java_Excel 导出
1 package Demo; 2 3 import java.io.IOException; 4 import java.io.OutputStream; 5 import java.io.PrintWriter; 6 import java.text.SimpleDateFormat; 7 import java.util.Date; 8 9 import javax.servlet.ServletException; 10 import javax.servlet.http.HttpServlet; 11 import javax.servlet.http.HttpServletRequest; 12 import javax.servlet.http.HttpServletResponse; 13 14 import org.apache.poi.hssf.usermodel.HSSFCell; 15 import org.apache.poi.hssf.usermodel.HSSFCellStyle; 16 import org.apache.poi.hssf.usermodel.HSSFFont; 17 import org.apache.poi.hssf.usermodel.HSSFRow; 18 import org.apache.poi.hssf.usermodel.HSSFSheet; 19 import org.apache.poi.hssf.usermodel.HSSFWorkbook; 20 import org.apache.poi.hssf.util.CellRangeAddress; 21 import org.apache.poi.hssf.util.HSSFColor; 22 23 public class Excel2 extends HttpServlet { 24 25 public void doPost(HttpServletRequest request, HttpServletResponse response) 26 throws ServletException, IOException { 27 response.setContentType("text/html"); 28 PrintWriter out = response.getWriter(); 29 try { 30 response.reset(); // 必须重置一下,不然必报错 31 // 创建HSSFWorkbook对象 (Excel文档对象) 32 HSSFWorkbook hw = new HSSFWorkbook(); 33 // 创建sheet对象 (excel表单) 34 HSSFSheet sheet = hw.createSheet("成绩表"); 35 /** 36 * 基本字体 样式 居中 大小 37 */ 38 HSSFFont font = hw.createFont(); 39 font.setFontName(HSSFFont.FONT_ARIAL); 40 font.setFontHeightInPoints((short) 10); 41 /** 42 * 单元格样式style 43 */ 44 HSSFCellStyle style = hw.createCellStyle(); 45 style.setFont(font); 46 style.setBorderTop((short) 1); // 上 47 style.setBorderBottom((short) 1); // 下 48 style.setBorderLeft((short) 1); // 左 49 style.setBorderBottom((short) 1); // 右 50 style.setAlignment(HSSFCellStyle.ALIGN_CENTER); 51 style.setVerticalAlignment(HSSFCellStyle.VERTICAL_CENTER); 52 style.setWrapText(true); 53 /** 54 * 列表头 单元格样式 titlefont 55 */ 56 HSSFFont titlefont = hw.createFont(); 57 titlefont.setFontName("微软雅黑"); 58 titlefont.setFontHeightInPoints((short) 10); 59 titlefont.setBoldweight(HSSFFont.BOLDWEIGHT_BOLD); 60 /** 61 * titleCellStyle 62 */ 63 HSSFCellStyle titleCellStyle = hw.createCellStyle(); 64 titleCellStyle.setFont(titlefont); 65 titleCellStyle.setBorderTop((short) 1); // 上 66 titleCellStyle.setBorderBottom((short) 1); // 下 67 titleCellStyle.setBorderLeft((short) 1); // 左 68 titleCellStyle.setBorderBottom((short) 1); // 右 69 // 设置 对其方式 水平对齐 align center 居中 70 titleCellStyle.setAlignment(HSSFCellStyle.ALIGN_CENTER); 71 // 垂直对齐 居中 72 titleCellStyle.setVerticalAlignment(HSSFCellStyle.VERTICAL_CENTER); 73 titleCellStyle.setWrapText(true); // 是否自动换行 74 // 选择用户填充模式 75 titleCellStyle.setFillPattern(HSSFCellStyle.SOLID_FOREGROUND); 76 // 设置单元格填充样式 77 titleCellStyle.setFillForegroundColor(HSSFColor.GREY_25_PERCENT.index); 78 /** 79 * 标题头 字体 样式 headFont 80 */ 81 HSSFFont headFont = hw.createFont(); 82 headFont.setFontHeightInPoints((short) 18); // 列宽 83 headFont.setFontName("微软雅黑"); // 字体 84 headFont.setBoldweight(HSSFFont.BOLDWEIGHT_BOLD); // 粗体 85 /** 86 * headStyle 87 */ 88 HSSFCellStyle headStyle = hw.createCellStyle(); 89 headStyle.setFont(headFont); 90 // 段落对其方式 ALIGN_CENTER_SELECTION 居中 91 headStyle.setAlignment(HSSFCellStyle.ALIGN_CENTER_SELECTION); 