JSP
1.目前的问题
目前 Servlet 需要将数据发送给浏览器,需要在后台进行 HTML 页面的数据拼接。超级麻烦!!!超级恶心!!!
1. HTML + Java 代码混合很麻烦!!!
2. 字符串拼接想报警!!!
3. 不管是针对于 Java 不友好,针对于 HTML 不友好!!!
2. JSP 概述
JSP ==> JavaServer Page Java 服务器页面
是一门比较古老的技术,目前项目开发中使用的很少,学习 JSP 是为了更好的理解项目中的分层结构,数据处理,数据展示模式,数据传递模式和域对象特征
JSP支持 HTML,CSS,JS 和 Java。期望的 JSP 页面中不再出现 Java 代码,完全是前端页面布局设计控制和后端提交数据解析控制。
JSP ==> View 视图/显示
Servlet ==> Controller 控制
Service ==> 服务器
DAO ==> 数据持久化
3. 第一个 JSP 代码
<%--
Created by IntelliJ IDEA.
User: Anonymous
Date: 2022/4/20
Time: 9:02
To change this template use File | Settings | File Templates.
--%>
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>$Title$</title>
</head>
<body>
$END$
</body>
</html>
JSP注意事项
1. JSP 页面无法通过该浏览器直接打开,浏览器无法解析 JSP 内容
2. JSP 页面需要 Tomcat 服务器解析之后,转换成浏览器可以识别的 HTML 文件,浏览器才可以解析对应页面
3. JSP 页面实际上是一个 简化版的 Servlet 程序
Tomcat 解析 index.jsp 之后 ==> class index_jsp extends HttpServlet
index.jsp ==> Tomcat解析 ==>
index_jsp.java ==> 编译 ==>
index_jsp.class ==> 服务器加载 ==> 提供服务
所有的 HTML 内容和 Java 代码相关数据都是拼接 HTML 页面之后发送给浏览器
4. 浏览器想要获取对应的 jsp 页面,需要启动服务器之后,
index.jsp ==》 localhost:8080/Day43/index.jsp
tips:
Tomcat 默认配置
index.html index.jsp
用户如果默认申请资源是项目根目录 /Day43 ==> 自动跳转主页操作
【目标】JSP 页面中,没有任何一个 Java 代码
4. JSP 语法
4.1 JSP 脚本
<%--
Created by IntelliJ IDEA.
User: Anonymous
Date: 2022/4/20
Time: 11:34
To change this template use File | Settings | File Templates.
--%>
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>01-JSP脚本</title>
</head>
<body>
<%-- JSP 注释--%>
<!-- HTML 注释 -->
<%-- JSP 脚本 --%>
<%
out.write("<h1>JSP 页面</h1>");
System.out.println("Java 控制台输出");
%>
<%-- JSP 脚本声明变量 方法--%>
<%!int i = 0;%>
<%!int n = 10;%>
<%!
public void method() {
System.out.println("JSP 声明方法");
}
%>
<%
out.write("<h1>" + i + "</h1>");
out.write("<h1>" + n + "</h1>");
method();
%>
<%-- JSP 输出脚本--%>
<h1><%=10%>
</h1>
<h1><%=i%>
</h1>
<h1><%=10 > 5%>
</h1>
</body>
</html>
4.2 JSP 指令
4.2.1 Page 指令
<
里面可以定义 JSP 指令
<
属性 |
描述 |
contentType |
指定 JSP 页面最终解析文件类型和编码集 |
language |
解析 JSP 页面使用的语言 |
isErrorPage |
当前 JSP 页面是否为错误页面,默认为false,如果设置为 true 当前页面中可以使用 内置对象 exception,来处理页面异常,例如 404 500 |
errorPage |
当前 JSP 页面如果出现的错误,跳转到哪一个错误页面进行数据处理,用户提示,要求对应页面最好 isErrorPage 设置为 true |
session |
设置当前 JSP 页面是否可以使用 Session 对象,默认为 true ,如果设置为 false ,当前页面不可以获取 Session 对象 |
pageEncoding |
告知 Tomcat 读取解析当前 JSP 页面采用的编码情况 |
import |
可以导包 |
4.2.2 include 指令
<%--
Created by IntelliJ IDEA.
