设计一个有getMin功能的栈(C++实现)
代码:
#include <iostream>
#include <stack>
# include <string>
#include <stdexcept>
using namespace std;
class my_stack
{
public:
my_stack(){};
~my_stack(){};
int my_push(int var);
int my_pop();
int getmin();
bool Empty();
private:
stack<int> stackdata;
stack<int> stackmin;
};
//实现代码
int my_stack::my_push(int var)
{
stackdata.push(var);
if(stackmin.empty()||stackmin.top()>=var)
stackmin.push(var);
return 0;
}
int my_stack::my_pop()
{
if(stackdata.empty()){
throw runtime_error("Empty Stack!");
}
int top_value = stackdata.top();//返回stackdata栈顶元素
stackdata.pop();//弹出stackdata栈顶元素
return top_value;
}
int my_stack::getmin()
{
if(stackmin.empty())
throw runtime_error("Empty Stack!");
else
{
int min_value = stackmin.top();
return min_value;
}
}
bool my_stack::Empty()
{
if(stackdata.empty())
return true;
else
return false;
}
int main()
{
my_stack mystack;
int a[] = {1,3,2,2,1,5};
for(int i=0;i<6;i++)
{
mystack.my_push(a[i]);
}
while(!mystack.Empty())
{
std::cout <<"this is stack data:"<< mystack.my_pop() << '\n';
}
std::cout <<"this is min_data_of_stackdata:"<< mystack.getmin() << '\n';
cin.get();
return 0;
}
结果:
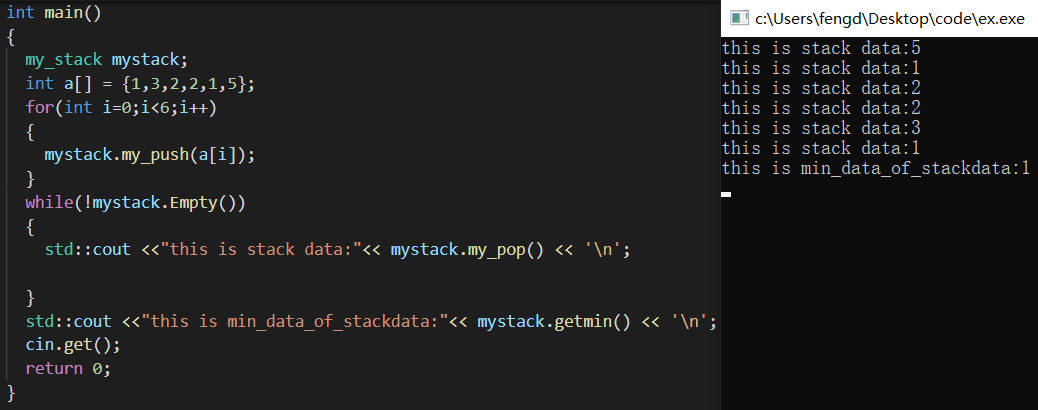