java基础练习
练习1:判断输入的值是否是偶数,另外,要处理输入错误
(目的:熟悉输入、输出,特别是Scanner对象的方法)
import java.util.InputMismatchException; import java.util.Scanner; public class test01 { public static void main(String[] args) { /* * 1、加一层循环判断,就不用执行多次 * 2、使用scanner对象的方法进行输入输出使用 * 3、使用try-catch方法用于异常判断,中间用if\else-if判断是否偶数 * */ while (true){ Scanner sc = new Scanner(System.in); System.out.print("请输入数字:"); try { int n=sc.nextInt(); if (n==0){ System.out.println(n+"不是偶数"); } else if (n%2==0) { System.out.println(n+"是偶数"); } else { System.out.println(n+"不是偶数"); } }catch (InputMismatchException e){ System.out.println("你输入的不是数字"); } } } }
练习2:输入并输出姓名、年龄、身高
import java.util.Scanner; public class Test02 { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print("请输入用户名:"); String name=sc.next(); System.out.print("请输入身高:"); double height = sc.nextDouble(); System.out.print("请输入年龄:"); int age = sc.nextInt(); System.out.println("用户名:"+name+",身高:"+height+",年龄:"+age); } }
练习3:从控制台获取Java、Oracle、HTML三门课程的成绩,计算总分和平均分(平均分保留2位小数,要求四舍五入;按如下格式输出)
import java.util.Scanner; public class Test03 { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print("Java成绩:"); int java= sc.nextInt(); System.out.print("Oracle成绩:"); int oracle= sc.nextInt(); System.out.print("Html成绩:"); int html= sc.nextInt(); System.out.println("---------------------"); System.out.println("Java Oracle Html"); System.out.println(""+java+'\t'+oracle+'\t'+html); System.out.println("---------------------"); System.out.println(); int c=java+oracle+html; System.out.println("总分:"+c); System.out.println("平均分:"+(c/3)); } }
练习4:输入一个数字,反转输出,如:输入123,输出321
import java.util.Scanner; public class Test05 { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print("请输入一个数:"); String num= sc.next(); for (int i = num.length()-1; i >=0; i--) { System.out.print(num.charAt(i)); } } }
练习5:输入tom和jack的年龄,比较年龄并输出相差多少岁,要求使用条件运算符
import java.util.Scanner; public class Test04 { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print("请输入tom的年龄:"); int tom=sc.nextInt(); System.out.print("请输入jack的年龄:"); int jack=sc.nextInt(); if (tom>jack){ System.out.println("tom比jack大"+(tom-jack)+"岁"); if (tom>18){ System.out.println("tom是成年人"); } if (tom<70){ System.out.println("tom未到退休年龄"); }else { System.out.println("tom是未成年人"); } }else if (tom==jack){ System.out.println("tom和jack同岁"); }else { System.out.println("tom比jack小" + (jack - tom) + "岁"); } } }
练习6:交换两个数值变量的值(至少两种方法)
public class Test06 { public static void main(String[] args) { //定义临时变量 int v1=1,v2=2; int temp; temp=v1; v1=v2; v2=temp; System.out.println("v1="+v1+",v2="+v2); //运算符 int v3=1,v4=2; v3=v3+v4; v4=v3-v4; v3=v3-v4; System.out.println("v3="+v3+",v4="+v4); //位运算法 int v5=1,v6=2; v5=v5^v6; v6=v5^v6; v5=v5^v6; System.out.println("v5="+v5+",v6="+v6); } }
练习7:下面结果分别是?说明原因
int a = 97; char b = a; System.out.println(b); java: 不兼容的类型: 从int转换到char可能会有损失 char c = 97; System.out.println(c); 结果:a,a的ASCII码是97 int m = 3; int n = m++ + ++m; System.out.println("m:"+m+", n:"+n); m:5, n:8 n=3+5 后面的m经过++m后:m=3+1+1+3,++的意思自增1
练习8:下面结果分别是?说明原因
public class Test { public static void main(String[] args) { short a=1; a = a+1; System.out.println(a); 结果:java: 不兼容的类型: 从int转换到short可能会有损失 short b=1; b+=1; System.out.println(b); 结果:b=2,b+=1===》b=b+1 } }
java基础练习:选择结构(if、switch)
if:if...else...及if嵌套
练习1:提示用户输入三个整数,判断并输出最大值、最小值
import java.util.Scanner; public class lx { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print("请输入第1个数:"); int a= sc.nextInt(); System.out.print("请输入第2个数:"); int b= sc.nextInt(); System.out.print("请输入第3个数:"); int c= sc.nextInt(); //a>b>c a>b<c if (a>=b){ if (a>=c){ if (b<=c) { System.out.println("最大值:"+a); System.out.println("最小值:"+b); }else { System.out.println("最大值:"+a); System.out.println("最小值:"+c); } } else { System.out.println("最大值:"+c); System.out.println("最小值:"+b); } } else { if (b>=c){ if (a<=c){ System.out.println("最大值:"+b); System.out.println("最小值:"+a); }else { System.out.println("最大值:"+b); System.out.println("最小值:"+c); } }else { System.out.println("最大值:"+c); System.out.println("最小值:"+a); } } } }
如果今天是周日且晴天,就出去嗨
如果今天是周日但不是晴天,睡懒觉
如果今天不是周日,继续996
说明:天气、星期几的变量,自己定义即可;主要练习if嵌套
import java.util.Scanner; public class Test04 { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print("今天星期几:"); int today= sc.nextInt(); switch (today){ case 1: System.out.println("跑步"); break; case 2: System.out.println("打羽毛球"); break; case 3: System.out.println("打羽毛球"); break; case 4: System.out.println("打羽毛球"); break; case 5: System.out.println("游泳"); break; default: System.out.println("休息"); } } }
练习3:由键盘输入三个整数分别存入变量num1、num2、num3,对它们进行排序(使用 if-else if-else),并且从小到大输出。
import java.util.Scanner; public class lx { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print("num1:"); int num1= sc.nextInt(); System.out.print("num2:"); int num2= sc.nextInt(); System.out.print("num3:"); int num3= sc.nextInt(); if (num1>=num2){ if (num1>=num3){ if (num2<=num3) { System.out.println(num1+","+num3+","+num2); }else { System.out.println(num1+","+num2+","+num3); } } else { System.out.println(num3+","+num1+","+num2); } } else { if (num2>=num3){ if (num1<=num3){ System.out.println(num2+","+num3+","+num1); }else { System.out.println(num2+","+num1+","+num3); } }else { System.out.println(num3+","+num2+","+num1); } } } }