User: Anonymous
Date: 2022/4/20
Time: 14:49
To change this template use File | Settings | File Templates.
--%>
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>主页</title>
</head>
<body>
<%@include file="03-top.jsp"%>
<h1>主页内容</h1>
<%@include file="03-footer.jsp"%>
</body>
</html>
4.2.3 taglib
可以引入其他 JSP 标签库 ==> 就是用来解决 JSP 页面 Java 代码替换问题
利用 taglib 指令包含 【JSTL】标签库
<%-- 引入 JSTL 标签库,使用 JSTL 标签替换一部分 Java 代码 --%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%>
4.3 JSP 内置对象【重点】
JSP文件 ==> Tomcat 解析 ==> Java 文件 ==> 编译之后 ==> .class 文件 ==> Tomcat 加载执行
test.jsp ==> Tomcat 解析
JSP 文件转换为一个 Java 文件,同时是一个 Servlet 程序,在转换过程中,给予 JSP 操作【九个 JavaWEB】相关数据的对象。【内置对象】 JSP 可以直接使用
test_jsp.java
内置对象 |
具体类型 |
功能描述 |
request |
javax.servlet.http.HttpServletRequest |
用户请求对应的 Request 对象 |
response |
javax.servlet.http.HttpServletResponse |
用户请求对应的响应 Response 对象 |
session |
javax.servlet.http.HttpSession |
Session 对象 JSP page 指令 session="true" 可以使用 |
application |
javax.servlet.ServletContext |
当前整个 Java Web Application 对象,对应当前项目 |
config |
javax.servlet.ServletConfig |
对应 Servlet 配置对象 |
exception |
java.lang.Throwable |
异常对象 JSP page 指令 isErrorPage="true" 可以使用 |
out |
javax.servlet.jsp.JspWriter |
PrintWriter resp.getWriter(); 对 HTML 页面输出内容 |
pageContext |
javax.servlet.jsp.PageContext |
当前 JSP 页面内部对象,可以获取其他内置对象 |
page |
当前 JSP 页面 ==> this 关键字 |
当前 JSP ==> Servlet 程序本身 |
<%--
Created by IntelliJ IDEA.
User: Anonymous
Date: 2022/4/20
Time: 15:14
