重视Linq技术_2
//Deferred Execution //1、Introduction var numbers = new List<int>(); numbers.Add (1); //10 IEnumerable<int> query = numbers.Select (n => n * 10);// Build query numbers.Add (2); //20 // Sneak in an extra element query.Dump ("Notice both elements are returned in the result set");
//2、Reevaluation var numbers = new List<int>() { 1, 2 }; IEnumerable<int> query = numbers.Select (n => n * 10); query.Dump ("Both elements are returned"); numbers.Clear(); query.Dump ("All the elements are now gone!");
//3、Defeating Reevaluation var numbers = new List<int>() { 1, 2 }; List<int> timesTen = numbers .Select (n => n * 10) .ToList(); // Executes immediately into a List<int> numbers.Clear(); timesTen.Count.Dump ("Still two elements present");
//4、Outer Variables in a Loop IEnumerable<char> query = "Not what you might expect"; query = query.Where (c => c != 'a'); query = query.Where (c => c != 'e'); query = query.Where (c => c != 'i'); query = query.Where (c => c != 'o'); query = query.Where (c => c != 'u'); new string (query.ToArray()).Dump ("All vowels are stripped, as you'd expect."); query = "Not what you might expect"; foreach (char vowel in "aeiou") query = query.Where (c => c != vowel); new string (query.ToArray()).Dump ("Notice that only the 'u' is stripped!");
//5、Inside the foreach Statement IEnumerable<char> query = "Not what you might expect"; IEnumerable<char> vowels = "aeiou"; IEnumerator<char> rator = vowels.GetEnumerator (); char vowel; while (rator.MoveNext()) { vowel = rator.Current; query = query.Where (c => c != vowel); } query.Dump();
//6、Working around Outer Vars IEnumerable<char> query = "Not what you might expect"; foreach (char vowel in "aeiou") { char temp = vowel; query = query.Where (c => c != temp); } query.Dump ("The workaround");
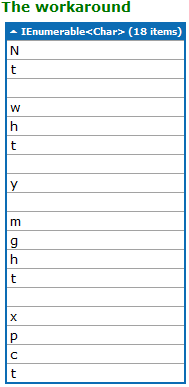
//Subqueries
//1、Basic Subquery
string[] musos = { "Roger Waters", "David Gilmour", "Rick Wright" };
musos.OrderBy (m => m.Split().Last()) .Dump ("Sorted by last name");
//2、Reformulating the Subquery
string[] names = { "Tom", "Dick", "Harry", "Mary", "Jay" };
names.Where (n => n.Length == names.OrderBy (n2 => n2.Length)
.Select (n2 => n2.Length).First())
.Dump();//”Tom,Jay”
var query =
from n in names
where n.Length == (from n2 in names orderby n2.Length select n2.Length).First()
select n;
query.Dump ("Same query in comprehension syntax");//”Tom,Jay”
query =
from n in names
where n.Length == names.OrderBy (n2 => n2.Length).First().Length
select n;
query.Dump ("Reformulated");//”Tom,Jay”
query =
from n in names
where n.Length == names.Min (n2 => n2.Length)
select n;
query.Dump ("Same result, using Min aggregation"); //”Tom,Jay”
string[] names = { "Tom", "Dick", "Harry", "Mary", "Jay" };
names.Where (n => n.Length == names.OrderBy (n2 => n2.Length)
.Select (n2 => n2.Length).First())
.Dump();//”Tom,Jay”
var query =
from n in names
where n.Length == (from n2 in names orderby n2.Length select n2.Length).First()
select n;
query.Dump ("Same query in comprehension syntax");//”Tom,Jay”
query =
from n in names
where n.Length == names.OrderBy (n2 => n2.Length).First().Length
select n;
query.Dump ("Reformulated");//”Tom,Jay”
query =
from n in names
where n.Length == names.Min (n2 => n2.Length)
select n;
query.Dump ("Same result, using Min aggregation"); //”Tom,Jay”
//Progreesive Query Building
var names = new[] { "Tom", "Dick", "Harry", "Mary", "Jay" }.AsQueryable();
(
names
.Select (n => n.Replace ("a", "").Replace ("e", "").Replace ("i", "").Replace ("o", "").Replace ("u", ""))
.Where (n => n.Length > 2)
.OrderBy (n => n)
)
