Python 格式化输出:f-string
简介
f-string,亦称为格式化字符串常量(formatted string literals),是Python3.6新引入的一种字符串格式化方法,该方法源于PEP 498 – Literal String Interpolation,主要目的是使格式化字符串的操作更加简便。f-string在形式上是以 f 或 F 修饰符引领的字符串(f'xxx' 或 F'xxx'),以大括号 {} 标明被替换的字段;f-string在本质上并不是字符串常量,而是一个在运行时运算求值的表达式:
While other string literals always have a constant value, formatted strings are really expressions evaluated at run time.
(与具有恒定值的其它字符串常量不同,格式化字符串实际上是运行时运算求值的表达式。)
—— Python Documentation
f-string在功能方面不逊于传统的%-formatting语句和str.format()函数,同时性能又优于二者,且使用起来也更加简洁明了,因此对于Python3.6及以后的版本,推荐使用f-string进行字符串格式化。
用法
此部分内容主要参考以下资料:
Python Documentation – Formatted String Literals
Python Documentation – Format String Syntax
PEP 498 – Literal String Interpolation
Python 3’s f-Strings: An Improved String Formatting Syntax (Guide)
python3 f-string格式化字符串的高级用法
Python 3: An Intro to f-strings
简单使用
f-string用大括号 {} 表示被替换字段,其中直接填入替换内容:
>>> name = 'Eric'
>>> f'Hello, my name is {name}'
'Hello, my name is Eric'
>>> number = 7
>>> f'My lucky number is {number}'
'My lucky number is 7'
>>> price = 19.99
>>> f'The price of this book is {price}'
'The price of this book is 19.99'
表达式求值与函数调用
f-string的大括号 {} 可以填入表达式或调用函数,Python会求出其结果并填入返回的字符串内:
>>> f'A total number of {24 * 8 + 4}'
'A total number of 196'
>>> f'Complex number {(2 + 2j) / (2 - 3j)}'
'Complex number (-0.15384615384615388+0.7692307692307692j)'
>>> name = 'ERIC'
>>> f'My name is {name.lower()}'
'My name is eric'
>>> import math
>>> f'The answer is {math.log(math.pi)}'
'The answer is 1.1447298858494002'
引号、大括号与反斜杠
f-string大括号内所用的引号不能和大括号外的引号定界符冲突,可根据情况灵活切换 ' 和 ":
>>> f'I am {"Eric"}'
'I am Eric'
>>> f'I am {'Eric'}'
File "<stdin>", line 1
f'I am {'Eric'}'
^
SyntaxError: invalid syntax
若 ' 和 " 不足以满足要求,还可以使用 ''' 和 """:
>>> f"He said {"I'm Eric"}"
File "<stdin>", line 1
f"He said {"I'm Eric"}"
^
SyntaxError: invalid syntax
>>> f'He said {"I'm Eric"}'
File "<stdin>", line 1
f'He said {"I'm Eric"}'
^
SyntaxError: invalid syntax
>>> f"""He said {"I'm Eric"}"""
"He said I'm Eric"
>>> f'''He said {"I'm Eric"}'''
"He said I'm Eric"
大括号外的引号还可以使用 \ 转义,但大括号内不能使用 \ 转义:
>>> f'''He\'ll say {"I'm Eric"}'''
"He'll say I'm Eric"
>>> f'''He'll say {"I\'m Eric"}'''
File "<stdin>", line 1
SyntaxError: f-string expression part cannot include a backslash
1
2
3
4
5
f-string大括号外如果需要显示大括号,则应输入连续两个大括号 {{ 和 }}:
>>> f'5 {"{stars}"}'
'5 {stars}'
>>> f'{{5}} {"stars"}'
'{5} stars'
上面提到,f-string大括号内不能使用 \ 转义,事实上不仅如此,f-string大括号内根本就不允许出现 \。如果确实需要 \,则应首先将包含 \ 的内容用一个变量表示,再在f-string大括号内填入变量名:
>>> f"newline: {ord('\n')}"
File "<stdin>", line 1
SyntaxError: f-string expression part cannot include a backslash
>>> newline = ord('\n')
>>> f'newline: {newline}'
'newline: 10'
多行f-string
f-string还可用于多行字符串:
>>> name = 'Eric'
>>> age = 27
>>> f"Hello!" \
... f"I'm {name}." \
... f"I'm {age}."
"Hello!I'm Eric.I'm 27."
>>> f"""Hello!
... I'm {name}.
... I'm {age}."""
"Hello!\n I'm Eric.\n I'm 27."
自定义格式:对齐、宽度、符号、补零、精度、进制等
f-string采用 {content:format} 设置字符串格式,其中 content 是替换并填入字符串的内容,可以是变量、表达式或函数等,format 是格式描述符。采用默认格式时不必指定 {:format},如上面例子所示只写 {content} 即可。
