行 k组,
输入k 组数据
问 k 组人能否被安排下, 而 这k 组人不相邻
【思路】
一行8 个座位 可以分为 2 座 和 4 座
n行 2 座 为 2n 四座为 n
对于 k组数据 可以 把每组 人 分成 4+2+1 这样的 可以为0
先 处理 4 座 在处理2 座 最后处理 1 个人的
先把所有 4 座的 处理掉 剩下的 4座 就可以 座2 个人 或者 1 个人 或者 2 +1 人 不相邻
【代码实现】
//#include <bits/stdc++.h> #include <iostream> #include <stdio.h> #include <algorithm> #include <cmath> #include <math.h> #include <cstring> #include <string> #include <queue> #include <deque> #include <stack> #include <stdlib.h> #include <list> #include <map> #include <set> #include <bitset> #include <vector> #define mem(a,b) memset(a,b,sizeof(a)) #define findx(x) lower_bound(b+1,b+1+bn,x)-b #define FIN freopen("input.txt","r",stdin) #define FOUT freopen("output.txt","w",stdout) #define S1(n) scanf("%d",&n) #define SL1(n) scanf("%I64d",&n) #define S2(n,m) scanf("%d%d",&n,&m) #define SL2(n,m) scanf("%I64d%I64d",&n,&m) #define Pr(n) printf("%d\n",n) #define lson rt << 1, l, mid #define rson rt << 1|1, mid + 1, r #define FI(n) IO::read(n) #define Be IO::begin() using namespace std; typedef long long ll; const double PI=acos(-1); const int INF=0x3f3f3f3f; const double esp=1e-6; const int maxn=2e6+5; const int MAXN=5005; const int MOD=1e9+7; const int mod=1e9+7; int dir[5][2]={0,1,0,-1,1,0,-1,0}; namespace IO { const int MT = 5e7; char buf[MT]; int c,sz; void begin(){ c = 0; sz = fread(buf, 1, MT, stdin);//一次性输入 } template<class T> inline bool read(T &t){ while( c < sz && buf[c] != '-' && ( buf[c]<'0' || buf[c] >'9')) c++; if( c>=sz) return false; bool flag = 0; if( buf[c]== '-') flag = 1,c++; for( t=0; c<=sz && '0' <=buf[c] && buf[c] <= '9'; c++ ) t= t*10 + buf[c]-'0'; if(flag) t=-t; return true; } } ll inv[maxn*2]; inline void ex_gcd(ll a,ll b,ll &d,ll &x,ll &y){if(!b){ x=1; y=0; d=a; }else{ ex_gcd(b,a%b,d,y,x); y-=x*(a/b);};} inline ll gcd(ll a,ll b){ return b?gcd(b,a%b):a;} inline ll exgcd(ll a,ll b,ll &x,ll &y){if(!b){x=1;y=0;return a;}ll ans=exgcd(b,a%b,x,y);ll temp=x;x=y;y=temp-a/b*y;return ans;} inline ll lcm(ll a,ll b){ return b/gcd(a,b)*a;} inline ll qpow(ll x,ll n){ll res=1;for(;n;n>>=1){if(n&1)res=(res*x)%MOD;x=(x*x)%MOD;}return res;} inline ll inv_exgcd(ll a,ll n){ll d,x,y;ex_gcd(a,n,d,x,y);return d==1?(x+n)%n:-1;} inline ll inv1(ll b){return b==1?1:(MOD-MOD/b)*inv1(MOD%b)%MOD;} inline ll inv2(ll b){return qpow(b,MOD-2);} int a[MAXN]; int main() { int n,k; int s2,s4; while(~scanf("%d %d",&n,&k)) { for(int i=1;i<=k;i++) { scanf("%d",&a[i]); } s2= 2*n; s4=n; int flag=1; for(int i=1;i<=k;i++) { int te= min(s4,a[i]/4); // 处理4 s4-= te; a[i]-= te*4; } s2+=s4; for(int i=1;i<=k;i++) { int te=min(s2,a[i]/2); //cout<<" te " << te<<endl; s2-=te; a[i]-= te*2; } int s1=s2+s4; //cout<<s2<<endl; for(int i=1;i<=k;i++) { s1-=a[i]; // cout<<s1<<endl; } if(s1>=0) printf("YES\n"); else printf("NO\n"); } return 0; }
\123