To change this template use File | Settings | File Templates.
--%>
<%@ page contentType="text/html;charset=UTF-8" language="java" isErrorPage="true" %>
<html>
<head>
<title>04-内置对象</title>
</head>
<body>
<h3>Request : <%=request%></h3>
<h3>Response : <%=response%></h3>
<h3>HttpSession : <%=session%></h3>
<h3>Java Web Application : <%=application%></h3>
<h3>JSP 页面内置对象 : <%=pageContext%></h3>
<h3>Throwable : <%=exception%></h3>
<h3>JspWriter : <%=out%></h3>
<h3>this : <%=page%></h3>
<h3>ServletConfig : <%=config%></h3>
<hr>
<%-- 可以通过 pageContext 获取当前 JSP 其他任意内置对象 --%>
<h3><%=pageContext.getRequest()%></h3>
<h3><%=pageContext.getResponse()%></h3>
<h3><%=pageContext.getServletContext()%></h3>
<h3><%=pageContext.getSession()%></h3>
<h3><%=pageContext.getPage()%></h3>
<h3><%=pageContext.getOut()%></h3>
<h3><%=pageContext.getServletConfig()%></h3>
<h3><%=pageContext.getException()%></h3>
<hr>
<h3>可以通过该 Request 对象获取用户请求参数:<%=request.getParameter("name")%></h3>
</body>
</html>
4.4 域对象【重点】
4.4.1 Request 和 Session 域对象
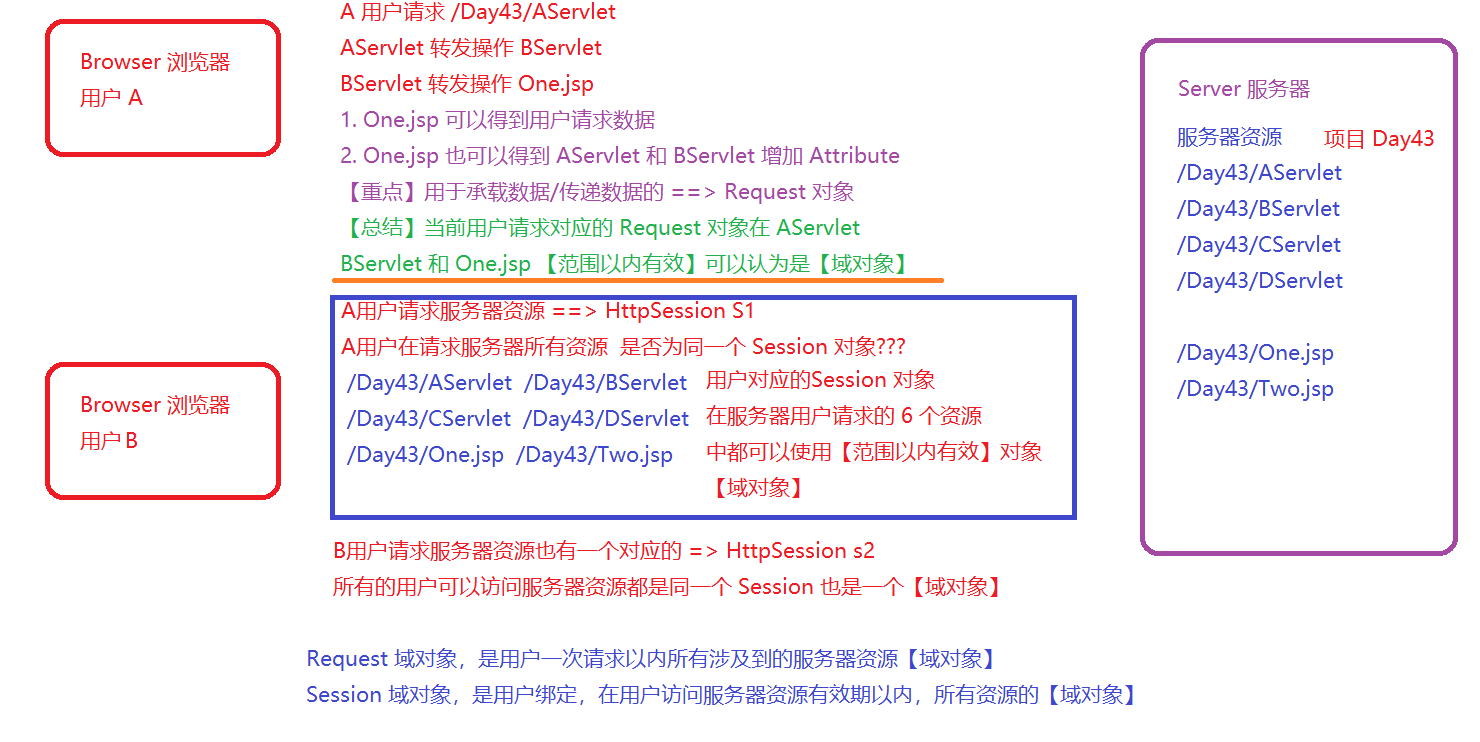
4.4.2 Application 和 pageContext
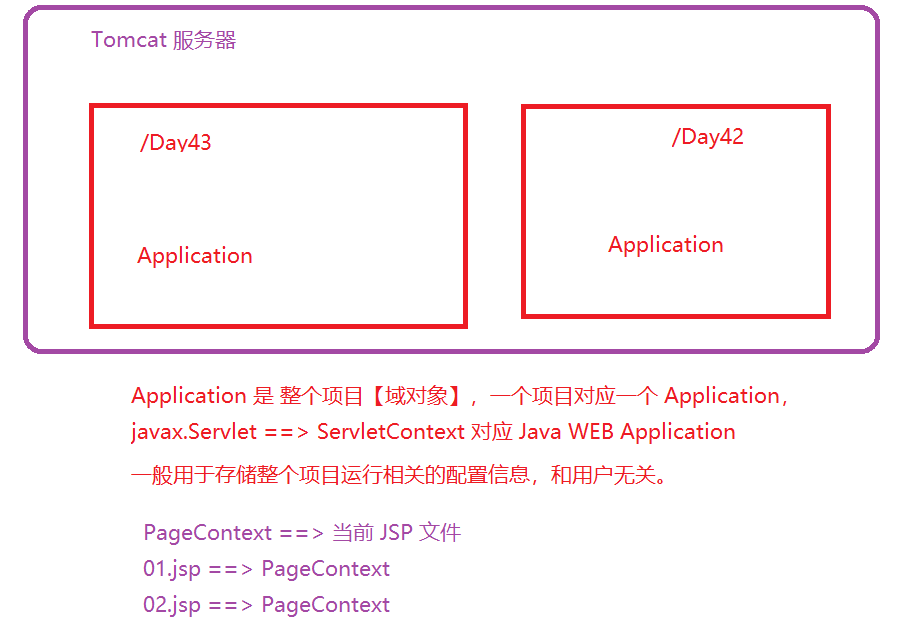
4.4.3 域对象代码演示
<%--
Created by IntelliJ IDEA.
User: Anonymous
Date: 2022/4/20
Time: 16:45
To change this template use File | Settings | File Templates.
--%>
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>05-域对象03</title>
</head>
<body>
<%
application.setAttribute("msg", "3 Application Message");
%>
<%--
EL 表达式,可以在域对象 Attribute 属性中获取指定名称数据
