通过反射访问构造方法代码分析
package Test; public class Example_01 { String s; //声明String型成员变量 int i, i2, i3; //声明int型成员变量 private Example_01() { //无参构造 } protected Example_01(String s, int i) { //两个参数构造方法 this.s = s; this.i = i; } public Example_01(String... strings) throws NumberFormatException { //不定长参数构造 if (0 < strings.length) i = Integer.valueOf(strings[0]); if (1 < strings.length) i2 = Integer.valueOf(strings[1]); if (2 < strings.length) i3 = Integer.valueOf(strings[2]); } public void print() { //打印参数值 System.out.println("s=" + s); System.out.println("i=" + i); System.out.println("i2=" + i2); System.out.println("i3=" + i3); } }
package Test; import java.lang.reflect.*; public class Main_01 { public static void main(String[] args) { Example_01 example = new Example_01("10", "20", "30"); //创建Example_01类对象example Class<? extends Example_01> exampleC = example.getClass(); //获得class对象 Constructor[] declaredConstructors = exampleC.getDeclaredConstructors(); //获得构造方法数组 for (int i = 0; i < declaredConstructors.length; i++) { //遍历数组获得构造方法 Constructor<?> constructor = declaredConstructors[i]; //获得构造方法constructor System.out.println("查看是否允许带有可变数量的参数:" + constructor.isVarArgs());//利用constructor.isVarArgs()方法判断是够有不定长参数的构造方法 System.out.println("该构造方法的入口参数类型依次为:"); Class[] parameterTypes = constructor.getParameterTypes();//利用constructor.getParameterTypes()获得参数类型数组 for (int j = 0; j < parameterTypes.length; j++) { System.out.println(" " + parameterTypes[j]);//遍历获得每个参数类型 } System.out.println("该构造方法可能抛出的异常类型为:"); Class[] exceptionTypes = constructor.getExceptionTypes();//利用constructor.getExceptionTypes()获得异常类型数组 for (int j = 0; j < exceptionTypes.length; j++) { System.out.println(" " + exceptionTypes[j]);//遍历获得每个异常类型 } Example_01 example2 = null; //声明循环变量 while (example2 == null) { //进入循环,当example2不重新赋值时,无限循环 try { if (i == 2) example2 = (Example_01) constructor.newInstance();//利用constructor.newInstance()创建Example_01类对象并赋给example2使其不是null退出循环; else if (i == 1) example2 = (Example_01) constructor.newInstance("7", 5);//利用constructor.newInstance(String s,int i)创建Example_01类对象并赋给example2使其不是null退出循环; else { Object[] parameters = new Object[] { new String[] { "100", "200", "300" } }; example2 = (Example_01) constructor .newInstance(parameters);//利用constructor.newInstance(String... strings)创建Example_01类对象并赋给example2使其不是null退出循环; } } catch (Exception e) {//当对象的访问权限为private时 System.out.println("在创建对象时抛出异常,下面执行setAccessible()方法"); constructor.setAccessible(true);//加入setAccessible(true)方法重新循环 } } if(example2!=null){//退出循环example2!=null后成立 example2.print();//打印新建的example2对象 System.out.println("");//在三个构造方法访问结果中加入分行 } } } }
运行结果:
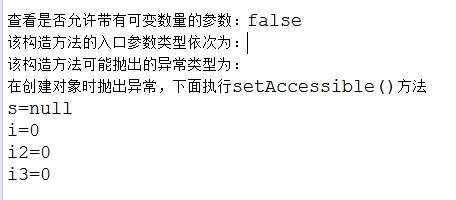
主要注意各个方法的返回值类型和使用方法;然后就是调用构造方法创建对象过程中循环方式的理解!