function
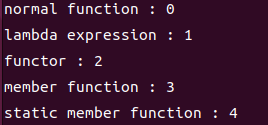
1 #include <iostream> 2 #include <vector> 3 #include <list> 4 #include <map> 5 #include <set> 6 #include <string> 7 #include <algorithm> 8 #include <functional> 9 #include <memory> 10 using namespace std; 11 12 //声明一个模板 13 typedef std::function<int(int)> Functional; 14 15 //normal function 16 int TestFunc(int a) 17 { 18 return a; 19 } 20 21 //lambda expression 22 auto lambda = [](int a)->int{return a;}; 23 24 //functor仿函数 25 class Functor 26 { 27 public: 28 int operator() (int a) 29 { 30 return a; 31 } 32 }; 33 34 //类的成员函数和类的静态成员函数 35 class CTest 36 { 37 public: 38 int Func(int a) 39 { 40 return a; 41 } 42 static int SFunc(int a) 43 { 44 return a; 45 } 46 }; 47 48 int main(int argc, char* argv[]) 49 { 50 //封装普通函数 51 Functional obj = TestFunc; 52 int res = obj(0); 53 cout << "normal function : " << res << endl; 54 55 //封装lambda表达式 56 obj = lambda; 57 res = obj(1); 58 cout << "lambda expression : " << res << endl; 59 60 //封装仿函数 61 Functor functorObj; 62 obj = functorObj; 63 res = obj(2); 64 cout << "functor : " << res << endl; 65 66 //封装类的成员函数和static成员函数 67 CTest t; 68 obj = std::bind(&CTest::Func, &t, std::placeholders::_1); 69 res = obj(3); 70 cout << "member function : " << res << endl; 71 obj = CTest::SFunc; 72 res = obj(4); 73 cout << "static member function : " << res << endl; 74 return 0; 75 }
2.绑定函数
1 TestFun t; 2 t.bindFun(std::bind(&QtTestBindAndFunction::AAA,this,99)); 3 t.test(); 4 5 class TestFun 6 { 7 public: 8 void test() { qDebug() << m_fun(); } 9 void bindFun(const std::function<int()>& f) { m_fun = f; } 10 11 private: 12 std::function<int()> m_fun; 13 };