练习4:提示用户输入三角形的边a、b、c,范围必须在 [1,100) 之间,判断三角形的类型:1.非三角形;2.等边三角形;3.直角三角形;4.等腰三角形;5.普通三角形
import java.util.Scanner; public class Lx2 { public static void main(String[] args) { //练习4:提示用户输入三角形的边a、b、c,范围必须在 [1,100) 之间, // 判断三角形的类型:1.非三角形;2.等边三角形;3.直角三角形;4.等腰三角形;5.普通三角形 Scanner sc = new Scanner(System.in); int[] arr=new int[3]; for (int i=0;i<arr.length;i++){ arr[i]=sc.nextInt(); if (!(1<= arr[i] && arr[i] <100)) { System.out.println("输入数字有误"); return; } } for (int i=0;i<arr.length;i++){ System.out.print(arr[i]+" "); } //边长排序,从小到大 int k,j; int l = arr.length; for(k=0;k<l-1;k++) for (j=k+1;j<l;j++) if (arr[k]>arr[j]){ int t = arr[k]; arr[k]=arr[j]; arr[j]=t; } //逻辑判断,是否三角形 if (arr[0]+arr[1]<=arr[2]){ System.out.println("不是三角形"); //等腰三角形? } else { if (arr[0] == arr[1] || arr[1]==arr[2]) { if (arr[0]==arr[1] && arr[1]==arr[2]) { System.out.println("等边三角形"); } else { //是等边三角形? if (arr[0] * arr[0] + arr[1] * arr[1] - arr[2] * arr[2]<=0.001) { System.out.println("等腰直角三角形"); } else { System.out.println("等腰三角形"); } } //不是等腰三角形 } else { //直角三角形?浮点数不精确,建议改成作差小于0.001 if (arr[0] * arr[0] + arr[1] * arr[1] == arr[2] * arr[2]) { System.out.println("直角三角形"); } else { System.out.println("一般三角形"); } } } } }
switch
练习1:周一跑步,周二、三、四打羽毛球,周五游泳,其它休息
int today=4;
import java.util.Scanner; public class Test04 { public static void main(String[] args) { //周一跑步,周二、三、四打羽毛球,周五游泳,其他休息 //int today=2; Scanner sc = new Scanner(System.in); System.out.print("今天星期几:"); int today= sc.nextInt(); switch (today){ case 1: System.out.println("跑步"); break; case 2: System.out.println("打羽毛球"); break; case 3: System.out.println("打羽毛球"); break; case 4: System.out.println("打羽毛球"); break; case 5: System.out.println("游泳"); break; default: System.out.println("休息"); } } }
练习2:输入 2022 年的某个月份和日期,例如 month=4,day=21,经过程序计算,打印出输入的月份和日期是 2022年的第几天:31+28+31+21
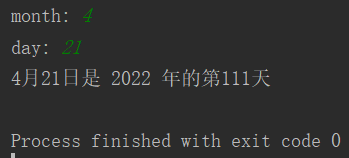
public class Test04 { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print("month:"); int month=sc.nextInt(); System.out.print("day:"); int day=sc.nextInt(); int sum=0; switch (month){ case 12: sum += 30; case 11: sum += 31; case 10: sum += 30; case 9: sum += 31; case 8: sum += 31; case 7: sum += 30; case 6: sum += 31; case 5: sum += 30; case 4: sum += 31; case 3: sum += 28; case 2: sum += 31; case 1: sum += day; break; default: System.out.println("月份输入错误"); } System.out.println("输入的日期是2022年的第"+sum+"天"); } }
java基础练习:循环结构(while、do...while、for、break、continue、return)
while
练习1:输出比i(i=5)小的正整数
public class Lx { public static void main(String[] args) { int i=1; while (i<5){ System.out.println(i); i++; } } }
int i=5;
while (--i>0){
System.out.println(i);
}
练习2:求1到100之间所有偶数的和
public class Lx { public static void main(String[] args) { int sum=0; for(int i=1;i<=100;i++){ if (i%2==0){ sum += i; } } System.out.println(sum); } }
int sum=0,i=0;
while (i<=100){
sum += i;
i += 2;
}
System.out.println(sum);
public class Lx { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print("输入一个非零整数(0表示结束):"); int n=sc.nextInt(); int max=n; int min=n; while (n != 0) { System.out.print("输入一个非零整数(0表示结束):"); n=sc.nextInt(); if (n !=0){ if (n>max) { max=n; } if (n<min) { min=n; } } } System.out.println("最大值"+max); System.out.println("最小值"+min); } }
练习4:从键盘读入个数不确定的整数,并判断读入的正数和负数的个数,输入为0时结束程序
import java.util.Scanner; public class lx { public static void main(String[] args) { //练习4:从键盘读入个数不确定的整数,并判断读入的正数和负数的个数,输入为0时结束程序 Scanner sc = new Scanner(System.in); //定义正数个数,初始是0 int positive = 0; //定义负数个数,初始是0 int negative = 0; //定义一个需要输入的数据 int n; do { System.out.print("请输入:"); n=sc.nextInt(); if (n<0){ negative++; } else if (n>0) { positive++; }else { System.out.println("输入的数据不合法"); } }while (n != 0 ); //n=0不符合条件,程序结束,跳出do-while循环 System.out.println("正数的个数是:"+positive); System.out.println("负数的个数是:"+negative); } }

public class lx { public static void main(String[] args) { //练习4:从键盘读入个数不确定的整数,并判断读入的正数和负数的个数,输入为0时结束程序 Scanner sc = new Scanner(System.in); //定义正数个数,初始是0 int positive = 0; //定义负数个数,初始是0 int negative = 0; //定义一个需要输入的数据 int n=sc.nextInt(); while (n != 0){ System.out.print("请输入:"); n=sc.nextInt(); if (n<0){ negative++; } else if (n>0) { positive++; }else { System.out.println("输入的数据不合法"); } } System.out.println("正数的个数是:"+positive); System.out.println("负数的个数是:"+negative); } }
练习5:如果输入的是整数,输出是偶数还是奇数,然后结束;如果输入的不是整数,提示用户重新输入,直到输入整数
import java.util.Scanner; public class lx { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print("请输入一个数:"); String str = sc.next(); char[] chars = str.toCharArray(); for(int i=0;i<chars.length;i++){ if(!Character.isDigit(str.charAt(i))){ System.out.println("输入有误"); break; } else { System.out.println(str+"是整数"); //将string转为int Integer n = Integer.valueOf(str); if (n%2 == 0){ System.out.println(str+"是偶数"); }else { System.out.println(str+"是奇数"); } } } } }
do while
练习1:下面输出结果是?说明原因
1
2
3
4
5
6
7
8
9
10
11
|
package com.qzcsbj.demo.entity; public class T2 { public static void main(String[] args) { int i = 5 ; do { System.out.println(i); i--; } while (i> 5 ); } } |