关于格式描述符的详细语法及含义可查阅Python官方文档,这里按使用时的先后顺序简要介绍常用格式描述符的含义与作用:
对齐相关格式描述符
格式描述符含义与作用
<左对齐(字符串默认对齐方式)
>右对齐(数值默认对齐方式)
^居中
数字符号相关格式描述符
格式描述符含义与作用
+负数前加负号(-),正数前加正号(+)
-负数前加负号(-),正数前不加任何符号(默认)
(空格)负数前加负号(-),正数前加一个空格
注:仅适用于数值类型。
数字显示方式相关格式描述符
格式描述符含义与作用
#切换数字显示方式
注1:仅适用于数值类型。
注2:# 对不同数值类型的作用效果不同,详见下表:
数值类型不加#(默认)加#区别
二进制整数'1111011''0b1111011'开头是否显示 0b
八进制整数'173''0o173'开头是否显示 0o
十进制整数'123''123'无区别
十六进制整数(小写字母)'7b''0x7b'开头是否显示 0x
十六进制整数(大写字母)'7B''0X7B'开头是否显示 0X
宽度与精度相关格式描述符
格式描述符含义与作用
width整数 width 指定宽度
0width整数 width 指定宽度,开头的 0 指定高位用 0 补足宽度
width.precision整数 width 指定宽度,整数 precision 指定显示精度
注1:0width 不可用于复数类型和非数值类型,width.precision 不可用于整数类型。
注2:width.precision 用于不同格式类型的浮点数、复数时的含义也不同:用于 f、F、e、E 和 % 时 precision 指定的是小数点后的位数,用于 g 和 G 时 precision 指定的是有效数字位数(小数点前位数+小数点后位数)。
注3:width.precision 除浮点数、复数外还可用于字符串,此时 precision 含义是只使用字符串中前 precision 位字符。
示例:
>>> a = 123.456
>>> f'a is {a:8.2f}'
'a is 123.46'
>>> f'a is {a:08.2f}'
'a is 00123.46'
>>> f'a is {a:8.2e}'
'a is 1.23e+02'
>>> f'a is {a:8.2%}'
'a is 12345.60%'
>>> f'a is {a:8.2g}'
'a is 1.2e+02'
>>> s = 'hello'
>>> f's is {s:8s}'
's is hello '
>>> f's is {s:8.3s}'
's is hel '
千位分隔符相关格式描述符
格式描述符含义与作用
,使用,作为千位分隔符
_使用_作为千位分隔符
注1:若不指定 , 或 _,则f-string不使用任何千位分隔符,此为默认设置。
注2:, 仅适用于浮点数、复数与十进制整数:对于浮点数和复数,, 只分隔小数点前的数位。
注3:_ 适用于浮点数、复数与二、八、十、十六进制整数:对于浮点数和复数,_ 只分隔小数点前的数位;对于二、八、十六进制整数,固定从低位到高位每隔四位插入一个 _(十进制整数是每隔三位插入一个 _)。
示例:
>>> a = 1234567890.098765
>>> f'a is {a:f}'
'a is 1234567890.098765'
>>> f'a is {a:,f}'
'a is 1,234,567,890.098765'
>>> f'a is {a:_f}'
'a is 1_234_567_890.098765'
>>> b = 1234567890
>>> f'b is {b:_b}'
'b is 100_1001_1001_0110_0000_0010_1101_0010'
>>> f'b is {b:_o}'
'b is 111_4540_1322'
>>> f'b is {b:_d}'
'b is 1_234_567_890'
>>> f'b is {b:_x}'
'b is 4996_02d2'
格式类型相关格式描述符
基本格式类型
格式描述符含义与作用适用变量类型
s普通字符串格式字符串
b二进制整数格式整数
c字符格式,按unicode编码将整数转换为对应字符整数
d十进制整数格式整数
o八进制整数格式整数
x十六进制整数格式(小写字母)整数
X十六进制整数格式(大写字母)整数
e科学计数格式,以 e 表示 ×10^浮点数、复数、整数(自动转换为浮点数)
E与 e 等价,但以 E 表示 ×10^浮点数、复数、整数(自动转换为浮点数)
f定点数格式,默认精度(precision)是6浮点数、复数、整数(自动转换为浮点数)
F与 f 等价,但将 nan 和 inf 换成 NAN 和 INF浮点数、复数、整数(自动转换为浮点数)
g通用格式,小数用 f,大数用 e浮点数、复数、整数(自动转换为浮点数)
G与 G 等价,但小数用 F,大数用 E浮点数、复数、整数(自动转换为浮点数)
%百分比格式,数字自动乘上100后按 f 格式排版,并加 % 后缀浮点数、整数(自动转换为浮点数)
常用的特殊格式类型:标准库 datetime 给定的用于排版时间信息的格式类型,适用于 date、datetime 和 time 对象
格式描述符含义显示样例
%a星期几(缩写)'Sun'
%A星期几(全名)'Sunday'
%w星期几(数字,0 是周日,6 是周六)'0'
%u星期几(数字,1 是周一,7 是周日)'7'
%d日(数字,以 0 补足两位)'07'
%b月(缩写)'Aug'
%B月(全名)'August'
%m月(数字,以 0 补足两位)'08'
%y年(后两位数字,以 0 补足两位)'14'
%Y年(完整数字,不补零)'2014'
%H小时(24小时制,以 0 补足两位)'23'
%I小时(12小时制,以 0 补足两位)'11'
%p上午/下午'PM'
%M分钟(以 0 补足两位)'23'
%S秒钟(以 0 补足两位)'56'
%f微秒(以 0 补足六位)'553777'
%zUTC偏移量(格式是 ±HHMM[SS],未指定时区则返回空字符串)'+1030'
%Z时区名(未指定时区则返回空字符串)'EST'
%j一年中的第几天(以 0 补足三位)'195'
%U一年中的第几周(以全年首个周日后的星期为第0周,以 0 补足两位)'27'
%w一年中的第几周(以全年首个周一后的星期为第0周,以 0 补足两位)'28'
%V一年中的第几周(以全年首个包含1月4日的星期为第1周,以 0 补足两位)'28'
综合示例
>>> a = 1234
>>> f'a is {a:^#10X}' # 居中,宽度10位,十六进制整数(大写字母),显示0X前缀
'a is 0X4D2 '
>>> b = 1234.5678
>>> f'b is {b:<+10.2f}' # 左对齐,宽度10位,显示正号(+),定点数格式,2位小数
'b is +1234.57 '
>>> c = 12345678
>>> f'c is {c:015,d}' # 高位补零,宽度15位,十进制整数,使用,作为千分分割位
