Flutter 常用的特殊组件
1、Cupertino
Cupertino -- iOS风格的组件
引用:import 'package:flutter/cupertino.dart';
文档:https://flutter.cn/docs/development/ui/widgets/cupertino
Material -- 安卓风格的组件
引用:import 'package:flutter/material.dart';
import 'package:flutter/cupertino.dart'; import 'package:flutter/material.dart'; import 'dart:io'; class Home extends StatelessWidget { const Home({Key? key}) : super(key: key); @override Widget build(BuildContext context) { Widget dialogBox = Container(); // 判断当前的平台信息 // iOS if (Platform.isIOS) { dialogBox = const CupertinoDialog(); } // Android if (Platform.isAndroid) { dialogBox = const MaterialDialog(); } return Scaffold( appBar: AppBar( title: const Text('Dialog'), ), body: dialogBox, ); } } // 安卓风格的弹窗 class MaterialDialog extends StatelessWidget { const MaterialDialog({Key? key}) : super(key: key); @override Widget build(BuildContext context) { return Container( padding: const EdgeInsets.symmetric(horizontal: 10), child: AlertDialog( title: const Text('提示'), content: const Text('确认删除吗?'), actions: [ TextButton(onPressed: () {}, child: const Text('取消')), TextButton(onPressed: () {}, child: const Text('确认')), ], ), ); } } // iOS风格的弹窗 class CupertinoDialog extends StatelessWidget { const CupertinoDialog({Key? key}) : super(key: key); @override Widget build(BuildContext context) { return Container( padding: const EdgeInsets.symmetric(horizontal: 10), child: CupertinoAlertDialog( title: const Text('提示'), content: const Text('确认删除吗?'), actions: [ CupertinoDialogAction( child: const Text('取消'), onPressed: () {}, ), CupertinoDialogAction( child: const Text('确认'), onPressed: () {}, ), ], ), ); } }
2、SafeArea
该组件会为其子组件插入足够的内边距以避免操作系统的影响,例如:iPhone X 的刘海屏
文档:https://api.flutter-io.cn/flutter/widgets/SafeArea-class.html
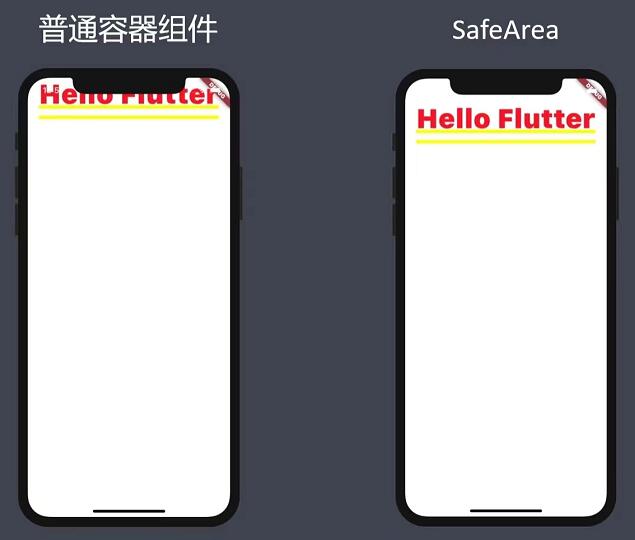
3、dio
dio是一个强大的Dart Http请求库,支持Restful API、FormData、拦截器、请求取消、Cookie管理、文件上传/下载、超时、自定义适配器等...
pub地址:https://pub.dev/packages/dio
中文文档:https://github.com/flutterchina/dio/blob/master/README-ZH.md
安装:flutter pub add dio
引入:import 'package:dio/dio.dart';
// ignore_for_file: avoid_print import 'package:flutter/material.dart'; import 'package:dio/dio.dart'; class Home extends StatelessWidget { const Home({Key? key}) : super(key: key); // 异步获取图片 void getPic() async { try { var response = await Dio().get('https://dog.ceo/api/breed/hound/images/random'); print(response); print(response.data); print(response.data['message']); print(response.data['status']); } catch (e) { print(e); } } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: const Text('dio'), ), body: Container( width: double.infinity, padding: const EdgeInsets.all(10), child: ElevatedButton( // 点击事件,调用 HTTP 请求 onPressed: getPic, child: const Text('点击发送请求'), ), ), ); } }
4、flutter_swiper
Flutter 中最好的轮播组件,支持多种布局、无限滚动,可同时兼容 Android 和 iOS
文档:https://pub.flutter-io.cn/packages/flutter_swiper
安装:flutter pub add flutter_swiper
使用:import 'package:flutter_swiper/flutter_swiper.dart';
基本属性:
scrollDirection:默认值 Axis.horizontal,轮播的方向
loop:默认值 true,取值为 false 时,禁止无限滚动
index:默认值 0,初始的下标
autoplay:默认值 false,取值为 true 时,启动自动轮播
duration:默认值 3000ms,每次动画(轮播切换)耗时
分页器 Pagination 属性:
继承自 SwiperPlugin,为 Swiper 提供了额外的 UI,通过 new SwiperPagination() 来创建 pagination
alignment:默认值 Alignment.bottomCenter,分页器的位置
margin:默认值 EdgeInsets.all(10.0),与父容器的间距
builder:默认值 SwiperPagination.dots,两种不同的样式 SwiperPagination.dots 和 SwiperPagination.fraction