public class lx { public static void main(String[] args) { int n = 5; int count=0; for (int i=0;i<=200;i++){ if (i%7==0 & i%4!=0){ System.out.print(" "+i); n++; count++; if (n%5==0){ System.out.println(); } } } System.out.println(); System.out.println("总个数:"+count); } }
for
练习1:求1到100之间所有偶数的和
public class lx { public static void main(String[] args) { int sum = 0; for (int i = 2; i <= 100; i+=2) { sum += i; } System.out.println(sum); } }
import java.util.Scanner; public class lx { public static void main(String[] args) { Scanner sc = new Scanner(System.in); int score=0; for (int i = 1; i <= 5; i++) { System.out.print("请输入第"+i+"门课程的成绩:"); int n=sc.nextInt(); score = score+n; } System.out.println("平均分:"+(score/5)); } }
练习3:任意输入一个整数,根据这个值输出加法表
import java.util.Scanner; public class lx { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print("请输入一个数:"); int n=sc.nextInt(); for (int i = 1; i <= n; i++) { System.out.println(i+"+"+(n-i)+"="+n); } } }
练习4:打印 1-100000之间的所有素数,并统计耗时,如果耗时大于200ms,就需要优化
(素数: 有且仅有两个正约数的整数. 即 2 ~ i-1 之间没有一个是其约数)
public class lx { public static void main(String[] args) { //100000以内的所有质数的输出以及代码优化 boolean isFlag = true; int count=0; long start = System.currentTimeMillis(); for (int i = 2; i <= 100000 ; i++) { for (int j = 2; j <=Math.sqrt(i) ; j++) { if (i%j == 0) { isFlag = false; break; } } if (isFlag == true) { count++; } isFlag = true; } //获取当前时间距离的毫秒级 long end = System.currentTimeMillis(); System.out.println("质数的个数为:"+count); System.out.println("所花费的时间为:"+(end-start)); } }
break
public class lx { public static void main(String[] args) { int sum=0; for (int i = 1; i <=10 ; i++) { sum+=i; if (sum > 20) { System.out.println("当前整数值:"+i); System.out.println("累加值:"+sum); break; } } } }
continue
练习1:输出1到10之间所有的奇数
public class lx { public static void main(String[] args) { int i=0; while (i<10){ i++; if (i%2==0) { continue; //偶数则跳过这轮循环 } System.out.println(i); } } }
return
练习1:下面输出结果是?说明原因
0 1 1 0 1
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
|
package com.qzcsbj; import java.util.Scanner; /** * @公众号 : 全栈测试笔记 * @博客 : www.cnblogs.com/uncleyong * @微信 : ren168632201 * @描述 : <> */ public class Test { public static void main(String[] args) { System.out.println(test()); test2(); } public static int test(){ for ( int i = 0 ; i < 5 ; i++) { System.out.println(i); if (i== 1 ){ return i; } } return 10 ; } public static void test2(){ for ( int i = 0 ; i < 5 ; i++) { System.out.println(i); if (i== 1 ){ return ; } } System.out.println( "hello" ); return ; } } |
双重for循环
public class lx { public static void main(String[] args) { for (int i = 1; i < 9; i++) { for (int j = 1; j <= i; j++) { System.out.print(j+"*"+i+"="+i*j+'\t'); } System.out.println(); } } }
练习2:某次技能大赛,共有3个班参加,每个班有4名学生参加,计算每个班级的平均分(只统计成绩≥80分的学生的平均分)
import java.util.Scanner; public class lx { public static void main(String[] args) { Scanner sc = new Scanner(System.in); //外层控制班级 for (int i = 1; i <= 3; i++) { int sum=0; int avg=0;
//内层控制学生 for (int j = 1; j <= 4 ; j++) { System.out.print("请输入第"+i+"个班级第"+j+"个学生的成绩:"); System.out.println(); int score = sc.nextInt(); sum += score; } //计算每个班级的平均分 avg = sum/4; System.out.println("第"+i+"个班级的平均分为:"+avg); } } }
import java.util.Scanner; public class lx { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print("输入行数:"); int num = sc.nextInt(); //直角三角形 for (int i = 1; i <=num ; i++) { for (int j = 1; j <=i ; j++) { System.out.print("*"); } System.out.println(" "); } System.out.println(); for (int i2 = 1; i2 <=num ; i2++) { for (int j2 = 1; j2 <= 2*i2-1 ; j2++) { System.out.print("*"); } System.out.println(" "); } //倒三角形 System.out.println(); for (int k = num; k >=0 ; k--) { for (int l = 1; l <=k ; l++) { System.out.print("*"); } System.out.println(" "); } for (int k2 = num; k2 > 0; k2--) { for (int l2 = 1; l2 <= 2 * k2 - 1; l2++) { System.out.print("*"); } System.out.println(" "); } } }
import java.util.Scanner; public class lx { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print("输入行数:"); int num = sc.nextInt(); //等腰三角形 for (int i = 1; i <=num ; i++) { for (int j = 1; j <=num ; j++) { System.out.print(" "); } for (int k = 1; k <=i ; k++) { System.out.println("*"); } System.out.println(); } } }
练习5:打印等边三角形
import java.util.Scanner; public class lx { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print("输入行数:"); int num = sc.nextInt(); for (int i = 1; i <=num ; i++) { for (int j = num; j >=i ; j--) { System.out.print(" "); } for (int k = 1; k <=2*i-1 ; k++) { System.out.println("*"); } System.out.println(); } } }
import java.util.Arrays; import java.util.Scanner; public class lx { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print("输入带*字符串:"); String str = sc.nextLine(); System.out.println("移动前:"+str); //String str = "as*c*gkl**o*p*h"; char[] arr = str.toCharArray(); for (int i = 0; i <= arr.length-1; i++) { for (int j = 1; j <= arr.length-1-i ; j++) { //把*左移 if (arr[j] == '*' && arr[j-1]!='*') { char temp = arr[j]; arr[j] = arr[j-1]; arr[j-1] = temp; } } System.out.println("第"+(i+1)+"轮冒泡:"+ Arrays.toString(arr)); String s = ""; for (int k = 0; k <= arr.length-1; k++) { s += arr[k]; } System.out.println("移动后:"+s); } } }
方法
练习1:定义方法(不用jdk提供的方法),计算x的y次方,如2的5次方
