1 #include <iostream>
2 using namespace std;
3
4 class Box
5 {
6 double width;
7 public:
8 friend void printWidth( Box box ); //printWidth()为Box的友元
9 void setWidth( double wid );
10 };
11
12 // 成员函数定义
13 void Box::setWidth( double wid )
14 {
15 width = wid;
16 }
17
18 // 请注意:printWidth() 不是任何类的成员函数
19 void printWidth( Box box )
20 {
21 /* 因为 printWidth() 是 Box 的友元,它可以直接访问该类的任何成员 */
22 cout << "Width of box : " << box.width <<endl;
23 }
24
25 // 程序的主函数
26 int main( )
27 {
28 Box box;
29
30 // 使用成员函数设置宽度
31 box.setWidth(10.0);
32
33 // 使用友元函数输出宽度
34 printWidth( box );
35 system("pause");
36 return 0;
37 }
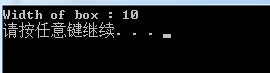