<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<script src="../js/vue.js"></script>
</head>
<body>
<div id="app">
<input type="text" v-model="inputValue">
<button v-on:click="handleBtnClick">提交</button>
<ul>
<li v-for="item in list">{{item}}</li>
</ul>
</div>
<script>
var app = new Vue({
el:'#app',
data:{
list:[],
inputValue:''
},
methods:{
handleBtnClick(){
this.list.push(this.inputValue)
this.inputValue=''
}
}
})
</script>
</body>
</html>
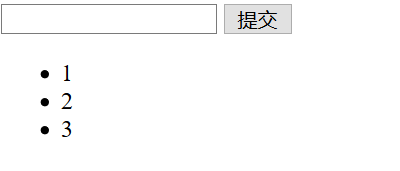
子组件给父组件传值
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<script src="../js/vue.js"></script>
</head>
<body>
<div id="app">
<div>
<input type="text" v-model="todoValue"/>
<button @click="handleBtnClick">提交</button>
<ul>
<todo-item v-bind:content="item" v-for="item in list">
</todo-item>
</ul>
</div>
</div>
</body>
<script>
var TodoItem={
props:['content'],
template:"<li>{{content}}</li>"
}
new Vue({
el:'#app',
components:{
TodoItem:TodoItem,
},
data:{
todoValue:"",
list:[]
},
methods:{
handleBtnClick(){
this.list.push(this.todoValue)
this.todoValue=''
}
}
})
</script>
</html>
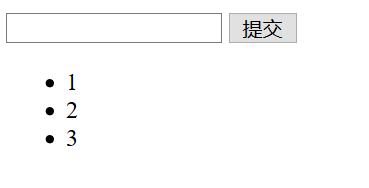
删除功能——父组件给子组件传值
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<script src="../js/vue.js"></script>
</head>
<body>
<div id="app">
<div>
<input type="text" v-model="todoValue"/>
<button @click="handleBtnClick">提交</button>
<ul>
<todo-item v-bind:content="item"
v-bind:index="index"
v-for="(item,index) in list"
@delete="handleItemDelete">
</todo-item>
</ul>
</div>
</div>
</body>
<script>
var TodoItem={
props:['content','index'],
template:"<li @click='handleItemClick'>{{content}}</li>",
methods: {
handleItemClick:function () {
this.$emit("delete",this.index);
}
}
}
new Vue({
el:'#app',
components:{
TodoItem:TodoItem,
},
data:{
todoValue:"",
list:[]
},
methods:{
handleBtnClick(){
this.list.push(this.todoValue)
this.todoValue=''
},
handleItemDelete:function(index){
this.list.splice(index,1)
}
}
})
</script>
</html>
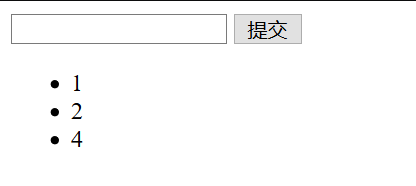