import java.util.Scanner; public class lx { public static void main(String[] args) { int num = (int) (Math.random() * 100); System.out.println(num); Scanner sc = new Scanner(System.in); int count = 0; while (true){ count++; System.out.print("请输入你要猜的数字:"); int num1 = sc.nextInt(); if (num1 < num) { System.out.println("猜小了"); } if (num1 > num) { System.out.println("猜大了"); } if (num1 == num) { System.out.println("恭喜你,猜对了,共猜了:"+count+"次"); } } } }
递归
练习1:用递归实现,计算x的y次方,如2的5次方
class Digui{ static int fac(int n, int m){ if (m == 0 ) { return 1; } else if (m == 1) { return n; } else { return n * fac(n,m-1); } } } public class lx { public static void main(String[] args) { Digui dg = new Digui(); System.out.println(dg.fac(2, 3)); } }
练习2:用递归实现,输出n(n=5)到0的整数
public class lx { public static int fac(int n){ if (n == 0) { System.out.println(0); return 0; } fac(n-1); System.out.println(n); return n; } public static void main(String[] args) { fac(5); } }
java基础练习:数组(一维、二维、传值传地址)
一维数组
练习1:定义一个int类型数组,动态赋值,然后将数组中元素反转,最后输出,要求:动态赋值定义方法;反转定义方法
import java.util.Arrays; import java.util.Scanner; public class lx { public static int[] reverse(int[] arr){ int[] res = new int[arr.length]; //反转操作 for (int i = 0,j=res.length-1; i < arr.length; i++,j--) { res[j] = arr[i]; } return res; } public static void main(String[] args) { Scanner sc = new Scanner(System.in); int[] arr = new int[3]; for (int i = 0; i <= arr.length-1; i++) { System.out.print("请输入数组的第"+(i+1)+"个数字:"); arr[i]=sc.nextInt(); } System.out.println("动态赋值后:"+Arrays.toString(arr)); //反转打印 int[] reverse = reverse(arr); System.out.println("数组反转后:"+Arrays.toString(reverse)); } }
练习2:有一个数组 [1, 3, 66, 16, 28, 666, 168] ,循环输出数组中的元素并计算所有数的总和,并将最大值放到最前面,最小值放到最后面,然后输出数组
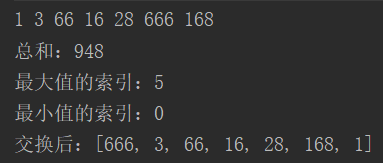
import java.util.Arrays; public class lx { //反转数组 public static int[] reverse(int[] arr){ int[] res = new int[arr.length]; //反转操作 for (int i = 0,j=res.length-1; i < arr.length; i++,j--) { res[j] = arr[i]; } return res; } //数组的元素交换 public static void swap(int[] arr,int i,int j){ int t = arr[i]; arr[i] = arr[j]; arr[j]=t; } //获取最大值索引 public static int getmaxIndex(int[] arr){ //假设第一个元素为最大值(最小值),那么下标设为0 int maxIndex = 0; for (int i = 0; i < arr.length-1; i++) { if (arr[maxIndex] < arr[i + 1]) { maxIndex = i + 1; } } return maxIndex; } //获取最小值索引 public static int getminIndex(int[] arr){ //假设第一个元素为最大值(最小值),那么下标设为0 int minIndex = 0; for (int i = 0; i < arr.length-1; i++) { if (arr[minIndex] > arr[i + 1]) { minIndex = i + 1; } } return minIndex; } //获取数组最大值 public static void getMax(int[] arr){ int max = arr[0]; for (int i = 0; i < arr.length; i++) { if (arr[i] > max) { max = arr[i]; } } System.out.println(max); } public static void main(String[] args) { int[] arr = {1, 3, 66, 16, 28, 666, 168}; int s=0; //循环输出数组元素 for (int i = 0; i < arr.length ; i++) { System.out.print(arr[i]+" "); s = s+arr[i]; } System.out.println(" "); System.out.println("总和:"+s); //索引 int maxindex = getmaxIndex(arr); //交换索引的值 swap(arr,0,maxindex); //最大值索引值交换到第一位 int minindex = getminIndex(arr); swap(arr,arr.length-1,minindex); //最小值索引值交换到最后一位 System.out.println("最大值索引:"+maxindex); System.out.println("最小值索引:"+minindex); System.out.println("交换后:"+Arrays.toString(arr)); } }
增强for
练习1:输出数组的值[1, 3, 66, 16, 28, 666, 168]
public class lx { public static void main(String[] args) { int[] arr = {1, 3, 66, 16, 28, 666, 168}; for (int x: arr) { System.out.println(x); } } }
传值、传地址
练习1:下面输出结果是?说明原因
5
8
[666, 2, 3]
[666, 2, 3]
666
[666, 2, 999]
5
[666, 2, 999]
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
|
package com.qzcsbj; import java.util.Arrays; import java.util.Scanner; /** * @公众号 : 全栈测试笔记 * @博客 : www.cnblogs.com/uncleyong * @微信 : ren168632201 * @描述 : <> */ public class Test { public static void main(String[] args) { /* * 基本数据类型 */ int a = 5 ; int b = a; b = 8 ; System.out.println(a); System.out.println(b); /* * 引用数据类型 */ int [] c = { 1 , 2 , 3 }; int [] d = c; d[ 0 ] = 666 ; System.out.println(Arrays.toString(c)); System.out.println(Arrays.toString(d)); change(a,c); System.out.println(a); System.out.println(Arrays.toString(c)); } public static void change( int i, int [] array) { i = 666 ; array[array.length - 1 ] = 999 ; System.out.println(i); System.out.println(Arrays.toString(array)); } } |
二维数组(含冒泡)
练习1:输出二维数组的每个元素,int[][] d = { { 1, 2, 9 }, { 5 }, { 1, 3 }, { 9, 7, 0 } };
public class lx { public static void main(String[] args) { int[][] d = { { 1, 2, 9 }, { 5 }, { 1, 3 }, { 9, 7, 0 } }; //输出二维数组各元素的值 for (int i = 0; i < d.length; i++) { for (int j = 0; j < d[i].length; j++) { System.out.print(d[i][j]+" "); } System.out.println(); } } }
练习2:有2个班,每个班有3名学生,提示用户分别输入学生的成绩,将数据保存到二维数组中,并计算每个班的平均分、全校的最高分、最低分,最后输出平均分最高的班以及对应的平均分
练习3:冒泡实现排序,[9,6,4,5,3],输出:[3, 4, 5, 6, 9],要求打印每一轮每一次冒泡后的结果
public class lx2 { public static void main(String[] args) { int[] arr = {9,6,4,5,3}; int temp = 0; for (int i = 0; i < arr.length-1; i++) { for (int j = 0; j < arr.length-1-i; j++) { if (arr[j] > arr[j+1]) { temp = arr[j]; arr[j] = arr[j+1]; arr[j+1] = temp; } System.out.println("第"+(i+1)+"轮,第"+(j+1)+"次:"+ Arrays.toString(arr)); } System.out.println("===第"+(i+1)+"轮结果:"+Arrays.toString(arr)); } } }
不定长参数
练习1:下面的输出结果是?说明原因,另外,不定长参数的本质是什么?
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
|
package com.qzcsbj; import java.util.Arrays; import java.util.Scanner; /** * @公众号 : 全栈测试笔记 * @博客 : www.cnblogs.com/uncleyong * @微信 : ren168632201 * @描述 : <> */ public class Test { public static void main(String[] args) { // String[] names=new String[]{"tom","jack"}; m1( 6 , new String[] { "tom" , "jack" }); m2( 6 , "tom" , "jack" , "alice" ); m2( 6 , "tom" ); m2( 6 ); m2( 6 , new String[] { "tom" , "jack" }); } public static void m1( int n, String[] args) { System.out.println( "m1" ); } public static void m2( int n,String... args){ System.out.println( "不定长参数个数:" +args.length); if (args.length > 0 ){ System.out.println( "不定长参数第一个:" +args[ 0 ]); } } } |
m1 不定长参数个数:3 不定长参数第一个:tom 不定长参数个数:1 不定长参数第一个:tom 不定长参数个数:0 不定长参数个数:2 不定长参数第一个:tom
笔试题
练习1:数组中有一个数字出现的次数超过数组长度的一半
public class test1 { public int Vote(int [] array){ int cond=-1; int cnt=0; for (int i = 0; i < array.length; i++) { if (cnt == 0) { cond = array[i]; cnt++; }else { if (cond == array[i]) { cnt++; }else { cnt--; } } } cnt = 0; for (int i:array){ if (i == cond) { cnt++; } } if (cnt > array.length/2) { System.out.println(""); return cond; }else { return 0; } } public static void main(String[] args) { test1 vote1 = new test1(); int[] arr = {1,2,3,2,2,6,2}; System.out.println(vote1.Vote(arr)); } }
java基础练习: 类(对象属性、对象数组)、构造方法及this、方法重载、对象初始化
类
练习1:创建一个用户类,为用户设置年龄(0-120),并打印年龄,如果设置的不合法,给出提示
Person.java类 package qzcsbj; public class Person { public String name; public int age; public String getName() { return name; } public void setName(String name){ this.name=name; } public int getAge(){ return age; } public void setAge(int age){ if (age < 0 || age > 130) { System.out.println("年龄范围只能是0-130,您输入的是:"+age); return; }else { System.out.println("年龄范围正确,您输入的是:"+age); } this.age=age; } @Override public String toString() { return "Person{" + "name='" + name + '\'' + ",age=" + age + '}'; } } TestPerson.java package qzcsbj; public class TestPerson { public static void main(String[] args) { Person p = new Person(); p.setAge(139); System.out.println("age=" + p.getAge()); Person p2 = new Person(); p2.setAge(130); System.out.println("age=" + p2.getAge()); } }
练习2:定义Boy、Girl类,对象boy给对象girl求婚,且girl答复;要求,boy对象的求婚方法传入girl对象,girl对象的答复方法传入求婚的对象
package qzcsbj; class Girl{ private String name; public Girl(String name){ this.name=name; } public String getName(){ return name; } public void answer(Boy boy){ System.out.println(boy.getName() +",你有房么,有车么?"); } } class Boy { private String name; public Boy(String name){ this.name=name; } public String getName(){ return name; } public void marry(Girl girl){ System.out.println(girl.getName() +",你愿意嫁给我吗?"); girl.answer(this); } } public class Test { public static void main(String[] args) { Boy boy = new Boy("tom"); Girl girl = new Girl("lucky"); boy.marry(girl); } }
练习3:分别用面向过程和面向对象的思维,计算半径为3的圆的面积
import java.util.Scanner; //面向对象的方法,计算圆面积 public class Circle { public static void main(String[] args) { CircleTest c1 = new CircleTest(); Scanner sc = new Scanner(System.in); System.out.println("请输入圆半径:"); c1.radius=sc.nextDouble(); c1.area(); System.out.println(c1.area()); } } class CircleTest{ double radius; public double area(){ double area = Math.PI*radius*radius; return area; } } //面向过程,计算圆面积 public class Yuan { public static void main(String[] args) { double r=3.0; //double circle = 2*r*Math.PI; //圆周长 double area = Math.PI*r*r; System.out.println("圆的面积"+area); } }
全局变量、局部变量、this
练习1:写代码演示为什么要用this,解决了什么问题?
this调用当前属性:其主要作用是当需要给类中的数据进行初始化时,可以通过this来进行赋值,而不用随便定义一个变量来进行赋值,更有利于代码的阅读与理解
练习2:下面结果分别是?并说明原因
1
2
3
4
5
6
7
8
|
public class Test { static String str; public static void main(String[] args) { static String str; System.out.println(str); } } |
main里面的str不用再次定义static
1
2
3
4
5
6
7
8
|
public class Test { static String str; public static void main(String[] args) { String str; System.out.println(str); } } |
str没有初始化,没有赋值
1
2
3
4
5
6
7
|
public class Test { static String str; public static void main(String[] args) { System.out.println(str); } } |
执行代码后为:null
对象属性
练习1:创建Person类(name,age,address),address是对象属性,Address类(province、city),创建一个Person类对象person,并给所以属性赋值,最后打印person
package qzcsbj; public class Test3 { public static void main(String[] args) { PersonTest person=new PersonTest("tom",23); Address add=new Address(); add.setProvince("广东省"); add.setCity("深圳市"); person.setAddress(add); System.out.println("name:"+person.getName()); System.out.println("age:"+person.getAge()); System.out.println("省份:"+person.getAddress().getProvince()); System.out.println("城市:"+person.getAddress().getCity()); } } class PersonTest{ private String name; private int age; private Address address; public PersonTest(){ super(); } public PersonTest(String name,int age){ super(); this.name=name; this.age=age; } public String getName(){ return name; } public void setName(String name){ this.name=name; } public int getAge(){ return age; } public void setAge(int age){ this.age=age; } public Address getAddress(){ return address; } public void setAddress(Address address){ this.address=address; } } class Address{ private String province; private String city; public Address(){ } public Address(String province,String city){ this.province=province; this.city=city; } public String getProvince(){ return province; } public void setProvince(String province){ this.province=province; } public String getCity(){ return city; } public void setCity(String city){ this.city=city; } }
对象数组
练习1:定义学生类(姓名、学号),录入学生信息,并将学生保存到数组中