可以获取 四个域对象 request session application pageContext
4 PageContext Message
1 Request Message
2 Session Message
3 Application Message
问题:
1. 域对象 同名属性获取获取数据优先级???
就近原则,PageContext > Request > Session > Application
2. 为什么 Session 代码注释了,依然可以获取 Session 数据???
因为用户请求服务器之后,HttpSession 对象存在,注释仅取消了对 Session Attribute 数据属性赋值操作
并没有销毁Session ,所以依然获取的是 Session 对应数据
1. Session 销毁
2. 浏览器清除 Cookie
--%>
<h1>${msg}</h1>
</body>
</html>
5. EL 表达式【重点】
5.1 概述
主要用于
1. 简化/优化 JSP 代码方式。
2. 替换 Java 代码!!!
3. EL 表达式是直接可以从 【域对象】中获取attribute数据,获取数据的顺序
pageContext > Request > Session > Application
常用域对象 request 和 session 并且在实际使用过程中,通常会避免数据属性名称一致的情况
EL 表达式
<h1>${msg}</h1>
==>
<h1><%=request.getAttribute("msg")%></h1>
如果 request 没有 属性 msg
<h1>${msg}</h1> ==> 空字符串,前端页面没有任何的数据显示
<h1><%=request.getAttribute("msg")%></h1> null
5.2 EL基本语法特征演示
<%--
Created by IntelliJ IDEA.
User: Anonymous
Date: 2022/4/20
Time: 17:11
To change this template use File | Settings | File Templates.
--%>
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>01-el</title>
</head>
<body>
<%
String str = "字符串";
request.setAttribute("str", str);
%>
<%--
EL 表达式 只可以从域对象属性中获取数据
--%>
<h1>EL表达式 : ${str}</h1>
<h1>JSP输出语句 : <%=request.getAttribute("str")%></h1>
</body>
</html>
5.2 EL表达式解析Java常见类型
5.2.1 JavaBean 对象
<%@ page import="com.qfedu.a_jsp.Student" %><%--
Created by IntelliJ IDEA.
User: Anonymous
Date: 2022/4/20
Time: 17:21
To change this template use File | Settings | File Templates.
