烂翻译系列之面向.NET开发人员的Dapr——示例应用程序
Traffic Control sample application
交通控制示例应用程序
In the first chapters, you've learned about basic Dapr concepts. You saw how Dapr can help you and your team construct distributed applications while reducing architectural and operational complexity. This chapter introduces the sample application that you'll use to explore the Dapr building blocks. The application targets .NET 7 and uses the latest C# 11 language features.
在第一章中,您已经了解了 Dapr 的基本概念。您了解了 Dapr 如何帮助您和您的团队构建分布式应用程序,同时降低体系结构和运作的复杂性。本章将介绍用于探索 Dapr 构建块的示例应用程序。该应用程序以.NET7为目标,并使用最新的 C # 11语言特性。
Note
注意 Download the sample application code from the Dapr Traffic Control GitHub repo. This repository contains a detailed description on how you can run the sample application on your machine.
从Dapr 交通控制 GitHub 存储库下载示例应用程序代码。此存储库包含有关如何在计算机上运行示例应用程序的详细说明。
The Traffic Control sample application simulates a highway traffic control system. Its purpose is to detect speeding vehicles and send the offending driver a fine notice. These systems actually exist in real life and here's how they work. A set of cameras (one above each lane) is placed at the beginning and end of a highway stretch (say 10 kilometers) without on- or off-ramps. As a vehicle passes underneath a camera, it takes a photograph of the vehicle. Using Optical Character Recognition (OCR) software, it extracts the license number of the vehicle from the photo. Using the entry- and exit-timestamp of each vehicle, the system calculates the average speed of that vehicle. If the average speed is above the maximum speed limit for that highway stretch, the system retrieves the driver information and automatically sends a fine notice.
交通控制示例应用程序模拟高速公路交通控制系统。 其用途是检测超速车辆,并向违规司机发送罚款通知。 这些系统实际上存在于现实生活中,下面是它们的工作原理。 一组摄像头(每个车道上方各一个)被放置在高速公路的起点和终点(假设该路段为 10 公里),没有上匝道或下匝道。 当车辆在摄像头下方经过时,摄像头会拍摄车辆照片。 使用光学字符识别 (OCR) 软件,从照片中提取车辆的车牌号。 系统使用每个车辆的入口和出口时间戳来计算该车辆的平均速度。 如果平均速度高于高速公路的最大速度限制,系统会检索司机信息并自动发送罚款通知。
Although the simulation is simple, responsibilities within the system are separated into several microservices. Figure 4.1 shows an overview of the services that are part of the application:
尽管模拟非常简单,但是系统中的职责被分为几个微服务。图4.1显示了应用程序中的服务概述:
Figure 4-1. The services in the sample application.
图 4-1. 示例应用程序中的服务。
-
The Camera Simulation is a console application that simulates vehicles and sends messages to the TrafficControl service. Every simulated car invokes both the entry and exit service endpoints. 相机模拟是一个控制台应用,它模拟车辆并向 TrafficControl 服务发送信息。每个模拟汽车都会调用入口和出口服务终结点。
-
The TrafficControl service is an ASP.NET Core Web API application that exposes the
/entrycam
and/exitcam
endpoints. Invoking an endpoint simulates a car passing under one of the entry- or exit-cameras respectively. The request message payload simply contains the license plate of the car (no actual OCR is implemented). TrafficControl 服务是一种 ASP.NET Core Web API 应用程序,它会公开/entrycam
和/exitcam
终结点。调用的终结点分别模拟一辆汽车从一个进口或出口摄像头下经过。请求消息有效载荷只包含汽车的牌照(没有实际的 OCR 实现)。 -
The FineCollection service is an ASP.NET Core Web API application that offers 1 endpoint:
/collectfine
. Invoking this endpoint will send a fine notice to the driver of the speeding vehicle. The payload of the request contains all the information about the speeding violation. FineCollection 服务是一种 ASP.NET Core Web API 应用程序,它提供 1 个终结点:/collectfine
。调用此终结点将向超速车辆的司机发送罚款通知。 有效负载包含关于超速违规的所有信息。 -
The VehicleRegistration service is an ASP.NET Core Web API application that offers 1 endpoint:
/vehicleinfo/{licensenumber}
. It's used for obtaining vehicle- and owner-information for a speeding vehicle based on the license number sent in the URL (for example,/vehicleinfo/RV-752-S
). VehicleRegistration 服务是一种 ASP.NET Core Web API 应用程序,它提供 1 个终结点:/vehicleinfo/{licensenumber}
。 它用于根据 URL 中发送的车牌号码(例如/vehicleinfo/RV-752-S
)获取超速车辆的车辆信息和车主信息。
The sequence diagram in figure 4.2 shows the simulation flow:
图 4.2 中的序列图显示了此模拟流:
Figure 4-2. Sequence diagram of the simulation flow.
