python删除雨滴谱不需要的直径列值
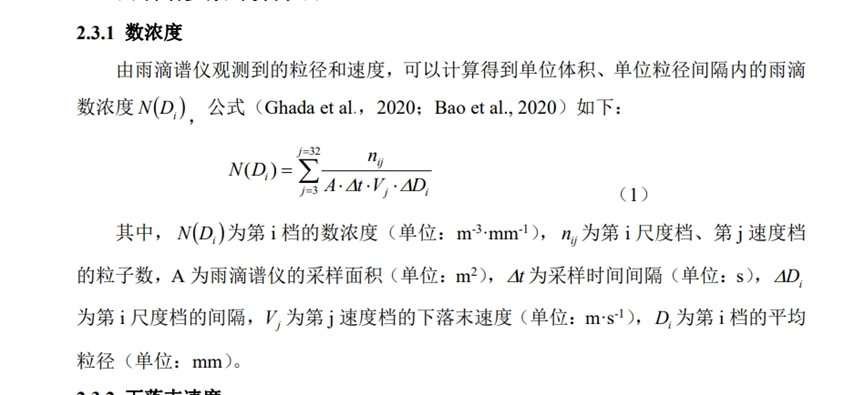
粒径的取值范围为:0.31~8mm,标记红色的都需要删除,变化直径:当前直径 — 前一个直径
txt文件为(红框为留下来的数据),一共五组数,也就是五个时间的数:
那么我只留下我需要的d列数据,删除不需要的列:
# -*- coding:utf-8 -*- """ @author: suyue @file: deletlie.py @time: 2024/05/01 @desc: """ import numpy as np import pandas as pd file_path = '/NM004-20230627224400-20230627224859-0.txt' # 读整个txt文件读取到单个字符串 with open(file_path, 'r', errors='ignore') as file: file_content = file.read() # 按时间戳拆分内容以查找单独的部分 # 时间戳的格式为 YYYY-MM-DD HH:MM:SS,因此我们将使用正则表达式根据此模式进行拆分 import re sections = re.split(r'\d{4}-\d{2}-\d{2} \d{2}:\d{2}:\d{2}\n', file_content) # print(sections) date = [x for x in file_content.split('\n') if len(x) == 19] # 如果txt第一个元素为空值(由于拆分),则将其删除 if not sections[0]: sections.pop(0) final_data = {} for i in range(len(sections)): final_data[date[i]] = sections[i] # 删除不要的列数 df_final_values = [] for key, value in final_data.items(): lines = value.strip().split('\n') matrix = [line.split() for line in lines] df = pd.DataFrame(matrix) # 删除前2列 df.drop(df.columns[:2], axis=1, inplace=True) # 删除后9列 df.drop(df.columns[-9:], axis=1, inplace=True) df_final_values.append(df.values.tolist())
# 将结果写入txt,采用追加写入的方式 index = 0 with open('/output.txt', 'w', errors='ignore') as file: for key, _ in final_data.items(): file.write(key + '\n') for df_values in df_final_values[index]: file.write('\t'.join(df_values) + '\n') index += 1 file.write('\n')
得到: