Modularizing your graphQL schemas
Modularizing your graphQL schemas
Working in a kinda big graphql server, schemas can easily get out of hand with all the types, inputs, queries, mutations, subscriptions and whatever else I’m missing. So there should be a way of separating them, modularizing them into smaller and more readable chunks right?
I found a way, and I wanna share it in case it can help you.
The Directory Structure
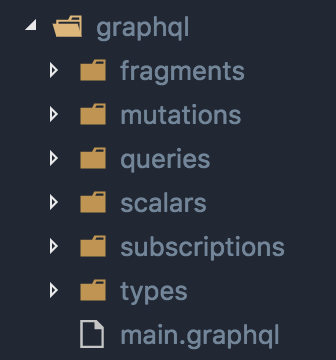
In this way you have a clear picture of the different type of graphQL files you have and where you should place new ones. I’m still not using Enums so that could be another category.
We’ll talk about the main.graphql
in a minute.
Mashing them together
To combine the different graphql files we will use the graphql-import npm package. This is developed by the guys doing Prisma. It lets you import other graphql files with its import
statement.
Let’s take this example type.
# import JSON from "../scalars/JSON.graphql"
# import JSON from "./Address.graphql"
"Represents a person"
type Person {
"The id by how you identify the person"
id: ID!
"The person's name"
name: String!
"A mock content field for the person, as JSON. For this article."
content: JSON!
"This person address"
address: Address!
}
- First we see that we are imporing the JSON scalar, this is a scalar provided by the graphql-type-json npm package. I added it as a colorful detail.
- Second, the same happens for a type but for Address.
You can image that the rest of the files will be pretty much the same, imports and usages in the schema.
So how do we create the final schema.graphql
that the server will consume??
The “main.graphql”
This file will, recursively, have all your queries, mutations, subscriptions and their used types.
# import Query.* from "./queries/queries.graphql"
# import Mutation.* from "./mutations/mutations.graphql"
# import Subscription from "./subscriptions/subscriptions.graphql"
That’s it. graphql-import
lets you use wildcards for consuming elements in the .graphql
. Check more options in their docs.
The usage is pretty much like this:
import { importSchema } from 'graphql-import'
import { makeExecutableSchema } from 'graphql-tools'
const typeDefs = importSchema('main.graphql');
const resolvers = {};
const schema = makeExecutableSchema({ typeDefs, resolvers });
// etc
Bonus Tracks
Webpack integration
You can use graphql-import-loader to let webpack manage the combining them.
Build the schema in a NPM Script
I need to generate this schema before publishing my npm package, so on the prepublishOnly
I run this:
const fs = require('fs');
const gqlImport = require('graphql-import');
const newSchema = gqlImport.importSchema('path/2/main.graphql');
fs.writeFileSync('path/2/new/generated/schema.graphql', newSchema);
Minified to get it in the script:
{
"scripts": {
"generateGqlSchema": "node -e \"const fs=require('fs'),i=require('graphql-import');fs.writeFileSync('src/main/resources/graphql/generated/schema.graphql',i.importSchema('src/main/resources/graphql/main.graphql'));\""
}
}
I will scale this more in the future so I’ll see how it stands the test of time, but for now it seems to work!
Let me know if you have an idea around it! Cheers!