'c is 000,012,345,678'
>>> d = 0.5 + 2.5j
>>> f'd is {d:30.3e}' # 宽度30位,科学计数法,3位小数
'd is 5.000e-01+2.500e+00j'
>>> import datetime
>>> e = datetime.datetime.today()
>>> f'the time is {e:%Y-%m-%d (%a) %H:%M:%S}' # datetime时间格式
'the time is 2018-07-14 (Sat) 20:46:02'
lambda表达式
f-string大括号内也可填入lambda表达式,但lambda表达式的 : 会被f-string误认为是表达式与格式描述符之间的分隔符,为避免歧义,需要将lambda表达式置于括号 () 内:
>>> f'result is {lambda x: x ** 2 + 1 (2)}'
File "<fstring>", line 1
(lambda x)
^
SyntaxError: unexpected EOF while parsing
>>> f'result is {(lambda x: x ** 2 + 1) (2)}'
'result is 5'
>>> f'result is {(lambda x: x ** 2 + 1) (2):<+7.2f}'
'result is +5.00 '
————————————————
版权声明:本文为CSDN博主「sunxb10」的原创文章,遵循CC 4.0 BY-SA版权协议,转载请附上原文出处链接及本声明。
原文链接:https://blog.csdn.net/sunxb10/article/details/81036693
目录
%用法
1、整数的输出
%o —— oct 八进制
%d —— dec 十进制
%x —— hex 十六进制
1 >>> print('%o' % 20) 2 24 3 >>> print('%d' % 20) 4 20 5 >>> print('%x' % 20) 6 14
2、浮点数输出
(1)格式化输出
%f ——保留小数点后面六位有效数字
%.3f,保留3位小数位
%e ——保留小数点后面六位有效数字,指数形式输出
%.3e,保留3位小数位,使用科学计数法
%g ——在保证六位有效数字的前提下,使用小数方式,否则使用科学计数法
%.3g,保留3位有效数字,使用小数或科学计数法
1 >>> print('%f' % 1.11) # 默认保留6位小数 2 1.110000 3 >>> print('%.1f' % 1.11) # 取1位小数 4 1.1 5 >>> print('%e' % 1.11) # 默认6位小数,用科学计数法 6 1.110000e+00 7 >>> print('%.3e' % 1.11) # 取3位小数,用科学计数法 8 1.110e+00 9 >>> print('%g' % 1111.1111) # 默认6位有效数字 10 1111.11 11 >>> print('%.7g' % 1111.1111) # 取7位有效数字 12 1111.111 13 >>> print('%.2g' % 1111.1111) # 取2位有效数字,自动转换为科学计数法 14 1.1e+03
(2)内置round()
round(number[, ndigits])
参数:
number - 这是一个数字表达式。
ndigits - 表示从小数点到最后四舍五入的位数。默认值为0。
返回值
该方法返回x的小数点舍入为n位数后的值。
round()函数只有一个参数,不指定位数的时候,返回一个整数,而且是最靠近的整数,类似于四舍五入,当指定取舍的小数点位数的时候,一般情况也是使用四舍五入的规则,但是碰到.5的情况时,如果要取舍的位数前的小数是奇数,则直接舍弃,如果是偶数则向上取舍。
注:“.5”这个是一个“坑”,且python2和python3出来的接口有时候是不一样的,尽量避免使用round()函数吧
1 >>> round(1.1125) # 四舍五入,不指定位数,取整 2 1 3 >>> round(1.1135,3) # 取3位小数,由于3为奇数,则向下“舍” 4 1.113 5 >>> round(1.1125,3) # 取3位小数,由于2为偶数,则向上“入” 6 1.113 7 >>> round(1.5) # 无法理解,查阅一些资料是说python会对数据进行截断,没有深究 8 2 9 >>> round(2.5) # 无法理解 10 2 11 >>> round(1.675,2) # 无法理解 12 1.68 13 >>> round(2.675,2) # 无法理解 14 2.67 15 >>>
3、字符串输出
%s
%10s——右对齐,占位符10位
%-10s——左对齐,占位符10位
%.2s——截取2位字符串
%10.2s——10位占位符,截取两位字符串
1 >>> print('%s' % 'hello world') # 字符串输出 2 hello world 3 >>> print('%20s' % 'hello world') # 右对齐,取20位,不够则补位 4 hello world 5 >>> print('%-20s' % 'hello world') # 左对齐,取20位,不够则补位 6 hello world 7 >>> print('%.2s' % 'hello world') # 取2位 8 he 9 >>> print('%10.2s' % 'hello world') # 右对齐,取2位 10 he 11 >>> print('%-10.2s' % 'hello world') # 左对齐,取2位 12 he
4、 其他
(1)字符串格式代码
(2)常用转义字符
format用法
相对基本格式化输出采用‘%’的方法,format()功能更强大,该函数把字符串当成一个模板,通过传入的参数进行格式化,并且使用大括号‘{}’作为特殊字符代替‘%’
位置匹配
(1)不带编号,即“{}”
(2)带数字编号,可调换顺序,即“{1}”、“{2}”
(3)带关键字,即“{a}”、“{tom}”
1 >>> print('{} {}'.format('hello','world')) # 不带字段 2 hello world 3 >>> print('{0} {1}'.format('hello','world')) # 带数字编号 4 hello world 5 >>> print('{0} {1} {0}'.format('hello','world')) # 打乱顺序 6 hello world hello 7 >>> print('{1} {1} {0}'.format('hello','world')) 8 world world hello 9 >>> print('{a} {tom} {a}'.format(tom='hello',a='world')) # 带关键字 10 world hello world