--%>
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>02-el</title>
<style>
td {
text-align: center;
}
</style>
</head>
<body>
<%
Student student = new Student(1, "王乾", 80);
request.setAttribute("stu", student);
%>
<table width="200px" border="1" align="center">
<tr>
<th colspan="2">学生信息</th>
</tr>
<tr>
<td>ID</td>
<%--
可以解析 Java 对象 stu.id ==> stu.getId()
EL 表达式 ${stu.id}
==>
JSP 输出语句 <%=request.getAttribute("stu").getId()%>
--%>
<td>${stu.id}</td>
</tr>
<tr>
<td>Name</td>
<td>${stu.name}</td>
</tr>
<tr>
<td>Age</td>
<td>${stu.age}</td>
</tr>
</table>
</body>
</html>
5.2.2 数组
<%--
Created by IntelliJ IDEA.
User: Anonymous
Date: 2022/4/20
Time: 17:28
To change this template use File | Settings | File Templates.
--%>
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>03-el</title>
<style>
li {
list-style: none;
}
</style>
</head>
<body>
<%
int[] arr = {1, 3, 5, 7, 9, 2, 4, 6, 8, 10};
request.setAttribute("arr", arr);
%>
<ul>
<%-- 属性为数组类型,可以直接使用下标操作 --%>
<li>${arr[0]}</li>
<li>${arr[1]}</li>
<li>${arr[2]}</li>
<li>${arr[3]}</li>
<li>${arr[4]}</li>
<li>${arr[5]}</li>
<li>${arr[6]}</li>
<li>${arr[7]}</li>
<li>${arr[8]}</li>
<li>${arr[9]}</li>
</ul>
</body>
</html>
5.2.3 集合
<%@ page import="java.util.ArrayList" %>
<%@ page import="com.qfedu.a_jsp.Student" %><%--
Created by IntelliJ IDEA.
User: Anonymous
Date: 2022/4/20
Time: 17:36
To change this template use File | Settings | File Templates.
--%>
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<html>
<head>
<title>Title</title>
</head>
<body>
<%
ArrayList<Student> list = new ArrayList<>();
list.add(new Student(1, "王乾", 80));
list.add(new Student(2, "张三", 80));
list.add(new Student(3, "李四", 80));
list.add(new Student(4, "王五", 80));
list.add(new Student(5, "赵六", 80));
request.setAttribute("list", list);
%>
<table width="200px" border="1" align="center">
<tr>
<th colspan="3">学生信息</th>
</tr>
<tr>
<th>ID</th>
<th>Name</th>
<th>Age</th>
</tr>
<%-- JSTL 表达式 for (Student stu : list)--%>
<c:if test="${not empty list}">
<c:forEach items="${list}" var="stu">
<tr>
<td>${stu.id}</td>
<td>${stu.name}</td>
<td>${stu.age}</td>
</tr>
</c:forEach>
</c:if>
</table>
</body>
</html>
5.2.4 Map 双边队列
<%@ page import="java.util.HashMap" %><%--
Created by IntelliJ IDEA.
User: Anonymous
Date: 2022/4/20
Time: 17:44
To change this template use File | Settings | File Templates.
--%>
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>05-el</title>
</head>
<body>
<%
HashMap<String, Object> map = new HashMap<>(3);
map.put("id", 1);
map.put("name", "张三");
map.put("age", 16);
request.setAttribute("map", map);
%>
<ul>
<%-- 方式一: . map key 对应名称 --%>
<li>${map.id}</li>
<li>${map.name}</li>
<li>${map.age}</li>
<%-- 方式二: map["key对应名称"] --%>
<li>${map["id"]}</li>
<li>${map["name"]}</li>
<li>${map["age"]}</li>
</ul>
<%--
JavaBean规范对象
List<JavaBean规范对象>
Object数组
List<Object数组>
Map<String, Object>
List<Map<String, Object>>
--%>
</body>
</html>
5.3 EL 运算符
算术运算符:
+ - * / %
关系运算符:
> < >= <= ! ==
逻辑运算符:
&& || !
【重点】
empty
5.4 EL 隐含对象
隐含对象 |
描述 |
pageScope |
指定从 page 域获取数据 |
requestScope |
指定从 request 域获取数据 |
sessionScope |
指定从 sesion 域获取数据 |
applicationScope |
指定从 application 域获取数据 |
param |
Request 对象的参数,字符串类型 |
paramValues |
Request 对象参数,字符串集合 |
initParam |
初始化参数集合 |
pageContext |
获取当前页面的 pageContext |
cookie |
Cookie值 |
<%--
Created by IntelliJ IDEA.
