重视Linq技术_6
Filtering
//1、Simple Local Filter
string[] names = { "Tom", "Dick", "Harry", "Mary", "Jay" };
IEnumerable<string> query =
names.Where (name => name.EndsWith ("y"));
query.Dump ("In lambda syntax");
query =
from n in names
where n.EndsWith ("y")
select n;
query.Dump ("In query comprehension syntax");
IEnumerable<string> query =
names.Where (name => name.EndsWith ("y"));
query.Dump ("In lambda syntax");
query =
from n in names
where n.EndsWith ("y")
select n;
query.Dump ("In query comprehension syntax");
//2、Multiple Where Clauses
var names = new[] { "Tom", "Dick", "Harry", "Mary", "Jay" }.AsQueryable();
var query =
from n in names
where n.Length > 3
let u = n.ToUpper()
where u.EndsWith ("Y")
select u;
query.Dump();
//3、Indexed Filtering
var query =
from n in names
where n.Length > 3
let u = n.ToUpper()
where u.EndsWith ("Y")
select u;
query.Dump();
//3、Indexed Filtering
string[] names = { "Tom", "Dick", "Harry", "Mary", "Jay" };
names.Where ((n, i) => i % 2 == 0).Dump ("Skipping every second element");
names.Where ((n, i) => i % 2 == 0).Dump ("Skipping every second element");
//4、Contains and LIKE with LINQ to SQL
Customers.Where (c => c.Name.Contains ("a"))
.Dump ("Notice the SQL translation uses LIKE");
Customers.Where (c => c.Name.StartsWith ("J"))
.Dump ("StartsWith and EndsWith also translate to LIKE");
Customers.Where (c => SqlMethods.Like (c.Name, "_ar%y"))
.Dump ("A more complex use of LIKE");
// The following string functions all have translations to SQL:
// StartsWith, EndsWith, Contains, Length, IndexOf,
// Trim, Substring, Insert, Remove, Replace,
// ToUpper, ToLower
.Dump ("Notice the SQL translation uses LIKE");
Customers.Where (c => c.Name.StartsWith ("J"))
.Dump ("StartsWith and EndsWith also translate to LIKE");
Customers.Where (c => SqlMethods.Like (c.Name, "_ar%y"))
.Dump ("A more complex use of LIKE");
// The following string functions all have translations to SQL:
// StartsWith, EndsWith, Contains, Length, IndexOf,
// Trim, Substring, Insert, Remove, Replace,
// ToUpper, ToLower
//5、IN and NOT IN with LINQ to SQL
string[] chosenOnes = { "Tom", "Jay" };
Customers.Where (c => chosenOnes.Contains (c.Name))
.Dump ("This translates to SQL WHERE ... IN");
Customers.Where (c => !chosenOnes.Contains (c.Name))
.Dump ("This translates to SQL WHERE NOT ... IN");
Customers.Where (c => chosenOnes.Contains (c.Name))
.Dump ("This translates to SQL WHERE ... IN");
Customers.Where (c => !chosenOnes.Contains (c.Name))
.Dump ("This translates to SQL WHERE NOT ... IN");
//6、Extra - Where-based Subqueries
var names = new[] { "Tom", "Dick", "Harry", "Mary", "Jay" }.AsQueryable();
(
from n in names
where n.Length == names.Min (n2 => n2.Length)
select n
)
.Dump ("Basic subquery");
// The same principle works well in LINQ to SQL:
(
from c in Customers
where c.Name.Length == Customers.Min (c2 => c2.Name.Length)
select c
)
.Dump ("Basic subquery, LINQ to SQL");
// We can construct similar subqueries across association properties:
(
from c in Customers
where c.Purchases.Any (p => p.Price > 1000)
select c
)
.Dump ("Customers who have purchased at least one item > $1000");
(
from n in names
where n.Length == names.Min (n2 => n2.Length)
select n
)
.Dump ("Basic subquery");
// The same principle works well in LINQ to SQL:
(
from c in Customers
where c.Name.Length == Customers.Min (c2 => c2.Name.Length)
select c
)
.Dump ("Basic subquery, LINQ to SQL");
// We can construct similar subqueries across association properties:
(
from c in Customers
where c.Purchases.Any (p => p.Price > 1000)
select c
)
.Dump ("Customers who have purchased at least one item > $1000");
//6、Extra - Where-based Subqueries with let
// The let keyword in comprehension queries comes in useful with subqueries: it lets
// you re-use the subquery in the projection:
// you re-use the subquery in the projection:
from c in Customers
let highValuePurchases = c.Purchases.Where (p => p.Price > 1000)
where highValuePurchases.Any()
select new
{
c.Name,
highValuePurchases
}
let highValuePurchases = c.Purchases.Where (p => p.Price > 1000)
where highValuePurchases.Any()
select new
{
c.Name,
highValuePurchases
}
//7、Take and Skip
// reflection (using LINQ, of course!) The following query extracts all type
// names in the mscorlib assembly:
string[] typeNames =
(from t in typeof (int).Assembly.GetTypes() select t.Name).ToArray();
typeNames
.Where (t => t.Contains ("Exception"))
.OrderBy (t => t)
.Take (20)
.Dump ("The first 20 matches");
typeNames
.Where (t => t.Contains ("Exception"))
.OrderBy (t => t)
.Skip (20)
.Take (20)
.Dump ("Matches 21 through 40");
(from t in typeof (int).Assembly.GetTypes() select t.Name).ToArray();
typeNames
.Where (t => t.Contains ("Exception"))
.OrderBy (t => t)
.Take (20)
.Dump ("The first 20 matches");
typeNames
.Where (t => t.Contains ("Exception"))
.OrderBy (t => t)
.Skip (20)
.Take (20)
.Dump ("Matches 21 through 40");
//8、TakeWhile and SkipWhile
int[] numbers = { 3, 5, 2, 234, 4, 1 };
numbers.TakeWhile (n => n < 100).Dump ("TakeWhile");
numbers.SkipWhile (n => n < 100).Dump ("SkipWhile");
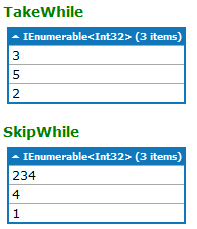
numbers.TakeWhile (n => n < 100).Dump ("TakeWhile");
numbers.SkipWhile (n => n < 100).Dump ("SkipWhile");
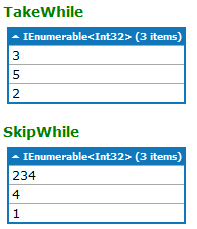
//9、Distinct
"HelloWorld".Distinct()
Purchases.Select (p => p.Description).Distinct()
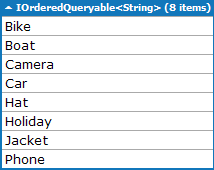