线程的死锁问题
- 死锁
- 不同的线程分别占用对方需要的同步资源不放弃,都在等对方放弃自己需要的同步资源,就形成了线程的死锁
- 出现死锁后,不会出现异常,不会出现提示,只是所有的线程都处于阻塞状态,无法继续
- 解决方法
- 专门的算、原则
- 尽量减少同步资源的定义
- 尽量避免嵌套同步
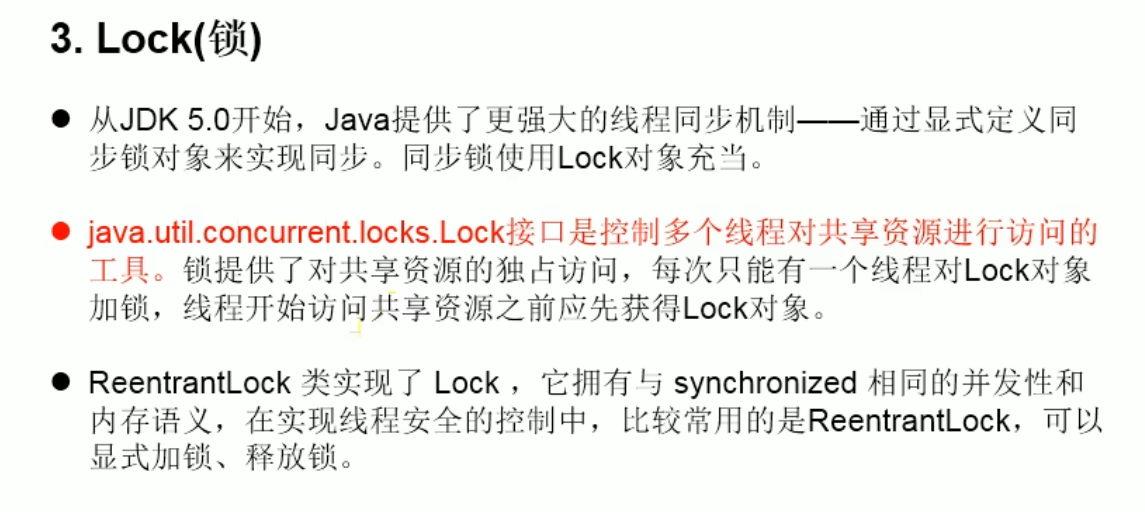
/*
1.面试题:synchronized 与 lock的异同
同:二者都可以解决线程安全问题
不同:synchronized机制在执行完相应的同步代码以后,自动的释放同步监视器
Lock需要手动的启动同步(lock(0)),同时结束同步也需要手动的实现(unlock())
2.优先使用顺序
Lock->同步代码块(已经进入方法体,分配了相应资源)->同步方法(在方法体外)
3.面试题:如何解决线程安全问题?有几种方式
*/
public class WindowTest5 {
public static void main(String[] args) {
Window5 w = new Window5();
Thread t1 = new Thread(w);
Thread t2 = new Thread(w);
Thread t3 = new Thread(w);
t1.start();
t2.start();
t3.start();
}
}
class Window5 implements Runnable{
private int ticket = 100;
//1.实例化ReentrantLock
private ReentrantLock lock = new ReentrantLock();
@Override
public void run() {
while (true){
try {
//调用锁定方法
lock.lock();
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
if (ticket>0){
System.out.println(Thread.currentThread().getName()+"卖出第"+ticket+"张票");
ticket--;
}else {
break;
}
} finally {
//调用解锁方法
lock.unlock();
}
}
}
}
线程的通信
/*
线程通信例子:使用两个线程打印1-100,线程1,线程2,交替打印
涉及到的三个方法:
wait():一旦执行此方法,当前线程就进入阻塞状态,并释放同步监视器。
notify():一旦执行此方法,就会唤醒wait的一个线程,如果多个线程被wait,就唤醒优先级高的那一个
notifyAll():一旦执行此方法:就会唤醒所有被wait的线程
说明:
1. wait(),notify(),notifyAll()三个方法必须使用在同步代码块或同步方法中。
2. wait(),notify(),notifyAll()三个方法的调用者必须是同步代码块或同步方法中的同步监视器
3. wait(),notify(),notifyAll()三个方法定义在java.lang.Object类中
sleep和wait方法的异同:
1.相同点:一旦执行方法,都可是当前线程处于阻塞状态
2.不同点:1)两个方法声明的位置不同,Thread类中声明sleep(),Object类中声明wait()
2)调用要求不同:sleep()可以在任何需要的场景下调用。wait()必须使用在同步代码块或同步方法中。
3)关于是否释放同步监视器,如果两个方法都是用在同步代码块或同步方法之中,sleep()不会释放同步监视器,wait()会释放同步监视器
*/
public class CommunicationTest {
public static void main(String[] args) {
Number n = new Number();
Thread t1 = new Thread(n);
Thread t2 = new Thread(n);
t1.start();
t2.start();
}
}
class Number implements Runnable{
private int number = 1;
@Override
public void run() {
while (true){
synchronized (this) {
notify();
if (number<=100){
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println(Thread.currentThread().getName()+":"+number);
number++;
}else{
break;
}
try {
//使得调用wait方法的线程进入阻塞状态
wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
}
/*
线程通信的应用:经典例题:生产者/消费者问题
生产者(Productor)将产品交给店员(Clerk),而消费者(Customer)
从店员处取走产品,电源一次只能持有固定数量的产品(比如:20
),如果生产者试图生产更多的产品,店员会叫生产者停一下,如果店中有空位放产品了在通知生产者继续生产;如果店中没有产品了,店员会告诉消费者等一下,如果店中有产品了在通知消费者来取走产品
分析:
1.是否是多线程问题?是,生产者线程,消费者线程
2.是否有共享数据?是,店员(产品)
3.如何解决线程安全问题?同步机制,有三种方法
4.是否涉及线程通信?是
*/
class Clerk{
private int productCount =0;
//生产产品
public synchronized void produceProduct() {
if (productCount<20){
productCount++;
System.out.println(Thread.currentThread().getName()+":开始生产第"+productCount+"产品");
notify();
}else {
try {
wait();//等待
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
//消费产品
public synchronized void consumeProduct() {
if (productCount>0){
System.out.println(Thread.currentThread().getName()+":开始消费第"+productCount+"产品");
productCount--;
notify();
}else {
try {
wait(); //等待
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
class Producer extends Thread{//生产者
private Clerk clerk;
public Producer(Clerk clerk){
this.clerk = clerk;
}
@Override
public void run() {
System.out.println(getName()+":开始生产商品");
while (true){
try {
Thread.sleep(10);
} catch (InterruptedException e) {
e.printStackTrace();
}
clerk.produceProduct();
}
}
}
class Consumer extends Thread{//消费者
private Clerk clerk;
public Consumer(Clerk clerk) {
this.clerk = clerk;
}
@Override
public void run() {
System.out.println(getName()+":开始消费产品");
while (true){
try {
Thread.sleep(10);
} catch (InterruptedException e) {
e.printStackTrace();
}
clerk.consumeProduct();
}
}
}
public class ProductTest {
public static void main(String[] args) {
Clerk clerk = new Clerk();
Producer p1 = new Producer(clerk);
p1.setName("生产者1");
Consumer c1 = new Consumer(clerk);
c1.setName("消费者1");
p1.start();
c1.start();
}
}