[Java] 接口-01
接口
*1 接口(interface)是抽象方法和常量值的定义的集合
*2 从本质上讲,接口是一种特殊的抽象类,这种抽象类只包含常量和方法的定义,而没有变量和方法的实现。
*3 接口定义举例 :
*1 接口可以多重实现
*2 接口中声明的属性默认为 public static final 的; 也只能是 public static final的;
*3 接口中只能定义抽象方法, 而且这些方法默认为public的,也只能是public的。
*4 接口可以继承其他的接口,并添加新的属性和抽象方法。
*5 多个无关的类可以实现同一个接口
*6 一个类可以实现多个无关的接口
*7 与继承关系类似,接口与实现类之间存在多态性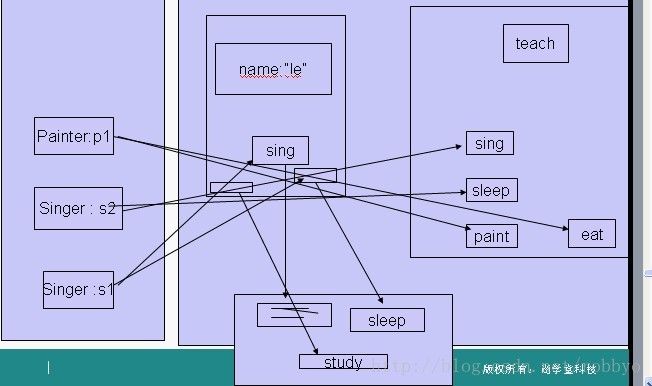
*1 接口(interface)是抽象方法和常量值的定义的集合
*2 从本质上讲,接口是一种特殊的抽象类,这种抽象类只包含常量和方法的定义,而没有变量和方法的实现。
*3 接口定义举例 :
public interface Runner { //public static final int id = 1; int id = 1; public void start(); public void run(); void stop(); // public void stop(); }接口特性
*1 接口可以多重实现
*2 接口中声明的属性默认为 public static final 的; 也只能是 public static final的;
*3 接口中只能定义抽象方法, 而且这些方法默认为public的,也只能是public的。
*4 接口可以继承其他的接口,并添加新的属性和抽象方法。
*5 多个无关的类可以实现同一个接口
*6 一个类可以实现多个无关的接口
*7 与继承关系类似,接口与实现类之间存在多态性
package com.bjsxt.chap03; public interface Singer { public void sing(); public void sleep(); } interface Painter { // 默认 public 不写也是 public void paint(); void eat(); // 默认,不写也是 public , 就是 public } class Student implements Singer { private String name; Student(String name) { this.name = name; } public void study() { System.out.println("studying"); } public String getName() { return name; } public void sing() { System.out.println("Student is singing"); } public void sleep() { System.out.println("Student is Sleeping"); } } class Teacher implements Singer, Painter { private String name; public String getName() { return name; } Teacher(String name) { this.name = name; } public void teach() { System.out.println("Teaching"); } public void sing() { System.out.println("Teacher is Singing"); } public void sleep() { System.out.println("Teacher is Sleeping"); } public void paint() { System.out.println("Teacher is Painting"); } public void eat() { System.out.println("Teacher is Eating"); } }
package com.bjsxt.chap03; public class TestInterface { public static void main(String[] args) { Singer s1 = new Student("le"); s1.sing(); s1.sleep(); Singer s2 = new Teacher("steven"); s2.sing(); s2.sleep(); Painter p1 = (Painter)s2; p1.paint(); p1.eat(); } }