import java.util.Scanner; public class Student { public static void main(String[] args) { Scanner sca = new Scanner(System.in); Stu [] stude =new Stu [10]; //创建学生数组 System.out.print("输入学生数目:"); int n= sca.nextInt(); for (int i = 0; i < n; i++) { stude[i]=new Stu(); System.out.println("======请输入第"+(i+1)+"个学生的信息====="); stude[i].input(); } System.out.println("--------学生信息--------"); for (int i = 0; i < n; i++) { stude[i].output(); } } } class Stu { String name; int id; public void input() { Scanner sc = new Scanner(System.in); System.out.print("请输入姓名:"); name = sc.next(); System.out.print("请输入学号:"); id = sc.nextInt(); } public void output(){ System.out.println("学生姓名:"+name+",学生编号:"+id); } }
构造方法、this、方法重载
练习1:定义一个类(三个属性),三参构造方法中,调用两参构造方法
package qzcsbj; public class Test4 { private String name; private int age; private String phone; public Test4(){ System.out.println("无参"); } public Test4(String name){ System.out.println("一个参数"); this.name=name; } public Test4(String name,int age){ this(); System.out.println("两个参数"); this.name=name; this.age=age; } public Test4(String name,int age,String phone){ this(name, age); System.out.println("三个参数"); this.phone=phone; } public void study(String type){ System.out.println(this.name + "的学习方式是:"+type); } public void study(String type,String subject){ System.out.println(this.name+"的学习方式是:"+type+",学习科目是:"+subject); } public String getName(){ return name; } public void setName(String name){ this.name=name; } public int getAge(){ return age; } public void setAge(int age){ this.age=age; } public String getPhone(){ return phone; } public void setPhone(String phone){ this.phone=phone; } @Override public String toString() { return "Desine{" + "name='" + name +'\''+ ", age=" + age + ",phone='" +phone + '\'' + '}'; } }
对象初始化顺序
练习1:写代码验证对象初始化顺序:代码块(静态、非静态)、构造
package qzcsbj; public class User { public User(){ System.out.println(name); System.out.println("构造方法"); this.name="性能测试"; } public void a(){ System.out.println("实力方法"); } public static void b(){ System.out.println("静态方法"); } { System.out.println("代码块"); name = "qzcsbj"; } String name = "测试"; static String job="tester"; static { System.out.println(job); System.out.println("静态代码块"); } public static void main(String[] args) { User user = new User(); System.out.println(user.name); } }
综合练习
练习1:定义一个Person类,要求:属性(name,age,phone),方法(study)并实现方法重载,实现封装,实例化多个对象,输出某个对象的信息,最后输出创建了多少对象
package qzcsbj; public class PersonStudy { private String name; private int age; private String phone; private static int obj_nums; private PersonStudy(){ System.out.println("无参"); obj_nums++; } public PersonStudy(String name,int age,String phone){ System.out.println("有参"); this.name = name; this.age=age; this.phone=phone; obj_nums++; } public void study(String type){ System.out.println(this.name+"的学习方式是:"+type); } public void study(String type,String subject){ System.out.println(this.name+"的学习方式是:"+type+",学习的科目是:"+subject); } public String getName(){ return name; } public void setName(String name){ this.name=name; } public int getAge(){ return age; } public void setAge(int age){ this.age=age; } public String getPhone(){ return phone; } public void setPhone(String phone){ this.phone=phone; } public static int getObj_nums(){ return obj_nums; } public static void setObj_nums(int obj_nums){ PersonStudy.obj_nums=obj_nums; } @Override public String toString() { return "PersonStudy{" + "name='" + name + '\'' + ", age=" + age + ", phone='" + phone + '\'' + '}'; } public static void main(String[] args) { PersonStudy p1=new PersonStudy("wawa",18,"13000000000"); System.out.println(p1.toString()); System.out.println("当前累计已经创建了"+PersonStudy.getObj_nums()+"个PersonStudy对象"); System.out.println(p1.getName()); System.out.println(p1.getAge()); System.out.println(p1.getPhone()); PersonStudy p2=new PersonStudy("tom",20,"13888888888"); System.out.println("当前累计已经创建了"+PersonStudy.getObj_nums()+"个PersonStudy对象"); PersonStudy p3=new PersonStudy("lucky",10,"13200000000"); System.out.println(); System.out.println("当前累计已经创建了"+PersonStudy.getObj_nums()+"个PersonStudy对象"); p3.study("book"); p2.study("vedio","java"); PersonStudy p4=new PersonStudy(); System.out.println("当前累计已经创建了"+PersonStudy.getObj_nums()+"个PersonStudy对象"); } }
java基础练习:封装、继承、多态
封装
练习1:举例演示封装的好处
package qzcsbj; public class Employee { private String name; private int age; private String phone; private String address; public String getName(){ return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { if (age < 18 || age >40) { System.out.println("年龄必须在18到40之间"); this.age=20; //默认年龄 } else { this.age = age; } } public String getPhone() { return phone; } public void setPhone(String phone) { this.phone = phone; } public String getAddress() { return address; } public void setAddress(String address) { this.address = address; } public static void main(String[] args) { Employee p = new Employee(); p.setName("lili"); p.setAge(30); p.setPhone("13000000000"); p.setAddress("广东省深圳市"); System.out.println("姓名:"+p.getName()+" 年龄:"+p.getAge()+" 电话:"+p.getPhone()+" 家庭住址:"+p.getAddress()); } }
继承、构造调用、super
练习1:在子类中调用父类无参、带参构造方法
方法重写
练习1:创建父类Person,子类Japan、Chinese,重写父类方法,实现子类的差异化
多态
练习1:设计几个类,实现多态,主人给宠物喂食物
笔试题
练习1:定义MyDate类,在MyDate类中覆盖equals方法,使其判断当两个MyDate类型对象的年月日都相同是,结果为ture,否则为false。
MyDate m1 = new MyDate(14, 3, 2022);
MyDate m2 = new MyDate(14, 3, 2022);
java基础练习:抽象类、接口
笔试题
练习1:下面输出结果是?并说明原因,如有问题,修改方案至少2种
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
|
package com.qzcsbj; /** * @公众号 : 全栈测试笔记 * @博客 : www.cnblogs.com/uncleyong * @微信 : ren168632201 * @描述 : <> */ interface A{ int x = 0 ; } class B{ int x = 1 ; } public class Test extends B implements A{ public void p(){ System.out.println(x); } public static void main(String[] args) { new Test().p(); } } |
面向对象综合练习
练习1:写一个宠物超市系统(java面向对象练习题,综合运用:封装、继承、多态),需求详见:https://www.cnblogs.com/uncleyong/p/12580618.html
java基础练习:访问修饰符、非访问修饰符
练习1:访问修饰符public、private、protected,以及不写(默认)时的区别?写代码验证
练习2:如何访问非本类的private修饰的属性、方法?