import 'package:flutter/material.dart'; import 'package:flutter_swiper/flutter_swiper.dart'; class Home extends StatelessWidget { const Home({ Key? key, this.imgs = const [ 'assets/image1.jpg', 'assets/image2.jpg', 'assets/image3.jpg' ], }) : super(key: key); final List<String> imgs; @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: const Text('轮播图'), ), body: ListView( children: [ const Text( '轮播图基本用法:', style: TextStyle( fontSize: 32, ), textAlign: TextAlign.left, ), Container( padding: const EdgeInsets.symmetric(horizontal: 10), height: 150, child: Swiper( autoplay: true, itemCount: imgs.length, itemBuilder: (context, index) { return Image.asset( imgs[index], fit: BoxFit.cover, ); }, // 默认的分页器(轮播图的指示点) pagination: const SwiperPagination(), // 默认的控制器(左右箭头导航) control: const SwiperControl(), ), ), const Text( '轮播图3D效果:', style: TextStyle( fontSize: 32, ), textAlign: TextAlign.left, ), Container( padding: const EdgeInsets.symmetric(horizontal: 10), height: 150, child: Swiper( autoplay: true, itemCount: imgs.length, itemBuilder: (context, index) { return Image.asset( imgs[index], fit: BoxFit.cover, ); }, // 主轮播图的缩放比例,默认是1 viewportFraction: 0.7, // 非主轮播图的缩放比例 scale: 0.7, ), ), const Text( '轮播图布局:', style: TextStyle( fontSize: 32, ), textAlign: TextAlign.left, ), Container( padding: const EdgeInsets.symmetric(horizontal: 10), height: 150, child: Swiper( autoplay: true, itemCount: imgs.length, itemBuilder: (context, index) { return Image.asset( imgs[index], fit: BoxFit.cover, ); }, itemWidth: 300, // 当 layout 取值为 TINDER 时,必须指明高度,否则会报错 // itemHeight: 150, // layout: SwiperLayout.TINDER, layout: SwiperLayout.STACK, ), ), ], ), ); } }
5、shared_preferences
是一个异步的本地数据缓存库,支持的数据类型有:int, double, bool, String, List<String>
文档:https://pub.flutter-io.cn/packages/shared_preferences
安装:flutter pub add shared_preferences
引用:import 'package:shared_preferences/shared_preferences.dart';
使用:SharedPreferences prefs = await SharedPreferences.getInstance();
增/改:setString(key, value)
查:getString(key)
删:remove(key), clear()
// ignore_for_file: avoid_print import 'package:flutter/material.dart'; import 'package:shared_preferences/shared_preferences.dart'; class Home extends StatelessWidget { const Home({Key? key}) : super(key: key); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: const Text('shared_preferences'), ), body: Container( width: double.infinity, padding: const EdgeInsets.all(10), child: Column( mainAxisAlignment: MainAxisAlignment.spaceAround, crossAxisAlignment: CrossAxisAlignment.center, children: [ ElevatedButton( onPressed: _incrementCounter, child: const Text('递增'), ), ElevatedButton( onPressed: _decrementCounter, child: const Text('递减'), ), ElevatedButton( onPressed: _removeCounter, child: const Text('删除'), ), ElevatedButton( onPressed: _addStr, child: const Text('缓存字符串'), style: ButtonStyle( backgroundColor: MaterialStateProperty.all(Colors.pink), ), ), ElevatedButton( onPressed: _getStr, child: const Text('读取字符串'), style: ButtonStyle( backgroundColor: MaterialStateProperty.all(Colors.pink), ), ), ElevatedButton( onPressed: _clearPrefs, child: const Text('清空'), style: ButtonStyle( backgroundColor: MaterialStateProperty.all(Colors.pink), ), ), ], ), ), ); } // 递增 _incrementCounter() async { // 获取保存实例 SharedPreferences prefs = await SharedPreferences.getInstance(); // 需要注意运算的优先级 + 先于 ?? 执行 int counter = (prefs.getInt('counter') ?? 0) + 1; print('Pressed $counter times.'); await prefs.setInt('counter', counter); } // 递减 _decrementCounter() async { // 获取保存实例 SharedPreferences prefs = await SharedPreferences.getInstance(); int counter = prefs.getInt('counter') ?? 0; if (counter > 0) { counter--; } print('Pressed $counter times.'); await prefs.setInt('counter', counter); } // 删除 _removeCounter() async { SharedPreferences prefs = await SharedPreferences.getInstance(); await prefs.remove('counter'); } // 缓存字符串 _addStr() async { SharedPreferences prefs = await SharedPreferences.getInstance(); await prefs.setString('msg', 'Hello World !'); } // 读取缓存 _getStr() async { SharedPreferences prefs = await SharedPreferences.getInstance(); String msg = prefs.getString('msg') ?? ''; print('缓存的字符串内容是:$msg'); } // 清空所有缓存内容 _clearPrefs() async { SharedPreferences prefs = await SharedPreferences.getInstance(); prefs.clear(); } }