Ultra-QuickSort - poj 2299 (归并排序+统计逆序数)
利用归并排序统计逆序数,利用归并求逆序在对子序列s1和s2在归并时(s1,s2已经排好序),若s1[i]>s2[j](逆序状况),则逆序数加上s1.length-i,因为s1中i后面的数字对于s2[j]都是逆序的。
1 #include <stdio.h> 2 #include <stdlib.h> 3 int N; 4 int num[500001]; 5 int tmp[500001]; 6 __int64 count; 7 void Merge(int l,int mid,int r){ 8 int i=l,j=mid+1,k=0; 9 while(i<=mid&&j<=r){ 10 if(num[i]>num[j]) { 11 tmp[k++]=num[j++]; 12 count+=mid-i+1; 13 } else 14 { 15 tmp[k++]=num[i++]; 16 } 17 } 18 while(i<=mid) tmp[k++]=num[i++]; 19 while(j<=r) tmp[k++]=num[j++]; 20 21 for(i=0; i<k; i++) 22 { 23 num[l+i]=tmp[i]; 24 } 25 } 26 void Mergesort(int l,int r){ 27 int mid=(l+r)/2; 28 if(l<r){ 29 Mergesort(l,mid); 30 Mergesort(mid+1,r); 31 Merge(l,mid,r); 32 } 33 } 34 int main(void) { 35 scanf("%d",&N); 36 int i=0; 37 while(N){ 38 for(i=0;i<N;i++){ 39 scanf("%d",&num[i]); 40 } 41 count=0; 42 Mergesort(0,N-1); 43 printf("%I64d \n",count); 44 scanf("%d",&N); 45 } 46 47 48 49 return EXIT_SUCCESS; 50 }
附:
Time Limit: 7000MS | Memory Limit: 65536K | |
Total Submissions: 48082 | Accepted: 17536 |
Description
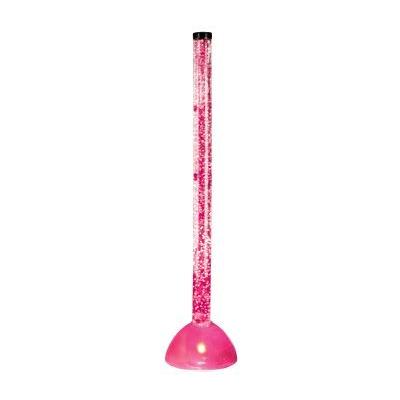
Ultra-QuickSort produces the output
Your task is to determine how many swap operations Ultra-QuickSort needs to perform in order to sort a given input sequence.
Input
The input contains several test cases. Every test case begins with a line that contains a single integer n < 500,000 -- the length of the input sequence. Each of the the following n lines contains a single integer 0 ≤ a[i] ≤ 999,999,999, the i-th input sequence element. Input is terminated by a sequence of length n = 0. This sequence must not be processed.
Output
For every input sequence, your program prints a single line containing an integer number op, the minimum number of swap operations necessary to sort the given input sequence.
Sample Input
5 9 1 0 5 4 3 1 2 3 0
Sample Output
6 0