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
|
public class Person{ private String name; private int age; public Person(String name, int age) { this .name = name; this .age = age; } private String getName() { return name; } private int getAge() { return age; } } |
Person p = new Person("jack", 18);
练习3:下面输出结果是?并说明原因
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
|
package com.qzcsbj; /** * @公众号 : 全栈测试笔记 * @博客 : www.cnblogs.com/uncleyong * @微信 : ren168632201 * @描述 : <> */ public class Test { public static void main(String[] args) { Person.test( 2 ); } } class Person{ public static void test( final int m){ final int n = 1 ; n= 2 ; System.out.println(n); m= 3 ; System.out.println(m); } } |
java基础练习:常用类(Object、String、StringBuffer、包装类)
Object
练习1:根据给定的类(类的属性:name,age,phone,job),重写equals方法,要求是name,age相同,即认为对象相同,要求:不能用工具生成,自己实现的equals不能和工具生成的一样
String
上面图中输出的数组,对应0-9的出现次数
正则
练习1:一次性获取三门课程的成绩,保存到数组,然后输出
String常量池
练习1:下面输出结果是?并说明原因
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
|
package com.qzcsbj; public class Test { public static void main(String[] args) { System. out .println( "=========使用双引号直接创建字符串=======" ); String addr = "北京" ; System. out .println(Integer.toHexString(addr.hashCode())); addr = "上海" ; System. out .println(Integer.toHexString(addr.hashCode())); String name = "jack" ; System. out .println(Integer.toHexString(name.hashCode())); String name2 = "jack" ; System. out .println(Integer.toHexString(name2.hashCode())); System. out .println( "equals:" + name. equals (name2)); System. out .println( "name==name2: " +(name==name2)); System. out .println(); System. out .println( "=========使用构造方法创建字符串=======" ); String name3 = new String( "jack" ); System. out .println(Integer.toHexString(name3.hashCode())); String name4 = new String( "jack" ); System. out .println(Integer.toHexString(name4.hashCode())); System. out .println( "equals:" + name3. equals (name4)); System. out .println( "name3==name4: " +(name3==name4)); System. out .println(); System. out .println( "=========两种方式对象比较=======" ); System. out .println( "name==name3: " +(name==name3)); System. out .println( "equals:" + name. equals (name3)); } } |
练习2:下面的输出结果是?并说明原因
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
|
String s1 = new String( "qzcsbj" ); String s2 = new String( "qzcsbj" ); String s3 = "qzcsbj" ; String s4 = "qzcsbj" ; String s5 = "qz" ; String s6 = "csbj" ; String s7 = s5 + s6; String s8 = "qz" + "csbj" ; String s9 = s5 + "csbj" ; System. out .println(s1==s2); System. out .println(s1. equals (s2)); System. out .println(s3==s4); System. out .println(s3. equals (s4)); System. out .println(s1==s3); System. out .println(s1. equals (s3)); System. out .println(s3==s7); System. out .println(s3. equals (s7)); System. out .println(s3==s8); System. out .println(s3. equals (s8)); System. out .println(s3==s9); System. out .println(s3. equals (s9)); System. out .println(s8==s7); System. out .println(s8. equals (s7)); System. out .println(s9==s7); System. out .println(s9. equals (s7)); |
练习3:下面的输出结果是?并说明原因
1
2
3
4
5
6
7
8
|
public class Test { public static void main(String[] args) { Integer a = 666, b = 666; System. out .println(a == b); Integer c = 66, d = 66; System. out .println(c == d); } } |
String和StringBuffer性能对比
练习1:写代码测试字符串拼接的性能,比如拼接10万次
练习2:下面输出结果是?并说明原因
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
|
package com.qzcsbj; /** * @公众号 : 全栈测试笔记 * @博客 : www.cnblogs.com/uncleyong * @微信 : ren168632201 * @描述 : <> */ public class Test { public static void main(String[] args) { String s1 = "hello" ; String s2 = "java" ; System. out .println(s1+ "," + s2); test(s1,s2); System. out .println(s1+ "," + s2); StringBuffer stringBuffer1 = new StringBuffer( "hello" ); StringBuffer stringBuffer2 = new StringBuffer( "java" ); System. out .println(stringBuffer1+ "," + stringBuffer2); test2(stringBuffer1,stringBuffer2); System. out .println(stringBuffer1+ "," + stringBuffer2); } public static void test(String s1,String s2){ s1 = s2; s2=s1+s2; System. out .println(s1+ "," + s2); } public static void test2(StringBuffer stringBuffer1,StringBuffer stringBuffer2){ stringBuffer1 = stringBuffer2; stringBuffer2.append(stringBuffer1); System. out .println(stringBuffer1+ "," + stringBuffer2); } } |
包装类
练习1:下面输出结果是?并说明原因
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
|
package com.qzcsbj; /** * @公众号 : 全栈测试笔记 * @博客 : www.cnblogs.com/uncleyong * @微信 : ren168632201 * @描述 : <> */ public class Test { public static void main(String[] args) { int m = 6 ; Integer n = new Integer( 1 ); int p = m + n; System.out.println(p); int x= 3 ; Integer y = new Integer( 3 ); Integer z = new Integer( 3 ); System.out.println(y==z); System.out.println(x==z); Integer integer = new Integer( 10 ); test(integer); System.out.println(integer); } public static void test(Integer integer){ integer= 666 ; // 修改为666 } } |
练习2:下面代码是否正确?并说明原因
Object obj=5;
笔试题
练习2:输入一个字符串,输出出现次数最多的前2个字符及出现次数
练习3:输入字符串(中间有若干个空格隔开),要求:以空格隔开,删除空格两边字符串的重复字符再输出,例:aabbbcc ddaaaffggbb变为abc dafgb(注意:输入的空格得保留下来)
java基础练习:常用类(Date、SimpleDateFormat、Calendar、Math、Random)
Date、SimpleDateFormat
练习1:假设1910年1月1日是星期一,请写一个函数:该函数输入为1910年1月1日后的任意一天日期,格式为字符串YYYY-MM-DD,输出为该日期是星期几的英文。
Calendar
练习1:获取2008年2月有多少天
练习2:输入4位年份,输出当年2月天数,不能用判断是否闰年的方式。如:输入2019,输出的是28天
笔试题
练习1:实现一个类,打印昨天的当前时刻
java基础练习:集合-Collection(ArrayList、LinkedList等)
ArrayList
练习1:定义Student类并创建对象,把对象放入ArrayList,循环ArrayList,打印学生信息
练习2:提示用户输入整数,存放到ArrayList集合中,输入0代表结束。分别使用for、foreach、iterator循环集合,并删除所有是3的倍数的元素
练习3:定义一个学生类Stu,属性:id、name、age
1.提示用户循环输入学生的信息,存储到ArrayList集合中
2.输出所有学生的信息
3.根据学号修改指定学生信息
4.根据学号删除学生
练习4:list1=[A,B,C],list2=[B,C,A],对比两个列表,内容相同返回ture,不同返回false
假设list中是String类型对象,下面运行结果是true
1
2
3
4
|
String[] strArray = { "A" , "B" , "C" }; List list1 = Arrays.asList(strArray); String[] strArray2 = { "B" , "C" , "A" }; List list2 = Arrays.asList(strArray2); |
ArrayList和LinkedList性能对比:读取操作
java基础练习:集合-Map(HashMap)、Collections工具类
练习2:统计一个字符串中每个字符出现的次数,保存到HashMap集合中
练习3:统计一个字符串中每个字符出现的次数,保存到HashMap集合中,通过排序,打印输出次数最多的前三个字符及次数
java基础练习:异常
练习1:下面结果是?并说明原因;另外,多个catch的时候,有什么要求?