图 4-2. 模拟流的序列图。
The services communicate by directly invoking each other's APIs. This design works fine, but it has some drawbacks.
服务通过直接调用彼此的 API 进行通信。 此设计可以正常运作,但有一些缺点。
The biggest challenge is that the call-chain will break if one of the services is off-line. Decoupling services by replacing direct calls with asynchronous messaging would solve this issue. Asynchronous messaging is typically implemented with a message broker like RabbitMQ or Azure Service Bus.
最大的难题是,如果其中一项服务处于脱机状态,则调用链将中断。 通过将直接调用替换为异步消息传递来分离服务,可以解决此问题。 异步消息传送通常使用消息代理(如 RabbitMQ 或 Azure 服务总线)来实现。
Another drawback is that the vehicle state for every vehicle is stored in memory in the TrafficControl service. This state is lost when the service is restarted after an update or a crash. To increase system durability, state should be stored outside the service.
另一个缺点是每个车辆的车辆状态都存储在 TrafficControl 服务的内存中。 如果服务在更新或崩溃后重新启动,则此状态将丢失。 要提高系统持久性,应将状态存储在服务外部。
Using Dapr building blocks
使用 Dapr 构建基块
One of the goals of Dapr is to provide cloud-native capabilities for microservices applications. The Traffic Control application uses Dapr building blocks to increase robustness and mitigate the design drawbacks described in the previous paragraph. Figure 4.shows a Dapr-enabled version of the traffic control application:
Dapr 的目标之一是为微服务应用程序提供云原生功能。 交通控制应用程序使用 Dapr 构建基块来提高可靠性并缓解上文所述的设计缺陷所带来的影响。 图 4 展示的是启用 Dapr 版本的交通控制应用程序:
Figure 4-3. Traffic Control application with Dapr building blocks.
图 4-3. 使用 Dapr 构建基块的交通控制应用程序。
- Service invocation The Dapr service invocation building block handles request/response communication between the FineCollectionService and the VehicleRegistrationService. Because the call is a query to retrieve required data to complete the operation, a synchronous call is acceptable here. The service invocation building block provides service discovery. The FineCollection service no longer has to know where the VehicleRegistration service lives. It also implements automatic retries if the VehicleRegistration service is off-line. 服务调用 Dapr 服务调用构建块处理 FineCollectionService 和 VehicleRegistrationService 之间的请求/响应通信。 因为该调用是检索完成操作所需数据的查询,所以此处可以接受同步调用。 服务调用构建基块提供服务发现。 FineCollection 服务不再需要知道 VehicleRegistration 服务所在的位置。 如果 VehicleRegistration 服务脱机,它还会实现自动重试。
- Publish & subscribe The publish and subscribe building block handles asynchronous messaging for sending speeding violations from the TrafficControl service to the FineCollectionService. This implementation decouples the TrafficControl and FineCollection service. If the FineCollectionService were to become temporarily unavailable, data would accumulate in the queue and resume processing at a later time. RabbitMQ is the current message broker that transports messages from the producers to the consumers. As the Dapr pub/sub building block abstracts the message broker, developers don't need to learn the details of the RabbitMQ client library. Switching to another message broker doesn't require code changes, only configuration. 发布 & 订阅 发布和订阅构建基块可处理异步消息传送,以便将 TrafficControl 服务中的超速违规信息发送到 FineCollectionService。 此实现能分离 TrafficControl 和 FineCollection 服务。 如果 FineCollectionService 暂时不可用,数据会在队列中累积,并在稍后恢复处理。 当前使用RabbitMQ作为消息代理,将消息从生产者传输到消费者。 因为 Dapr 发布/订阅构建基块将消息代理抽象化,所以开发人员无需了解 RabbitMQ 客户端库的详细信息。 切换到另一个消息代理时,不需要更改代码,只需完成配置。