>>> '{0}, {1}, {2}'.format('a', 'b', 'c') 'a, b, c' >>> '{}, {}, {}'.format('a', 'b', 'c') # 3.1+版本支持 'a, b, c' >>> '{2}, {1}, {0}'.format('a', 'b', 'c') 'c, b, a' >>> '{2}, {1}, {0}'.format(*'abc') # 可打乱顺序 'c, b, a' >>> '{0}{1}{0}'.format('abra', 'cad') # 可重复 'abracadabra'

>>> 'Coordinates: {latitude}, {longitude}'.format(latitude='37.24N', longitude='-115.81W') 'Coordinates: 37.24N, -115.81W' >>> coord = {'latitude': '37.24N', 'longitude': '-115.81W'} >>> 'Coordinates: {latitude}, {longitude}'.format(**coord) 'Coordinates: 37.24N, -115.81W'

>>> c = 3-5j >>> ('The complex number {0} is formed from the real part {0.real} ' ... 'and the imaginary part {0.imag}.').format(c) 'The complex number (3-5j) is formed from the real part 3.0 and the imaginary part -5.0.' >>> class Point: ... def __init__(self, x, y): ... self.x, self.y = x, y ... def __str__(self): ... return 'Point({self.x}, {self.y})'.format(self=self) ... >>> str(Point(4, 2)) 'Point(4, 2)'

