重视Linq技术_1
推荐一个Linq学习工具:LinqPad
下载:
for .net 3.5:http://www.linqpad.net/GetFile.aspx?LINQPad.exe
for .net 4.0:http://www.linqpad.net/GetFile.aspx?LINQPad4.zip
//1、Now for a simple LINQ-to-objects query expression (notice no semicolon):
from word in "The quick brown fox jumps, over the lazy dog".Split()
orderby word.Length
select word
orderby word.Length
select word
//2、 Setting the query language to "C# Statement(s)" permits multiple statements:
var words =
from word in "The quick brown fox jumps over the lazy dog".Split()
orderby word.ToUpper()
select word;
from word in "The quick brown fox jumps over the lazy dog".Split()
orderby word.ToUpper()
select word;
var duplicates =
from word in words
group word.ToUpper() by word.ToUpper() into g
where g.Count() > 1
select new { g.Key, Count = g.Count() };
from word in words
group word.ToUpper() by word.ToUpper() into g
where g.Count() > 1
select new { g.Key, Count = g.Count() };
// The Dump extension method writes out queries: // Notice that we do need semicolons now!
//3、Key Pionts
from p in Products
let spanishOrders = p.OrderDetails.Where (o => o.Order.ShipCountry == "Spain")
where spanishOrders.Any()
orderby p.ProductName
select new
{
p.ProductName,
p.Category.CategoryName,
Orders = spanishOrders.Count(),
TotalValue = spanishOrders.Sum (o => o.UnitPrice * o.Quantity)
}
let spanishOrders = p.OrderDetails.Where (o => o.Order.ShipCountry == "Spain")
where spanishOrders.Any()
orderby p.ProductName
select new
{
p.ProductName,
p.Category.CategoryName,
Orders = spanishOrders.Count(),
TotalValue = spanishOrders.Sum (o => o.UnitPrice * o.Quantity)
}
//4、Chaining Query Operatorys string[] names = { "Tom", "Dick", "Harry", "Mary", "Jay" }; IEnumerable<string> query = names .Where (n => n.Contains ("a")) .OrderBy (n => n.Length) .Select (n => n.ToUpper()); query.Dump();
//5、natural ordering int[] numbers = { 10, 9, 18, 7, 6 }; numbers.Take (3)
.Dump("Take(3) returns the first three numbers in the sequence");
numbers.Skip (3)
.Dump ("Skip(3) returns all but the first three numbers in the sequence");
numbers.Reverse()
.Dump ("Reverse does exactly as it says");
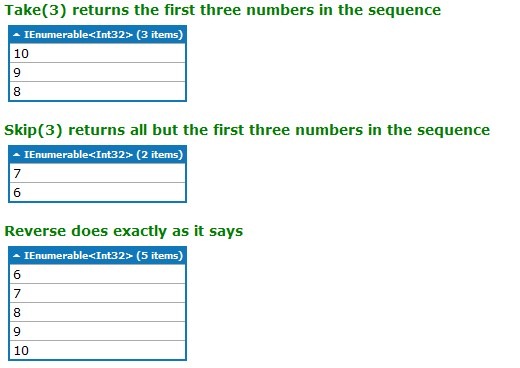
//6、 Other Operators
int[] numbers = { 10, 9, 8, 7, 6 }; // Element operators: numbers.First().Dump ("First");//10 numbers.Last().Dump ("Last");//6 numbers.ElementAt (1).Dump ("Second number");//9 numbers.OrderBy (n => n).First().Dump ("Lowest number");//6 // Aggregation operators: numbers.Count().Dump ("Count");//5 numbers.Min().Dump ("Min");//6 // Quantifiers: numbers.Contains (9).Dump ("Contains (9)");//true numbers.Any().Dump ("Any");//true numbers.Any (n => n % 2 == 1).Dump ("Has an odd numbered element");//true // Set based operators: int[] seq1 = { 1, 2, 3 }; int[] seq2 = { 3, 4, 5 }; seq1.Concat (seq2).Dump ("Concat"); seq1.Union (seq2).Dump ("Union");![]()
//8、 With AsQueryable() added, you can see the translation to lambda var names = new[] { "Tom", "Dick", "Harry", "Mary", "Jay" }.AsQueryable();
IEnumerable<string> query =
from n in names
where n.Contains ("a") // Filter elements
orderby n.Length // Sort elements
select n.ToUpper(); // Translate each element (project)
query.Dump();
//9、Mixing Syntax
string[] names = { "Tom", "Dick", "Harry", "Mary", "Jay" };
string[] names = { "Tom", "Dick", "Harry", "Mary", "Jay" };
(from n in names where n.Contains ("a") select n).Count()
.Dump ("Names containing the letter 'a'");//3
string first = (from n in names orderby n select n).First()
.Dump ("First name, alphabetically");//Dick
names.Where (n => n.Contains ("a")).Count()
.Dump ("Original query, entirely in lambda syntax");//3
names.OrderBy (n => n).First()
.Dump ("Second query, entirely in lambda syntax");//Dick
//10、LINQ: Query Comprehension Syntax
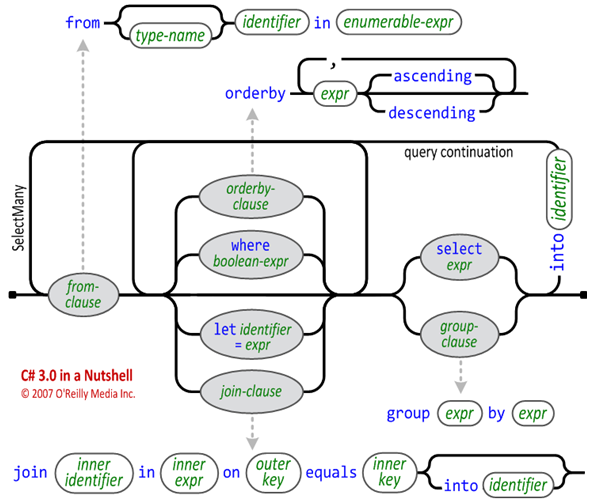