- State management The TrafficControl service uses the state management building block to persist vehicle state outside of the service in a Redis cache. As with pub/sub, developers don't need to learn Redis specific APIs. Switching to another data store requires no code changes. 状态管理 TrafficControl 服务使用状态管理构建基块将车辆状态持久保存服务之外的 Redis 缓存中。 与发布/订阅一样,开发人员无需了解 Redis 特定的 API。 切换到另一个数据存储时,不需要更改代码。
- Output binding The FineCollection service sends fines to the owners of speeding vehicles by email. The Dapr output binding for SMTP abstracts the email transmission using the SMTP protocol. 输出绑定 FineCollection 服务通过电子邮件将罚款信息发送给超速车辆的车主。 SMTP 的 Dapr 输出绑定使用 SMTP 协议将电子邮件传输抽象化。
- Input binding The CameraSimulation sends messages with simulated car info to the TrafficControl service using the MQTT protocol. It uses a .NET MQTT library for sending messages to Mosquitto - a lightweight MQTT broker. The TrafficControl service uses the Dapr input binding for MQTT to subscribe to the MQTT broker and receive messages. 输入绑定 CameraSimulation 使用 MQTT 协议将包含模拟车辆信息的消息发送至 TrafficControl 服务。 它使用 .NET MQTT 库将消息发送到 Mosquitto,Mosquitto 是轻量型的 MQTT 代理。 TrafficControl 服务使用 MQTT 的 Dapr 输入绑定来订阅 MQTT 代理并接收消息。
- Secrets management The FineCollectionService needs credentials for connecting to the smtp server and a license-key for a fine calculator component it uses internally. It uses the secrets management building block to obtain the credentials and the license-key. 机密管理 FineCollectionService 需要用于连接到 SMTP 服务器的凭据以及内部使用的罚款计算器组件的许可证密钥。 它使用机密管理构建块来获取凭据和许可证密钥。
- Actors The TrafficControlService has an alternative implementation based on Dapr actors. In this implementation, the TrafficControl service creates a new actor for every vehicle that is registered by the entry camera. The license number of the vehicle forms the unique actor Id. The actor encapsulates the vehicle state, which it persists in the Redis cache. When a vehicle is registered by the exit camera, it invokes the actor. The actor then calculate the average speed and possibly issue a speeding violation. 执行组件 TrafficControlService 具有基于 Dapr 执行组件的替代实现。 在此实现中,TrafficControl 服务会针对入口摄像头记录的每个车辆创建一个新的执行组件。 车辆的牌照号码构成唯一的执行组件 ID。执行组件封装车辆状态,并将其持久保存在 Redis 缓存中。 当出口摄像头记录到车辆时,会调用该执行组件。 执行组件随后会计算平均车速,并可能得出超速违规结果。
Figure 4.4 shows a sequence diagram of the flow of the simulation with all the Dapr building blocks in place:
图 4.4 展示的是包含所有 Dapr 构建块的模拟流序列图:
Figure 4-4. Sequence diagram of simulation flow with Dapr building blocks.
图 4-4. 包含 Dapr 构建块的模拟流序列图。
The rest of this book features a chapter for each of the Dapr building blocks. Each chapter explains in detail how the building block works, its configuration, and how to use it. Each chapter explains how the Traffic Control sample application uses the building block.
本书的其余部分会分章节介绍每个 Dapr 构建块。 每章详细说明了构建块的工作原理、配置以及使用方法。 每章都会介绍交通控制示例应用程序对构建块的使用方式。
Hosting
承载模式
The Traffic Control sample application can run in self-hosted mode or in Kubernetes.
交通控制示例应用程序可以在自承载模式或 Kubernetes 中运行。
Self-hosted mode
自承载模式
The sample repository contains PowerShell scripts to start the infrastructure services (Redis, RabbitMQ, and Mosquitto) as Docker containers on your machine. They're located in the src/Infrastructure
folder. For every application service in the solution, the repository contains a separate folder. Each of these folders contains a start-selfhosted.ps1
PowerShell script to start the service with Dapr.