>>> >>> coord = (3, 5) >>> 'X: {0[0]}; Y: {0[1]}'.format(coord) 'X: 3; Y: 5' >>> a = {'a': 'test_a', 'b': 'test_b'} >>> 'X: {0[a]}; Y: {0[b]}'.format(a) 'X: test_a; Y: test_b'
格式转换
1 >>> print('{0:b}'.format(3)) 2 11 3 >>> print('{:c}'.format(20)) 4 5 >>> print('{:d}'.format(20)) 6 20 7 >>> print('{:o}'.format(20)) 8 24 9 >>> print('{:x}'.format(20)) 10 14 11 >>> print('{:e}'.format(20)) 12 2.000000e+01 13 >>> print('{:g}'.format(20.1)) 14 20.1 15 >>> print('{:f}'.format(20)) 16 20.000000 17 >>> print('{:n}'.format(20)) 18 20 19 >>> print('{:%}'.format(20)) 20 2000.000000% 21 >>>
进阶用法
进制转换

>>> # format also supports binary numbers >>> "int: {0:d}; hex: {0:x}; oct: {0:o}; bin: {0:b}".format(42) 'int: 42; hex: 2a; oct: 52; bin: 101010' >>> # with 0x, 0o, or 0b as prefix: >>> "int: {0:d}; hex: {0:#x}; oct: {0:#o}; bin: {0:#b}".format(42) # 在前面加“#”,则带进制前缀 'int: 42; hex: 0x2a; oct: 0o52; bin: 0b101010'
左中右对齐及位数补全
(1)< (默认)左对齐、> 右对齐、^ 中间对齐、= (只用于数字)在小数点后进行补齐
(2)取位数“{:4s}”、"{:.2f}"等

>>> print('{} and {}'.format('hello','world')) # 默认左对齐 hello and world >>> print('{:10s} and {:>10s}'.format('hello','world')) # 取10位左对齐,取10位右对齐 hello and world >>> print('{:^10s} and {:^10s}'.format('hello','world')) # 取10位中间对齐 hello and world >>> print('{} is {:.2f}'.format(1.123,1.123)) # 取2位小数 1.123 is 1.12 >>> print('{0} is {0:>10.2f}'.format(1.123)) # 取2位小数,右对齐,取10位 1.123 is 1.12 >>> '{:<30}'.format('left aligned') # 左对齐 'left aligned ' >>> '{:>30}'.format('right aligned') # 右对齐 ' right aligned' >>> '{:^30}'.format('centered') # 中间对齐 ' centered ' >>> '{:*^30}'.format('centered') # 使用“*”填充 '***********centered***********' >>>'{:0=30}'.format(11) # 还有“=”只能应用于数字,这种方法可用“>”代替 '000000000000000000000000000011'
正负符号显示