User: Anonymous
Date: 2022/4/20
Time: 19:21
To change this template use File | Settings | File Templates.
--%>
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>Title</title>
</head>
<body>
<%
request.setAttribute("msg", "Request Message");
session.setAttribute("msg", "Session Message");
application.setAttribute("msg", "Application Message");
pageContext.setAttribute("msg", "PageContext Message");
%>
<%--
如果出现同名情况下,可以利用 scope 限制从哪一个域对象中获取数据,不常用
在整个 JSP 页面解析数据的过程中,域对象存储属性名称不重复。
--%>
<h1>${pageScope.msg}</h1>
<h1>${requestScope.msg}</h1>
<h1>${sessionScope.msg}</h1>
<h1>${applicationScope.msg}</h1>
<hr>
<%--
cookie.JSESSIONID 获取 Cookie 名称为 JSESSIONID Cookie 对象
.value 直接获取对应的 cookie 存储数据
符合 JavaBean 对象都可以通过 . 成员变量名称获取对应数据
.value ==> getValue();
--%>
<h1>${cookie.JSESSIONID.value}</h1>
<hr>
<%-- 获取当前项目的虚拟路径根目录 ==> Application Context Path /Day43 --%>
<h1>${pageContext.request.contextPath}</h1>
</body>
</html>
6. JSTL 表达式【重点】
6.1 JSTL 导入
第三方 Jar 包
jstl-1.2.jar
需要在使用 JSTL 对应的 JSP 页面中引入
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%>
告知当前 JSP 页面,需要引入 JSTL 标签库,同时设置标签前缀为 c
6.2 解决的问题
配合 EL 表达式,更好的解析 Java 数据,可以利用的一些模版
if分支结构
<c:if></c:if>
choose分支结构
<c:choose>
<c:when></c:when>
<c:when></c:when>
<c:when></c:when>
</c:choose>
foreach循环结构
<c:foreach></c:foreach>
6.3 c:if
<%--
Created by IntelliJ IDEA.
User: Anonymous
Date: 2022/4/20
Time: 19:35
To change this template use File | Settings | File Templates.
--%>
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<html>
<head>
<title>JSTL if</title>
</head>
<body>
<%
request.setAttribute("num", 2);
%>
<%--
c:if JSTL 表达式 if 标签
必要属性
test
test 属性内容是需要判断条件,一般都是 EL 表达式
如果需要类似于 Java 的 if - else 分支结构,需要两个 c:if 配合进行条件拆解
--%>
<c:if test="${num > 5}">
<h1>成立</h1>
</c:if>
<c:if test="${num < 5}">
<h1>不成立</h1>
</c:if>
</body>
</html>
6.4 c:choose c:when
<%--
Created by IntelliJ IDEA.
User: Anonymous
Date: 2022/4/20
Time: 19:41
To change this template use File | Settings | File Templates.
--%>
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<html>
<head>
<title>02-chooseAndWhen</title>
</head>
<body>
<%
request.setAttribute("score", 59);
%>
<c:choose>
<%--
c:when 标签必须有一个 test 属性
test 属性值就是判断条件,EL 表达式操作
--%>
<c:when test="${score >= 90}"><h3>秀儿</h3></c:when>
<c:when test="${score >= 80}"><h3>良儿</h3></c:when>
<c:when test="${score >= 70}"><h3>中儿</h3></c:when>
<c:when test="${score >= 60}"><h3>过儿</h3></c:when>
<c:otherwise><h3>拖hai儿</h3></c:otherwise>
</c:choose>
</body>
</html>
6.5 c:foreach
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<%@ page import="java.util.ArrayList" %><%--
Created by IntelliJ IDEA.
User: Anonymous
Date: 2022/4/20
Time: 19:46
To change this template use File | Settings | File Templates.
