Flutter 导航
Drawer 导航
Scaffold
drawer(左侧抽屉菜单)
endDrawer(右侧抽屉菜单)
UserAccountsDrawerHeader
抽屉菜单头部组件
AboutListTile
关于弹窗
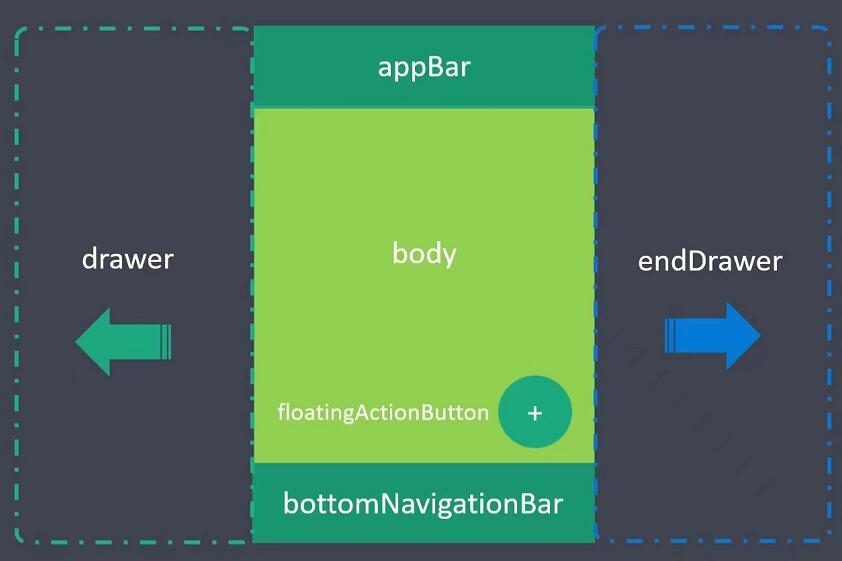
import 'package:flutter/material.dart'; class Home extends StatelessWidget { const Home({Key? key}) : super(key: key); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: const Text('Drawer 抽屉'), ), body: const HomePage(), // 左侧抽屉菜单 drawer: const DrawerList(), // 右侧抽屉菜单 endDrawer: const DrawerList(), ); } } class HomePage extends StatelessWidget { const HomePage({Key? key}) : super(key: key); @override Widget build(BuildContext context) { return Container( padding: const EdgeInsets.all(10), child: const Text('首页'), ); } } class DrawerList extends StatelessWidget { const DrawerList({Key? key}) : super(key: key); @override Widget build(BuildContext context) { // 创建抽屉组件 return Drawer( child: ListView( children: [ // 抽屉菜单头部组件 const UserAccountsDrawerHeader( // 当前账户名称 accountName: Text('史蒂夫 罗杰斯'), // 当前账户邮箱 accountEmail: Text('rogers@cap.com'), // 当前账户头像 currentAccountPicture: CircleAvatar( backgroundImage: AssetImage('assets/image4.jpg'), ), // 背景 decoration: BoxDecoration( image: DecorationImage( image: AssetImage('assets/image3.jpg'), fit: BoxFit.cover, ), ), ), const ListTile( leading: Icon(Icons.settings), title: Text('设置'), trailing: Icon(Icons.arrow_forward_ios), ), const Divider( thickness: 2, ), const ListTile( leading: Icon(Icons.account_balance), title: Text('余额'), trailing: Icon(Icons.arrow_forward_ios), ), const Divider( thickness: 2, ), const ListTile( leading: Icon(Icons.person), title: Text('我的'), trailing: Icon(Icons.arrow_forward_ios), ), const Divider( thickness: 2, ), ListTile( leading: const Icon(Icons.person), title: const Text('返回'), trailing: const Icon(Icons.arrow_forward_ios), onTap: () => Navigator.pop(context), ), // 关于弹窗 const AboutListTile( child: Text('关于'), applicationName: '应用名称', applicationVersion: '1.0.1', applicationLegalese: '法律条款', aboutBoxChildren: [ Text('条例一:xxxx'), Text('条例二:xxxx'), ], applicationIcon: FlutterLogo(), icon: CircleAvatar( child: Text('图片'), ), ), ], ), ); } }
BottomNavigationBar 导航
items:List<BottomNavigationBarItem>
包含导航(BottomNavigationBarItem)的列表
currentIndex:int,默认值 0
当前选中的导航索引
type:BottomNavigationBarType
导航类型(BottomNavigationBarType),取值 fixed (各导航等分宽度),shifting(点击时导航背景色会有动画效果)
onTap()
导航的点击事件(一般会更新导航索引)
