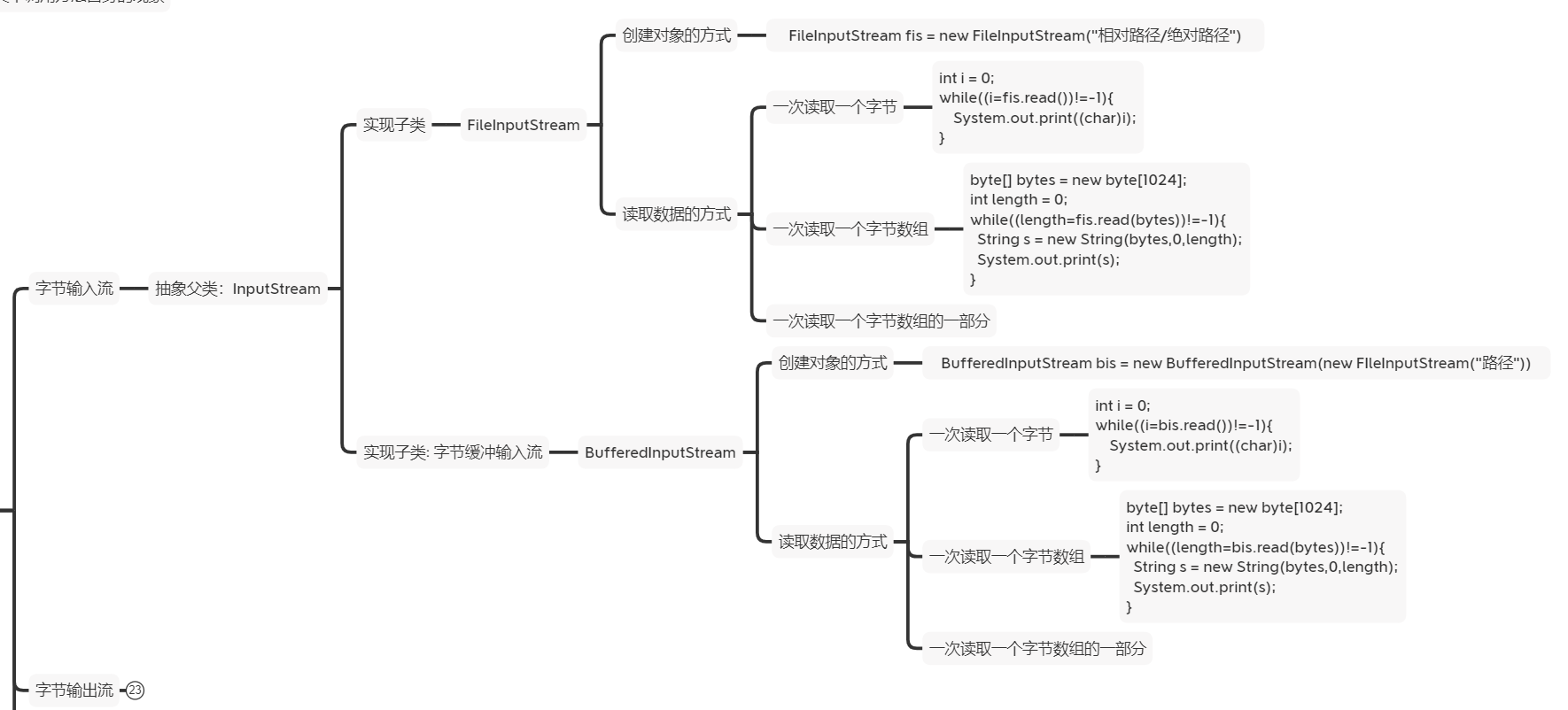
package com.shujia.day16.ketang;
import java.io.File;
import java.io.FileInputStream;
/*
字节输入流:FileInputStream
构造方法:
FileInputStream(File file) 通过打开与实际文件的连接创建一个 FileInputStream ,该文件由文件系统中的 File对象 file命名。
FileInputStream(String name) 通过打开与实际文件的连接来创建一个 FileInputStream ,该文件由文件系统中的路径名 name命名。
*/
public class FileInputStreamDemo1 {
public static void main(String[] args) throws Exception{
//FileInputStream(File file) 将要进行读取的文件封装成File对象放入到构造方法
//输入必须要求目标文件要已经存在,不然报错
// File file = new File("src/com/shujia/day16/data/a3.txt");
// FileInputStream fis = new FileInputStream(file);
//FileInputStream(String name)
FileInputStream fis = new FileInputStream("src/com/shujia/day16/data/a3.txt");
fis.close();
}
}
package com.shujia.day16.ketang;
import java.io.FileInputStream;
/*
FileInputStream读数据的方法:
public int read() 一次只读取一个字节
public int read(byte[] b) 读取一个数组,返回的是实际读取到的字节数
*/
public class FileInputStreamDemo2 {
public static void main(String[] args) throws Exception {
//创建字节输入流对象
FileInputStream fis = new FileInputStream("src/com/shujia/day16/data/a3.txt");
//public int read() // 一次只读取一个字节
// System.out.println((char) fis.read());
// System.out.println((char) fis.read());
// System.out.println((char) fis.read());
// System.out.println((char) fis.read());
// System.out.println(fis.read());
// int i = 0;
// while ((i = fis.read()) != -1) {
// System.out.print((char) i);
// }
//public int read(byte[] b)
//创建一个字节数组
// byte[] bytes = new byte[1024];
// int length = fis.read(bytes); // 返回的是实际读取到的字节数
// //字节数组转字符串
// String s = new String(bytes,0,length);
// System.out.println(s);
// byte[] bytes = new byte[2];
// //第一次读
// int length = fis.read(bytes); // 返回的是实际读取到的字节数
// //字节数组转字符串
// String s = new String(bytes,0,length);
// System.out.print(s);
//
// //第二次读
// int length2 = fis.read(bytes); // 返回的是实际读取到的字节数
// //字节数组转字符串
// String s2 = new String(bytes,0,length2);
// System.out.print(s2);
// //第三次读
// int length3 = fis.read(bytes); // 返回的是实际读取到的字节数
// //字节数组转字符串
// String s3 = new String(bytes,0,length3);
// System.out.print(s3);
// //第四次读
// int length4 = fis.read(bytes); // 返回的是实际读取到的字节数
// //字节数组转字符串
// String s4 = new String(bytes,0,length4);
// System.out.print(s4);
//
// //第五次读
// int length5 = fis.read(bytes); // 返回的是实际读取到的字节数
// //字节数组转字符串
// String s5 = new String(bytes,0,length5);
// System.out.print(s5);
//
// //第六次读
// int length6 = fis.read(bytes); // 返回的是实际读取到的字节数
// //字节数组转字符串
// String s6 = new String(bytes,0,length6);
// System.out.print(s6);
//使用while循环读取
byte[] bytes = new byte[1024];
int length = 0;
while ((length = fis.read(bytes)) != -1) {
String s = new String(bytes, 0, length);
System.out.print(s);
}
//释放资源
fis.close();
}
}