示例存储库包含 PowerShell 脚本,用于在计算机上启动基础结构服务(Redis、RabbitMQ 和 Mosquitto)作为 Docker 容器。 此类脚本位于 src/Infrastructure
文件夹中。 对于解决方案中的每个应用程序服务,存储库都包含一个单独的文件夹。 其中每个文件夹都包含 start-selfhosted.ps1
PowerShell 脚本以通过 Dapr 启动对应的服务。
Kubernetes
The src/k8s
folder in the sample repository contains the Kubernetes manifest files to run the application (including the infrastructure services) with Dapr in Kubernetes. This folder also contains a start.ps1
and stop.ps1
PowerShell script to start and stop the solution in Kubernetes. All services will run in the dapr-trafficcontrol
namespace.
示例存储库中的 src/k8s
文件夹包含清单文件,用于在 Kubernetes 中使用 Dapr 运行应用程序(包括基础结构服务)。 此文件夹还包含用于在 Kubernetes 中启动和停止解决方案的 start.ps1
和 stop.ps1
PowerShell 脚本。 所有服务都将在 dapr-trafficcontrol
命名空间中运行。
Summary
总结
The Traffic Control sample application is a microservices application that simulates a highway speed trap.
交通控制示例应用程序是模拟高速公路超速监控的微服务应用程序。
The application uses several Dapr building blocks to make it robust and cloud-native. The domain is kept simple to keep the focus on Dapr.
其中使用多个 Dapr 构基块以实现可靠的云原生应用程序。 领域简单,以专注于 Dapr。
The application will be used in the following chapters that focus on Dapr building block.
该应用程序将在以下几章中使用,这些章节会重点介绍 Dapr 构建基块。
References
参考
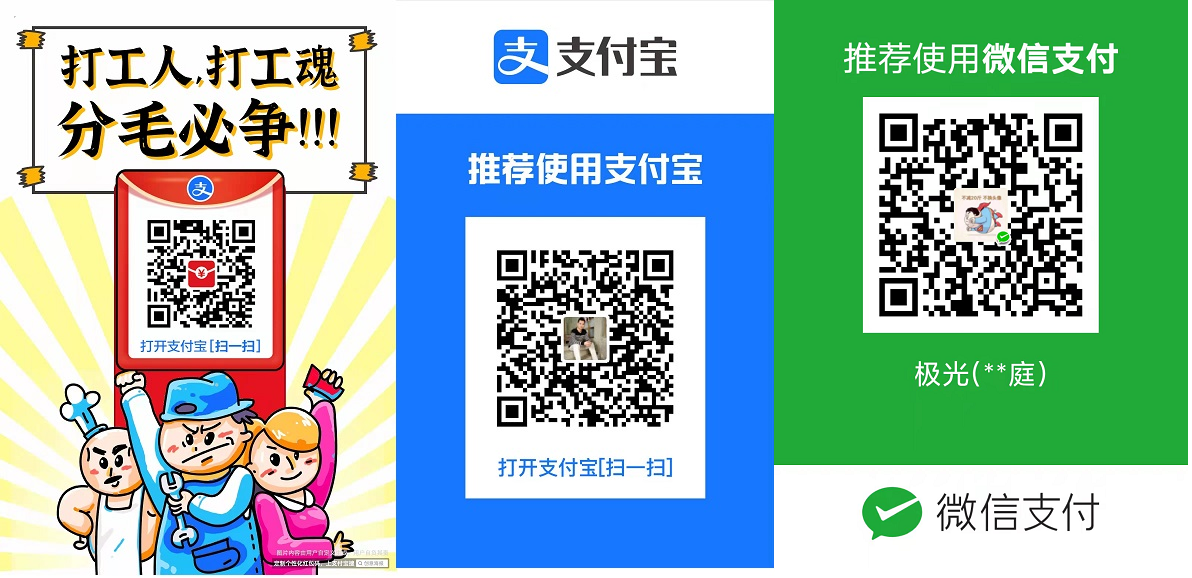