92 93 // 在sheet里创建第一行,,参数为行的索引(excel的行) 可以死0~65535之间的任意 94 HSSFRow headRow = sheet.createRow(0); 95 // 创建单元格 参数为列索引 ,可以死0~255之间的任意 96 HSSFCell cell = headRow.createCell(0); 97 cell.setCellValue("学生表"); // 设置单元格内容 98 // 合并单元格 CellRangeAddress() 构造参数依次表示 起始行 截止行 起始列 截止列 99 sheet.addMergedRegion(new CellRangeAddress(0, 0, 0, 6)); 100 cell.setCellStyle(headStyle); 101 // 创建第二行 102 HSSFRow row2 = sheet.createRow(1); 103 String[] arr = { "年级", "班级", "姓名", "性别", "年龄", "职务", "成绩", }; 104 /** 105 * 为单元格赋值(列名) 106 */ 107 for (int i = 0; i < arr.length; i++) { 108 HSSFCell cell2 = row2.createCell((short) i);// 创建单元格 i第几个(索引) 109 sheet.setColumnWidth(i, 5000); // 列宽 110 cell2.setCellValue((String) arr[i]); // 列名 111 cell2.setCellStyle(titleCellStyle); // 列样式 112 } 113 /** 114 * 数据集 115 */ 116 String[][] arrs = { 117 { "高一", "六班", "阿杰", "男", "18", "劳动委员", "86" }, 118 { "高二", "七班", "阿明", "男", "19", "体育委员", "54" }, 119 { "高三", "八班", "阿敏", "女", "20", "文艺委员", "56" }, 120 { "初一", "九班", "阿梅", "女", "18", "生活委员", "78" }, 121 { "高四", "十班", "阿刚", "男", "19", "纪录委员", "65" } 122 }; 123 int createRow = 2; 124 /** 125 * 为单元格赋值 赋样式 126 */ 127 for (int i = 0; i < arrs.length; i++) { 128 //创建行 前面占了两行 现在从第三行 算起 129 HSSFRow GetRow = sheet.createRow(createRow); 130 GetRow.setHeight((short) 400); //单元格height高度 131 int count = 0; 132 HSSFCell SetCell = null; //创建单元格 133 for (int j = 0; j < arrs[i].length; j++) { 134 String string = arrs[i][j]; //得到单元格数据 135 SetCell = GetRow.createCell(count); //创建单元格到第几列 136 SetCell.setCellStyle(style);// 设置当前单元格的样式 137 SetCell.setCellValue(string);// 设置当前单元格的显示文本 138 count++; // 列单元格索引 139 } 140 createRow++; // 行单元格索引 141 } 142 143 //日期: 左对齐 144 HSSFRow RowTime = sheet.createRow(createRow+4);//创建一行 145 HSSFCell cellTime = RowTime.createCell(arr.length-1);//创建单元格 146 HSSFCellStyle timeStyle = hw.createCellStyle(); //创建单元格样式 147 timeStyle.setAlignment((short) HSSFCellStyle.ALIGN_LEFT);//文字左对齐 148 cellTime.setCellStyle(timeStyle); //设置单元格样式 149 cellTime.setCellValue("日期:"); //设置value 150 //2015-01-31 左对齐 151 HSSFRow EndRow = sheet.createRow(createRow+5); 152 HSSFCell EndCell = EndRow.createCell(arr.length-1); 153 HSSFCellStyle endStyle=hw.createCellStyle(); 154 endStyle.setAlignment((short) HSSFCellStyle.ALIGN_LEFT);//文字左对齐 155 endStyle.setFillPattern(HSSFCellStyle.SOLID_FOREGROUND);//单元格颜色自定义 156 endStyle.setFillForegroundColor(HSSFColor.RED.index); //设定颜色 157 EndCell.setCellStyle(endStyle); 158 /** 159 * 获取 本地时间(服务器时间) 160 */ 161 Date now = new Date(); 162 SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy/MM/dd HH:mm:ss"); 163 EndCell.setCellValue(dateFormat.format(now)); 164 // 输出Excel 165 OutputStream outputStream = response.getOutputStream(); 166 response.setHeader("Content-Disposition", "attachment;filename=" 167 + System.currentTimeMillis() + ".xls");// filename 保存文件的名字 168 // 设置ASP输出的文档MIME类型 msexcel 表格 169 response.setContentType("application/msexcel"); 170 hw.write(outputStream); // 写 171 } catch (RuntimeException e) { 172 // TODO Auto-generated catch block 173 e.printStackTrace(); 174 } 175 out.flush(); 176 out.close(); 177 } 178 }
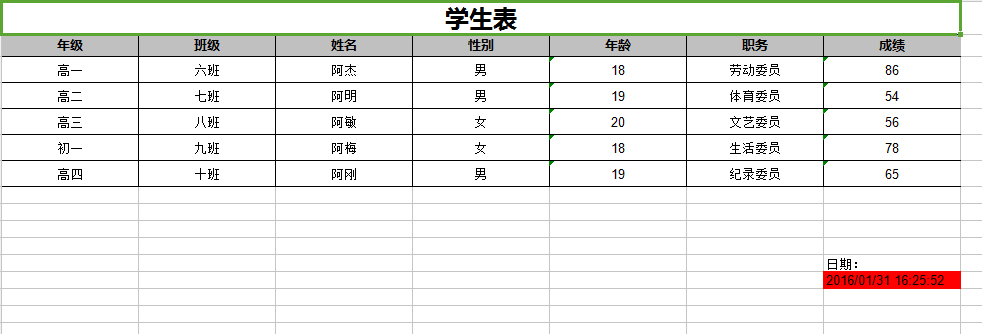