--%>
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>03-jstl foreach</title>
</head>
<body>
<%
ArrayList<String> list = new ArrayList<>();
list.add("胡辣汤");
list.add("羊肉烩面");
list.add("牛肉汤");
list.add("羊肉汤");
list.add("驴肉汤");
request.setAttribute("list", list);
%>
<%--
重点属性
items 对应需要进行循环的集合,数组
var 用于在 c:forEach 循环中临时使用的变量,表示集合或者数组中一个数据
类似于 Java 中的 增强 for 循环
for (String s : list) {
}
了解
begin 从集合或者数组,第几个数据开始
end 限制到集合或者数组的哪一个数据解释
step 默认为 1 ,步进数据个数为 1,可以限制循环间隔
varStatus 当前循环对应的下标位置
--%>
<ul>
<c:forEach items="${list}" var="s" >
<li>${s}</li>
</c:forEach>
</ul>
</body>
</html>
7. Servlet 和 JSP 解析案例
package com.qfedu.a_jsp;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashMap;
@WebServlet("/test")
public class TestServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
HttpSession session = req.getSession();
session.setAttribute("username", "苟磊");
req.setAttribute("msg", "JSP页面你听好了!!!");
req.setAttribute("strArr", new String[]{"羊肉汤", "羊肉泡馍", "羊肉烩面", "烤羊排", "羊肉串"});
ArrayList<Student> studentList = new ArrayList<>();
studentList.add(new Student(1, "王乾不知天高地厚", 99));
studentList.add(new Student(2, "王乾不知火舞", 99));
studentList.add(new Student(3, "王乾不知深浅", 99));
req.setAttribute("studentList", studentList);
HashMap<String, Object> map = new HashMap<String, Object>(3);
map.put("王乾", 168);
map.put("河霖", 268);
map.put("玉玮", 368);
req.setAttribute("map", map);
req.getRequestDispatcher("06-JSTLAndEL.jsp").forward(req, resp);
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
doGet(req, resp);
}
}
<%--
Created by IntelliJ IDEA.
User: Anonymous
Date: 2022/4/20
Time: 19:53
To change this template use File | Settings | File Templates.
--%>
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<html>
<head>
<title>06-JSTLAndEL</title>
<style>
td {
text-align: center;
}
</style>
</head>
<body>
<h1>用户名: ${username}</h1>
<hr>
<h3>后端提交的数据信息:${msg}</h3>
<hr>
<h3>数组数据</h3>
<c:if test="${not empty strArr}">
<h4>我请你吃!!!</h4>
<c:forEach items="${strArr}" var="s">
<h4>${s}</h4>
</c:forEach>
</c:if>
<c:if test="${empty strArr}">
<h4>你坑我呢!!!</h4>
</c:if>
<hr>
<h3>集合数据</h3>
<table width="300" border="1" align="center">
<tr>
<th colspan="3">学生数据</th>
</tr>
<tr>
<th>Id</th>
<th>Name</th>
<th>Age</th>
</tr>
<c:if test="${not empty studentList}">
<c:forEach items="${studentList}" var="student">
<tr>
<td>${student.id}</td>
<td>${student.name}</td>
<td>${student.age}</td>
</tr>
</c:forEach>
</c:if>
<c:if test="${empty studentList}">
<tr>
<td colspan="3">查无数据</td>
</tr>
</c:if>
</table>
<hr>
<h3>Map数据</h3>
<table width="300" border="1" align="center">
<tr>
<th colspan="2">强哥梁山好汉会所</th>
</tr>
<tr>
<td>王乾</td>
<td>${map.王乾}</td>
</tr>
<tr>
<td>河霖</td>
<td>${map.河霖}</td>
</tr>
<tr>
<td>玉玮</td>
<td>${map.玉玮}</td>
</tr>
</table>
</body>
</html>
【推荐】国内首个AI IDE,深度理解中文开发场景,立即下载体验Trae
【推荐】编程新体验,更懂你的AI,立即体验豆包MarsCode编程助手
【推荐】抖音旗下AI助手豆包,你的智能百科全书,全免费不限次数
【推荐】轻量又高性能的 SSH 工具 IShell:AI 加持,快人一步