python基础 12. 元组
元组
什么是元组
元组(tuple)是Python内置的数据结构之一,是不可变序列
可变序列和不可变序列
不可变序列:字符串、元组(没有增删改的操作)
可变序列:列表、字典(可对序列执行增删改的操作,对象地址不发生改变)
元组的创建方式
1.直接小括号创建,这种方法的小括号可省略
2.使用内置函数tuple()创建
3.只包含一个元组的元素需要在元素后加,
# ----------元组----------
# 元组的创建
t = (1, 2, 4, 5) #
print(t, type(t), id(t))
t0 = 'java', 'c', 'python' #小括号可省
print(t0, type(t0), id(t0))
t1 = tuple(('java', 'c+', 'python'))
print(t1, type(t1), id(t1))
t = (1, )
print(t, type(t), id(t))
t1 = 2,
print(t1, type(t1), id(t1))
t = tuple()
print(t, type(t), id(t))
t2 = ()
print(t2, type(t2), id(t2))
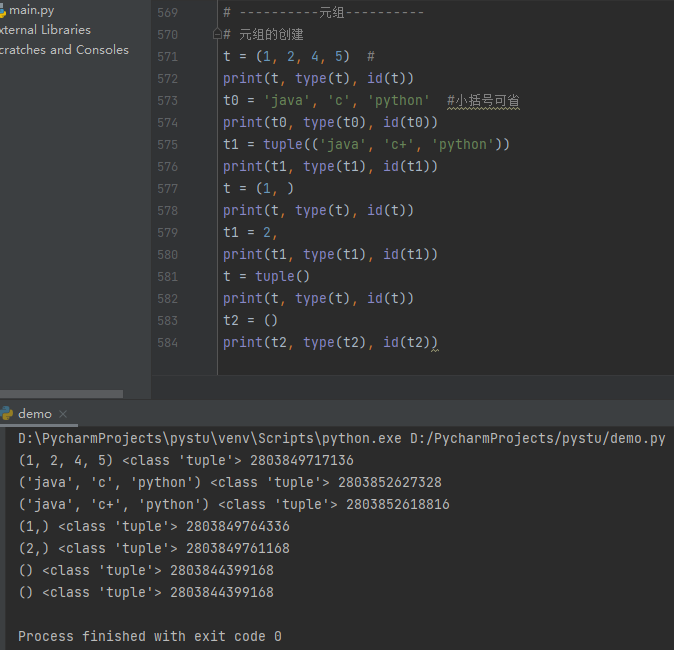
元组为什么是不可变序列
在多任务环境下,同时操作对象是不需要加锁因此在程序中尽量使用不可变序列
注意:元组中存储的是对象的引用
如果元组中对象本身是不可变对象,则不能引用其他对象
如果元组中对象时可变对象,则可变对象的引用不允许改变,但数据可以改变
t = (1, [2, 3], 4)
print(t)
print(t[1], type(t[1]))
# t[0] = 2
# print(t)
# 修改元组中的元素会出现以下错误:
# Traceback (most recent call last):
# File "D:\PycharmProjects\pystu\demo.py", line 593, in <module>
# t[0] = 2
# TypeError: 'tuple' object does not support item assignment
t[1].append(-111)
t[1].insert(1, 3)
t[1].sort()
t[1].remove(2)
print(t)
# 由于t[1]是列表,列表是可变序列,所以可对t[1]中的元素进行增、删、改的操作,但不可对t[1]本身进行修改
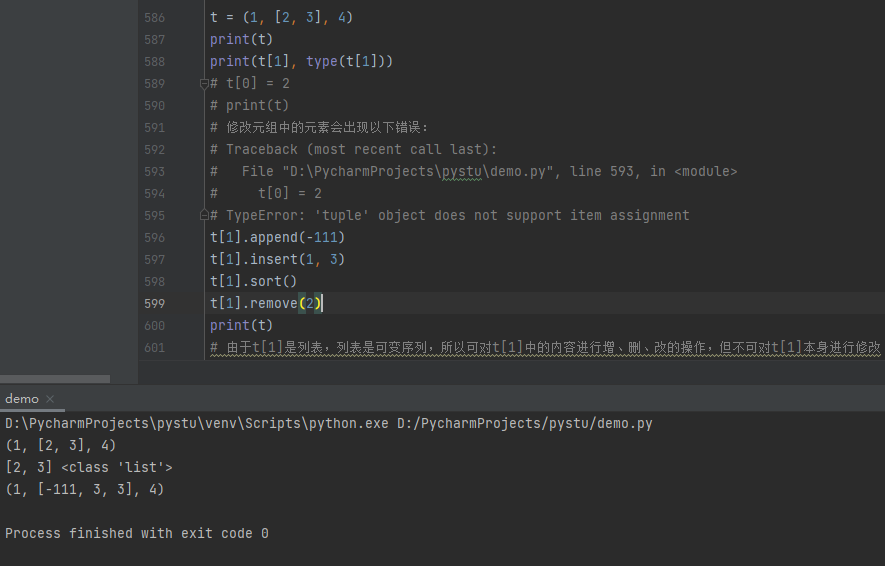
元组遍历
元组是可迭代对象,可以使用for..in进行遍历
# -----元组遍历-----
t = (1, [2, 3], 4)
print(t)
for i in t:
print(i, type(i), id(i))
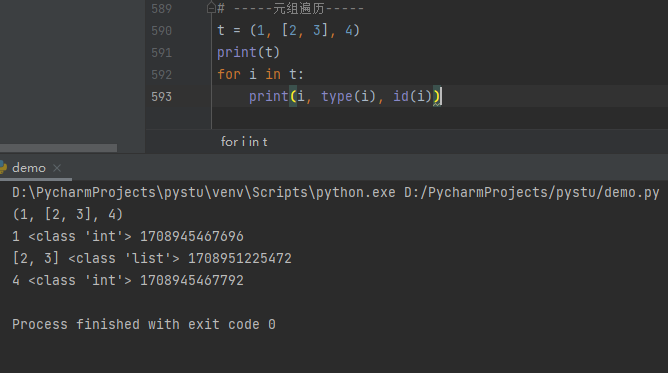