.Dump ("A lambda syntax query");//Dck,Hrry,Mry
(
names
.Select (n => n.Replace ("a", "").Replace ("e", "").Replace ("i", "").Replace ("o", "").Replace ("u", ""))
.Where (n => n.Length > 2)
.OrderBy (n => n)
)
.Dump ("A lambda syntax query");//Dck,Hrry,Mry
(
from n in names
where n.Length > 2
orderby n
select n.Replace ("a", "").Replace ("e", "").Replace ("i", "").Replace ("o", "").Replace ("u", "")
)
.Dump ("An incorrect translation to comprehension syntax");/Dck,Hrry,Jy,Mry,Tm
IEnumerable<string> query =
from n in names
select n.Replace ("a", "").Replace ("e", "").Replace ("i", "").Replace ("o", "").Replace ("u", "");
query = from n in query where n.Length > 2 orderby n select n; //Dck,Hrry,Mry
query.Dump ("A correct translation to comprehension syntax, querying in two steps");
//Wrapping Queries
var names = new[] { "Tom", "Dick", "Harry", "Mary", "Jay" }.AsQueryable();
IEnumerable<string> query =
from n1 in
(
from n2 in names
select n2.Replace ("a", "").Replace ("e", "").Replace ("i", "").Replace ("o", "").Replace ("u", "")
)
where n1.Length > 2 orderby n1 select n1;
query.Dump ("Here, one query wraps another");
var sameQuery = names
.Select (n => n.Replace ("a", "").Replace ("e", "").Replace ("i", "").Replace ("o", "").Replace ("u", ""))
.Where (n => n.Length > 2)
.OrderBy (n => n);
sameQuery.Dump ("In lambda syntax, such queries translate to a linear chain of query operators");
IEnumerable<string> query =
from n1 in
(
from n2 in names
select n2.Replace ("a", "").Replace ("e", "").Replace ("i", "").Replace ("o", "").Replace ("u", "")
)
where n1.Length > 2 orderby n1 select n1;
query.Dump ("Here, one query wraps another");
var sameQuery = names
.Select (n => n.Replace ("a", "").Replace ("e", "").Replace ("i", "").Replace ("o", "").Replace ("u", ""))
.Where (n => n.Length > 2)
.OrderBy (n => n);
sameQuery.Dump ("In lambda syntax, such queries translate to a linear chain of query operators");
//Projection Strategies
//1、Ojbect Initializers
void Main()
{
var names = new[] { "Tom", "Dick", "Harry", "Mary", "Jay" }.AsQueryable();
IEnumerable<TempProjectionItem> temp =
from n in names
select new TempProjectionItem
{
Original = n,
Vowelless = n.Replace ("a", "").Replace ("e", "").Replace ("i", "").Replace ("o", "").Replace ("u", "")
};
temp.Dump();
}
class TempProjectionItem
{
public string Original; //Original name
public string Vowelless; //Vowel-stripped name
}
//Define other methods and classes here
//2、Anonymous Types
//With the into keyword we can do this in one step:
(
from n in names
select new
{
Original = n,
Vowelless = n.Replace ("a", "").Replace ("e", "").Replace ("i", "").Replace ("o", "").Replace ("u", "")
}
into temp
where temp.Vowelless.Length > 2
select temp.Original
)
.Dump ("With the 'into' keyword"); //Dick,Harry,Mary
from n in names
select new
{
Original = n,
Vowelless = n.Replace ("a", "").Replace ("e", "").Replace ("i", "").Replace ("o", "").Replace ("u", "")
}
into temp
where temp.Vowelless.Length > 2
select temp.Original
)
.Dump ("With the 'into' keyword"); //Dick,Harry,Mary
//3、The let Keyword
var names = new[] { "Tom", "Dick", "Harry", "Mary", "Jay" }.AsQueryable();
(
from n in names
let vowelless = n.Replace ("a", "").Replace ("e", "").Replace ("i", "").Replace ("o", "").Replace ("u", "")
where vowelless.Length > 2
orderby vowelless
select n //Thanks to let, n is still in scope.
)
.Dump();
(
from n in names
let vowelless = n.Replace ("a", "").Replace ("e", "").Replace ("i", "").Replace ("o", "").Replace ("u", "")
where vowelless.Length > 2
orderby vowelless
select n //Thanks to let, n is still in scope.
)
.Dump();