UVA 673 Parentheses Balance 解题心得
原题
Description
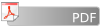
You are given a string consisting of parentheses () and []. A string of this type is said to be correct:
- (a)
- if it is the empty string
- (b)
- if A and B are correct, AB is correct,
- (c)
- if A is correct, (A ) and [A ] is correct.
Write a program that takes a sequence of strings of this type and check their correctness. Your program can assume that the maximum string length is 128.
Input
The file contains a positive integer n and a sequence of n strings of parentheses () and [], one string a line.
Output
A sequence of Yes or No on the output file.
Sample Input
3 ([]) (([()]))) ([()[]()])()
Sample Output
Yes No Yes
分析:简单题,直接用栈就可以
我的代码:
1 #include<iostream> 2 #include<stack> 3 #include<cstring> 4 #include<cstdio> 5 using namespace std; 6 7 char s[150]; 8 9 bool ifpop(const char a, const char b) 10 { 11 if (a == '('&&b == ')') return 1; 12 if (a == '['&&b == ']') return 1; 13 return 0; 14 } 15 16 17 int main() 18 { 19 int t; 20 cin >> t; 21 getchar(); 22 while (t--) 23 { 24 stack<char> z; 25 gets(s); 26 if (s[0] == '\0') 27 { 28 puts("Yes"); 29 continue; 30 } 31 else 32 { 33 for (int i = 0;s[i]!='\0'; i++) 34 { 35 if (z.empty()) 36 { 37 z.push(s[i]); 38 } 39 else 40 { 41 if (ifpop(z.top(), s[i])) 42 { 43 z.pop(); 44 } 45 else 46 { 47 z.push(s[i]); 48 } 49 } 50 } 51 if (z.empty()) puts("Yes"); 52 else puts("No"); 53 } 54 55 56 } 57 return 0; 58 }