>>> '{:+f}; {:+f}'.format(3.14, -3.14) # 总是显示符号 '+3.140000; -3.140000' >>> '{: f}; {: f}'.format(3.14, -3.14) # 若是+数,则在前面留空格 ' 3.140000; -3.140000' >>> '{:-f}; {:-f}'.format(3.14, -3.14) # -数时显示-,与'{:f}; {:f}'一致 '3.140000; -3.140000'
百分数%

>>> points = 19 >>> total = 22 >>> 'Correct answers: {:.2%}'.format(points/total) 'Correct answers: 86.36%'
时间

>>> import datetime >>> d = datetime.datetime(2010, 7, 4, 12, 15, 58) >>> '{:%Y-%m-%d %H:%M:%S}'.format(d) '2010-07-04 12:15:58'
逗号","分隔金钱,没以前进位

>>> '{:,}'.format(1234567890) '1,234,567,890'
占位符嵌套

>>> for align, text in zip('<^>', ['left', 'center', 'right']): ... '{0:{fill}{align}16}'.format(text, fill=align, align=align) ... 'left<<<<<<<<<<<<' '^^^^^center^^^^^' '>>>>>>>>>>>right' >>> >>> octets = [192, 168, 0, 1] >>> '{:02X}{:02X}{:02X}{:02X}'.format(*octets) 'C0A80001' >>> int(_, 16) # 官方文档给出来的,无法在IDLE复现 3232235521 >>> >>> width = 5 >>> for num in range(5,12): ... for base in 'dXob': ... print('{0:{width}{base}}'.format(num, base=base, width=width), end=' ') ... print() ... 5 5 5 101 6 6 6 110 7 7 7 111 8 8 10 1000 9 9 11 1001 10 A 12 1010 11 B 13 1011
占位符%s和%r

""" replacement_field ::= "{" [field_name] ["!" conversion] [":" format_spec] "}" conversion ::= "r" | "s" | "a" 这里只有三个转换符号,用"!"开头。 "!r"对应 repr();"!s"对应 str(); "!a"对应ascii()。 """ >>> "repr() shows quotes: {!r}; str() doesn't: {!s}".format('test1', 'test2') "repr() shows quotes: 'test1'; str() doesn't: test2" # 输出结果是一个带引号,一个不带
format的用法变形
# a.format(b) >>> "{0} {1}".format("hello","world") 'hello world' # f"xxxx"
# 可在字符串前加f以达到格式化的目的,在{}里加入对象,此为format的另一种形式:
>>> a = "hello" >>> b = "world" >>> f"{a} {b}" 'hello world' name = 'jack' age = 18 sex = 'man' job = "IT" salary = 9999.99 print(f'my name is {name.capitalize()}.') print(f'I am {age:*^10} years old.') print(f'I am a {sex}') print(f'My salary is {salary:10.3f}') # 结果 my name is Jack. I am ****18**** years old. I am a man My salary is 9999.990
如果这篇文章帮助到了你,你可以请作者喝一杯咖啡
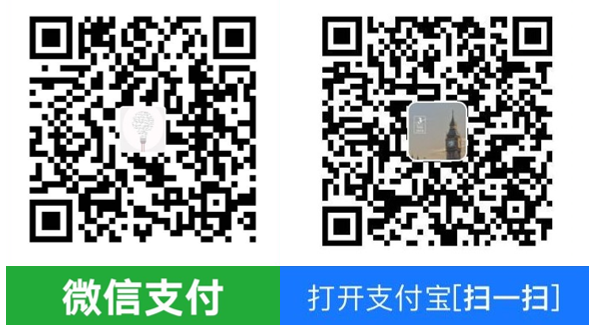