leetcode 261 以图判树
题目
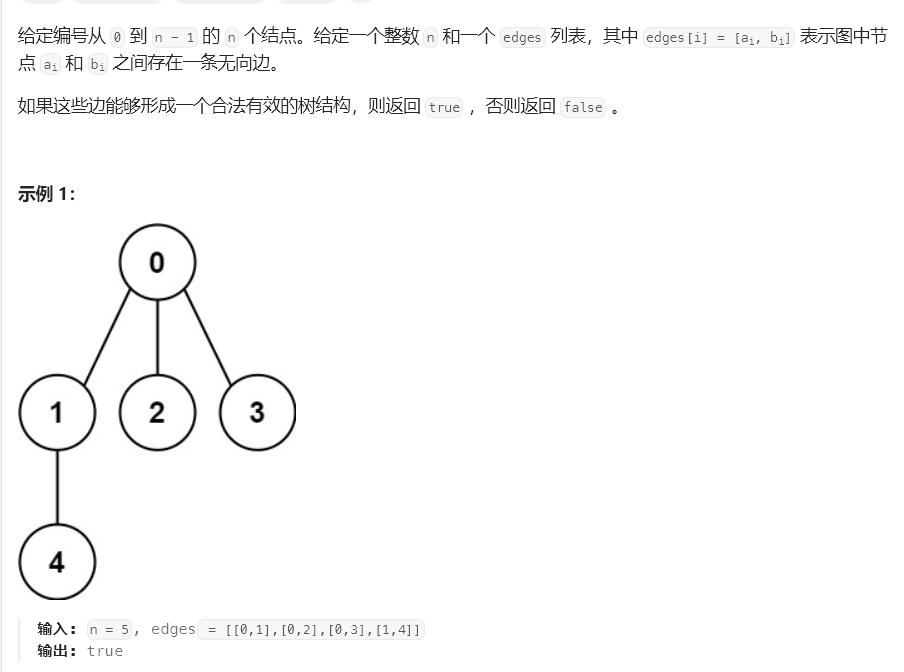
并查集
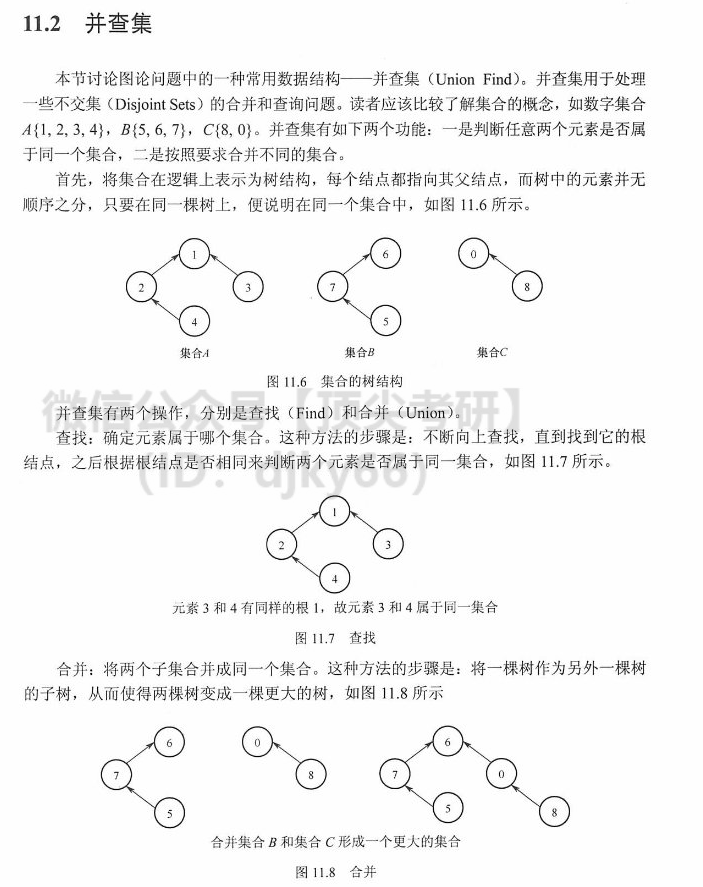
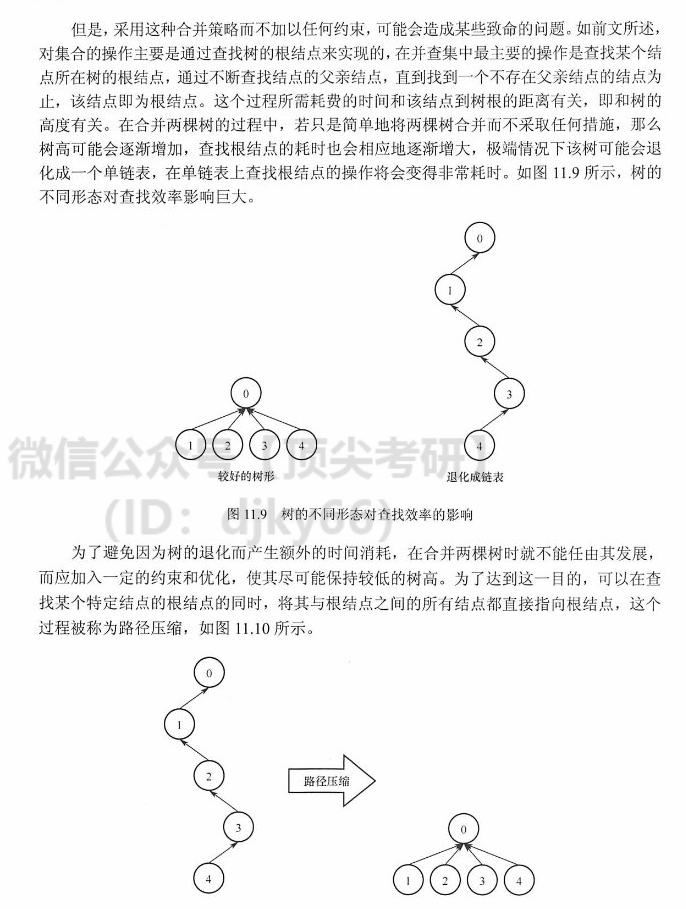
模板
class UF {
public:
UF(int size) {
father.resize(size);
for (int i = 0; i < size; ++i) {
father[i] = i;
}
}
int find (int x) {
return x == father[x] ? x : father[x] = find(father[x]);
}
void connect (int x, int y) {
int xx = find(x);
int yy = find(y);
if (xx == yy) { return; }
if (xx < yy) {
father[yy] = xx;
} else {
father[xx] = yy;
}
}
bool same(int x, int y) {
return find(x) == find(y);
}
private:
vector<int> father;
};
代码
class Solution {
public:
bool validTree(int n, vector<vector<int>>& edges) {
UF uf(n);
if (edges.size() != n-1) {
return false;
}
for (auto& e : edges) {
int v1 = e[0], v2 = e[1];
int v1_par = uf.find(v1);
int v2_par = uf.find(v2);
if (v1_par == v2_par) {
return false;
}
uf.connect(v1, v2);
}
return true;
}
};