import 'package:flutter/material.dart'; class Home extends StatefulWidget { const Home({Key? key}) : super(key: key); @override State<Home> createState() => _HomeState(); } class _HomeState extends State<Home> { // 底部导航菜单列表 final List<BottomNavigationBarItem> bottomNavItems = const [ BottomNavigationBarItem( icon: Icon(Icons.home), backgroundColor: Colors.blue, label: '首页', ), BottomNavigationBarItem( icon: Icon(Icons.message), backgroundColor: Colors.green, label: '消息', ), BottomNavigationBarItem( icon: Icon(Icons.shopping_cart), backgroundColor: Colors.amber, label: '购物车', ), BottomNavigationBarItem( icon: Icon(Icons.person), backgroundColor: Colors.red, label: '我的', ), ]; // 页面 final List<Center> pages = const [ Center( child: Text('Home', style: TextStyle(fontSize: 50)), ), Center( child: Text('Message', style: TextStyle(fontSize: 50)), ), Center( child: Text('Cart', style: TextStyle(fontSize: 50)), ), Center( child: Text('Profile', style: TextStyle(fontSize: 50)), ), ]; // 选中的导航下标 int currentIndex = 0; // 切换导航 void _changePage(int index) { if (index != currentIndex) { setState(() { currentIndex = index; }); } } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: const Text('底部导航'), ), // 底部导航 bottomNavigationBar: BottomNavigationBar( items: bottomNavItems, currentIndex: currentIndex, // 导航类型:效果如下图所示 type: BottomNavigationBarType.fixed, // type: BottomNavigationBarType.shifting, onTap: (index) { _changePage(index); }, ), body: pages[currentIndex], ); } }
type: BottomNavigationBarType.fixed 效果:
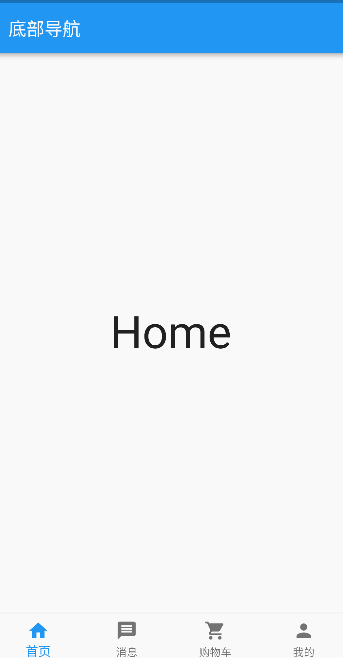
type: BottomNavigationBarType.shifting 效果:
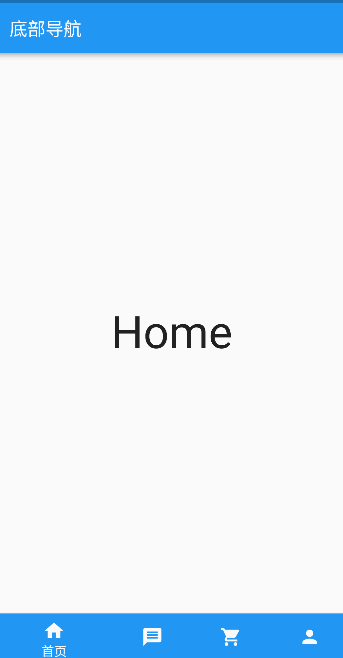
Tab 导航
DefaultTabController:整个 Tab 导航的容器
length:声明导航数量
child:指定子组件
TabBar:导航菜单
tabs:导航菜单数组
TabBarView:导航页面
children:多个导航页面内容
import 'package:flutter/material.dart'; class Home extends StatelessWidget { const Home({Key? key}) : super(key: key); // 菜单数组 final List<Widget> _tabs = const [ Tab(text: '首页', icon: Icon(Icons.home)), Tab(text: '添加', icon: Icon(Icons.add)), Tab(text: '搜索', icon: Icon(Icons.search)), ]; // 页面数组 final List<Widget> _tabViews = const [ Icon(Icons.home, size: 120, color: Colors.red), Icon(Icons.add, size: 120, color: Colors.green), Icon(Icons.search, size: 120, color: Colors.amber), ]; @override Widget build(BuildContext context) { // Tab导航的容器 return DefaultTabController( // 导航的总个数 length: _tabs.length, child: Scaffold( appBar: AppBar( title: const Text('Tab 导航'), // 展示在 appbar 底部的导航 bottom: TabBar( tabs: _tabs, // 点击时的水波纹效果 overlayColor: MaterialStateProperty.all( Colors.red, ), // 导航高亮的颜色 labelColor: Colors.yellow, // 未选中导航的颜色 unselectedLabelColor: Colors.grey, // 导航高亮的线条 indicatorColor: Colors.pink, // 线条的长度 tab:跟导航同宽,label:跟文本同宽 indicatorSize: TabBarIndicatorSize.tab, // 线条的高度 indicatorWeight: 10, ), ), body: TabBarView(children: _tabViews), // 底部导航 bottomNavigationBar: TabBar( tabs: _tabs, // 导航高亮的文本颜色 labelColor: Colors.blue, // 未选中导航的文本颜色 unselectedLabelColor: Colors.grey, ), ), ); } }