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
|
package com.qzcsbj; public class TestException { public static void main(String[] args) { int [] nums = { 12, 4, 23 }; try { System. out .println(nums[3]); System. out .println(5 / 0); System. out .println( "try中最后的代码。。。" ); } catch (ArrayIndexOutOfBoundsException e) { System. out .println( "数组下标越界异常。。。:" + e.getMessage()); } catch (ArithmeticException e) { System. out .println( "算术异常。。。:" + e.getMessage()); } System. out .println( "异常之后的代码。。。" ); } } |
java基础练习:枚举、泛型
练习1:list1=[A,B,C],list2=[B,C,A],对比两个列表,内容相同返回ture,不同返回false。要求定义一个泛型方法实现(解决类型侵入的问题)
说明:A可以任意类型的对象,
比如Student对象
1
2
3
4
5
6
7
|
List<Student> stu1s = new ArrayList<>(); stu1s.add( new Student( "jack" , 18 )); stu1s.add( new Student( "tom" , 19 )); List<Student> stu2s = new ArrayList<>(); stu2s.add( new Student( "tom" , 19 )); stu2s.add( new Student( "jack" , 18 )); |
也可以是String对象
1
2
3
4
|
String[] strArray = { "A" , "B" , "C" }; List list1 = Arrays.asList(strArray); String[] strArray2 = { "B" , "C" , "A" }; List list2 = Arrays.asList(strArray2); |
java基础练习:I/O输入输出流
练习1:获取目录下的所有文件,包括子目录下的文件
练习2:删除给定目录下的空目录(包含子目录下的),测试文件夹见Q群文件
1
2
3
4
5
6
7
|
qzcsbj(目录) |-test1(目录) |-test2(目录) |-test3(目录) |-test4(目录) |-test2.txt(文件) |-test1.txt(文件) |
练习3:将一个音频文件复制一份
练习4:将一个文本文件复制一份
练习5:提示用户输入一行英文(比如:hello java),将英文单词的首尾字母大写,其它字母小写,然后逐行保存到文件test.txt中,文件内容为:
1
2
|
Hello Java |
java基础练习:反射
练习1:读取所有数据并保存到对象集合
1
|
List<TestCase> testcases = new ArrayList<TestCase>(); |
下面excel的表单名是:testcase
java基础练习:JDBC
练习1:解析配置文件jdbc.properties
1
2
3
4
|
jdbc.driver=com.mysql.jdbc.Driver jdbc.url=jdbc:mysql: //192.168.168.168:3360/gifts?useUnicode=true&characterEncoding=utf-8&useSSL=true jdbc.username=root jdbc.password= 123456 |
练习2:从数据库查询数据
sql查询结果:
代码操作结果
详见:https://www.cnblogs.com/uncleyong/p/15867779.html
java自动化基础:配置文件解析(properties)
练习1:解析配置文件jdbc.properties
1
2
3
4
|
jdbc.driver=com.mysql.jdbc.Driver jdbc.url=jdbc:mysql: //192.168.168.168:3360/gifts?useUnicode=true&characterEncoding=utf-8&useSSL=true jdbc.username=root jdbc.password= 123456 |
详见:https://www.cnblogs.com/uncleyong/p/15867779.html
java自动化基础:fastjson的使用(处理json字符串、json数组)
练习1:将json字符串转化成map,字符串:{"username":"qzcsbj", "password":"123456"}
练习2:json数组转换为对象集合,[{"name":"jack","age":"18"},{"name":"tom","age":"19"}]
详见:https://www.cnblogs.com/uncleyong/p/16683578.html
java自动化基础:jsonpath的使用
练习1:从下面提取token
1
|
{ "code" :9420, "msg" : "恭喜qzcsbj,登录成功" , "token" : "538bbaba44be5d3d3856718e6c637d02" } |
格式化
1
2
3
4
5
|
{ "code" : 9420, "msg" : "恭喜qzcsbj,登录成功" , "token" : "538bbaba44be5d3d3856718e6c637d02" } |
练习2:从下面提取username是“韧”的phone
1
|
{ "code" : "0" , "msg" : "sucess" , "data" :[{ "username" : "韧" , "realname" : "tester1" , "sex" : "1" , "phone" : "13800000001" },{ "username" : "全栈测试笔记" , "realname" : "tester2" , "sex" : "1" , "phone" : "13800000002" }]} |
格式化
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
|
{ "code" : "0" , "msg" : "sucess" , "data" : [ { "username" : "韧" , "realname" : "tester1" , "sex" : "1" , "phone" : "13800000001" }, { "username" : "全栈测试笔记" , "realname" : "tester2" , "sex" : "1" , "phone" : "13800000002" } ] } |
详见:https://www.cnblogs.com/uncleyong/p/16676791.html
java自动化基础:java操作excel(通过POI)
练习1:读取所有数据,并打印出来,表单名:testcase
详见:https://www.cnblogs.com/uncleyong/p/15867741.html
java自动化基础:HttpClient的使用(get、post请求)
详见:https://www.cnblogs.com/uncleyong/p/15867745.html
java自动化基础:TestNG用法
详见:https://www.cnblogs.com/uncleyong/p/15867747.html
★★★★☆【综合应用】:java接口自动化测试框架实现
java + testng + httpclient + allure + ...
详见:https://www.cnblogs.com/uncleyong/p/15867903.html
参考答案
登录后可以查看参考答案,也可以私聊作者获取。
原文:https://www.cnblogs.com/uncleyong/p/15828510.html
更多笔试题:https://www.cnblogs.com/uncleyong/p/11119489.html