使用countDownLatch 实现 三体动漫中航天服检查流程
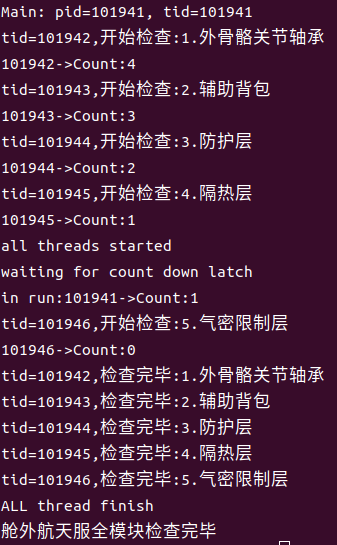
1 #include <boost/foreach.hpp> //-lboost_iostreams 2 #include "muduo/base/CountDownLatch.h" 3 #include "muduo/base/Thread.h" 4 #include <iostream> 5 #include <vector> 6 #include <string> 7 #include <vector> 8 9 using namespace std; 10 11 class TestFun 12 { 13 14 public: 15 TestFun(int numThreads,vector<string>* p) : latch_(numThreads),pVector(p) 16 { 17 for (int i = 0; i < numThreads; ++i) 18 { 19 threads_.emplace_back(new muduo::Thread(std::bind(&TestFun::threadFunc,this),p->at(i))); 20 } 21 } 22 23 void run() 24 { 25 for (auto& thr : threads_) 26 { 27 thr->start(); 28 } 29 printf("all threads started\n"); 30 31 printf("waiting for count down latch\n"); 32 printf("in run:%d->Count:%d\n",muduo::CurrentThread::tid(),latch_.getCount()); 33 34 latch_.wait(); //会原子unlock mutex并进入等待,wait执行完毕会自动重新加锁 35 } 36 37 void threadFunc() 38 { 39 printf("tid=%d,开始检查:%s\n",muduo::CurrentThread::tid(),muduo::CurrentThread::name()); 40 latch_.countDown(); //必须在while前,启动一个减1,0会唤醒wait()启动完毕 41 printf("%d->Count:%d\n",muduo::CurrentThread::tid(),latch_.getCount()); 42 bool running = true; 43 while (running) 44 { 45 sleep(1); 46 running = false; 47 } 48 49 printf("tid=%d,检查完毕:%s \n",muduo::CurrentThread::tid(),muduo::CurrentThread::name()); 50 } 51 52 53 void joinAll() 54 { 55 for (auto& thr : threads_) 56 { 57 thr->join(); 58 } 59 60 printf("ALL thread finish\n"); 61 } 62 63 64 private: 65 muduo::CountDownLatch latch_; 66 std::vector<std::unique_ptr<muduo::Thread>> threads_; 67 vector<string>* pVector; 68 }; 69 70 int main() 71 { 72 vector<string> vec; 73 vec.emplace_back("1.外骨骼关节轴承"); 74 vec.emplace_back("2.辅助背包"); 75 vec.emplace_back("3.防护层"); 76 vec.emplace_back("4.隔热层"); 77 vec.emplace_back("5.气密限制层"); 78 //BOOST_FOREACH(std::string s,vec) cout<<s<<endl; 79 80 printf("Main: pid=%d, tid=%d\n", ::getpid(), muduo::CurrentThread::tid()); 81 82 83 TestFun t(vec.size(),&vec); 84 t.run(); 85 t.joinAll(); 86 87 printf("舱外航天服全模块检查完毕\n"); 88 89 90 return 0; 91 }
1 #include <boost/foreach.hpp> //-lboost_iostreams 2 #include "muduo/base/CountDownLatch.h" 3 #include "muduo/base/Thread.h" 4 #include <iostream> 5 #include <vector> 6 #include <string> 7 #include <vector> 8 9 using namespace std; 10 11 #define THREAD_NUM 2 12 13 muduo::CountDownLatch order_latch(1); 14 muduo::CountDownLatch answer_latch(THREAD_NUM); 15 16 void workerThread() 17 { 18 printf("work thread pid = %d->Wait\n" , muduo::CurrentThread::tid()); 19 order_latch.wait(); //等待发令 20 printf("work thread pid = %d->Start\n", muduo::CurrentThread::tid()); //收到起跑 21 22 bool running = true; 23 while (running) 24 { 25 sleep(1); 26 answer_latch.countDown(); //结束 27 running = false; 28 } 29 30 printf("work thread pid = %d->Stop\n" , muduo::CurrentThread::tid()); //收到起跑 31 } 32 33 int main() 34 { 35 char c[10]; 36 vector<unique_ptr<muduo::Thread>> threads; 37 38 for (int i = 0; i < THREAD_NUM; i++) 39 { 40 sprintf(c,"%d",i); 41 auto p = new muduo::Thread(workerThread,string(c)); 42 threads.emplace_back(p); 43 p->start(); 44 } 45 46 sleep(1); 47 48 printf("order thread pid = %d->Start\n", muduo::CurrentThread::tid()); 49 order_latch.countDown(); //发起跑令 50 51 printf("order thread pid = %d->Wait\n", muduo::CurrentThread::tid()); 52 answer_latch.wait(); 53 54 printf("order thread pid = %d->Stop\n", muduo::CurrentThread::tid()); //结束 55 56 for (auto& thr : threads) 57 { 58 thr->join(); 59 } 60 61 printf("All thread finish\n"); 62 return 0; 63 }
备注:编译需链接 muduo库和boost库
-lmuduo_base -lboost_iostreams